How to Connect to MySQL: A Comprehensive Guide for Beginners
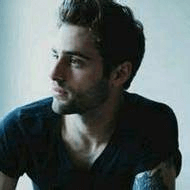
Understanding MySQL and Its Importance in Web Development
MySQL is a widely used open-source relational database management system (RDBMS) that plays a crucial role in modern web development and data storage solutions. Its importance stems from its ability to efficiently store, retrieve, and manage data in a structured manner. MySQL powers millions of applications and websites, ranging from small projects to large enterprise systems, due to its scalability, security, and robust transactional support.
In today's data-driven environment, MySQL is an indispensable tool for developers and data professionals. Its seamless integration with various programming languages like PHP, Java, and Python makes it a versatile choice for developers. Organizations across industries, from e-commerce to healthcare and finance, rely on MySQL for its performance and reliability in managing data.
MySQL Architecture
To effectively connect to MySQL, it’s essential to understand its architecture. The key components include:
Component | Description |
---|---|
MySQL Server | The central part that handles database operations. |
Client | The interface through which users interact with the server. |
Storage Engines | Different engines manage how data is stored and retrieved, each with distinct advantages. |
Key terms in MySQL include:
- Tables: Structures that hold data organized in rows and columns.
- Queries: Instructions written in SQL (Structured Query Language) to interact with the database.
- SQL: The language used to create, modify, and manage database data.
Setting Up Your Environment to Connect to MySQL
Before diving into connecting to MySQL, it’s crucial to set up your development environment. This process involves downloading and installing MySQL, which varies slightly across operating systems like Windows, macOS, and Linux.
Download and Install MySQL
- Visit the MySQL Downloads page (opens in a new tab) to download the latest version.
- Select your operating system and follow the installation instructions provided for your specific environment.
Setting a Secure Root Password
During installation, setting a secure root password is critical to protect your database from unauthorized access. Ensure that you store this password securely, as you will need it to connect to MySQL later.
Environment Variables and PATH Settings
After installing MySQL, you may need to configure environment variables to enable command-line access. This involves adding the MySQL bin directory to your system PATH, allowing you to run MySQL commands from any command prompt or terminal window.
Graphical User Interfaces (GUIs)
For users who prefer a visual approach, utilizing a GUI can simplify database management. Tools like MySQL Workbench provide user-friendly interfaces for managing databases and running queries. However, for a more advanced experience, consider exploring Chat2DB (opens in a new tab), which combines database management with AI capabilities, significantly enhancing your workflow.
Establishing a Connection to MySQL
Now that your environment is set up, let’s explore how to connect to a MySQL database. This section provides a step-by-step guide for establishing a connection using the MySQL command-line client.
Using the MySQL Command-Line Client
To connect to MySQL, open your command prompt or terminal and use the following syntax:
mysql -h host -u username -p
-h host
: Specify the hostname or IP address of your MySQL server (default islocalhost
).-u username
: Your MySQL username (commonlyroot
).-p
: Prompts for your password.
Example
Here’s how you might connect to your local MySQL server:
mysql -h localhost -u root -p
After entering your password, you should see the MySQL prompt, indicating a successful connection.
Common Connection Errors
While connecting to MySQL, you may encounter errors. Here are some common issues and tips for troubleshooting:
- Access denied: Ensure that the username and password are correct, and the user has the necessary permissions.
- Could not connect: Verify that the MySQL service is running and that there are no firewall restrictions blocking the connection.
Secure Connections
For enhanced security, using SSL/TLS for your MySQL connections is critical. This encrypts the data transmitted between your client and the server, safeguarding sensitive information.
Additionally, tools like Chat2DB (opens in a new tab) simplify managing database connections and enhance security features.
Executing Basic SQL Commands in MySQL
Once connected to MySQL, you can start executing SQL commands. This section covers fundamental commands used to interact with your database.
Creating, Modifying, and Dropping Tables
Creating a table involves defining its structure. Here’s an example SQL statement to create a simple table:
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(255) NOT NULL,
email VARCHAR(255) NOT NULL UNIQUE,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
To modify an existing table, use the ALTER TABLE
command. Example:
ALTER TABLE users ADD COLUMN last_login TIMESTAMP;
To drop a table, use:
DROP TABLE users;
Basic SQL Statements
Here are some essential SQL commands for interacting with database tables:
- SELECT: Retrieve data from a table.
SELECT * FROM users;
- INSERT: Add new records to a table.
INSERT INTO users (username, email) VALUES ('john_doe', 'john@example.com');
- UPDATE: Modify existing records.
UPDATE users SET email = 'john.doe@example.com' WHERE username = 'john_doe';
- DELETE: Remove records from a table.
