How to Effectively Utilize dbms_output.put_line in Your PL/SQL Programs for Debugging and Logging
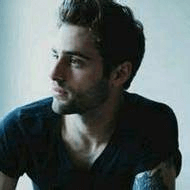
Understanding dbms_output.put_line: A Key Tool for PL/SQL Development
In the realm of Oracle PL/SQL programming, the function dbms_output.put_line
serves as a fundamental tool for developers. This built-in package allows you to display output from PL/SQL blocks and triggers, making it invaluable for debugging and educational purposes. The primary function of dbms_output.put_line
is to provide an easy method for developers to output information, helping them track variable values and execution flow within their programs.
Unlike other output methods such as UTL_FILE (opens in a new tab) and DBMS_OUTPUT.PUT
, dbms_output.put_line
is designed for session-based output, meaning that the data is not stored permanently and is limited to the duration of the session. This feature makes it particularly useful for quick debugging sessions and educational demonstrations, where developers can visually inspect the execution of their PL/SQL logic.
However, it is essential to recognize the limitations of dbms_output.put_line
. For instance, it struggles with large volumes of data, often resulting in truncated outputs if not configured correctly. Despite these limitations, its straightforward syntax makes it an excellent choice for beginners learning the basics of PL/SQL programming.
BEGIN
DBMS_OUTPUT.PUT_LINE('Hello, World!');
END;
This simple code snippet illustrates how dbms_output.put_line
can display a basic string message in the console.
Configuring Your Environment for dbms_output.put_line
Before you can effectively use dbms_output.put_line
, you must ensure that your environment is properly configured. One of the first steps is to enable server output in your SQL*Plus environment by executing the command:
SET SERVEROUTPUT ON;
This command activates the output buffer, allowing any messages generated by dbms_output.put_line
to be displayed in your SQL*Plus console.
Adjusting Buffer Size to Prevent Output Truncation
To avoid output truncation, especially when dealing with larger outputs, you might also want to adjust your buffer size. The default buffer size in SQL*Plus is often limited to 20,000 bytes. You can increase this limit with the following command:
SET SERVEROUTPUT ON SIZE 1000000; -- Increases buffer size to 1,000,000 bytes
When using dbms_output.put_line
in environments like Oracle SQL Developer (opens in a new tab) or Chat2DB (opens in a new tab), ensure that the server output settings are configured for optimal use. In Chat2DB, this can be done in the settings menu under "SQL Execution."
Common Configuration Pitfalls
When configuring your environment, be mindful of common pitfalls. Forgetting to enable server output or failing to set an adequate buffer size can result in missing output. If you encounter issues, troubleshooting can often be as simple as rechecking these settings.
Practical Use Cases for dbms_output.put_line in PL/SQL
dbms_output.put_line
shines in various scenarios, particularly in debugging PL/SQL procedures and triggers. For example, when tracking the values of variables or checking the flow of execution, you can use this function like so:
DECLARE
v_name VARCHAR2(50) := 'Alice';
BEGIN
DBMS_OUTPUT.PUT_LINE('The name is: ' || v_name);
END;
Educational Applications
In educational contexts, dbms_output.put_line
serves as a great tool for demonstrating SQL logic and control structures. For instance, you can illustrate how loops work:
DECLARE
v_counter NUMBER := 1;
BEGIN
WHILE v_counter <= 5 LOOP
DBMS_OUTPUT.PUT_LINE('Counter value: ' || v_counter);
v_counter := v_counter + 1;
END LOOP;
END;
This code will output the counter values from 1 to 5, helping students visually understand the loop's behavior.
Logging and Monitoring Execution Flow
During the development phase, dbms_output.put_line
can also be used for logging and monitoring. By printing statements at various checkpoints in your code, you can easily track execution flow and identify where any issues may arise.
BEGIN
DBMS_OUTPUT.PUT_LINE('Starting procedure execution');
-- Your PL/SQL code here
DBMS_OUTPUT.PUT_LINE('Procedure completed successfully');
EXCEPTION
WHEN OTHERS THEN
DBMS_OUTPUT.PUT_LINE('Error occurred: ' || SQLERRM);
END;
Advanced Techniques for Utilizing dbms_output.put_line
To maximize the utility of dbms_output.put_line
, consider employing advanced techniques. For managing large data sets, you can break output into smaller chunks to avoid buffer overflow.
