How to Count Distinct Values in MySQL Efficiently: A Comprehensive Guide
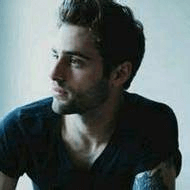
Counting distinct values in MySQL is a fundamental operation that every database developer should master. This guide will delve into various methods, optimizations, and advanced techniques to efficiently perform this operation in MySQL, a widely-used relational database management system.
Understanding Distinct Values in MySQL
In the context of MySQL, distinct values refer to the unique entries present in a specific column of a database table. Counting these distinct values is crucial for multiple reasons, including data analysis, reporting, and ensuring data integrity. For instance, when analyzing customer data, businesses often need to determine how many unique customers made purchases within a given timeframe. Understanding the distinct values can provide insights into customer behavior and help with inventory management.
MySQL plays a pivotal role in managing large datasets thanks to its robust architecture. Its capabilities include the ability to execute complex queries, support for various data types, and an extensive set of built-in functions. With this foundational knowledge, we can now explore various techniques and optimizations for counting distinct values efficiently.
Basic Methods for Counting Distinct Values in MySQL
The simplest approach to counting distinct values involves the use of the SELECT DISTINCT
statement along with the COUNT()
function. The basic syntax looks like this:
SELECT COUNT(DISTINCT column_name) FROM table_name;
For example, if you have a table customers
and you want to count the distinct cities customers are from, you could use:
SELECT COUNT(DISTINCT city) FROM customers;
However, it's essential to understand the limitations of this method, particularly regarding performance when dealing with large datasets. A common pitfall is forgetting to specify the correct column or misunderstanding the output of the query.
Common Mistakes to Avoid
Mistake | Description |
---|---|
Forgetting to Specify the Column | Developers sometimes forget to define which column they want to count distinct values from, leading to incorrect results or SQL errors. |
Assuming Performance is Always Good | While the COUNT(DISTINCT) function is straightforward, it can lead to performance issues with large datasets as it requires scanning the entire dataset. |
A thorough understanding of data structure and indexing is crucial for achieving accurate results. As a developer, you should familiarize yourself with the underlying schema and explore how indexes can enhance performance.
Optimizing Performance: Indexing and Query Optimization
To improve the performance of counting distinct values in MySQL, indexing is a critical technique. Indexes can significantly speed up query execution and reduce resource consumption. The types of indexes commonly used include B-tree and hash indexes, each with its strengths.
Types of Indexes
-
B-tree Indexes: These are the most common type of index in MySQL and are particularly useful for range queries and ordering results.
-
Hash Indexes: These are more efficient for exact match queries but do not support range queries.
When counting distinct values, a well-designed index can drastically reduce the time it takes to retrieve results. You can create an index on the column of interest using:
CREATE INDEX idx_city ON customers(city);
After creating the index, you can analyze the performance of your query using the EXPLAIN
statement:
EXPLAIN SELECT COUNT(DISTINCT city) FROM customers;
The output will detail how MySQL executes the query and where potential bottlenecks may occur. By analyzing the query plan, you can adjust your indexing strategy to enhance performance.
Leveraging MySQL Functions for Enhanced Counting
MySQL offers built-in functions that aid in counting distinct values more effectively. For instance, using the GROUP BY
and HAVING
clauses allows you to group data and filter results based on specific conditions.
Example of GROUP BY
Suppose you want to count the number of distinct customers per city:
SELECT city, COUNT(DISTINCT customer_id) AS distinct_customers
FROM customers
GROUP BY city;
This query groups the data by city and counts the distinct customer_id
s, providing a summary of unique customers in each city.
Additionally, you can use subqueries or derived tables to handle complex counting scenarios:
SELECT city, COUNT(*)
FROM (SELECT DISTINCT customer_id, city FROM customers) AS distinct_customers
GROUP BY city;
This structure first retrieves distinct customer-city pairs and then counts them in the outer query.
Handling Large Datasets: Partitioning and Sharding
Counting distinct values in large datasets can be challenging. Implementing partitioning and sharding techniques can mitigate performance issues.
What is Partitioning?
Partitioning divides a database into smaller, more manageable pieces. This division can improve query performance by allowing MySQL to scan only relevant partitions instead of the entire dataset.
