How to Execute Common SQL Commands with PSQL: A Comprehensive Guide with Examples
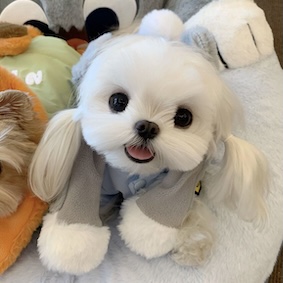
Executing SQL commands effectively is essential for efficient database management, and using PSQL (PostgreSQL interactive terminal) is a powerful method to achieve this. This guide will cover the basics of using PSQL, setting up your environment, navigating the interface, and executing both basic and advanced SQL commands. We will also introduce you to Chat2DB, an AI-driven tool that enhances your SQL command execution experience. This article provides an in-depth understanding of PSQL commands with practical examples to help you manage your databases efficiently.
Understanding PSQL and Its Role in SQL Command Execution
PSQL is the command-line interface for interacting with PostgreSQL databases. It simplifies database management by allowing users to execute SQL commands directly, manage database objects, and automate tasks through scripting. PSQL's integration with PostgreSQL makes it an indispensable tool for developers and database administrators alike. It enables efficient querying of databases and management of schemas, which is crucial for maintaining data integrity and performance.
Benefits of Using PSQL
Benefit | Description |
---|---|
Direct Command Execution | Run SQL commands without needing a graphical interface. |
Scripting and Automation | Write scripts to automate repetitive tasks, enhancing productivity. |
Compatibility | Works seamlessly with PostgreSQL, making it a reliable choice for database management. |
To begin using PSQL, ensure you have it installed and configured properly.
Setting Up Your Environment for PSQL
Setting up PSQL is straightforward. Here’s a step-by-step guide to get you started:
-
Prerequisites: Ensure your system meets the requirements for installing PostgreSQL, including compatible operating systems like Windows, macOS, or Linux.
-
Download PostgreSQL: Visit the official PostgreSQL website (opens in a new tab) to download the installer for your operating system.
-
Installation:
- Windows: Run the installer and follow the prompts to install PostgreSQL, which includes PSQL.
- macOS: Use Homebrew with the command
brew install postgresql
or download the installer from the website. - Linux: Use your package manager, e.g.,
sudo apt-get install postgresql
for Debian-based systems.
-
Environment Variables: After installation, ensure that the PostgreSQL bin directory is added to your system’s PATH variable to run PSQL commands from any command prompt.
-
Configuration: Modify the
postgresql.conf
file for performance optimization, such as increasing shared buffers and setting the appropriate work memory. -
Troubleshooting: If you encounter issues, consult the PostgreSQL documentation (opens in a new tab) for troubleshooting tips.
By following these steps, you will have PSQL ready for executing SQL commands.
Navigating the PSQL Interface
Once PSQL is installed, you can start navigating its interface. Here’s how to effectively use PSQL:
-
Starting PSQL: Open your command line and type
psql -U username -d database_name
to connect to your PostgreSQL database. Replaceusername
with your database user anddatabase_name
with the name of your database. -
Understanding the Command Prompt: The PSQL prompt displays the current database you are connected to, indicating that you can start executing SQL commands.
-
Modes of Operation: PSQL operates in two primary modes:
- Interactive Mode: Ideal for executing single commands and immediate feedback.
- Script Mode: Use this mode to run a batch of commands saved in a
.sql
file using the command\i filename.sql
.
-
Help System: Access built-in help by typing
\?
for command help or\h
for SQL command help. -
Switching Databases: To connect to a different database, use the command
\c database_name
.
Here’s a simple command to create a new database:
CREATE DATABASE my_database;
You can then connect to it using:
\c my_database
Executing Basic SQL Commands with PSQL
Now that you are familiar with the PSQL interface, let’s dive into executing basic SQL commands. Here are some commonly used SQL commands along with practical examples:
-
Creating Tables: To create a new table in your database, use the following command:
CREATE TABLE employees ( id SERIAL PRIMARY KEY, name VARCHAR(100), position VARCHAR(100), salary NUMERIC );
-
Inserting Records: To insert data into the
employees
table, execute:INSERT INTO employees (name, position, salary) VALUES ('John Doe', 'Software Engineer', 60000);
-
Updating Records: To update an employee's salary, use:
UPDATE employees SET salary = 65000 WHERE name = 'John Doe';
-
Deleting Records: To delete an employee record:
DELETE FROM employees WHERE name = 'John Doe';
-
Selecting Records: To retrieve data from the
employees
table, use theSELECT
statement:SELECT * FROM employees;
You can filter results with a
WHERE
clause:SELECT * FROM employees WHERE position = 'Software Engineer';
To sort the results, you can use:
SELECT * FROM employees ORDER BY salary DESC;
Example of Using JOIN Clauses
When working with multiple tables, you might need to combine data using JOIN clauses. Here’s a quick example:
CREATE TABLE departments (
id SERIAL PRIMARY KEY,
department_name VARCHAR(100)
);
INSERT INTO departments (department_name) VALUES ('Engineering');
SELECT e.name, d.department_name
FROM employees e
JOIN departments d ON e.department_id = d.id;
This SQL command will return a list of employee names along with their respective department names.
