Transforming Natural Language into SQL: A Comprehensive Guide to Text-to-SQL Techniques
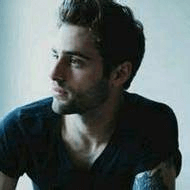
Understanding Text-to-SQL Systems: A Deep Dive into Natural Language Processing for Database Management
As businesses increasingly rely on data-driven decisions, the demand for accessible text-to-SQL systems has significantly increased. These systems empower users to convert natural language queries into SQL commands without requiring extensive SQL knowledge. This transition from human language to structured query language involves several critical components, including language parsing, query generation, and result interpretation.
Key Components of Text-to-SQL Systems
Component | Description |
---|---|
Language Parsing | Initial breakdown of natural language input into parts using tokenization. |
Query Generation | Translating parsed data into SQL commands through rule-based or ML models. |
Result Interpretation | Presenting SQL execution results back to users in an understandable format. |
-
Language Parsing: This is the initial step where natural language input is broken down into its constituent parts. Techniques such as tokenization and part-of-speech tagging are essential here. Libraries like spaCy (opens in a new tab) and NLTK (opens in a new tab) are commonly used for this task.
-
Query Generation: After parsing, the next step is to translate the parsed data into SQL commands. This can be achieved through rule-based systems or more advanced machine learning models.
-
Result Interpretation: Finally, once the SQL command is executed, the results need to be interpreted and presented back to the user in a comprehensible format.
Challenges in Text-to-SQL Systems
Despite their advantages, text-to-SQL systems face several challenges:
-
Ambiguous Queries: Natural language can be vague, leading to multiple interpretations. Systems must be capable of context understanding to generate accurate SQL commands.
-
Complex Queries: Handling nested queries and complex joins can be difficult. Advanced techniques like semantic parsing are crucial for these scenarios.
-
Domain Adaptation: Different industries may use specialized terminologies, making it challenging for a single text-to-SQL system to adapt across various domains.
The implementation of AI and machine learning techniques is essential for overcoming these challenges. By leveraging algorithms that can learn from data, text-to-SQL systems can improve their accuracy and efficiency over time.
Key Techniques in Text-to-SQL Conversion
Transforming natural language queries into SQL requires various techniques. Here are some of the most effective methods:
Rule-Based Approaches
Rule-based systems utilize predefined rules to map natural language expressions to SQL syntax. While they can be effective for simple queries, they often struggle with more complex requests.
Machine Learning-Based Methods
Machine learning techniques, particularly supervised learning, have gained prominence in text-to-SQL systems. These systems are trained on datasets containing pairs of natural language queries and corresponding SQL commands.
Deep Learning Models
Recent advancements in deep learning, particularly with models like BERT (opens in a new tab) and GPT (opens in a new tab), have revolutionized the field of natural language processing. These models can understand context better and generate more accurate SQL queries.
Semantic Parsing
Semantic parsing plays a critical role in understanding the meaning behind natural language queries. It involves the creation of a structured representation that can be directly mapped to SQL commands.
Reinforcement Learning
Reinforcement learning techniques can optimize query generation by rewarding systems for producing accurate SQL. This approach allows continual improvement of the text-to-SQL conversion process.
Hybrid Approaches
Combining rule-based and machine learning techniques can yield better results. This hybrid approach leverages the strengths of both methods to improve accuracy and flexibility.
Example Code Snippet
Here is a simple Python code snippet that demonstrates how to use a rule-based approach to convert a natural language query into SQL:
def text_to_sql(query):
if "select" in query.lower() and "from" in query.lower():
# Extract table name and columns
columns = query.split("select")[1].split("from")[0].strip()
table = query.split("from")[1].strip()
return f"SELECT {columns} FROM {table};"
else:
return "Invalid query"
# Example usage
nl_query = "SELECT name FROM users"
sql_query = text_to_sql(nl_query)
print(sql_query) # Output: SELECT name FROM users;
Challenges and Solutions in Text-to-SQL Systems
Handling Ambiguous Queries
Ambiguity in natural language can lead to misinterpretations. To address this, systems can be designed to ask clarifying questions, enhancing user interaction and improving query accuracy.
Generating Efficient SQL Queries
The goal should not only be to create functional SQL queries but also to ensure they are optimized for performance. Techniques like query rewriting and optimization algorithms can help in this regard.
Domain Adaptation
To improve domain adaptation, developers can create specialized models trained on industry-specific datasets. This approach allows the system to understand unique terminologies and structures relevant to different fields.
