Effective Database Caching Techniques: A Practical Guide to Boost Application Performance
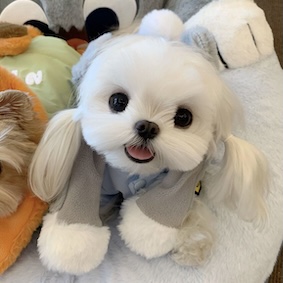
Database caching is a crucial element of modern software architecture that significantly enhances application performance, reduces latency, and optimizes resource utilization. By implementing effective database caching techniques, developers can markedly improve the speed and efficiency of their applications. This guide delves into the essential concepts of database caching, explores its various types, discusses strategies for designing an effective caching layer, and details tools and technologies for implementation. Additionally, we will cover best practices for monitoring and optimizing cache performance. Key terms such as cache, cache hit, cache miss, and cache invalidation will be defined to provide a solid understanding of the importance of caching in application development. As we explore this topic, we will also highlight the benefits of using tools like Chat2DB (opens in a new tab), which leverages advanced AI capabilities to streamline database management and caching.
Understanding Database Caching Techniques
Database caching refers to the process of storing frequently accessed data in a temporary storage location, known as a cache, to reduce the time required to retrieve data from the primary database. By doing so, applications can serve data requests more quickly, enhancing user experience and system performance.
Key Terms
- Cache: A storage layer that temporarily holds data for quick access.
- Cache Hit: When a requested piece of data is found in the cache.
- Cache Miss: When a requested piece of data is not found in the cache, necessitating retrieval from the primary database.
Caching can be categorized into two main types: client-side caching and server-side caching. Client-side caching occurs within the application layer, while server-side caching is managed by the database server. Understanding the appropriate use cases for each type is crucial for optimizing performance.
Cache Consistency and Invalidation
Maintaining cache consistency is vital to ensure that the cache accurately reflects the most current state of the primary database. Cache invalidation strategies are employed to manage the data lifecycle in the cache. Common strategies include time-based expiration and event-driven invalidation.
Types of Caches
Various types of database caches are available, including:
- In-Memory Caches: Tools like Memcached (opens in a new tab) and Redis (opens in a new tab) provide low-latency data retrieval by storing data in memory.
- On-Disk Caches: These caches store larger datasets that cannot fit entirely in memory, providing a balance between speed and capacity.
- Distributed Caches: These are designed to scale applications across multiple servers, ensuring high availability and performance.
Cache Type | Features | Use Cases |
---|---|---|
In-Memory Caches | Fast data retrieval, low latency | Real-time applications |
On-Disk Caches | Larger storage capacity, moderate speed | Archiving and large datasets |
Distributed Caches | Scalability, fault tolerance | Cloud applications |
Types of Database Caches
When selecting the right caching strategy, developers must understand the available options. Here, we will explore various types of database caches.
In-Memory Caches
In-memory caching tools like Redis and Memcached are popular choices for applications that require rapid data access. These caches store data directly in RAM, enabling lightning-fast retrieval times.
Code Example: Using Redis for Caching
import redis
# Connect to Redis server
r = redis.StrictRedis(host='localhost', port=6379, db=0)
# Set a value in the cache
r.set('user:1000', 'John Doe')
# Retrieve the value from the cache
user = r.get('user:1000')
print(user) # Output: b'John Doe'
On-Disk Caches
On-disk caching is especially useful for applications handling large datasets that exceed memory capacity. This type of cache writes data to disk, allowing for substantial storage without consuming RAM.
Distributed Caches
Distributed caching systems, such as Apache Ignite or Hazelcast, facilitate horizontal scaling across multiple servers. They enhance reliability and performance by distributing cache data among nodes.
Code Example: Setting Up a Distributed Cache with Hazelcast
import com.hazelcast.core.Hazelcast;
import com.hazelcast.core.HazelcastInstance;
import com.hazelcast.map.IMap;
public class DistributedCacheExample {
public static void main(String[] args) {
HazelcastInstance hz = Hazelcast.newHazelcastInstance();
IMap<Integer, String> map = hz.getMap("users");
// Adding data to the cache
map.put(1, "Alice");
map.put(2, "Bob");
// Retrieving data from the cache
System.out.println(map.get(1)); // Output: Alice
}
}
Write-Through, Write-Around, and Write-Back Caching Strategies
Caching strategies dictate how data is written to the cache.
- Write-Through: Data is written to both the cache and the database simultaneously.
- Write-Around: Data is written directly to the database, with the cache updated upon retrieval.
- Write-Back: Data is written only to the cache, with periodic writes to the database.
Designing an Effective Caching Strategy
Creating a successful caching strategy necessitates careful consideration of various factors, including application workload and data access patterns.
