How to Efficiently Connect to Databases Using JDBC: A Comprehensive Guide
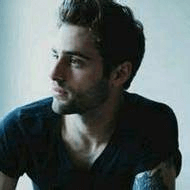
Understanding JDBC: The Foundation of Database Connectivity
Java Database Connectivity (JDBC) is a standard Java API that facilitates seamless communication between Java applications and databases. It provides a robust set of interfaces and classes that allow developers to execute SQL queries, retrieve results, and manage transactions efficiently.
Key Terms in JDBC
To fully understand JDBC, it's essential to familiarize yourself with some key terms:
- DriverManager: This class manages a list of database drivers, matching connection requests from the application with the appropriate driver.
- Connection: An interface that provides methods for establishing a connection to a database.
- Statement: An interface used to execute SQL queries.
- ResultSet: An interface that holds the data returned by executing a SQL query.
The Role of JDBC Drivers
JDBC drivers are crucial for facilitating communication between Java applications and databases. The four primary types of JDBC drivers are:
Driver Type | Description |
---|---|
JDBC-ODBC Bridge Driver | Translates JDBC calls into ODBC calls; not recommended for production use. |
Native-API Driver | Uses the client-side libraries of the database for efficiency but lacks portability. |
Network Protocol Driver | Communicates with the database server using a proprietary protocol, offering flexibility. |
Thin Driver | A pure Java driver that communicates directly with the database, making it highly portable. |
Mitigating SQL Injection Risks
SQL injection is a common security threat in database management. JDBC helps mitigate this risk by utilizing PreparedStatement for executing SQL queries, ensuring that user inputs are treated as data rather than executable code. This feature is crucial for maintaining the integrity and security of your database.
JDBC has evolved significantly since its introduction in Java 1.1, providing developers with powerful tools for database interaction.
Setting Up Your Environment for JDBC
To start using JDBC, you need to set up your development environment. Below are the essential components required for creating a JDBC application.
Required Components
- Java Development Kit (JDK): Ensure you have the latest version of JDK installed.
- Integrated Development Environment (IDE): Recommended tools include IntelliJ IDEA (opens in a new tab) and Eclipse (opens in a new tab).
Downloading and Installing JDBC Drivers
Selecting the correct JDBC driver is crucial. For example, if you're working with MySQL, you would use the MySQL Connector/J (opens in a new tab).
To install the JDBC driver:
- Download the JDBC driver JAR file.
- Add the JAR file to your project's classpath.
Configuring Your IDE
Once the JDBC driver is downloaded, configure your IDE to include the driver in the project's build path. This typically involves right-clicking on your project, selecting properties, and adding the JDBC driver under libraries.
Connection URL Syntax
Understanding the database URL syntax is essential for establishing a connection. A typical MySQL connection URL looks like this:
jdbc:mysql://localhost:3306/yourDatabaseName
This URL specifies the protocol (jdbc:mysql
), the server address (localhost
), the port (3306
), and the database name (yourDatabaseName
).
Chat2DB: Efficient Database Management
Using tools like Chat2DB (opens in a new tab) can significantly streamline database connection management. Chat2DB is an AI-powered database visualization management tool that supports over 24 databases and enhances management efficiency. It features natural language SQL generation, intelligent SQL editors, and visual data analysis capabilities, simplifying the connection setup process.
Establishing a Connection with JDBC
After setting up your environment, you can establish a connection to your database.
Using DriverManager to Connect
The DriverManager.getConnection()
method is essential for initiating a database connection. Here’s a basic example of connecting to a MySQL database:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class JDBCExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/yourDatabaseName";
String user = "yourUsername";
String password = "yourPassword";
try {
Connection connection = DriverManager.getConnection(url, user, password);
System.out.println("Connection established successfully!");
// Close the connection
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Managing Database Credentials Securely
When connecting to a database, it’s vital to manage credentials securely. Avoid hardcoding sensitive information into your application. Instead, consider using environment variables or configuration files.
Connection Pooling
Connection pooling is a technique used to enhance performance by reusing existing database connections instead of creating new ones for each request. Libraries like HikariCP (opens in a new tab) are popular for implementing connection pooling in JDBC applications.
Common Connection Issues
When establishing a connection, you may encounter various exceptions. Common issues include:
- SQLSyntaxErrorException: Indicates a syntax error in your SQL statement.
- CommunicationsException: Suggests that the database server is unreachable.
Executing SQL Statements with JDBC
JDBC provides multiple ways to execute SQL queries, including Statement, PreparedStatement, and CallableStatement.
Using Statement and PreparedStatement
For executing basic SQL queries, a Statement
can be utilized:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
import java.sql.Statement;
public class StatementExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/yourDatabaseName";
String user = "yourUsername";
String password = "yourPassword";
try (Connection connection = DriverManager.getConnection(url, user, password);
Statement statement = connection.createStatement()) {
String sql = "SELECT * FROM yourTableName";
ResultSet resultSet = statement.executeQuery(sql);
while (resultSet.next()) {
System.out.println("Column1: " + resultSet.getString("column1"));
}
System.out.println("Query executed successfully!");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
When handling user input, it is advisable to use PreparedStatement
to prevent SQL injection attacks:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class PreparedStatementExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/yourDatabaseName";
String user = "yourUsername";
String password = "yourPassword";
String insertSQL = "INSERT INTO yourTableName (column1, column2) VALUES (?, ?)";
try (Connection connection = DriverManager.getConnection(url, user, password);
PreparedStatement preparedStatement = connection.prepareStatement(insertSQL)) {
preparedStatement.setString(1, "value1");
preparedStatement.setString(2, "value2");
preparedStatement.executeUpdate();
System.out.println("Data inserted successfully!");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Handling SQL Exceptions
It's important to handle SQL exceptions effectively. Utilize try-catch blocks and log exceptions for debugging purposes to maintain application stability.