To partition a table, consider the following example of partitioning by range:
CREATE TABLE sales (
id INT,
amount DECIMAL(10,2),
sale_date DATE
) PARTITION BY RANGE (YEAR(sale_date)) (
PARTITION p0 VALUES LESS THAN (2020),
PARTITION p1 VALUES LESS THAN (2021),
PARTITION p2 VALUES LESS THAN (2022)
);
The Benefits of Sharding
Sharding involves distributing data across multiple servers, allowing for horizontal scaling and enhanced performance. Each shard operates as an independent database, reducing the load on a single server.
When implementing sharding, consider your data distribution and query execution patterns to optimize performance. For example, customer data could be sharded by geographic region, allowing for localized queries.
Case Study: Real-world Application of Distinct Value Counting
To illustrate the application of distinct value counting, consider a retail company needing to analyze customer data to identify unique purchasing trends. The company stored customer transaction data in a MySQL database.
Problem
The business faced challenges in analyzing large datasets to derive insights into customer behavior, such as how many unique customers made purchases each month.
Approach Taken
The company implemented indexing on the customer_id
column and utilized the GROUP BY
clause to summarize the data. The following query was executed:
SELECT MONTH(purchase_date) AS purchase_month, COUNT(DISTINCT customer_id) AS unique_customers
FROM transactions
GROUP BY purchase_month;
Outcomes
By optimizing their queries and leveraging indexing, the company achieved faster query performance, reduced resource consumption, and gained valuable insights into customer behavior, ultimately leading to improved marketing strategies.
Exploring Tools and Extensions: Chat2DB and Beyond
When it comes to managing and analyzing MySQL databases, tools like Chat2DB (opens in a new tab) stand out thanks to their innovative AI capabilities. Chat2DB offers features that facilitate counting distinct values and enhance overall database management efficiency.
Advantages of Using Chat2DB
-
Natural Language Processing: Chat2DB allows users to generate SQL queries using natural language, simplifying database interaction.
-
Smart SQL Editor: The intelligent SQL editor provides real-time suggestions and error-checking, ensuring your queries are optimized for performance.
-
Data Visualization: With Chat2DB, users can easily create visualizations from their data, making it simpler to interpret count results.
By incorporating AI features, Chat2DB enhances the user experience and boosts productivity when managing MySQL databases. If you're currently using tools like DBeaver, MySQL Workbench, or DataGrip, consider switching to Chat2DB to take advantage of its powerful AI functionalities.
Code Examples Recap
Here's a recap of some of the key SQL commands discussed:
Counting Distinct Values
SELECT COUNT(DISTINCT column_name) FROM table_name;
Using GROUP BY
SELECT city, COUNT(DISTINCT customer_id) AS distinct_customers
FROM customers
GROUP BY city;
Partitioning Example
CREATE TABLE sales (
id INT,
amount DECIMAL(10,2),
sale_date DATE
) PARTITION BY RANGE (YEAR(sale_date)) (
PARTITION p0 VALUES LESS THAN (2020),
PARTITION p1 VALUES LESS THAN (2021),
PARTITION p2 VALUES LESS THAN (2022)
);
These examples demonstrate practical applications of counting distinct values and optimizing queries in MySQL.
FAQ
-
What is the difference between COUNT and COUNT(DISTINCT)?
COUNT()
counts all rows, whileCOUNT(DISTINCT)
counts only unique values.
-
How can I optimize my queries for counting distinct values?
- Use indexing on the columns you're counting distinct values from and analyze your query performance with the
EXPLAIN
statement.
- Use indexing on the columns you're counting distinct values from and analyze your query performance with the
-
What is partitioning in MySQL?
- Partitioning divides a table into smaller, manageable pieces to improve performance.
-
Can I count distinct values in a large dataset efficiently?
- Yes, utilizing indexing, partitioning, and sharding can help manage large datasets effectively.
-
Why should I use Chat2DB for MySQL management?
- Chat2DB leverages AI to simplify SQL queries, offers smart editing features, and provides data visualization tools, enhancing productivity and user experience.
By enhancing your understanding of these techniques and tools, you can efficiently count distinct values in MySQL and elevate your database management skills. Explore Chat2DB (opens in a new tab) to leverage its advanced features for your database projects.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!