Assessing Application Workload
Identifying high-read operations is essential for determining which data should be cached. Analyze the application to find frequently requested data that is rarely updated.
Setting TTL Values
Time to Live (TTL) values dictate how long data should remain in the cache before being considered stale. Setting appropriate TTLs ensures the cache does not serve outdated data.
Cache Partitioning and Sharding
Cache partitioning involves dividing cache data into smaller, manageable pieces, while sharding distributes data across multiple nodes. Both techniques help optimize cache performance and scalability.
Cache Warming Techniques
Cache warming involves pre-populating the cache with frequently accessed data, reducing the likelihood of cache misses.
Cache Prefetching
Cache prefetching anticipates future data requests based on historical access patterns, pre-loading data into the cache before it is requested.
Monitoring Cache Performance Metrics
Regularly monitoring cache performance metrics is vital for refining caching strategies. Key metrics include cache hit ratio, eviction rates, and latency.
Cache Invalidation and Consistency
One of the most significant challenges in caching is maintaining cache consistency. Cache invalidation strategies must be implemented to ensure the cache reflects the current state of the database.
Invalidation Approaches
Two primary cache invalidation approaches are:
- Time-Based Invalidation: Data is automatically invalidated after a specified time period.
- Event-Based Invalidation: Data is invalidated in response to specific events, such as updates to the primary database.
Cache Coherence
Cache coherence ensures that all cache copies of data remain synchronized with the primary database, which is particularly important in distributed systems where multiple caches may exist.
Practical Example of Cache Invalidation
# Invalidate cache based on time
import time
def cache_data_with_ttl(key, value, ttl):
r.set(key, value)
r.expire(key, ttl)
# Cache user data for 60 seconds
cache_data_with_ttl('user:1001', 'Jane Smith', 60)
Tools and Technologies for Database Caching
Several tools and technologies can facilitate effective database caching.
Leveraging Chat2DB for Caching Management
Chat2DB (opens in a new tab) is an AI-powered database management tool that simplifies the caching process by offering features like natural language SQL generation, intelligent SQL editing, and data analysis visualizations. Its integration of AI allows developers and database administrators to efficiently manage their caching strategies while focusing on more critical tasks. Compared to alternatives like DBeaver, MySQL Workbench, or DataGrip, Chat2DB stands out with its user-friendly interface and advanced AI capabilities that significantly enhance productivity.
Popular Caching Tools
Tool | Key Features | Best Use Cases |
---|---|---|
Chat2DB | AI-driven management, SQL generation | Database management efficiency |
Redis | High-speed data retrieval, persistence options | Real-time applications |
Memcached | Simple key-value store, multi-threaded performance | Caching session data |
Varnish | Web caching, reverse proxy capabilities | Accelerating web content |
Monitoring and Optimizing Cache Performance
Monitoring cache performance is crucial to ensure optimal application performance.
Key Performance Metrics
- Cache Hit Ratio: The percentage of requests served from the cache versus total requests.
- Eviction Rates: The rate at which data is removed from the cache.
- Latency: The time taken to retrieve data from the cache.
Using Monitoring Tools
Employ monitoring tools to track cache utilization and identify performance bottlenecks. Tools like Grafana or Prometheus can be beneficial for visualizing cache performance metrics.
Load Testing and Diagnostics
Conduct load testing to evaluate cache performance under various workloads. Identify common cache-related issues and implement corrective measures as needed.
Case Studies and Best Practices
Real-world case studies can provide insights into successful database caching implementations.
Leading Technology Companies and Caching Approaches
Many leading technology companies utilize caching strategies to enhance application performance. By studying their approaches, developers can learn valuable lessons and avoid common pitfalls.
Industry Best Practices
- Regularly review and update caching strategies based on application demands.
- Engage with community resources and forums to stay updated with the latest caching techniques.
- Experiment with different caching strategies to find the best fit for your applications.
In conclusion, effective database caching is essential for improving application performance. Implementing the right caching strategies, leveraging tools like Chat2DB (opens in a new tab), and continuously monitoring cache performance can significantly enhance the efficiency of your systems.
FAQs
-
What is database caching?
- Database caching is the process of storing frequently accessed data in a temporary storage location to reduce retrieval time from the primary database.
-
What are cache hits and cache misses?
- A cache hit occurs when requested data is found in the cache, while a cache miss occurs when the data needs to be retrieved from the primary database.
-
How do I choose a caching strategy?
- Assess your application workload, identify high-read operations, and determine which data should be cached to choose an appropriate caching strategy.
-
What is the significance of TTL in caching?
- Time to Live (TTL) values define how long data remains in the cache before it is considered stale, helping to maintain cache consistency.
-
How can Chat2DB help with caching?
- Chat2DB offers AI-driven features that simplify database management and caching, making it easier to implement and optimize caching strategies effectively.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!