How Functional Dependency Shapes Database Design: A Comprehensive Analysis
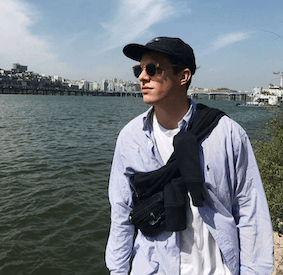
Functional dependency is a fundamental concept in database management systems (DBMS) that is essential for maintaining data integrity and consistency. It describes a relationship between two sets of attributes in a database table, where one attribute (or a set of attributes) uniquely determines another attribute. In this article, we will explore the key aspects of functional dependency, its significance in database design and normalization processes, and provide detailed code examples to illustrate these concepts.
What is Functional Dependency in DBMS?
In the realm of DBMS, functional dependency is represented as X → Y, where X and Y are attributes or sets of attributes. This notation signifies that if two tuples (records) have the same value for attribute X, they must also share the same value for attribute Y. For example, consider a student database table where the student ID uniquely identifies the student name:
CREATE TABLE Students (
student_id INT PRIMARY KEY,
student_name VARCHAR(100)
);
-- Functional Dependency Example
-- student_id → student_name
INSERT INTO Students (student_id, student_name) VALUES (1, 'John Doe'), (2, 'Jane Smith');
In this case, knowing the student_id
allows us to determine the corresponding student_name
.
The Importance of Functional Dependency in DBMS
Grasping functional dependencies is crucial for several reasons:
-
Data Integrity: Establishing functional dependencies helps maintain the accuracy and reliability of data by ensuring consistent relationships between attributes.
-
Reduction of Redundancy: Functional dependencies assist in identifying and minimizing redundancy in database design. Attributes that depend on one another can often be normalized into separate tables, reducing data duplication.
-
Normalization Process: Functional dependencies are pivotal in normalization, which organizes a database to minimize redundancy and dependency. This process involves dividing large tables into smaller, manageable ones without losing information.
Simple Examples of Functional Dependencies in DBMS
To clarify the concept further, here are some straightforward examples of functional dependencies:
- In an employee database:
CREATE TABLE Employees (
employee_id INT PRIMARY KEY,
employee_name VARCHAR(100)
);
-- Functional Dependency Example
-- employee_id → employee_name
INSERT INTO Employees (employee_id, employee_name) VALUES (1, 'Alice Johnson'), (2, 'Bob Brown');
- In an order database:
CREATE TABLE Orders (
order_id INT PRIMARY KEY,
customer_id INT
);
-- Functional Dependency Example
-- order_id → customer_id
INSERT INTO Orders (order_id, customer_id) VALUES (101, 1), (102, 2);
These examples illustrate how one attribute determines another, forming the basis for establishing relationships within the database.
The Role of Functional Dependency in Database Normalization
Database normalization is a critical process that organizes data to reduce redundancy and enhance data integrity. Functional dependency serves as the foundation for this process, guiding the transition through various normal forms.
Normal Forms and Functional Dependency
Normalization consists of several stages, known as normal forms (NF), including:
-
First Normal Form (1NF): A table is in 1NF if it contains only atomic values and each entry is unique. Functional dependencies help ensure that no repeating groups exist within the table.
-
Second Normal Form (2NF): A table is in 2NF if it is in 1NF and all non-key attributes are fully functionally dependent on the primary key, meaning no partial dependency exists.
-
Third Normal Form (3NF): A table is in 3NF if it is in 2NF and there are no transitive dependencies, removing any attributes that only depend on other non-key attributes.
Example of Normalization Using Functional Dependency
Let’s consider a simple table of student grades:
CREATE TABLE StudentGrades (
student_id INT,
student_name VARCHAR(100),
course VARCHAR(50),
course_grade CHAR(1)
);
-- Sample Data
INSERT INTO StudentGrades (student_id, student_name, course, course_grade) VALUES
(1, 'John Doe', 'Math', 'A'),
(1, 'John Doe', 'Science', 'B'),
(2, 'Jane Smith', 'Math', 'A');
To normalize this table, we identify the functional dependencies:
student_id → student_name
student_id, course → course_grade
We can separate the data into two tables to achieve normalization:
Student Table:
CREATE TABLE Students (
student_id INT PRIMARY KEY,
student_name VARCHAR(100)
);
INSERT INTO Students (student_id, student_name) VALUES
(1, 'John Doe'),
(2, 'Jane Smith');
Grades Table:
CREATE TABLE Grades (
student_id INT,
course VARCHAR(50),
course_grade CHAR(1),
PRIMARY KEY (student_id, course),
FOREIGN KEY (student_id) REFERENCES Students(student_id)
);
INSERT INTO Grades (student_id, course, course_grade) VALUES
(1, 'Math', 'A'),
(1, 'Science', 'B'),
(2, 'Math', 'A');
This normalization process effectively reduces redundancy while preserving data integrity.
