How to Integrate Python LDAP for Seamless Directory Access
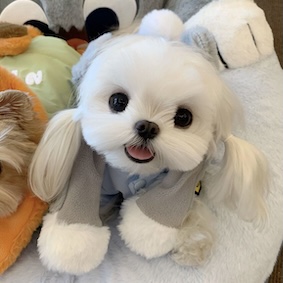
Integrating Python LDAP is vital for seamless directory access, enabling developers to efficiently interact with directory servers like Active Directory and OpenLDAP. This article explores the fundamental concepts, setup procedures, user management, optimization strategies, and security best practices associated with using Python LDAP. We will also examine how tools like Chat2DB (opens in a new tab) can enhance the user experience through its AI-powered features.
Understanding Python LDAP for Efficient Directory Access
Python LDAP provides a powerful framework for managing directory services through the Lightweight Directory Access Protocol (LDAP). LDAP allows applications to access and manage directory information services over a network, which is crucial in enterprise environments for managing user authentication, authorization, and information retrieval.
Key Concepts of LDAP
Concept | Description |
---|---|
Entries | Each entry in an LDAP directory is a collection of attributes that hold data, such as user information and group memberships. |
Attributes | Attributes are the data fields associated with entries. Common attributes include cn (common name), mail (email), and uid (user ID). |
Distinguished Names (DNs) | The DN uniquely identifies an entry in the LDAP directory, including the entry's name and its position in the directory hierarchy. |
Benefits of Using Python for LDAP Integration
- Simplicity: Python's clear syntax makes it an ideal choice for integrating LDAP.
- Flexibility: Libraries like
python-ldap
offer extensive features for directory manipulation. - Security: Enhanced security measures can protect sensitive user data.
The python-ldap library (opens in a new tab) is a widely-used tool that facilitates connections to LDAP servers, enabling user authentication, information retrieval, and user directory management with ease.
Setting Up Your Python LDAP Environment
Establishing an appropriate environment is essential for successful integration with Python LDAP. Here’s how to get started.
Prerequisites
- Python Installation: Ensure Python is installed on your system. Download it from the official Python website (opens in a new tab).
- Pip Installation: Pip is a package manager for Python that simplifies library installations.
Installing the Python LDAP Library
Once Python and pip are set up, install the python-ldap
library using the following command:
pip install python-ldap
Setting Up a Virtual Environment
Creating a virtual environment to manage dependencies is recommended:
# Create a virtual environment
python -m venv ldap_env
# Activate the virtual environment
# On Windows
ldap_env\Scripts\activate
# On macOS/Linux
source ldap_env/bin/activate
Configuring the LDAP Connection
Establish a connection to your LDAP server by specifying the server address, port number, and authentication credentials.
import ldap
# Set up the connection
ldap_server = "ldap://your_ldap_server.com"
username = "uid=your_username,ou=users,dc=example,dc=com"
password = "your_password"
# Connect to the LDAP server
try:
conn = ldap.initialize(ldap_server)
conn.protocol_version = ldap.VERSION3
conn.simple_bind_s(username, password)
print("LDAP connection established.")
except ldap.LDAPError as e:
print(f"LDAP connection failed: {e}")
Handling SSL/TLS for Secure Connections
To secure connections to your LDAP server, use SSL/TLS as follows:
import ldap
# Use ldaps for secure connection
ldap_server = "ldaps://your_ldap_server.com"
# Connect securely
try:
conn = ldap.initialize(ldap_server)
conn.start_tls_s() # Initiate TLS
conn.simple_bind_s(username, password)
print("Secure LDAP connection established.")
except ldap.LDAPError as e:
print(f"Secure LDAP connection failed: {e}")
Common Setup Challenges
- Version Compatibility: Ensure that the
python-ldap
version is compatible with your LDAP server. - Firewall Issues: Check that your firewall allows connections to the LDAP port (usually 389 or 636 for SSL).
For efficient management and testing of LDAP queries, tools like Chat2DB (opens in a new tab) can be invaluable. Its AI capabilities streamline database interactions, making it easier to test LDAP queries without extensive coding.
Implementing Python LDAP for Authentication and User Management
Implementing Python LDAP for user authentication and management is straightforward with the right approach.
User Authentication
Authenticate a user against the LDAP directory with the following:
def authenticate_user(username, password):
try:
user_dn = f"uid={username},ou=users,dc=example,dc=com"
conn.simple_bind_s(user_dn, password)
print("User authenticated successfully.")
return True
except ldap.INVALID_CREDENTIALS:
print("Invalid credentials.")
return False
Retrieving User Information
You can retrieve user information using search operations:
def get_user_info(username):
search_base = "ou=users,dc=example,dc=com"
search_filter = f"(uid={username})"
try:
result = conn.search_s(search_base, ldap.SCOPE_SUBTREE, search_filter)
if result:
user_info = result[0][1]
return user_info
else:
print("User not found.")
return None
except ldap.LDAPError as e:
print(f"Error retrieving user info: {e}")
Managing User Entries
You can add, modify, or delete user entries programmatically.