Working with ResultSets
ResultSets represent the data returned from executing a SQL query. Iterating over a ResultSet allows you to retrieve query results:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class ResultSetExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/yourDatabaseName";
String user = "yourUsername";
String password = "yourPassword";
try (Connection connection = DriverManager.getConnection(url, user, password);
Statement statement = connection.createStatement()) {
String sql = "SELECT * FROM yourTableName";
ResultSet resultSet = statement.executeQuery(sql);
while (resultSet.next()) {
System.out.println("Column1: " + resultSet.getString("column1"));
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Advanced Features: Batch Processing and Transactions
JDBC also supports batch processing and transaction management. Here’s an example of batch processing:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class BatchProcessingExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/yourDatabaseName";
String user = "yourUsername";
String password = "yourPassword";
String insertSQL = "INSERT INTO yourTableName (column1) VALUES (?)";
try (Connection connection = DriverManager.getConnection(url, user, password);
PreparedStatement preparedStatement = connection.prepareStatement(insertSQL)) {
for (int i = 1; i <= 10; i++) {
preparedStatement.setString(1, "value" + i);
preparedStatement.addBatch();
}
preparedStatement.executeBatch();
System.out.println("Batch insert executed successfully!");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Handling Transactions in JDBC
Transactions are essential for ensuring data integrity in databases. JDBC provides methods for managing transactions effectively.
Key Transaction Terms
- Commit: Finalizes the changes made in the current transaction.
- Rollback: Reverts the changes made in the current transaction.
- Savepoint: Allows you to set a point within a transaction to which you can roll back.
Managing Transactions Manually
To manage transactions effectively, disable auto-commit mode:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class TransactionExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/yourDatabaseName";
String user = "yourUsername";
String password = "yourPassword";
try (Connection connection = DriverManager.getConnection(url, user, password)) {
connection.setAutoCommit(false);
// Perform multiple operations
// connection.commit(); // Uncomment to commit
// connection.rollback(); // Uncomment to rollback
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Isolation Levels and Data Integrity
Isolation levels determine how transaction integrity is visible to other transactions. Understanding these levels is crucial for concurrency control.
ACID Properties
The ACID properties (Atomicity, Consistency, Isolation, Durability) are fundamental for transactions in database systems, ensuring that transactions are processed reliably.
Optimizing JDBC Performance
Performance optimization is vital for JDBC applications, especially when dealing with large datasets.
Minimizing Network Latency
Network latency can significantly affect performance. Optimize connection management by using connection pooling libraries like Chat2DB (opens in a new tab) to minimize overhead.
Selecting the Right JDBC Driver
Choosing the appropriate JDBC driver and configuring it correctly can lead to performance improvements. Make sure to review the documentation for your specific driver for best practices.
Query Optimization Techniques
Implement query optimization techniques, such as:
- Indexing: Improves the speed of data retrieval.
- Caching: Reduces the number of times a query is executed.
- Query Rewriting: Simplifies complex queries to improve performance.
Resource Management
Always close connections, statements, and result sets promptly to minimize resource usage:
try (Connection connection = DriverManager.getConnection(url, user, password);
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery(sql)) {
// Process results
} catch (SQLException e) {
e.printStackTrace();
}
Lazy Loading in JDBC
Lazy loading is a design pattern that delays the initialization of an object until the point at which it is needed. This can be particularly useful in JDBC to improve application performance.
Advanced JDBC Features
JDBC is not just limited to basic SQL execution; it also supports advanced features that enhance its functionality.
Working with Stored Procedures
You can call stored procedures in JDBC using CallableStatement
:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.CallableStatement;
import java.sql.SQLException;
public class StoredProcedureExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/yourDatabaseName";
String user = "yourUsername";
String password = "yourPassword";
String sql = "{call yourStoredProcedure(?, ?)}";
try (Connection connection = DriverManager.getConnection(url, user, password);
CallableStatement callableStatement = connection.prepareCall(sql)) {
callableStatement.setString(1, "param1");
callableStatement.setString(2, "param2");
callableStatement.execute();
System.out.println("Stored procedure executed successfully!");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
Working with Large Objects (LOBs)
JDBC supports handling large objects (LOBs) such as BLOBs (Binary Large Objects) and CLOBs (Character Large Objects).
Metadata Retrieval
Retrieving metadata allows you to dynamically examine database structures using the DatabaseMetaData
interface. This can be useful for generating dynamic queries based on the database schema.
Row Sets
Row sets are a wrapper around ResultSets that make it easier to work with data in a more object-oriented way. They provide features like scrollable and updatable result sets.
JDBC 4.0 Enhancements
JDBC 4.0 introduced features like automatic driver loading, simplifying the connection process by eliminating the need to register JDBC drivers manually.
FAQ
-
What is JDBC? JDBC stands for Java Database Connectivity, a standard API for connecting Java applications to databases.
-
What are the types of JDBC drivers? There are four types of JDBC drivers: JDBC-ODBC Bridge Driver, Native-API Driver, Network Protocol Driver, and Thin Driver.
-
How does JDBC help prevent SQL injection? JDBC uses PreparedStatements, which separate SQL code from data, effectively preventing SQL injection attacks.
-
What is connection pooling? Connection pooling is a technique used to manage database connections efficiently by reusing existing connections rather than creating new ones for every request.
-
What is Chat2DB? Chat2DB is an AI-powered database visualization management tool that enhances database management efficiency through features like natural language SQL generation and intelligent SQL editors.
For those interested in exploring an intuitive database management solution, consider switching to Chat2DB (opens in a new tab) to take advantage of its AI capabilities and streamlined database management features, surpassing traditional tools like DBeaver, MySQL Workbench, and DataGrip.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!