Advanced Functional Dependency Concepts in DBMS
As we delve deeper into functional dependencies, we encounter more complex concepts, such as multivalued dependencies and transitive dependencies.
Multivalued Dependencies
A multivalued dependency occurs when one attribute determines multiple values of another attribute. For example, in a table of employees where each employee can have multiple phone numbers, we might have:
CREATE TABLE EmployeePhones (
employee_id INT,
phone_number VARCHAR(15)
);
-- Functional Dependency Example
-- employee_id →→ phone_number
INSERT INTO EmployeePhones (employee_id, phone_number) VALUES
(1, '123-456-7890'),
(1, '098-765-4321');
This indicates that an employee can have multiple phone numbers.
Transitive Dependencies
A transitive dependency exists when an attribute depends on another attribute indirectly through a third attribute. For example:
CREATE TABLE CourseInfo (
course_id INT,
course_name VARCHAR(100),
instructor_name VARCHAR(100)
);
-- Functional Dependency Example
-- course_id → instructor_name and instructor_name → department implies course_id → department
INSERT INTO CourseInfo (course_id, course_name, instructor_name) VALUES
(101, 'Math', 'Prof. Smith'),
(102, 'Science', 'Dr. Johnson');
Understanding transitive dependencies is crucial for achieving higher normal forms, particularly in the transition from 2NF to 3NF.
Closure of a Set of Attributes
The closure of a set of attributes is a fundamental concept in functional dependency analysis. It represents all attributes that can be functionally determined by a given set of attributes. For example, given attributes A, B, and C in a table with functional dependencies:
-- Given Functional Dependencies
-- A → B
-- B → C
The closure of A would be (A, B, C), as knowing A allows us to determine both B and C.
Challenges in Managing Functional Dependencies in DBMS
Managing functional dependencies in large-scale databases presents several challenges, such as evolving business requirements and the complexity of distributed databases.
Impact of Evolving Business Requirements
As business requirements change, existing functional dependencies may need to be reassessed. This can lead to potential complications in maintaining data integrity and consistency.
Tools and Techniques for Managing Functional Dependencies
Automating the detection and management of functional dependencies can significantly alleviate the challenges faced by developers. Tools like Chat2DB (opens in a new tab) offer valuable features that assist in identifying and managing functional dependencies efficiently.
Practical Applications of Functional Dependencies in DBMS
Understanding functional dependencies has direct applications in database design, optimization, and query performance. Here are some practical scenarios:
Query Optimization and Indexing
By analyzing functional dependencies, developers can optimize queries and create effective indexing strategies. For instance, knowing that student_id
determines student_name
allows for efficient indexing on student_id
, speeding up query performance:
CREATE INDEX idx_student_id ON Students(student_id);
Continuous Integration and Deployment
Integrating functional dependency analysis into continuous integration and deployment pipelines can enhance database management. By consistently analyzing and maintaining functional dependencies, organizations can avoid potential issues during deployment.
Conclusion
Functional dependency is a fundamental aspect of database management that ensures data integrity, reduces redundancy, and facilitates effective normalization processes. Understanding and managing functional dependencies is crucial for developers as they design and maintain complex databases.
FAQs
-
What is functional dependency in DBMS? Functional dependency is a relationship between attributes in a database table that describes how one attribute uniquely determines another.
-
Why is functional dependency important? It ensures data integrity, minimizes redundancy, and guides the normalization process in database design.
-
What are the different normal forms? The normal forms include First Normal Form (1NF), Second Normal Form (2NF), and Third Normal Form (3NF), each addressing different types of dependencies.
-
What is transitive dependency? A transitive dependency occurs when one attribute depends on another attribute indirectly through a third attribute.
-
How can Chat2DB assist in managing functional dependencies? Chat2DB provides tools for visualizing and analyzing functional dependencies, making it easier for developers to maintain data integrity and optimize database performance.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!