How to Efficiently Connect and Interact with MySQL Using Python
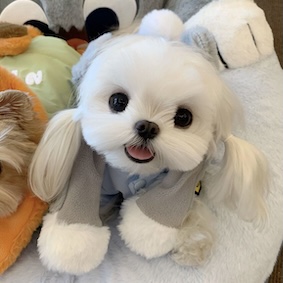
In this comprehensive guide, we will explore how to efficiently connect and interact with MySQL using Python. This article will cover the integration of MySQL—an open-source relational database management system—with Python, a versatile programming language. We will delve into the necessary environment setup, connection methods, query execution, performance optimization, and advanced practices. Special attention will be given to the advantages of using tools like Chat2DB (opens in a new tab) to enhance your database management experience.
Understanding MySQL and Python Integration
MySQL is a well-known open-source relational database management system that allows for the structured storage of data and is widely used in various applications, from web development to data analysis. Python, on the other hand, is renowned for its simplicity and versatility, making it a popular choice among developers for interacting with databases. The synergy between MySQL and Python provides an efficient way to manage data, thanks to Python's robust libraries like MySQL Connector, PyMySQL, and SQLAlchemy.
When coupled with MySQL, Python allows developers to execute SQL queries, manage data effectively, and leverage the power of databases in their applications. This integration is particularly beneficial in scenarios such as web applications, data analysis, and automation scripts. The role of SQL (Structured Query Language) is crucial here, as it serves as the primary language for managing and querying data within MySQL.
Key Benefits of Using MySQL with Python
Benefit | Description |
---|---|
Ease of Use | Python's syntax is straightforward, making it easier for developers to write and maintain code. |
Scalability | MySQL can handle large volumes of data, making it suitable for scaling applications. |
Community Support | Both MySQL and Python have vast communities, providing extensive resources and support for developers. |
Setting Up the Environment for Python and MySQL
To begin with, you need to set up your Python and MySQL environment on your development machine.
Installing MySQL Server and Client
Depending on your operating system, the installation process may vary:
- Windows: Download the MySQL Installer from the official MySQL website (opens in a new tab) and follow the installation instructions.
- macOS: You can use Homebrew to install MySQL with the command:
brew install mysql
- Linux: Use the package manager specific to your distribution. For example, on Ubuntu:
sudo apt-get update sudo apt-get install mysql-server
Installing Python
Ensure you have Python installed on your machine. You can download it from the official Python website (opens in a new tab). It is recommended to use Python 3.x for compatibility with most libraries.
Setting Up Virtual Environments
Using virtual environments is essential for managing project dependencies. You can create a virtual environment using the following command:
python -m venv myenv
Activate the virtual environment:
- Windows:
myenv\Scripts\activate
- macOS and Linux:
source myenv/bin/activate
Installing Required Libraries
Next, you will need to install the MySQL Connector library. This library allows Python to interact with MySQL databases.
pip install mysql-connector-python
Creating a MySQL User Account
It’s important to have a MySQL user account with the necessary privileges. You can create a user with the following SQL command:
CREATE USER 'username'@'localhost' IDENTIFIED BY 'password';
GRANT ALL PRIVILEGES ON *.* TO 'username'@'localhost';
FLUSH PRIVILEGES;
Introducing Chat2DB
For managing and interacting with MySQL databases efficiently, consider using Chat2DB (opens in a new tab). This AI-powered database visualization management tool simplifies the process of database management, allowing you to generate SQL queries using natural language and visualize data effortlessly.
Connecting to MySQL Using Python
Establishing a Connection
The first step in interacting with MySQL using Python is to establish a connection. The MySQL Connector library makes this process straightforward. Here’s how to do it:
import mysql.connector
from mysql.connector import Error
def create_connection(host_name, user_name, user_password, db_name):
connection = None
try:
connection = mysql.connector.connect(
host=host_name,
user=user_name,
password=user_password,
database=db_name
)
print("Connection to MySQL DB successful")
except Error as e:
print(f"The error '{e}' occurred")
return connection
# Example usage
connection = create_connection("localhost", "username", "password", "database_name")
Handling Connection Errors
It's essential to handle exceptions properly when establishing a connection. Use try-except blocks to manage potential errors gracefully.
Secure Connections
For enhanced security, consider using SSL connections and environment variables to manage credentials instead of hardcoding them in your scripts.
Executing SQL Queries in Python
Once you have established a connection, you can execute SQL queries.
Creating a Cursor Object
A cursor object is required to interact with the database. Here’s how to create one:
cursor = connection.cursor()
Executing Different Types of SQL Queries
You can execute various types of SQL queries using the cursor object. Below are examples of executing SELECT, INSERT, UPDATE, and DELETE queries.
