How to Efficiently Generate UUIDs in Python: A Comprehensive Guide for Developers
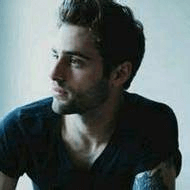
Understanding UUIDs: What They Are and Why They Matter
Universally Unique Identifiers, or UUIDs, serve a critical role in software development. They provide a mechanism for uniquely identifying information across computer systems, making them invaluable for database indexing, distributed systems, and API development. Unlike traditional numeric identifiers, UUIDs are designed to be globally unique, significantly minimizing the risk of collision.
UUIDs come in five versions, each tailored for specific use cases. For instance, UUID version 1 is time-based and includes both the timestamp and the MAC address of the generating node, making it suitable for scenarios that require temporal uniqueness. Conversely, UUID version 4 is randomly generated, which provides simplicity and anonymity. Understanding the differences and appropriate usage of each version is crucial for developers looking to effectively implement UUIDs.
In database systems, UUIDs facilitate horizontal scaling, as they can be generated independently across multiple nodes without the need for coordination. This is particularly beneficial in distributed database architectures and microservices. Additionally, UUIDs enhance security and data privacy, as they can obscure the ordering of records, mitigating risks associated with sequential identifiers.
Setting Up Your Python Environment for Efficient UUID Generation
To efficiently generate UUIDs in Python, you need to prepare your development environment. Follow these steps to get started:
-
Install Python: Ensure that you have Python 3.x installed on your machine. You can download it from the official Python website (opens in a new tab).
-
Create a Virtual Environment: It's best practice to use a virtual environment to manage your project dependencies. You can create one using the following command:
python -m venv myenv
-
Activate the Virtual Environment:
- On Windows:
myenv\Scripts\activate
- On macOS/Linux:
source myenv/bin/activate
- On Windows:
-
Install Required Libraries: While Python's built-in
uuid
module suffices for UUID generation, installing additional libraries can enhance your capabilities. For example, consider libraries for data manipulation or web frameworks like Flask or Django.
Now that your environment is set, you can leverage Python's uuid
module for generating UUIDs efficiently.
Generating UUIDs in Python: Step-by-Step Instructions
Python’s uuid
module provides various methods for generating UUIDs. Below are the methods you can use, along with code examples for each.
Using uuid1()
This method generates a UUID based on the host's MAC address and the current timestamp.
import uuid
uuid1 = uuid.uuid1()
print("UUID1:", uuid1)
Using uuid3()
UUID version 3 generates a UUID based on the MD5 hash of a namespace identifier and a name.
namespace = uuid.NAMESPACE_DNS
name = 'example.com'
uuid3 = uuid.uuid3(namespace, name)
print("UUID3:", uuid3)
Using uuid4()
This method generates a random UUID. It is widely used due to its simplicity and randomness.
uuid4 = uuid.uuid4()
print("UUID4:", uuid4)
Using uuid5()
Similar to uuid3()
, but it uses the SHA-1 hash instead.
namespace = uuid.NAMESPACE_DNS
name = 'example.com'
uuid5 = uuid.uuid5(namespace, name)
print("UUID5:", uuid5)
Each of these methods has its own advantages and use cases, which are essential for effective UUID generation.
UUID Version | Description | Use Case |
---|---|---|
uuid1() | Time-based with MAC address | Temporal uniqueness |
uuid3() | MD5 hash-based | Namespaced identifier |
uuid4() | Randomly generated | General-purpose use |
uuid5() | SHA-1 hash-based | Namespaced identifier with SHA-1 |
Optimizing Performance: Efficient UUID Generation Techniques
When generating UUIDs, performance can be critical, especially in high-load applications. Here are some techniques to optimize UUID generation:
-
Bulk UUID Generation: If you know you will need a set of UUIDs, consider generating them in bulk to reduce the overhead at runtime.
def generate_bulk_uuids(count): return [uuid.uuid4() for _ in range(count)] bulk_uuids = generate_bulk_uuids(1000) print("Bulk UUIDs generated:", bulk_uuids)
-
Using Threads or Multiprocessing: For applications requiring high performance, consider using threading or multiprocessing to parallelize UUID generation.
import threading def generate_uuid(): return uuid.uuid4() threads = [threading.Thread(target=generate_uuid) for _ in range(10)] for thread in threads: thread.start() for thread in threads: thread.join()
-
Evaluate UUID Version: Choosing the right UUID version can significantly impact performance. For example,
uuid4()
is generally faster thanuuid1()
due to the absence of timestamp calculations.
