How to Format Dates in MySQL: A Practical Guide for Beginners
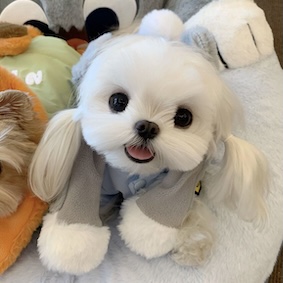
When working with databases, particularly MySQL, mastering date formatting is essential for effective data management. This guide covers various MySQL date formats, how to use the DATE_FORMAT()
function, handle time zones, and perform date arithmetic. Additionally, we will explore how tools like Chat2DB (opens in a new tab) can significantly enhance your experience with MySQL date management. From key formats such as DATE, DATETIME, and TIMESTAMP to practical examples and best practices, this article aims to provide a comprehensive overview for both beginners and experienced developers.
Understanding MySQL Date Formats
MySQL supports several date formats, including DATE, DATETIME, TIMESTAMP, TIME, and YEAR. Each format serves a unique purpose, and understanding these differences is crucial for effective database management.
Key MySQL Date Formats
Format | Description | Storage Size | Range |
---|---|---|---|
DATE | Stores a date value (YYYY-MM-DD) | 3 bytes | '1000-01-01' to '9999-12-31' |
DATETIME | Stores date and time (YYYY-MM-DD HH:MM:SS) | 8 bytes | '1000-01-01 00:00:00' to '9999-12-31 23:59:59' |
TIMESTAMP | Stores date and time, adjusting for time zones | 4 bytes | '1970-01-01 00:00:01' UTC to '2038-01-19 03:14:07' UTC |
TIME | Stores time (HH:MM:SS) | 3 bytes | '-838:59:59' to '838:59:59' |
YEAR | Stores year in a 2-digit or 4-digit format | 1 byte | 1901 to 2155 |
DATE is useful for storing dates without any time component, while DATETIME is ideal for applications requiring both date and time information. On the other hand, TIMESTAMP is particularly useful for tracking events across different time zones, as it automatically adjusts based on the server's time zone settings.
Default Display Formats
MySQL uses default display formats for these date types, but you can customize how dates are displayed using the DATE_FORMAT()
function. For instance, you can convert a date from the default format to a more user-friendly format:
SELECT DATE_FORMAT(NOW(), '%W, %M %d, %Y') AS formatted_date;
This example would output something like "Friday, March 10, 2023". Custom formatting is essential for applications where user experience is a priority.
Implications for Data Retrieval
Consistency in date formats is crucial for data integrity. When storing dates, it's important to choose a format that meets your application's needs. For example, using TIMESTAMP for events that require time zone awareness helps prevent data issues when retrieving records.
Using the DATE_FORMAT() Function
The DATE_FORMAT()
function in MySQL allows developers to format date values according to specific requirements. This function is invaluable for generating user-friendly date strings in application outputs.
Syntax and Usage
The basic syntax of the DATE_FORMAT()
function is as follows:
DATE_FORMAT(date, format)
Here are some common format specifiers you can use:
%Y
: Year (4 digits)%y
: Year (2 digits)%m
: Month (01 to 12)%M
: Month name (January to December)%d
: Day of the month (01 to 31)%H
: Hour (00 to 23)
Common Formatting Patterns
You can apply various formatting patterns using the DATE_FORMAT()
function. For example, to convert a date to 'MM-DD-YYYY', use:
SELECT DATE_FORMAT('2023-03-10', '%m-%d-%Y') AS formatted_date;
This would return "03-10-2023". Similarly, to format a date to 'YYYY/MM/DD':
SELECT DATE_FORMAT('2023-03-10', '%Y/%m/%d') AS formatted_date;
This flexibility allows developers to present dates in ways that align with user expectations and cultural norms.
Practical Examples
Here are some practical examples of using DATE_FORMAT()
in different scenarios:
-
Generating Reports: Formatting dates for report generation can make your outputs more readable.
SELECT employee_name, DATE_FORMAT(hire_date, '%M %d, %Y') AS hire_date FROM employees;
-
Web Applications: When retrieving data for display in a web application, formatted dates improve user experience.
SELECT post_title, DATE_FORMAT(post_date, '%W, %M %d, %Y') AS post_date FROM blog_posts;
Addressing Locale Issues
One potential pitfall when using DATE_FORMAT()
is locale issues. Different users may expect dates in various formats based on their regional settings. Ensure that your application accounts for these variations by allowing users to select their preferred date format.
Handling Time Zones in MySQL
Managing time zones in MySQL databases can be challenging, especially when dealing with global applications. Understanding the differences between DATETIME and TIMESTAMP is essential.
