How to Calculate GPS Distance Using JavaScript and MySQL: A Comprehensive Guide for Developers
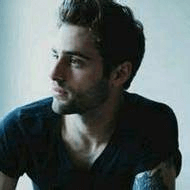
In today's digital landscape, calculating GPS distance is essential for various applications, including navigation systems and location-based services. This comprehensive guide will walk you through the process of calculating GPS distances using JavaScript and MySQL, while also integrating AI-driven database management with Chat2DB (opens in a new tab).
The Importance of GPS in Application Development
Global Positioning System (GPS) is a satellite-based navigation system that allows users to determine their exact location (latitude and longitude) anywhere on Earth. GPS plays a critical role in numerous applications, including mapping, navigation, and location-based services.
Key Terms Related to GPS Technology
- Latitude: Indicates how far north or south a location is from the equator.
- Longitude: Indicates how far east or west a location is from the Prime Meridian.
- Geolocation: The identification of the geographic location of a person or device.
Understanding these terms is crucial for developers working with GPS data.
The Impact of GPS Technology
GPS technology has transformed various industries. Precise GPS calculations enhance navigation systems, improve delivery services, and facilitate location-based applications. The ability to accurately calculate distances between GPS coordinates is vital for effective operations.
Challenges in GPS Technology
Despite its advantages, GPS technology faces challenges such as signal interference and accuracy issues that can affect distance calculations. Developers must be aware of these limitations to create robust applications.
Setting Up Your Development Environment for GPS Distance Calculation
To effectively calculate GPS distances using JavaScript and MySQL, you need to set up a suitable development environment. Here’s a step-by-step guide to get started.
Required Tools
- Node.js: A JavaScript runtime for building server-side applications.
- MySQL: A relational database management system.
- Visual Studio Code: A popular IDE for JavaScript development.
Step-by-Step Installation Guide
- Install Node.js: Download and install Node.js from the official website (opens in a new tab).
- Install MySQL: Set up MySQL Server by downloading it from the MySQL website (opens in a new tab).
- Set Up Visual Studio Code: Download and install Visual Studio Code from here (opens in a new tab).
- Use npm: Utilize Node Package Manager (npm) to manage your JavaScript libraries. To initialize a new project, run:
npm init -y
- Version Control with Git: Install Git from the official site (opens in a new tab) and set up a repository for collaborative development.
Starting a Local Server
You can use XAMPP or WAMP to set up a local server environment. This will allow you to run your MySQL database and JavaScript code seamlessly.
Calculating GPS Distance in JavaScript
In this section, we will delve into the mathematical formulas used to calculate distances between two GPS coordinates in JavaScript.
The Haversine Formula Explained
The Haversine formula is widely used for calculating the distance between two points on the Earth’s surface, taking into account the spherical shape of the planet.
Haversine Formula Implementation in JavaScript
Here’s how to implement the Haversine formula in JavaScript:
function haversineDistance(coord1, coord2) {
const R = 6371; // Radius of the Earth in kilometers
const dLat = (coord2.lat - coord1.lat) * Math.PI / 180;
const dLon = (coord2.lon - coord1.lon) * Math.PI / 180;
const a = Math.sin(dLat / 2) * Math.sin(dLat / 2) +
Math.cos(coord1.lat * Math.PI / 180) * Math.cos(coord2.lat * Math.PI / 180) *
Math.sin(dLon / 2) * Math.sin(dLon / 2);
const c = 2 * Math.atan2(Math.sqrt(a), Math.sqrt(1 - a));
return R * c; // Distance in kilometers
}
// Example usage
const pointA = { lat: 34.0522, lon: -118.2437 }; // Los Angeles
const pointB = { lat: 40.7128, lon: -74.0060 }; // New York City
console.log(`Distance: ${haversineDistance(pointA, pointB).toFixed(2)} km`); // Outputs distance
Choosing the Right Formula for GPS Distance Calculation
While the Haversine formula is commonly used, Vincenty's formulae may provide more accuracy for longer distances. Depending on your application's requirements, you may choose the most appropriate formula.
Handling Different Units
You can easily modify the above function to return the distance in miles by changing the radius constant R:
function haversineDistanceInMiles(coord1, coord2) {
const R = 3958.8; // Radius of the Earth in miles
// The rest of the function remains the same
}
Optimizing for Large Datasets
When dealing with large datasets, optimizations such as caching results or utilizing spatial indexes in MySQL can greatly improve performance.
Integrating MySQL for Storing and Querying GPS Data
Using MySQL to manage GPS data is an effective strategy for ensuring quick access and manipulation of large datasets.
