Creating a Chat Application with Supabase and WebSockets
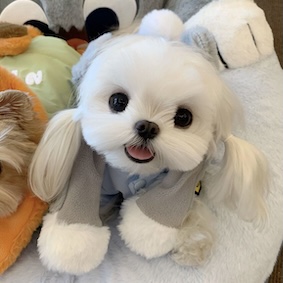
Introduction
In today's digital age, real-time communication has become a crucial aspect of many applications. Chat applications are widely used for instant messaging, collaboration, and customer support. Building a chat application with real-time capabilities requires a robust backend infrastructure that can handle concurrent connections and deliver messages instantly. In this tutorial, we will explore how to create a chat application using Supabase, a powerful open-source platform, and WebSockets, a communication protocol that enables real-time data transfer.
Supabase is a platform that combines the power of a relational database with the flexibility of serverless functions, making it an ideal choice for building scalable and real-time applications. WebSockets, on the other hand, provide a persistent connection between the client and server, allowing for bidirectional communication without the need for constant polling.
This tutorial will demonstrate the integration of Supabase and WebSockets to create a feature-rich chat application that supports real-time messaging, user authentication, and message persistence.
Core Concepts and Background
Supabase
Supabase is an open-source platform that offers a PostgreSQL database as a service, along with serverless functions and authentication services. It provides a simple and scalable solution for building applications that require a robust backend infrastructure. Supabase's real-time capabilities make it an excellent choice for developing chat applications where instant updates are essential.
WebSockets
WebSockets is a communication protocol that enables bidirectional communication between the client and server over a single, long-lived connection. Unlike traditional HTTP requests, WebSockets allow for real-time data transfer without the overhead of constant polling. This makes WebSockets ideal for applications that require instant updates, such as chat applications.
Chat Application Architecture
A typical chat application architecture consists of a frontend client, a backend server, and a database for storing messages and user data. The frontend client interacts with the backend server using WebSockets to send and receive messages in real-time. The backend server handles message routing, user authentication, and message persistence in the database.
Key Strategies, Technologies, or Best Practices
Integration of Supabase and WebSockets
To create a chat application with real-time capabilities, we need to integrate Supabase for data storage and WebSockets for real-time communication. Supabase provides a REST API for interacting with the database, while WebSockets enable real-time updates between clients.
User Authentication
User authentication is a critical aspect of any chat application to ensure secure access and data privacy. By leveraging Supabase's authentication services, we can implement user registration, login, and session management to authenticate users and authorize access to chat features.
Message Persistence
Message persistence is essential for storing chat messages and retrieving them when users reconnect to the chat application. By utilizing Supabase's PostgreSQL database, we can store messages in a structured format and query them efficiently to provide a seamless chat experience.
Practical Examples, Use Cases, or Tips
Example 1: Setting Up Supabase
To get started, create a Supabase project and configure the database schema for storing chat messages. Use the Supabase client library to interact with the database and perform CRUD operations on messages.
// Example code for initializing Supabase client
const supabase = createClient('https://your-project-url.supabase.co', 'your-api-key');
Example 2: Implementing WebSockets
Integrate WebSockets into the frontend client to establish a real-time connection with the backend server. Use libraries like Socket.IO or WebSocket API to handle WebSocket events and send/receive messages in real-time.
// Example code for connecting to WebSocket server
const socket = new WebSocket('wss://your-server-url');
Example 3: Real-Time Messaging
Implement real-time messaging functionality by sending and receiving messages over WebSockets. Use event-driven architecture to handle message events and update the chat interface in real-time.
// Example code for sending a message over WebSocket
socket.send(JSON.stringify({ type: 'message', content: 'Hello, world!' }));
Using Supabase and WebSockets in Projects
By combining Supabase and WebSockets, developers can build feature-rich chat applications with real-time capabilities, user authentication, and message persistence. These technologies provide a scalable and efficient solution for handling real-time communication and data storage in modern applications.
In conclusion, creating a chat application with Supabase and WebSockets offers a powerful and flexible solution for building real-time communication features. Developers can leverage the integration of these technologies to deliver seamless chat experiences to users.
For future projects, consider exploring advanced features such as message encryption, file sharing, and message notifications to enhance the functionality of your chat application.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!