Storing Dates with Time Zone Awareness
- DATETIME does not store time zone information. It is simply a static representation of date and time.
- TIMESTAMP, on the other hand, stores date and time in UTC and converts it to the current time zone when retrieved.
Configuring Time Zone Tables
To handle time zones effectively, it's important to configure MySQL's time zone tables. You can do this using the following command:
mysql_tzinfo_to_sql /usr/share/zoneinfo | mysql -u root -p mysql
This command loads the time zone data into the database, enabling you to perform time zone conversions.
Using CONVERT_TZ()
The CONVERT_TZ()
function allows you to convert date values to different time zones. Here is an example:
SELECT CONVERT_TZ('2023-03-10 12:00:00', 'UTC', 'America/New_York') AS converted_time;
This function converts the UTC time to Eastern Standard Time, allowing you to accurately reflect the time zone of your users.
Working with Date Arithmetic
Performing date arithmetic is essential for various applications, such as calculating age or determining event durations. MySQL provides several functions to facilitate date calculations.
Key Functions for Date Arithmetic
- DATE_ADD(): Adds a specified time interval to a date.
- DATE_SUB(): Subtracts a specified time interval from a date.
- DATEDIFF(): Returns the difference in days between two dates.
- TIMESTAMPDIFF(): Returns the difference between two date values in specified time units.
Practical Examples
-
Calculating Age:
To calculate a user's age from their birthdate:
SELECT name, DATEDIFF(CURDATE(), birthdate) / 365 AS age FROM users;
-
Determining Days Until an Event:
To find out how many days remain until a specific event:
SELECT event_name, DATEDIFF(event_date, CURDATE()) AS days_until_event FROM events;
Understanding Nuances of Date Arithmetic
When working with date arithmetic, it's important to consider leap years and month boundaries. MySQL handles these cases accurately, but developers should be aware of potential pitfalls, such as forgetting to account for time components in calculations.
Using Chat2DB for MySQL Date Management
Chat2DB (opens in a new tab) is an AI-driven database visualization management tool that significantly enhances your experience with MySQL, particularly in managing date formats and related functions. By leveraging Chat2DB’s intuitive interface, developers can easily build queries involving complex date manipulations.
Features of Chat2DB
- Intuitive Query Builder: Simplifies the process of constructing SQL queries, including those involving date formatting and arithmetic.
- AI-Powered SQL Generation: Automatically generates SQL queries based on natural language input, making it accessible for users unfamiliar with SQL syntax.
- Date Management Capabilities: Offers advanced tools for managing date formats and time zones, streamlining your workflow.
Optimizing Date-Related Queries
Using Chat2DB, developers can visualize date data and generate comprehensive reports with minimal effort. The AI functionality allows for quick adjustments and optimizations, making it easier to manage date-related queries efficiently.
Common Pitfalls and Best Practices
When working with dates in MySQL, developers often encounter several common mistakes. Awareness of these pitfalls can help you avoid errors and achieve better results.
Avoiding Common Mistakes
- Incorrect Format Specifiers: Always double-check your format specifiers when using
DATE_FORMAT()
. An incorrect specifier can lead to unexpected output. - Neglecting Time Zones: Be cautious when using DATETIME for time-sensitive data. Opt for TIMESTAMP to ensure time zone adjustments are accounted for.
- Inconsistent Date Formats: Standardize date formats across your application to maintain data integrity.
Best Practices for Date Management
- Use UTC for Storage: Storing all date and time values in UTC helps eliminate confusion and ensures consistency.
- Thorough Testing: Validate date formats and calculations through rigorous testing to prevent runtime errors.
- Documentation: Keep clear documentation on the date formats and functions used in your applications for future reference.
By following these practices, you can enhance the reliability and performance of your applications that rely on date management.
FAQ
-
What is the difference between DATE and DATETIME in MySQL?
- DATE stores only date values, while DATETIME includes both date and time information.
-
How can I format dates in MySQL?
- You can use the
DATE_FORMAT()
function to format dates according to your requirements.
- You can use the
-
What is the best way to handle time zones in MySQL?
- Use TIMESTAMP for time zone awareness and configure MySQL's time zone tables for accurate conversions.
-
How can I calculate the difference between two dates in MySQL?
- You can use the
DATEDIFF()
function to find the difference in days between two dates.
- You can use the
-
How does Chat2DB help with MySQL date management?
- Chat2DB offers an intuitive interface and AI-powered features that simplify date formatting, arithmetic, and overall database management.
By utilizing the insights and tools presented in this guide, you can become proficient in managing MySQL date formats and improve your application's efficiency. Consider switching to Chat2DB (opens in a new tab) for an enhanced database experience that streamlines your date management tasks and provides powerful AI capabilities to assist you in your development workflow.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!