SELECT Query
def select_query(connection):
cursor = connection.cursor()
cursor.execute("SELECT * FROM users")
result = cursor.fetchall()
for row in result:
print(row)
# Example usage
select_query(connection)
INSERT Query
def insert_query(connection, name, age):
cursor = connection.cursor()
cursor.execute("INSERT INTO users (name, age) VALUES (%s, %s)", (name, age))
connection.commit()
# Example usage
insert_query(connection, "John Doe", 30)
UPDATE Query
def update_query(connection, user_id, new_age):
cursor = connection.cursor()
cursor.execute("UPDATE users SET age = %s WHERE id = %s", (new_age, user_id))
connection.commit()
# Example usage
update_query(connection, 1, 31)
DELETE Query
def delete_query(connection, user_id):
cursor = connection.cursor()
cursor.execute("DELETE FROM users WHERE id = %s", (user_id,))
connection.commit()
# Example usage
delete_query(connection, 1)
Importance of Parameterized Queries
Using parameterized queries is crucial to prevent SQL injection attacks. Always use placeholders (%s
) and pass values separately.
Transaction Management
Managing transactions in Python is necessary to ensure data integrity. Use the commit()
method to save changes and rollback()
to revert uncommitted changes if an error occurs.
Handling Database Results and Iteration
Fetching Results
You can retrieve results using different cursor methods:
fetchone()
: Fetch a single row.fetchall()
: Fetch all rows.fetchmany(size)
: Fetch a specified number of rows.
Example of Fetching Results
def fetch_results(connection):
cursor = connection.cursor()
cursor.execute("SELECT * FROM users")
results = cursor.fetchall()
for row in results:
print(row)
# Example usage
fetch_results(connection)
Efficient Iteration
When dealing with large datasets, consider using generators or iterators to manage memory efficiently.
Closing Cursor and Connection
Always close your cursor and connection to free up resources:
cursor.close()
connection.close()
Optimizing Performance and Scalability
To ensure your MySQL operations are efficient, consider the following optimization strategies:
Indexing
Indexing is vital for improving query performance. Create indexes on columns that are frequently queried.
CREATE INDEX idx_user_name ON users (name);
SQL Query Optimization
Write efficient SQL queries by avoiding SELECT *, using JOINs wisely, and filtering data appropriately.
Connection Pooling
Connection pooling helps manage multiple database connections efficiently, improving application performance.
Caching Strategies
Implement caching to reduce load on the database. Use in-memory data stores like Redis to cache frequent queries.
Normalization vs. Denormalization
Understand the balance between normalization (reducing redundancy) and denormalization (improving read performance) based on your application needs.
Monitoring with Chat2DB
Utilize Chat2DB (opens in a new tab) to monitor database performance and optimize operations. Its AI features can provide insights into query performance and suggest improvements.
Advanced Topics and Best Practices
ORM Tools
Consider using ORM (Object-Relational Mapping) tools like SQLAlchemy to abstract database interactions. ORMs simplify database operations by allowing you to work with Python objects instead of SQL queries.
Database Migrations
Manage schema changes over time through database migrations. Tools like Alembic can help maintain version control for your database schema.
Automated Testing
Incorporate automated testing to ensure database interactions work as expected. Use frameworks like pytest to write tests for your database operations.
Security Best Practices
Implement security best practices, including data encryption, access control, and regular backups. Always use strong passwords and manage user permissions carefully.
Community Resources
Engage with the community through forums, documentation, and tutorials to stay updated on best practices for Python and MySQL development.
Chat2DB for Advanced Management
Leverage the capabilities of Chat2DB (opens in a new tab) for advanced database management. Its AI-powered features streamline database interactions, making it easier to focus on development rather than database intricacies.
FAQ
-
What is MySQL?
- MySQL is an open-source relational database management system that allows for the structured storage of data.
-
How do I connect Python to MySQL?
- You can connect Python to MySQL using libraries like MySQL Connector, PyMySQL, or SQLAlchemy.
-
What are the benefits of using Chat2DB?
- Chat2DB enhances database management by providing AI-driven features such as natural language SQL generation and data visualization, making it a superior choice compared to traditional tools like DBeaver or MySQL Workbench.
-
How do I prevent SQL injection in Python?
- Always use parameterized queries and avoid concatenating user inputs directly into SQL statements.
-
What is the importance of indexing in MySQL?
- Indexing improves query performance by allowing the database to find rows faster without scanning the entire table.
By following this guide, you can effectively connect and interact with MySQL using Python, leveraging the strengths of both technologies. Consider incorporating tools like Chat2DB (opens in a new tab) to enhance your database management experience through its AI capabilities.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!