Advanced SQL Techniques in PSQL
Once you're comfortable with basic commands, you can explore advanced SQL techniques. Here are some powerful features you can use:
-
Subqueries: Use subqueries to perform complex queries:
SELECT name FROM employees WHERE salary > (SELECT AVG(salary) FROM employees);
-
Common Table Expressions (CTEs): Simplify complex SQL queries with CTEs:
WITH HighEarners AS ( SELECT name, salary FROM employees WHERE salary > 60000 ) SELECT * FROM HighEarners;
-
Transaction Management: Ensure data consistency with transactions:
BEGIN; INSERT INTO employees (name, position, salary) VALUES ('Jane Doe', 'Manager', 75000); UPDATE employees SET salary = 70000 WHERE name = 'John Doe'; COMMIT;
-
Indexes: Improve query performance by creating indexes:
CREATE INDEX idx_employee_name ON employees (name);
-
Stored Procedures and Functions: Automate repetitive tasks with stored procedures:
CREATE FUNCTION increase_salary(emp_name VARCHAR, increment NUMERIC) RETURNS VOID AS $$ BEGIN UPDATE employees SET salary = salary + increment WHERE name = emp_name; END; $$ LANGUAGE plpgsql;
Using Execution Plans for Query Optimization
To analyze and optimize your queries, you can use the EXPLAIN
command:
EXPLAIN SELECT * FROM employees WHERE salary > 60000;
This will provide insights into how PostgreSQL plans to execute the query, helping you identify potential performance issues.
Leveraging Chat2DB for Enhanced SQL Command Execution
Chat2DB is an innovative AI-driven database management tool designed to enhance your SQL command execution experience. By integrating with PSQL and PostgreSQL, it offers an intuitive interface that simplifies database management tasks significantly.
Features of Chat2DB
-
Natural Language Processing: Chat2DB allows users to generate SQL queries using natural language commands, making it easier for those new to SQL.
-
Visual Query Builders: Users can build complex queries through a visual interface, eliminating the need for extensive SQL knowledge.
-
Real-time Query Execution: Execute queries and receive instant feedback, allowing for quick iterations and debugging.
-
Schema Visualization: Easily view and manage your database schemas, simplifying the understanding of relationships and data structures.
-
Collaboration Tools: Teams can work together seamlessly on database projects, enhancing productivity.
By leveraging the AI capabilities of Chat2DB, users can streamline their SQL workflows and enhance their productivity significantly. Compared to traditional tools like DBeaver, MySQL Workbench, and DataGrip, Chat2DB offers a more user-friendly and intuitive experience, making it the optimal choice for database management.
Optimizing Your Workflow with PSQL and Chat2DB
Combining PSQL with Chat2DB can significantly optimize your workflow. Here are some strategies to enhance your experience:
-
Automation Scripts: Use PSQL to create scripts that automate repetitive tasks, reducing manual effort and minimizing errors.
-
Configuration Management: Store configuration files tailored to specific projects, which can be loaded into PSQL as needed.
-
Database Migration Management: Use PSQL in conjunction with Chat2DB for effective database migrations, ensuring smooth transitions between versions.
-
Performance Monitoring: Utilize logging and monitoring tools within Chat2DB to maintain database performance and security.
-
Regular Backups: Implement a backup strategy to prevent data loss, utilizing both PSQL commands and Chat2DB features.
By applying these strategies, you will ensure that your database management practices are efficient and effective.
Frequently Asked Questions (FAQ)
-
What is PSQL? PSQL is the command-line interface for PostgreSQL that allows users to execute SQL commands and manage databases efficiently.
-
How do I install PSQL? You can install PSQL by downloading PostgreSQL from the official website (opens in a new tab) and following the installation instructions for your operating system.
-
Can I use Chat2DB with PSQL? Yes, Chat2DB integrates with PSQL and enhances your SQL command execution experience with AI features and intuitive tools.
-
What are the benefits of using Chat2DB? Chat2DB provides natural language query generation, visual query builders, real-time execution, and collaboration features that improve productivity in database management.
-
How do I optimize my SQL commands? You can optimize SQL commands by using indexes, analyzing execution plans with the
EXPLAIN
command, and leveraging advanced techniques like CTEs and subqueries.
For more information and to experience the benefits of streamlined database management, visit Chat2DB (opens in a new tab) and explore how its AI functionalities can enhance your SQL command execution.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!