Output Formatting for Enhanced Readability
Output formatting is another crucial aspect to enhance debugging efficiency. By using concatenation and line breaks, you can make outputs more readable:
BEGIN
DBMS_OUTPUT.PUT_LINE('User Details:');
DBMS_OUTPUT.PUT_LINE('---------------------');
DBMS_OUTPUT.PUT_LINE('Name: ' || v_name);
DBMS_OUTPUT.PUT_LINE('Age: ' || v_age);
END;
Conditional Output to Streamline Debugging
Implementing conditional output allows you to execute dbms_output.put_line
statements based on specific conditions. This strategy limits unnecessary output and helps keep your debugging process focused.
BEGIN
IF some_condition THEN
DBMS_OUTPUT.PUT_LINE('Condition met!');
END IF;
END;
Exception Handling with Detailed Error Reporting
Integrating dbms_output.put_line
with exception handling is an effective way to provide detailed error messages during runtime:
BEGIN
-- Code that might throw an exception
EXCEPTION
WHEN OTHERS THEN
DBMS_OUTPUT.PUT_LINE('Error: ' || SQLERRM);
END;
Comparison of dbms_output.put_line with Other Debugging Tools
When comparing dbms_output.put_line
with other PL/SQL debugging and logging tools, its simplicity and ease of use stand out, especially for smaller applications and educational settings. While UTL_FILE (opens in a new tab) provides file-based output for permanent logging, it comes with added complexity. In contrast, dbms_output.put_line
is straightforward for quick debugging tasks.
Other Tools to Consider
- DBMS_ALERT: Offers asynchronous messaging for inter-process communication.
- DBMS_PIPE: Facilitates message passing between sessions but is generally more complex than
dbms_output.put_line
.
Despite its advantages, dbms_output.put_line
has limitations, including its restriction to session-based output. Once the session terminates, the output is lost, unlike persistent logging methods.
Integrating dbms_output.put_line with Chat2DB for Enhanced Development
Integrating dbms_output.put_line
within the Chat2DB (opens in a new tab) environment can significantly enhance your PL/SQL development experience. Chat2DB's user-friendly interface allows for seamless management of PL/SQL scripts and outputs from dbms_output.put_line
.
Benefits of Using Chat2DB for dbms_output.put_line
With Chat2DB, developers benefit from real-time collaboration and script sharing, which complements the debugging process facilitated by dbms_output.put_line
. Setting up Chat2DB for optimal use with dbms_output.put_line
is straightforward:
- Enable output viewing in the Chat2DB settings.
- Set buffer management options to accommodate your output needs.
This integration not only simplifies PL/SQL script management but also enhances the overall development workflow.
Common Errors and Debugging Tips for Using dbms_output.put_line
When using dbms_output.put_line
, developers may encounter several common errors. For instance, buffer overflow can occur if the output exceeds the allocated size, leading to missing output. To troubleshoot these issues, start by checking your server output settings and adjusting buffer sizes accordingly.
Best Practices for Minimizing Errors
To minimize errors:
- Structure your
dbms_output.put_line
statements for clarity. - Maintain organized code to make debugging easier.
- Progressively test PL/SQL blocks to catch errors early in the development cycle.
By following these practices, you can effectively leverage dbms_output.put_line
in your PL/SQL programs.
Frequently Asked Questions (FAQ) About dbms_output.put_line
-
What is dbms_output.put_line used for?
dbms_output.put_line
is used to display output from PL/SQL blocks and triggers, primarily for debugging purposes.
-
How do I enable server output in SQL*Plus?
- You can enable server output by executing the command
SET SERVEROUTPUT ON;
in your SQL*Plus session.
- You can enable server output by executing the command
-
Can I increase the buffer size for dbms_output.put_line?
- Yes, you can increase the buffer size using the command
SET SERVEROUTPUT ON SIZE <size>;
, where<size>
is the desired buffer size.
- Yes, you can increase the buffer size using the command
-
What are the limitations of dbms_output.put_line?
- Its main limitations include session-based output, lack of persistence after session termination, and inefficiency in handling large volumes of data.
-
How can Chat2DB enhance my use of dbms_output.put_line?
- Chat2DB (opens in a new tab) provides a user-friendly interface for managing PL/SQL scripts and outputs, along with features like real-time collaboration that facilitate the debugging process.
By mastering dbms_output.put_line
and utilizing tools like Chat2DB, you can significantly enhance your PL/SQL development experience.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!