Firebase vs MongoDB: Key Differences and Use Cases
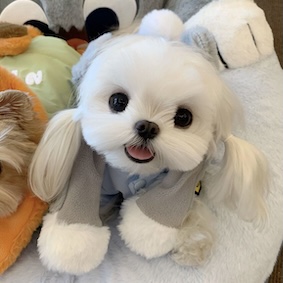
In the ever-evolving landscape of app development, selecting the right backend technology is paramount for success. This article delves into the key differences between Firebase and MongoDB, two prevalent solutions, while emphasizing their diverse use cases. With their distinct architectures, features, and functionalities, understanding how each platform operates can significantly influence your project’s effectiveness. Moreover, we will explore how these platforms can be integrated with tools like Chat2DB (opens in a new tab) to elevate your database management experience.
Understanding Firebase: A Powerful Backend-as-a-Service
Firebase (opens in a new tab) is a comprehensive Backend-as-a-Service (BaaS) platform developed by Google. It aims to simplify the complexities of app development by providing developers with a suite of tools to create high-quality applications quickly. Since its launch in 2011 as a real-time database, Firebase has evolved to include numerous features such as:
- Real-time Database: Firebase offers a NoSQL cloud database that facilitates real-time data syncing across all connected clients.
- Cloud Functions: This feature allows you to run backend code in response to events triggered by Firebase features or HTTPS requests.
- Authentication: Firebase streamlines user authentication with support for email/password, social media logins, and more.
- Hosting: It provides secure, fast, and reliable hosting for web applications.
Firebase seamlessly integrates with other Google services such as Google Analytics (opens in a new tab) and AdMob (opens in a new tab), enhancing its functionality for mobile app developers. The platform is well-suited for applications of varying sizes, from small projects to large-scale deployments, making it a versatile choice for developers.
Firebase Code Example
Here’s a simple example of using Firebase's real-time database:
// Initialize Firebase
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_APP.firebaseapp.com",
databaseURL: "https://YOUR_APP.firebaseio.com",
projectId: "YOUR_APP",
storageBucket: "YOUR_APP.appspot.com",
messagingSenderId: "YOUR_SENDER_ID",
appId: "YOUR_APP_ID"
};
firebase.initializeApp(firebaseConfig);
// Write data to the database
firebase.database().ref('users/').set({
username: "exampleUser",
email: "user@example.com"
});
// Read data from the database
firebase.database().ref('users/').on('value', (snapshot) => {
const data = snapshot.val();
console.log(data);
});
This example demonstrates how to initialize Firebase and perform basic read and write operations on the database.
Exploring MongoDB: A Leading NoSQL Database
MongoDB (opens in a new tab) is a popular NoSQL database known for its flexibility and scalability. Unlike traditional SQL databases, MongoDB employs a document-based storage model that allows for dynamic schemas. This means developers can easily manage large volumes of unstructured data, making it particularly useful for big data applications.
Key Features of MongoDB
- Document-Based Storage: MongoDB stores data in JSON-like documents, which allows for the representation of complex data structures without requiring a predefined schema.
- Horizontal Scaling: It supports sharding, which distributes data across multiple servers, enabling efficient handling of large-scale deployments.
- Powerful Querying Capabilities: MongoDB’s querying capabilities include ad hoc queries, indexing, and real-time aggregation, making it suitable for varied applications.
- Open Source: The open-source nature of MongoDB fosters community development and support, allowing for constant improvements and innovations.
MongoDB Code Example
Here’s a sample code snippet demonstrating how to interact with a MongoDB database using Node.js:
const { MongoClient } = require('mongodb');
// Connection URL
const url = 'mongodb://localhost:27017';
const client = new MongoClient(url);
// Database Name
const dbName = 'myDatabase';
async function main() {
// Connect the client to the server
await client.connect();
console.log('Connected to database');
const db = client.db(dbName);
const collection = db.collection('users');
// Insert a document
const insertResult = await collection.insertOne({ username: 'exampleUser', email: 'user@example.com' });
console.log('Inserted document:', insertResult.ops);
// Find a document
const findResult = await collection.findOne({ username: 'exampleUser' });
console.log('Found document:', findResult);
}
// Run the main function
main().catch(console.error).finally(() => client.close());
This snippet connects to a MongoDB database, inserts a document, and retrieves it, showcasing the simplicity of using MongoDB for data operations.
Key Differences Between Firebase and MongoDB
When comparing Firebase and MongoDB, it's essential to recognize their fundamental differences in structure, functionality, and use cases.