Improving Error Detection
Error detection mechanisms can be integrated to identify and correct common mistakes in generated SQL commands. This helps maintain the integrity of database operations.
By implementing these solutions, developers can enhance the robustness of text-to-SQL systems.
Implementing Text-to-SQL Systems: Tools and Frameworks
There are various tools and frameworks available for developing text-to-SQL systems. One notable tool is Chat2DB (opens in a new tab), which offers a user-friendly interface for integrating natural language processing with SQL databases.
Advantages of Chat2DB
-
AI-Powered SQL Generation: Chat2DB utilizes advanced AI techniques to convert natural language into SQL queries effortlessly. This reduces the learning curve for users unfamiliar with SQL.
-
Natural Language Data Analysis: Users can perform data analysis simply by asking questions in natural language. The system translates these inquiries into SQL commands and provides visual representations of the results.
-
Support for Multiple Databases: Chat2DB supports over 24 database systems, making it versatile for different applications.
Open-Source Libraries
Developers can leverage libraries like sqlparse (opens in a new tab) for SQL parsing and manipulation. This can improve the syntactical analysis of generated queries.
Machine Learning Frameworks
Frameworks such as TensorFlow (opens in a new tab) and PyTorch (opens in a new tab) are essential for training and deploying machine learning models that enhance text-to-SQL capabilities.
Cloud Services
Utilizing cloud services like AWS and Google Cloud can help scale text-to-SQL solutions efficiently, providing the necessary computational resources for complex queries.
Evaluating the Performance of Text-to-SQL Systems
Performance evaluation is crucial for ensuring the effectiveness of text-to-SQL systems. Here are some methodologies and metrics used in this process:
Accuracy Metrics
Metrics such as precision, recall, and F1-score are vital for assessing the quality of SQL generation. These metrics help determine how well the system translates natural language into accurate SQL commands.
Benchmark Datasets
Datasets like Spider (opens in a new tab) and WikiSQL (opens in a new tab) serve as benchmarks for comparing the performance of different text-to-SQL models.
User Studies
Conducting user studies can provide insights into the usability and effectiveness of text-to-SQL systems in real-world scenarios. Feedback from users can guide future improvements.
Error Analysis
Performing error analysis helps identify common failure modes in text-to-SQL systems. This understanding can lead to targeted improvements in the models.
Continuous Monitoring
Implementing continuous monitoring and feedback loops can help maintain system performance over time, ensuring that the system adapts to changing datasets and user needs.
Future Directions and Innovations in Text-to-SQL
The development of text-to-SQL systems is an evolving field, with several emerging trends:
Advanced AI Technologies
Incorporating technologies like federated learning and edge computing can improve scalability and privacy in text-to-SQL systems.
Multimodal Data Processing
Allowing systems to process queries via text, voice, or visual inputs can enhance user interaction and accessibility.
Real-Time Adaptive Systems
Future systems may incorporate real-time learning capabilities, enabling them to adapt and improve based on user interactions.
Explainability
As AI systems become more complex, ensuring that users understand how their queries translate into SQL commands will be crucial for building trust.
Blockchain Technology
Utilizing blockchain for data integrity and security in text-to-SQL interactions is a promising avenue for future exploration.
The role of collaboration between industry and academia will be vital in advancing research and development in text-to-SQL technologies.
FAQ
-
What is a text-to-SQL system? Text-to-SQL systems convert natural language queries into SQL commands, enabling users to interact with databases without needing extensive SQL knowledge.
-
How does Chat2DB improve database management? Chat2DB leverages AI technology to simplify database interactions, allowing users to generate SQL queries from natural language and visualize data analysis results effectively.
-
What are the challenges faced by text-to-SQL systems? Common challenges include handling ambiguous queries, generating efficient SQL commands, and domain adaptation across various industries.
-
How can I evaluate the performance of a text-to-SQL system? Performance can be evaluated using accuracy metrics, benchmark datasets, user studies, and error analysis.
-
What are the future trends in text-to-SQL technology? Future trends include integrating advanced AI technologies, multimodal data processing, real-time adaptive systems, and enhancing explainability and security through blockchain technology.
For those looking to streamline their database management and enhance their SQL query generation, consider switching to Chat2DB (opens in a new tab), an innovative solution that combines AI capabilities with user-friendly design, surpassing traditional tools like DBeaver, MySQL Workbench, and DataGrip.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!