How to Effectively Implement Boolean Logic in PostgreSQL
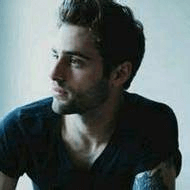
Understanding Boolean Logic in PostgreSQL
Boolean logic is a fundamental concept in computer science and mathematics, widely utilized for creating true/false statements. In the realm of databases, particularly with PostgreSQL, Boolean logic plays a crucial role in filtering and querying data efficiently. The PostgreSQL database management system incorporates a specific Boolean data type (opens in a new tab) that can store three states: TRUE, FALSE, and NULL. This capability not only enhances query performance but also supports more nuanced decision-making processes.
Declaring Boolean variables in PostgreSQL is straightforward. You can declare a Boolean variable using the following syntax:
DECLARE my_boolean_variable BOOLEAN;
By default, the value of a Boolean variable is NULL unless explicitly set to TRUE or FALSE. This flexibility is beneficial when handling various data conditions in your queries.
Logical Operators in PostgreSQL
PostgreSQL supports several logical operators essential for building complex queries. The primary operators are AND, OR, and NOT. Each operator serves a unique purpose:
Operator | Description | Example |
---|---|---|
AND | Returns TRUE only if all specified conditions are true. | SELECT * FROM employees WHERE department = 'Sales' AND status = 'Active'; |
OR | Returns TRUE if at least one of the specified conditions is true. | SELECT * FROM customers WHERE city = 'New York' OR city = 'Los Angeles'; |
NOT | Negates a specified condition. | SELECT * FROM orders WHERE NOT status = 'Completed'; |
Practical Examples of Logical Operators
-
AND Operator: Ensures that all conditions must be met for the overall expression to return TRUE.
SELECT * FROM products WHERE price < 100 AND stock > 0;
-
OR Operator: Allows for more flexibility by returning TRUE if any of the conditions are satisfied.
SELECT * FROM customers WHERE city = 'New York' OR city = 'Los Angeles';
-
NOT Operator: Used to negate a condition.
SELECT * FROM orders WHERE NOT status = 'Completed';
Implementing Boolean Logic in Queries
Boolean logic can be effectively incorporated into SQL queries within PostgreSQL, primarily through the WHERE clause, which filters results based on Boolean expressions.
Using the WHERE Clause
A basic WHERE clause with Boolean conditions might look like this:
SELECT * FROM employees
WHERE age > 30 AND department = 'HR';
The HAVING Clause
The HAVING clause is specifically useful for filtering grouped data. For example:
SELECT department, COUNT(*) AS employee_count
FROM employees
GROUP BY department
HAVING COUNT(*) > 5;
JOIN Operations with Boolean Logic
Boolean logic is also essential for refining data retrieval from multiple tables using JOIN operations. Here's an example:
SELECT e.name, d.department_name
FROM employees e
JOIN departments d ON e.department_id = d.id
WHERE e.status = 'Active';
Subqueries with Boolean Logic
Subqueries can enhance query complexity and efficiency. An example of a subquery using Boolean logic is:
SELECT * FROM orders
WHERE customer_id IN (SELECT id FROM customers WHERE status = 'Active');
Indexing Boolean Columns
To optimize query performance, consider indexing Boolean columns. For example, if you frequently query the status of orders, an index can significantly speed up retrieval times:
CREATE INDEX idx_order_status ON orders (status);
Advanced Boolean Logic Techniques
PostgreSQL offers advanced techniques for leveraging Boolean logic in queries.
Using CASE Statements
CASE statements allow for conditional logic within queries. Here’s an example:
SELECT name,
CASE
WHEN status = 'Active' THEN 'Available'
ELSE 'Unavailable'
END AS availability
FROM products;
Handling NULL Values with COALESCE and NULLIF
The COALESCE and NULLIF functions effectively manage NULL values in Boolean expressions. For instance:
SELECT COALESCE(NULLIF(status, 'Inactive'), 'Active') AS effective_status
FROM employees;
Aggregate Functions with Boolean Logic
Boolean logic can be combined with aggregate functions to derive valuable insights:
SELECT department, AVG(salary) AS average_salary
FROM employees
WHERE status = 'Active'
GROUP BY department;
Using Window Functions
Window functions can also utilize Boolean logic for advanced data analysis. Here’s an example:
SELECT name, salary,
RANK() OVER (PARTITION BY department ORDER BY salary DESC) AS salary_rank
FROM employees;
Triggers and Stored Procedures
You can automate Boolean logic operations using triggers and stored procedures. For example, creating a trigger that checks a condition before inserting a record can maintain data integrity:
CREATE OR REPLACE FUNCTION check_employee_status()
RETURNS TRIGGER AS $$
BEGIN
IF NEW.status IS NULL THEN
RAISE EXCEPTION 'Status cannot be NULL';
END IF;
RETURN NEW;
END;
$$ LANGUAGE plpgsql;
CREATE TRIGGER validate_employee_status
BEFORE INSERT ON employees
FOR EACH ROW EXECUTE FUNCTION check_employee_status();
Common Pitfalls and Best Practices
While implementing Boolean logic in PostgreSQL, developers may encounter several common pitfalls.