Feature | Firebase | MongoDB |
---|---|---|
Type | Backend-as-a-Service | NoSQL Database |
Data Model | Real-time Database (NoSQL) | Document-Based (JSON-like) |
Real-Time Sync | Yes | No, but can be implemented |
Scalability | Google Cloud infrastructure | Sharding and replication |
Authentication | Built-in support for user management | Requires additional setup |
Use Cases | Mobile apps, real-time applications | Big data, flexible schema applications |
Structural Differences
Firebase operates as a BaaS, handling various backend functionalities such as authentication and real-time data synchronization. In contrast, MongoDB functions as a NoSQL database that offers freedom in data modeling, allowing developers to define their schema dynamically.
Security Models
Firebase integrates seamlessly with Google Cloud Identity (opens in a new tab), providing robust security measures for user authentication. MongoDB offers its security features, including role-based access control, ensuring that sensitive data is adequately protected.
Scalability Strategies
Firebase leverages Google Cloud’s infrastructure for scalability, while MongoDB utilizes sharding and replication to distribute data across multiple servers. This distinction leads to different performance characteristics based on the application's requirements.
Pricing Models
Firebase operates on a pay-as-you-go pricing model, which can become costly for applications with high usage. Conversely, MongoDB’s open-source nature allows developers to set up and run their deployments, potentially reducing costs in the long run.
Use Cases for Firebase
Firebase excels in various scenarios, particularly those requiring real-time data updates. Here are some notable use cases:
- Chat Applications: The real-time database capabilities make Firebase ideal for applications that need live messaging features.
- Collaborative Tools: Tools requiring simultaneous updates from multiple users benefit from Firebase's synchronization features.
- Mobile App Development: Firebase’s suite of integrated services, such as analytics and crash reporting, streamlines mobile app creation.
- IoT Applications: Firebase’s real-time synchronization abilities are advantageous for IoT applications that rely on immediate data updates.
Use Cases for MongoDB
MongoDB is particularly useful in scenarios where flexibility and scalability are paramount. Some ideal use cases include:
- Big Data Analytics: Its ability to handle unstructured data makes MongoDB suitable for big data applications.
- Content Management Systems (CMS): The dynamic schema allows for efficient management of diverse data types and formats.
- E-commerce Platforms: MongoDB’s robust querying capabilities support applications with extensive product catalogs.
Integrating Firebase and MongoDB with Chat2DB
Chat2DB (opens in a new tab) is an AI-powered database visualization management tool designed to enhance your database management experience. Its features can bridge the functionality gaps between Firebase and MongoDB, allowing developers to leverage both platforms' strengths.
Enhanced Database Management
Chat2DB enables seamless data migration between Firebase and MongoDB, allowing developers to choose the best platform for their specific needs. It also facilitates monitoring and optimizing database performance, providing insights into resource usage.
AI-Powered Features
One of the standout features of Chat2DB is its AI capabilities. It offers natural language processing tools that help users generate SQL queries effortlessly, making database management more intuitive. Developers can leverage Chat2DB’s intelligent SQL editor to optimize their queries and improve performance, ensuring efficient data management.
Security and Authentication Management
Chat2DB can assist in managing security and authentication across both Firebase and MongoDB, ensuring that applications maintain high-security standards while providing easy access to authorized users.
Conclusion
In summary, both Firebase and MongoDB offer unique strengths tailored to different development needs. However, to maximize your database management experience, consider switching to Chat2DB. Its AI-driven features and capabilities provide a competitive edge over traditional tools, streamlining workflows and enhancing productivity.
FAQ
-
What is the primary difference between Firebase and MongoDB?
- Firebase is a Backend-as-a-Service platform, while MongoDB is a NoSQL database. Firebase focuses on real-time data sync and integrated services, whereas MongoDB offers document-based storage and flexible schema management.
-
Can Firebase and MongoDB be used together?
- Yes, developers can use both platforms in tandem, leveraging Firebase for real-time capabilities and MongoDB for flexible data handling. Tools like Chat2DB (opens in a new tab) can facilitate integration.
-
What are some common use cases for Firebase?
- Firebase is ideal for chat applications, collaborative tools, mobile app development, and IoT applications requiring real-time data synchronization.
-
Is MongoDB suitable for big data applications?
- Yes, MongoDB excels in handling large volumes of unstructured data, making it well-suited for big data analytics and applications.
-
How does Chat2DB enhance database management?
- Chat2DB provides AI-powered features for generating SQL queries, optimizing performance, and managing security across different database platforms, including Firebase and MongoDB.
By understanding the differences and use cases of Firebase and MongoDB, developers can make informed choices for their projects. For those looking to streamline their database management process, exploring Chat2DB (opens in a new tab) can be a game-changer.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!