How to Efficiently Perform MySQL Update Operations: A Step-by-Step Guide
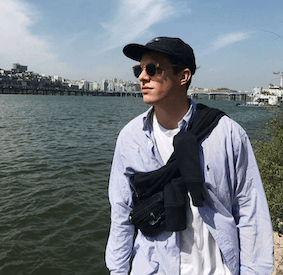
MySQL is a powerful relational database management system and offers various functions to manipulate data. One of the most crucial operations is the UPDATE operation. This operation allows users to modify existing records in a table. Understanding how to effectively use the MySQL UPDATE statement is essential for maintaining accurate data in your database.
Syntax and Structure of the MySQL UPDATE Statement
The basic syntax of the MySQL UPDATE statement is as follows:
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
- table_name: The name of the table where you want to update records.
- SET: This clause specifies the columns to be updated and their new values.
- WHERE: This clause is critical as it identifies which records should be updated. Omitting this clause can lead to unintentional updates of all records in the table.
Importance of the WHERE Clause
The WHERE clause is essential in an UPDATE statement. Without it, every record in the table will be updated, which is often not the desired outcome. For example:
UPDATE employees
SET salary = 50000; -- This will set the salary of all employees to 50000!
In contrast, using a proper WHERE clause ensures that only the intended records are modified:
UPDATE employees
SET salary = 50000
WHERE department = 'Sales'; -- This only updates the salary of employees in the Sales department.
Using the SET Clause
The SET clause defines what changes will be made to the specified columns. You can update multiple columns in a single statement:
UPDATE employees
SET salary = 60000, title = 'Senior Developer'
WHERE employee_id = 123;
In this example, both the salary and title of the employee with employee_id
123 will be updated.
Primary Key and Unique Identifiers
When performing updates, it is crucial to ensure that the table you are updating has a primary key or unique identifier. This helps in precisely identifying the records to be modified. Without a unique identifier, you risk updating multiple records unintentionally.
Potential Pitfalls of MySQL Update
A common mistake is to forget the WHERE clause. This can result in a full table update, which might lead to data loss or corruption. For example:
UPDATE products
SET price = price * 1.1; -- This increases the price of all products by 10%.
Instead, always double-check your WHERE clause to confirm that you are targeting the correct records.
Performance Considerations
When updating large datasets, performance can be a concern. Here are some factors that can impact update performance:
- Indexing: Proper indexing can speed up the search for records that need to be updated.
- Batching Updates: Instead of updating records one by one, consider batching updates to reduce lock contention.
- Dataset Size: Reducing the size of the dataset being updated can significantly enhance performance.
Example of Simple vs. Complex Updates
A simple update involves changing a single column for a specific record:
UPDATE customers
SET last_name = 'Smith'
WHERE customer_id = 456;
A complex update might involve multiple columns and conditions:
UPDATE orders
SET status = 'Shipped', shipment_date = NOW()
WHERE order_id IN (SELECT order_id FROM pending_orders);
Optimizing MySQL Update Performance
To enhance the performance of MySQL UPDATE operations, consider the following strategies:
Indexing
When you index the columns used in the WHERE clause, MySQL can locate the target rows faster, thus speeding up the update process.
Reducing Dataset Size
Updating a smaller subset of data improves performance. Consider filtering records with specific conditions to limit the data being modified.
Batching Updates
Batching allows you to group multiple updates into a single operation, reducing the overhead associated with each individual update.
Temporary Tables
Using temporary tables to stage updates can help manage large datasets more effectively. You can perform updates on the temporary table and then merge the changes back to the main table.
Query Caching
Effective configuration of query caching can significantly impact update performance. Analyze your queries to determine if caching can be beneficial.
Using EXPLAIN
The EXPLAIN command can help analyze your update statements and identify performance bottlenecks. Running EXPLAIN on a query provides insight into how MySQL executes it.
Monitoring Server Parameters
Regularly monitor and fine-tune MySQL server parameters related to updates to ensure optimal performance.
Best Practices for MySQL Update Operations
To ensure efficient and reliable MySQL update operations, follow these best practices:
Backup Data
Always back up your data before performing bulk updates. This protects against accidental data loss.
Test Before Applying
Test your update queries on a small subset of data to ensure they function as intended before applying them to the entire dataset.
Use Transactions
Utilize transactions to guarantee data integrity. If an update fails, you can roll back changes to maintain a consistent state.
Logging
Implement logging for update operations. This helps track changes and is useful for auditing purposes.
Stored Procedures
For complex update logic, consider using stored procedures. They improve maintainability and can encapsulate the update logic.
Keep Software Updated
Regularly update your software and libraries to avoid known bugs that could affect update operations.
Regular Maintenance
Perform regular database maintenance, such as optimizing tables and updating statistics, to ensure ongoing performance.
Handling Concurrency in MySQL Updates
Concurrency management is vital when multiple users or processes update records simultaneously. MySQL employs various strategies to handle this effectively.
Isolation Levels
Isolation levels determine how transaction integrity is visible to other transactions. Setting appropriate isolation levels can help avoid anomalies like dirty reads.
Locking Mechanisms
MySQL provides locking mechanisms such as row-level locking to prevent conflicts during concurrent updates. This ensures that one update does not interfere with another.
Optimistic Concurrency Control
Optimistic concurrency control can be implemented to manage concurrent updates. This approach assumes that conflicts are rare and checks for them before committing changes.
Long-Running Updates
Long-running updates can create issues in a concurrent environment. It’s essential to minimize the duration of these updates to reduce the impact on other operations.
Testing in Concurrent Environments
Test update operations in a concurrent environment to identify potential issues. This allows teams to address conflicts and optimize performance proactively.
Using Chat2DB for Efficient MySQL Updates
Chat2DB is a powerful tool that can streamline MySQL update operations. Its intuitive interface makes it easier to construct and test update queries effectively.
Visualizing Data Changes
Chat2DB helps users visualize data changes before and after updates, ensuring clarity and reducing the risk of errors.
Query Optimization Tools
The query optimization tools in Chat2DB assist in improving update performance by analyzing queries and suggesting enhancements.
Automating Routine Tasks
Chat2DB can automate routine update tasks, saving time and reducing the likelihood of manual errors.
Version Control Integration
With integration into version control systems, Chat2DB helps track changes to update queries, making collaboration among team members more efficient.
User Testimonials
Many users have reported significant improvements in managing MySQL updates with Chat2DB. Case studies demonstrate its effectiveness in streamlining database operations.
Troubleshooting Common MySQL Update Issues
When updating records in MySQL, you may encounter various issues. Here are some common problems and their solutions:
Duplicate Entry Errors
These occur when an update attempts to set a value that violates a unique constraint. Review your update logic and ensure values respect the constraints defined in your schema.
Performance Issues
Identify and resolve performance issues by reviewing your update queries. Utilize the EXPLAIN command to analyze query execution plans.
Update Conflicts
Conflicts can arise during concurrent updates. Implement proper locking mechanisms and test transactions to avoid deadlocks.
Data Type Mismatches
Ensure that the data types of values being updated match the column definitions. Mismatches can lead to conversion errors.
MySQL Logs
Use MySQL logs to diagnose and troubleshoot update issues. They provide valuable information about query performance and errors.
Foreign Key Constraints
During updates, ensure that foreign key constraints are not violated. This may require validating related data before performing updates.
Debugging Tools
Utilize debugging tools and techniques to identify and fix complex update-related problems. These resources can help streamline troubleshooting processes.
By understanding and implementing these strategies, you can enhance your MySQL update operations and maintain data integrity effectively. For further learning and practical application, consider using Chat2DB to optimize your database management processes.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!