Database Structure Example
A simple MySQL table structure for storing GPS coordinates can be as follows:
CREATE TABLE locations (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL,
latitude DECIMAL(9,6) NOT NULL,
longitude DECIMAL(9,6) NOT NULL
);
Querying GPS Data with SQL
You can write SQL queries to retrieve GPS coordinates and calculate distances. Here’s an example of using SQL to find nearby locations:
SELECT name, latitude, longitude,
(6371 * ACOS(COS(RADIANS(:lat)) * COS(RADIANS(latitude)) * COS(RADIANS(longitude) - RADIANS(:lon)) + SIN(RADIANS(:lat)) * SIN(RADIANS(latitude)))) AS distance
FROM locations
HAVING distance < :distance
ORDER BY distance;
Integrating MySQL with JavaScript
You can use libraries like Sequelize or MySQL2 to connect your JavaScript application with MySQL. Here’s an example using MySQL2:
const mysql = require('mysql2');
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
database: 'gps_db',
password: 'your_password'
});
connection.query('SELECT * FROM locations', (err, results) => {
if (err) throw err;
console.log(results);
});
Building a GPS Distance Calculation Application
Now, let’s build a simple application that calculates GPS distances using JavaScript and MySQL.
Project Overview
This application will allow users to input two locations and receive the distance between them.
User Interface Design
Design a simple HTML form for users to input coordinates:
<form id="distance-form">
<input type="text" id="lat1" placeholder="Latitude 1" required>
<input type="text" id="lon1" placeholder="Longitude 1" required>
<input type="text" id="lat2" placeholder="Latitude 2" required>
<input type="text" id="lon2" placeholder="Longitude 2" required>
<button type="submit">Calculate Distance</button>
</form>
<div id="result"></div>
Backend Logic for Distance Calculation
Handle form submissions and calculate the distance:
document.getElementById('distance-form').addEventListener('submit', function(e) {
e.preventDefault();
const lat1 = parseFloat(document.getElementById('lat1').value);
const lon1 = parseFloat(document.getElementById('lon1').value);
const lat2 = parseFloat(document.getElementById('lat2').value);
const lon2 = parseFloat(document.getElementById('lon2').value);
const distance = haversineDistance({lat: lat1, lon: lon1}, {lat: lat2, lon: lon2});
document.getElementById('result').innerText = `Distance: ${distance.toFixed(2)} km`;
});
Testing and Debugging Your Application
Regularly test your application to ensure accuracy. Use tools like Postman for API testing and Chrome DevTools for debugging.
Leveraging Chat2DB for Enhanced Database Management
To streamline your database management, consider using Chat2DB (opens in a new tab). This AI-powered database visualization management tool simplifies complex SQL queries and enhances your workflow.
Features Offered by Chat2DB
- Natural Language SQL Generation: Effortlessly create SQL queries using natural language.
- Smart SQL Editor: Enhance your coding efficiency with intelligent code suggestions.
- Data Analysis: Quickly generate visual data representations for better insights.
Integrating Chat2DB into your workflow can significantly improve productivity and efficiency when managing GPS data.
Exploring Advanced Topics and Continuous Learning
As you advance your understanding of GPS and database management, consider delving into advanced topics such as machine learning with GPS data. Continuous learning is essential to keep up with technological advancements.
Recommended Resources for Further Learning
- Books on GPS technology and database management.
- Online courses focusing on GIS and spatial data analysis.
- Developer communities and forums for knowledge exchange.
Stay updated by participating in industry conferences focused on GPS technology and consider pursuing certifications in GIS.
By leveraging tools like Chat2DB (opens in a new tab), you can enhance your database management and improve your development workflow. Embrace these technologies to build innovative applications that harness the power of GPS data.
Frequently Asked Questions (FAQ)
-
What is the Haversine formula?
- The Haversine formula calculates the distance between two points on the Earth's surface based on their latitude and longitude.
-
How can I store GPS data in MySQL?
- You can create a table with latitude and longitude fields in MySQL to store GPS coordinates.
-
What are some common applications of GPS technology?
- GPS technology is commonly used in mapping, navigation, fleet management, and location-based services.
-
How can I optimize distance calculations for large datasets?
- Consider using caching, spatial indexes, or optimizing your SQL queries for better performance.
-
What advantages does Chat2DB offer for database management?
- Chat2DB provides AI-driven features for natural language SQL generation, smart code editing, and visual data analysis, enhancing productivity and efficiency.
By following this guide, you will be well-equipped to calculate GPS distances using JavaScript and MySQL effectively. Don't forget to explore the powerful features of Chat2DB (opens in a new tab) to further streamline your database management tasks.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!