Integrating UUIDs into Your Application Architecture
UUIDs can be seamlessly integrated into various layers of application architecture. Here are best practices for their implementation:
Primary Keys in Relational Databases
Using UUIDs as primary keys can enhance the performance of distributed databases. When using UUIDs, ensure that your indexing strategies are optimized.
CREATE TABLE users (
id UUID PRIMARY KEY,
name TEXT
);
NoSQL Databases
In NoSQL databases like MongoDB, UUIDs can serve as unique identifiers for documents. This is particularly useful in applications with distributed data storage.
from pymongo import MongoClient
import uuid
client = MongoClient('mongodb://localhost:27017/')
db = client['mydatabase']
collection = db['users']
collection.insert_one({'_id': uuid.uuid4(), 'name': 'Alice'})
RESTful APIs
In RESTful APIs, UUIDs can be used to uniquely identify resources. This practice enhances data consistency and provides a clear structure for request handling.
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/users/<uuid:user_id>')
def get_user(user_id):
return jsonify({'user_id': str(user_id)})
if __name__ == '__main__':
app.run()
Debugging and Troubleshooting UUID-Related Issues
Developers may encounter several challenges when working with UUIDs. Here are some common issues and their solutions:
Collision Detection
While UUIDs are designed to be unique, collisions can occur in rare cases. Implement logging to track UUID generation and handle collisions gracefully.
Validating UUID Formats
Ensure that the UUIDs conform to standard formats. You can validate UUIDs using regular expressions.
import re
def is_valid_uuid(uuid_to_test, version=4):
regex = r'^[0-9a-f]{8}-[0-9a-f]{4}-' + str(version) + r'[0-9a-f]{3}-[89ab][0-9a-f]{3}-[0-9a-f]{12}$'
return re.match(regex, str(uuid_to_test)) is not None
Logging and Monitoring
In production environments, implement logging for UUID-related operations to facilitate troubleshooting and monitoring.
Exploring Advanced UUID Use Cases and Innovations
UUIDs have advanced applications in various domains:
-
Blockchain Technology: UUIDs can be utilized for generating transaction IDs, ensuring each transaction is uniquely identifiable.
-
IoT Devices: In the Internet of Things (IoT), UUIDs help uniquely identify devices, which is vital for communication and data tracking.
-
API Versioning: UUIDs can be used in API versioning, enabling developers to manage changes and maintain compatibility.
-
Data Analytics: UUIDs play a significant role in tracking events and correlating data across different datasets.
-
Emerging Technologies: The future of UUIDs may include adaptations for quantum-safe identifiers, enhancing security in data-intensive applications.
Incorporating tools like Chat2DB can streamline database management and enhance the use of UUIDs through AI-driven features. Chat2DB offers natural language SQL generation and intelligent data visualization, making it easier to manage and analyze data effectively.
FAQ
-
What is a UUID?
- A UUID is a universally unique identifier used to uniquely identify information in computer systems.
-
How do I generate a UUID in Python?
- You can generate a UUID in Python using the built-in
uuid
module, with methods likeuuid1()
,uuid3()
,uuid4()
, anduuid5()
.
- You can generate a UUID in Python using the built-in
-
Why are UUIDs preferred over traditional identifiers?
- UUIDs minimize the risk of collisions and are suitable for distributed systems, making them ideal for modern applications.
-
Can UUIDs be generated in bulk?
- Yes, you can generate UUIDs in bulk to improve performance and reduce runtime overhead.
-
What are the applications of UUIDs?
- UUIDs are used in databases, APIs, IoT devices, blockchain technology, and data analytics, among others.
For developers looking for an efficient way to manage databases and utilize UUIDs effectively, consider using Chat2DB. Its AI functionalities can significantly enhance your database management experience, providing features that outshine alternatives like DBeaver, MySQL Workbench, and DataGrip. With Chat2DB, you can generate SQL queries using natural language and visualize data intuitively, streamlining your workflow and improving productivity.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!