How to Effectively Utilize MySQL IF Statements in Your Database Queries
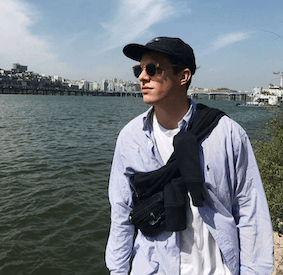
MySQL IF statements are fundamental for incorporating conditional logic into SQL queries. They enable developers to return different results based on whether a specified condition evaluates to TRUE or FALSE. This functionality resembles the IF-ELSE structure found in programming languages. It is essential to comprehend the syntax and usage of IF statements in MySQL to optimize your database interactions effectively.
What is MySQL IF?
The MySQL IF statement allows users to evaluate conditions and return values accordingly. The basic syntax of an IF statement in MySQL is:
IF(condition, true_value, false_value)
- condition: This is a Boolean expression that evaluates to TRUE or FALSE.
- true_value: The value returned if the condition is TRUE.
- false_value: The value returned if the condition is FALSE.
For example:
SELECT IF(age >= 18, 'Adult', 'Minor') AS age_group FROM users;
In this example, the query checks if the age is 18 or older. If TRUE, it returns 'Adult'; otherwise, it returns 'Minor'.
IF vs. IFNULL and COALESCE
While IF statements are useful for conditional logic, they differ from other functions like IFNULL and COALESCE. The IFNULL function specifically checks if a value is NULL and returns an alternative value if it is. Its syntax is:
IFNULL(value, alternative_value)
COALESCE, on the other hand, returns the first non-NULL value from a list of arguments:
COALESCE(value1, value2, ..., alternative_value)
Both functions are useful in scenarios where handling NULL values is critical, but they do not provide the same conditional branching capabilities as IF statements.
Basic Usage in SQL Operations
MySQL IF statements can be used across various SQL operations such as SELECT, INSERT, UPDATE, and DELETE.
Example in SELECT
Using IF in a SELECT statement helps in categorizing data:
SELECT name,
IF(score >= 60, 'Pass', 'Fail') AS result
FROM exams;
Example in INSERT
You can utilize IF statements during data insertion to set default values:
INSERT INTO users (name, status)
VALUES ('John', IF(active = 1, 'Active', 'Inactive'));
Example in UPDATE
IF statements can also conditionally update records:
UPDATE products
SET price = IF(sale = 1, price * 0.9, price);
Example in DELETE
While not common, IF statements can guide deletion logic:
DELETE FROM orders
WHERE IF(status = 'cancelled', order_date < CURDATE() - INTERVAL 30 DAY, FALSE);
Practical Applications of IF Statements
MySQL IF statements find numerous applications in real-world scenarios:
-
Handling NULL Values: By providing alternative values if a field is NULL, IF statements prevent errors in data retrieval.
SELECT name, IF(email IS NULL, 'No Email', email) AS user_email FROM users;
-
Complex Business Logic: Implementing business rules directly in SQL can help streamline application logic.
SELECT product_name, IF(stock > 0, 'In Stock', 'Out of Stock') AS availability FROM inventory;
-
Dynamic Calculations: Adjusting values based on conditions allows for flexible pricing models.
SELECT product_name, price, IF(customer_type = 'premium', price * 0.8, price) AS discounted_price FROM products;
-
Data Validation: Use IF statements to validate data before insertion or updates.
INSERT INTO users (name, age) VALUES ('Alice', IF(age >= 0, age, NULL));
-
Conditional Joins: Create dynamic joins based on certain criteria.
SELECT a.*, b.* FROM table_a a LEFT JOIN table_b b ON a.id = b.a_id WHERE IF(condition, b.status = 'active', TRUE);
-
Summary Reports: Generate reports that adapt to user requirements.
SELECT department, COUNT(IF(status = 'active', 1, NULL)) AS active_count FROM employees GROUP BY department;
Integrating MySQL IF with Other Functions
Combining IF statements with other MySQL functions enhances query capabilities dramatically.
CONCAT Function
Using CONCAT with IF statements allows for dynamic string creation.
SELECT CONCAT(name, ' is ', IF(age >= 18, 'an adult', 'a minor')) AS description
FROM users;
DATE Functions
You can conditionally manipulate dates using IF statements.
