Creating a table in PostgreSQL with proper data types and constraints
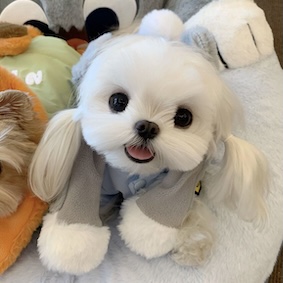
Introduction
PostgreSQL is a powerful open-source relational database management system that allows users to create tables with specific data types and constraints. This article will delve into the importance of defining proper data types and constraints when creating tables in PostgreSQL to maintain data integrity and optimize performance.
In today's data-driven world, the ability to store and retrieve data efficiently is crucial for businesses and organizations. By understanding how to create tables with appropriate data types and constraints in PostgreSQL, developers can ensure that their databases are well-structured and performant.
PostgreSQL's flexibility and robust features make it a popular choice for various applications, from small-scale projects to large enterprise systems. By leveraging PostgreSQL's capabilities to define data types and constraints, developers can design databases that meet their specific requirements and scale effectively.
Core Concepts and Background
When creating a table in PostgreSQL, it is essential to consider the data types and constraints for each column. Data types define the kind of data that can be stored in a column, such as integers, text, dates, or boolean values. Constraints, on the other hand, enforce rules or conditions on the data stored in a column to maintain data integrity.
Data Types
PostgreSQL offers a wide range of data types to accommodate different kinds of data. Some common data types include:
- Integer: Used for whole numbers.
- Text: Used for variable-length character strings.
- Date: Used for storing date values.
- Boolean: Used for true/false values.
Choosing the appropriate data type for each column ensures that the database stores data efficiently and accurately. For example, using an integer data type for a column that stores numerical values can optimize storage space and improve query performance.
Constraints
Constraints in PostgreSQL help enforce data integrity by defining rules that the data must follow. Some common constraints include:
- Primary Key: Ensures each row in a table is uniquely identified.
- Foreign Key: Establishes a link between two tables based on a column's values.
- Not Null: Ensures a column cannot contain null values.
- Check: Enforces a condition on the data stored in a column.
By applying constraints to columns, developers can prevent invalid data from being inserted into the database, maintain referential integrity between tables, and enforce business rules on the data.
Practical Examples
- Creating a Users Table
CREATE TABLE users (
user_id SERIAL PRIMARY KEY,
username VARCHAR(50) NOT NULL,
email VARCHAR(100) UNIQUE,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
In this example, we create a users
table with columns for user_id
, username
, email
, and created_at
. The user_id
column is defined as a serial primary key, ensuring each user has a unique identifier. The username
column is set to not allow null values, and the email
column is marked as unique to prevent duplicate email addresses.
- Defining a Products Table
CREATE TABLE products (
product_id SERIAL PRIMARY KEY,
name VARCHAR(100) NOT NULL,
price NUMERIC(10, 2) CHECK (price >= 0),
category VARCHAR(50)
);
In this example, we create a products
table with columns for product_id
, name
, price
, and category
. The price
column is defined as numeric with a check constraint to ensure it is a non-negative value.
- Setting Up an Orders Table
CREATE TABLE orders (
order_id SERIAL PRIMARY KEY,
user_id INT REFERENCES users(user_id),
product_id INT REFERENCES products(product_id),
quantity INT NOT NULL CHECK (quantity > 0),
order_date DATE
);
In this example, we create an orders
table with columns for order_id
, user_id
, product_id
, quantity
, and order_date
. The user_id
and product_id
columns are defined as foreign keys referencing the users
and products
tables, respectively. The quantity
column is set to not allow null values and must be greater than zero.
Key Strategies and Best Practices
-
Use Enumerated Data Types: Enumerated data types can be used to restrict a column's value to a predefined list of options. This can improve data consistency and readability.
-
Normalize Data: Normalize your database schema to reduce redundancy and improve data integrity. By breaking down data into smaller, related tables, you can avoid data anomalies and improve query performance.
-
Indexing: Properly indexing columns that are frequently used in queries can significantly improve query performance. Consider creating indexes on columns used in joins, where clauses, or order by statements.
Conclusion
Creating tables in PostgreSQL with appropriate data types and constraints is essential for maintaining data integrity and optimizing database performance. By understanding the core concepts of data types and constraints, developers can design well-structured databases that meet their application's requirements.
As technology continues to evolve, the importance of efficient data storage and retrieval will only increase. PostgreSQL's robust features and flexibility make it a valuable tool for developers looking to build scalable and performant applications.
For those looking to dive deeper into PostgreSQL's capabilities, exploring advanced features such as partitioning, replication, and advanced indexing techniques can further enhance database performance and scalability.
In conclusion, mastering the art of creating tables with proper data types and constraints in PostgreSQL is a fundamental skill for any developer working with relational databases.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!