How to Create Functions in MySQL: A Step-by-Step Tutorial
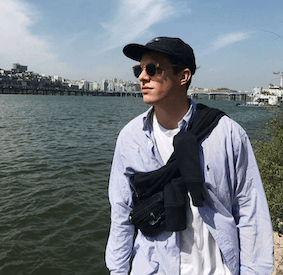
What You Need to Know About Creating Functions in MySQL
Creating functions in MySQL is a vital aspect of effective database management. Functions enable you to perform calculations and complex operations efficiently, allowing for streamlined data manipulation. Unlike stored procedures, which execute batch operations, MySQL functions return a single value and can be integrated directly into SQL statements, making them essential for data analysis.
Benefits of Implementing MySQL Functions
Utilizing functions in MySQL comes with numerous advantages:
- Code Reusability: Functions allow you to encapsulate code that can be reused across multiple queries, minimizing redundancy.
- Modular Design: By dividing complex operations into smaller, manageable functions, your code becomes easier to maintain and debug.
- Optimized Performance: Functions can enhance the efficiency of your queries, leading to improved database performance.
Exploring the Different Types of MySQL Functions
MySQL offers a variety of built-in functions, categorized as follows:
- String Functions: Functions like
CONCAT()
,LENGTH()
, andSUBSTRING()
manipulate string data effectively. - Numeric Functions: Functions such as
ROUND()
,AVG()
, andSUM()
perform calculations on numeric values. - Date/Time Functions: Functions like
NOW()
,CURDATE()
, andDATEDIFF()
manage date and time data proficiently.
Understanding these functions is crucial for data analysis, as they allow analysts to construct complex queries with ease. However, be mindful of potential performance issues stemming from inefficient function design.
Preparing Your MySQL Environment for Function Creation
Before diving into creating functions, it's important to set up your MySQL environment correctly.
Installing MySQL: A Step-by-Step Guide
If you haven't installed MySQL yet, download it from the official MySQL website and follow the installation instructions specific to your operating system—Windows, Mac, or Linux.
Granting Permissions for Function Creation
Ensure you possess the necessary permissions to create functions. Verify your privileges with the following SQL command:
SHOW GRANTS FOR CURRENT_USER;
Accessing MySQL for Function Management
You can access MySQL via the command line or graphical tools like phpMyAdmin. For a more intuitive experience, consider using Chat2DB, an AI-driven database visualization management tool that simplifies MySQL database management, including function creation.
Structuring Your Database
Before implementing functions, it’s essential to ensure your database structure is well-organized. Confirm that tables are correctly set up, and back up your database to prevent data loss during modifications.
Step-by-Step Guide to Creating a Basic Function in MySQL
Creating a function in MySQL involves several straightforward steps.
Syntax Overview for Function Creation
The basic syntax for creating a function in MySQL is as follows:
CREATE FUNCTION function_name (parameters)
RETURNS return_type
BEGIN
-- function logic
END;
Example: Creating a Simple Addition Function
Here’s a practical example of a MySQL function that adds two numbers:
CREATE FUNCTION add_numbers(a INT, b INT)
RETURNS INT
BEGIN
RETURN a + b;
END;
Testing Your Function
To test the newly created function, use the following SQL command:
SELECT add_numbers(5, 10) AS result;
Common Function Creation Errors to Avoid
While creating functions, be vigilant about potential errors, such as:
- Syntax errors in the function definition.
- Mismatched data types for parameters or return values.
- Neglecting to declare the function as
DETERMINISTIC
if its results are predictable.
Advanced Techniques for MySQL Functions
Once you're comfortable with the basics, you can explore more advanced concepts related to MySQL functions.
Utilizing Control Flow Statements
Control flow statements like IF
, CASE
, and LOOP
can be incorporated within functions. For example:
CREATE FUNCTION check_number(num INT)
RETURNS VARCHAR(20)
BEGIN
DECLARE result VARCHAR(20);
IF num > 0 THEN
SET result = 'Positive';
ELSEIF num < 0 THEN
SET result = 'Negative';
ELSE
SET result = 'Zero';
END IF;
RETURN result;
END;
Interacting with Database Tables
Functions can interact with database tables. For instance, you can create a function to count employees in a specific department:
CREATE FUNCTION get_employee_count(department_id INT)
RETURNS INT
BEGIN
DECLARE emp_count INT;
SELECT COUNT(*) INTO emp_count FROM employees WHERE department_id = department_id;
RETURN emp_count;
END;
Leveraging Aggregate Functions
You can also utilize aggregate functions within user-defined functions. For example, to calculate the average salary:
CREATE FUNCTION average_salary(department_id INT)
RETURNS DECIMAL(10,2)
BEGIN
DECLARE avg_salary DECIMAL(10,2);
SELECT AVG(salary) INTO avg_salary FROM employees WHERE department_id = department_id;
RETURN avg_salary;
END;
Enhancing Function Performance in MySQL
To maximize the performance of your MySQL functions, keep the following tips in mind:
Implementing Indexing Strategies
Proper indexing can drastically improve function performance. Ensure that relevant fields in your tables have appropriate indexes.
Analyzing Query Performance
Utilize the EXPLAIN
statement to analyze the performance of queries executed within functions, helping you pinpoint and resolve bottlenecks.
Minimizing Unnecessary Calculations
Avoid unnecessary computations within functions to enhance performance. Caching results instead of recalculating them can lead to efficiency gains.
Monitoring Resource Utilization
Keep an eye on memory and CPU usage while using functions. Efficient resource management can significantly boost overall database performance.
Integrating MySQL Functions with Other Database Features
MySQL functions can be seamlessly integrated with various database features, enhancing functionality.
Utilizing Functions in Stored Procedures
Functions can be called within stored procedures, allowing for complex operations that require multiple function calls.
Using Functions in Views
Incorporate functions in views for advanced data transformations, simplifying data manipulation.
Ensuring Data Validation
Functions are instrumental in data validation, helping maintain integrity constraints in your database.
Implementing Business Logic
You can embed business logic within your database through functions, ensuring data operations comply with specific rules.
Effective Troubleshooting and Debugging of MySQL Functions
When creating functions, you may face challenges. Here are strategies for troubleshooting and resolving common issues:
Recognizing Common Errors
Be aware of frequent errors when creating functions, such as syntax mistakes and incorrect data types.
Utilizing Error Logs for Diagnosis
Leverage MySQL error logs to diagnose performance issues related to function execution.
Testing with Diverse Input Scenarios
Conduct tests with various input scenarios to ensure your functions handle different conditions effectively.
Maintaining Version Control and Documentation
Keep version control and documentation for your functions to track changes and updates systematically.
Engaging with Community Resources
Tap into community resources and forums for assistance and advice on MySQL functions, enhancing your knowledge and troubleshooting abilities.
By adhering to these guidelines and utilizing Chat2DB, you can efficiently create and manage MySQL functions, significantly enhancing your database operations. Chat2DB's AI capabilities simplify database visualization and management, allowing you to focus more on critical data analysis tasks.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!