DELETE FROM users WHERE username = 'john_doe';
Data Types and Constraints
Understanding data types is crucial for defining table schemas. MySQL supports various data types, including:
- INT: Integer values.
- VARCHAR(n): Variable-length strings with a maximum length of
n
. - TIMESTAMP: Date and time values.
Additionally, constraints like PRIMARY KEY
and FOREIGN KEY
help maintain data integrity by establishing relationships between tables.
Managing MySQL Databases Effectively
Effective database management is essential for maintaining data integrity and performance. This section discusses key tasks involved in managing MySQL databases.
Creating and Switching Databases
You can create a new database using the following command:
CREATE DATABASE my_database;
To switch between databases, use the USE
command:
USE my_database;
Database Backup Strategies
Regular backups are vital for data recovery. You can use the mysqldump
command to export your database:
mysqldump -u username -p my_database > backup.sql
This command creates a backup file named backup.sql
, which you can restore later if needed.
Transaction Management
MySQL supports transactions, allowing you to execute a series of operations safely. Use the following commands:
START TRANSACTION;
-- Your SQL commands here
COMMIT; -- or ROLLBACK; to undo changes
User Management
Managing users and permissions is crucial for database security. You can create a new user with specific privileges:
CREATE USER 'new_user'@'localhost' IDENTIFIED BY 'password';
GRANT ALL PRIVILEGES ON my_database.* TO 'new_user'@'localhost';
Regularly review user permissions to ensure security.
Integrating MySQL with Applications
MySQL can be easily integrated with various programming languages and frameworks, making it a flexible choice for developers.
Connecting MySQL with Python
Using Python, connect to MySQL with the mysql-connector
library:
import mysql.connector
connection = mysql.connector.connect(
host='localhost',
user='username',
password='password',
database='my_database'
)
cursor = connection.cursor()
cursor.execute("SELECT * FROM users")
for row in cursor.fetchall():
print(row)
connection.close()
Connecting MySQL with Java
In Java, use JDBC to connect to MySQL:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class MySQLConnection {
public static void main(String[] args) {
try {
Connection connection = DriverManager.getConnection(
"jdbc:mysql://localhost:3306/my_database", "username", "password");
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM users");
while (resultSet.next()) {
System.out.println(resultSet.getString("username"));
}
connection.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Best Practices for Managing Connections
Efficiently managing database connections is crucial. Implementing connection pooling can enhance performance by reusing existing connections instead of creating new ones for each request.
For developers looking to simplify their database management experience, Chat2DB (opens in a new tab) offers advanced features powered by AI, making it easier to visualize and manage your database connections.
Advanced MySQL Features and Optimization Techniques
MySQL includes several advanced features that can significantly enhance database performance and capabilities.
Indexing Strategies
Indexes are crucial for optimizing query performance. When you create an index on a column, MySQL can quickly locate rows based on that column. Here’s how to create an index:
CREATE INDEX idx_username ON users (username);
Stored Procedures and Triggers
Stored procedures allow you to execute a set of SQL commands as a single unit, while triggers automatically execute commands based on specific events (like inserts or updates).
Partitioning
For large datasets, partitioning can improve performance by splitting a table into manageable pieces. This way, MySQL can efficiently query only the relevant partitions.
Replication and Clustering
MySQL supports replication, allowing you to copy data from one server to another for redundancy and load balancing. Clustering provides high availability, ensuring your database remains accessible even during failures.
Performance Tuning Tips
To optimize MySQL performance, consider the following tips:
- Analyze slow queries using the slow query log.
- Use EXPLAIN to understand how MySQL executes your queries.
- Regularly monitor performance metrics and adjust configurations accordingly.
Tools like MySQL Enterprise Monitor (opens in a new tab) can help with proactive database management.
Frequently Asked Questions (FAQ)
-
What is MySQL? MySQL is an open-source relational database management system (RDBMS) widely used for data storage and management.
-
How do I connect to MySQL? You can connect to MySQL using the command-line client or a graphical user interface like Chat2DB, which simplifies the process.
-
What are common SQL commands? Common SQL commands include SELECT, INSERT, UPDATE, and DELETE, used to interact with database tables.
-
How can I back up my MySQL database? You can back up your database using the
mysqldump
command, which exports your database to a file. -
What is Chat2DB? Chat2DB is an AI-powered database management tool that offers advanced features for visualizing and managing databases, making it easier for developers and data professionals alike.
For a more efficient and powerful database management experience, consider switching to Chat2DB (opens in a new tab). With its AI capabilities, Chat2DB streamlines the entire process of connecting to MySQL, executing commands, and managing data, setting it apart from other tools like MySQL Workbench or DBeaver. By using Chat2DB, you can enhance your productivity and focus on what truly matters—building innovative applications and solutions.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!