Adding a User Entry
def add_user(username, email):
dn = f"uid={username},ou=users,dc=example,dc=com"
attrs = {
'objectClass': [b'inetOrgPerson', b'organizationalPerson', b'person', b'top'],
'cn': [username.encode()],
'sn': [username.encode()],
'mail': [email.encode()],
'uid': [username.encode()],
'userPassword': [b'secure_password']
}
try:
conn.add_s(dn.encode(), [(key, value) for key, value in attrs.items()])
print("User added successfully.")
except ldap.LDAPError as e:
print(f"Error adding user: {e}")
Modifying a User Entry
def modify_user(username, new_email):
dn = f"uid={username},ou=users,dc=example,dc=com"
mod_attrs = [(ldap.MOD_REPLACE, 'mail', new_email.encode())]
try:
conn.modify_s(dn.encode(), mod_attrs)
print("User email updated successfully.")
except ldap.LDAPError as e:
print(f"Error modifying user: {e}")
Deleting a User Entry
def delete_user(username):
dn = f"uid={username},ou=users,dc=example,dc=com"
try:
conn.delete_s(dn.encode())
print("User deleted successfully.")
except ldap.LDAPError as e:
print(f"Error deleting user: {e}")
Common Use Cases
- Password Management: Use LDAP to manage and reset user passwords.
- User Role Assignments: Assign roles to users through group memberships in the directory.
Implementing error handling for LDAP operations is crucial for a seamless experience. Always anticipate potential exceptions and handle them accordingly.
Optimizing Directory Access with Python LDAP
Optimizing LDAP queries is vital for improving performance, especially in large directory structures. Here are some strategies:
Efficient Directory Browsing
- Pagination: Utilize pagination to break down search results into manageable chunks. This reduces the load on the LDAP server.
def paginated_search(base_dn, filter, page_size=100):
cookie = None
while True:
results = conn.search_ext_s(base_dn, ldap.SCOPE_SUBTREE, filter, serverctrls=[
ldap.control.SimplePagedResultsControl(page_size, cookie, True)
])
for dn, entry in results:
yield dn, entry
cookie = results.controls[0].cookie
if not cookie:
break
- Limiting Search Scopes: Narrow down searches to specific organizational units to minimize the search space.
Caching Mechanisms
Implement caching to reduce the load on LDAP servers and improve response times:
import functools
@functools.lru_cache(maxsize=128)
def cached_get_user_info(username):
return get_user_info(username)
Indexing Attributes
Indexing frequently searched attributes in the LDAP directory can significantly enhance retrieval times. Consult your directory server's documentation for guidance on indexing practices.
Virtual Directory Servers
Using virtual directory servers can improve access efficiency by abstracting backend directories, allowing consolidated access to multiple directories.
Monitoring LDAP Query Performance
Monitoring and analyzing LDAP query performance is essential to identify bottlenecks. Tools like Chat2DB (opens in a new tab) provide real-time insights into query performance, enhancing overall efficiency.
Security Best Practices for Python LDAP Integration
Security is paramount when integrating Python LDAP. Implement the following best practices:
Secure Connections
Always use SSL/TLS to protect data in transit, ensuring that sensitive information is encrypted and secure.
Authentication Methods
Utilize strong authentication methods, such as SASL (Simple Authentication and Security Layer), to enhance security.
Managing LDAP Credentials
Employ best practices for managing LDAP credentials, including:
- Regularly updating passwords.
- Using environment variables to store sensitive information.
Logging and Monitoring
Implement logging and monitoring of LDAP activities to help detect and respond to security incidents promptly.
Regular Updates
Keep the python-ldap
library and underlying systems updated to mitigate vulnerabilities.
Role of Encryption
Utilizing encryption and digital certificates is crucial for securing LDAP communications. Ensure that your LDAP server is configured to use strong encryption protocols.
Tools like Chat2DB (opens in a new tab) can assist in maintaining security standards through their advanced monitoring and alerting features, ensuring your directory access is both efficient and secure.
Conclusion
Integrating Python LDAP for seamless directory access is a powerful approach to managing user information in enterprise environments. By understanding the fundamental concepts, correctly setting up your environment, implementing user management, optimizing directory access, and adhering to security best practices, you can leverage Python LDAP effectively.
Additionally, consider utilizing tools like Chat2DB (opens in a new tab) for enhanced management capabilities, especially with its AI-driven features that streamline database interactions and provide superior performance compared to competitors like DBeaver, MySQL Workbench, and DataGrip.
FAQ
-
What is Python LDAP? Python LDAP is a library that allows developers to interact with LDAP servers for user authentication and directory management.
-
How do I install the python-ldap library? You can install it using pip with the command
pip install python-ldap
. -
How can I securely connect to an LDAP server? Use SSL/TLS by connecting to
ldaps://your_ldap_server.com
and initiating TLS withconn.start_tls_s()
. -
What are the common use cases for Python LDAP? Common use cases include user authentication, retrieving user information, and managing user accounts programmatically.
-
How does Chat2DB enhance LDAP management? Chat2DB offers AI-powered features for efficient database management, including real-time monitoring, intuitive interfaces for querying data, and enhanced security protocols, making it a superior choice for LDAP integration.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!