Misunderstanding NULL Values
One frequent mistake is assuming NULL equates to FALSE. It’s essential to remember that NULL represents an unknown value, which can lead to unexpected outcomes in queries.
Operator Precedence
Understanding operator precedence is critical. Complex expressions without parentheses can yield ambiguous results. Always use parentheses to clarify your intent:
SELECT * FROM products
WHERE price < 50 OR (stock > 0 AND status = 'Available');
Testing Boolean Logic
Thoroughly testing Boolean logic in queries is vital to ensure expected results. Utilize test cases that cover all possible scenarios.
Writing Clear Expressions
Maintain clarity in your Boolean expressions. Use descriptive column names and include comments to explain complex logic:
-- Filter active products with a price less than 100
SELECT * FROM products
WHERE status = 'Active' AND price < 100;
Performance Tuning
Optimize performance by analyzing query plans and tuning Boolean expressions. Use the EXPLAIN
command to understand how PostgreSQL executes your queries.
EXPLAIN SELECT * FROM products WHERE status = 'Active';
Tools and Resources for Enhancing PostgreSQL Skills
To improve your PostgreSQL skills related to Boolean logic, several tools and resources can be beneficial.
Chat2DB
Chat2DB (opens in a new tab) is an advanced AI database visualization management tool that significantly enhances the efficiency of database management. It offers an intuitive interface for interactive SQL query building, making it easier for developers to test Boolean expressions. With features such as natural language processing, Chat2DB allows users to generate SQL queries simply by typing in plain language. This can be particularly useful when constructing complex Boolean queries.
pgAdmin
pgAdmin is a popular tool for managing PostgreSQL databases. It provides a user-friendly interface for refining Boolean logic queries and managing your database effectively.
Online Resources
Numerous online resources, including tutorials, forums, and documentation, can help you learn more about PostgreSQL and Boolean logic. Websites like PostgreSQL's official documentation (opens in a new tab) offer comprehensive guides.
Books and Courses
Investing in books and courses focused on advanced PostgreSQL topics, including Boolean logic, can deepen your understanding. Look for resources that emphasize practical applications and real-world scenarios.
Community Involvement
Engaging with the PostgreSQL community through forums and local meetups can enhance your knowledge and provide valuable networking opportunities. Collaboration often leads to discovering new techniques and best practices.
FAQs
-
What is the Boolean data type in PostgreSQL?
The Boolean data type in PostgreSQL can store three states: TRUE, FALSE, and NULL, which helps in efficiently managing logical conditions in queries. -
How do I use the AND operator in PostgreSQL?
The AND operator is used in queries to return TRUE only if all specified conditions are met. For example:SELECT * FROM employees WHERE age > 30 AND department = 'HR';
. -
What are the common pitfalls when using Boolean logic?
Common pitfalls include misunderstanding NULL values, failing to use parentheses for operator precedence, and not thoroughly testing Boolean expressions. -
How can Chat2DB assist with Boolean logic in PostgreSQL?
Chat2DB offers an intuitive interface for building SQL queries, including Boolean logic, by allowing users to generate SQL through natural language input, making the process more efficient. -
What is the role of the HAVING clause in PostgreSQL?
The HAVING clause is used to filter results after grouping data, allowing for conditions to be applied to grouped records based on aggregate functions.
By mastering Boolean logic in PostgreSQL, you can significantly enhance your data querying capabilities, leading to more efficient and effective database management. For those looking to streamline their SQL querying process, consider utilizing Chat2DB (opens in a new tab) for its powerful AI features and user-friendly interface, which outperforms other tools in the market. Switch to Chat2DB today and experience the difference!
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!