SELECT event_name,
IF(event_date < CURDATE(), 'Past', 'Upcoming') AS status
FROM events;
Mathematical Functions
IF statements can perform conditional calculations.
SELECT item_name,
price,
IF(quantity > 100, price * 0.9, price) AS final_price
FROM items;
Using Aggregate Functions
IF statements work well with aggregate functions.
SELECT department,
SUM(IF(status = 'active', salary, 0)) AS total_active_salaries
FROM employees
GROUP BY department;
CASE Expressions
You can embed IF within CASE expressions for more complex evaluations.
SELECT name,
CASE
WHEN score >= 90 THEN 'A'
WHEN score >= 80 THEN 'B'
ELSE 'C'
END AS grade
FROM students;
JSON Functions
IF statements also integrate with JSON functions.
SELECT JSON_EXTRACT(data, '$.status') AS status,
IF(status = 'active', 'Active User', 'Inactive User') AS user_status
FROM user_data;
Advanced Techniques: Nested and Chained IF Statements
As you become more proficient with MySQL IF statements, you may need to use nested and chained statements for complex decision-making processes.
Nested IF Statements
Nesting IF statements allows for handling multiple conditions.
SELECT name,
IF(score >= 90, 'A',
IF(score >= 80, 'B',
IF(score >= 70, 'C', 'F'))) AS grade
FROM students;
Chained IF Statements
Chaining IF statements helps evaluate multiple conditions sequentially.
SELECT name,
IF(condition1, result1,
IF(condition2, result2,
result3)) AS final_result
FROM table;
Real-World Examples
In real-world applications, nested and chained IF statements simplify complex SQL logic. For instance, a retail company might use nested IF statements to determine customer discounts based on membership levels.
Handling Edge Cases
When using nested IF statements, handling edge cases is crucial to maintain query reliability.
SELECT name,
IFNULL(IF(score >= 90, 'A', 'Fail'), 'No Score') AS grade
FROM students;
Performance Considerations and Optimization
While IF statements are powerful, they can impact query performance. Understanding how to optimize their use is vital for maintaining efficient database operations.
Execution Cost
The execution cost of IF statements can influence query efficiency. Minimizing the complexity of your conditions can help improve performance.
Best Practices
- Minimize Overhead: Structure IF statements to reduce performance overhead.
- Utilize Indexes: Using indexes alongside IF statements can enhance query speed.
- Profile Queries: Profiling queries helps identify bottlenecks caused by conditional logic.
- Balance Complexity: Strive for a balance between complexity and maintainability in your IF statements.
- Alternative Logic Approaches: Evaluate using stored procedures or application-level logic for complex scenarios.
Real-World Examples and Use Cases
Examining practical examples of MySQL IF statement applications can provide valuable insights:
- Financial Reporting: A financial system reduced query complexity using IF statements to categorize transactions.
- Retail Personalization: A retail company utilized conditional logic to create personalized offers based on customer behavior.
- Healthcare Databases: Implementing IF statements in healthcare databases facilitated conditional data retrieval for patient records.
- E-commerce Platforms: E-commerce businesses manage regional pricing and taxes effectively with IF conditions.
- Real-Time Analytics: Conditional logic enhances decision-making systems in real-time analytics applications.
Utilizing Chat2DB for Enhanced Query Development
Chat2DB is a powerful tool that can aid in developing, testing, and optimizing MySQL IF statements effectively. Its intuitive interface simplifies the crafting of complex SQL queries.
Visualizing Queries
With Chat2DB, you can visualize and test the impact of IF statements in real-time, making it easier to understand how your conditions affect query results.
Debugging Tools
Chat2DB's debugging tools assist in troubleshooting and refining conditional logic, ensuring your queries run as expected.
Query Optimization Suggestions
Utilizing Chat2DB's optimization suggestions can improve the performance of your IF statements, helping you achieve better query efficiency.
Collaboration Features
The ability to collaborate and share query insights with your team seamlessly using Chat2DB enhances productivity and knowledge sharing.
Integration with Development Environments
Chat2DB integrates with popular development environments, streamlining your database management workflow and making it easier to manage your MySQL queries.
By leveraging tools like Chat2DB, you can enhance your understanding and application of MySQL IF statements, leading to more efficient and effective database interactions.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!