How to Implement and Optimize PostgreSQL Check Constraints for Enhanced Data Integrity
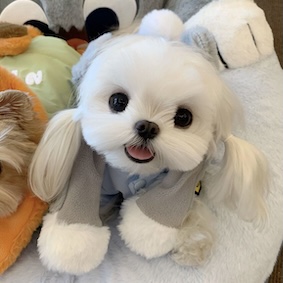
Implementing and optimizing PostgreSQL check constraints is essential for maintaining data integrity within your database. This article will guide you through understanding the importance of check constraints, the methods to implement them effectively, and strategies for optimizing their performance. Throughout the discussion, we will emphasize the advantages of using PostgreSQL check constraints compared to application-level validations, as well as how tools like Chat2DB (opens in a new tab) can simplify your database management tasks.
Understanding PostgreSQL Check Constraints
What are Check Constraints?
In PostgreSQL, check constraints are rules that enforce specific conditions on the values within a table's columns. They ensure that only valid data is entered into the database, enhancing data integrity and accuracy. By defining these constraints at the database level, developers can prevent invalid data from being processed, thereby minimizing potential errors and inconsistencies.
Importance of Check Constraints
Check constraints are crucial for maintaining domain-specific rules within a database. They provide a means to enforce data validation without relying solely on application logic. For example, a check constraint can validate that an employee's salary falls within a specific range or that a date of birth is in the past.
Syntax for Creating Check Constraints
Creating a check constraint in PostgreSQL is straightforward. The basic syntax is as follows:
ALTER TABLE table_name
ADD CONSTRAINT constraint_name
CHECK (condition);
Example of a Simple Check Constraint
Let's say we have a table called employees
. We want to ensure that no employee can have a salary lower than 30000. Here’s how you can create a check constraint:
CREATE TABLE employees (
id SERIAL PRIMARY KEY,
name VARCHAR(100),
salary NUMERIC CHECK (salary >= 30000)
);
Advantages Over Application-Level Validation
Using check constraints provides several advantages:
Advantage | Description |
---|---|
Automatic Enforcement | Constraints are enforced automatically by the database, reducing the chances of human error. |
Centralized Rule Management | With constraints defined in the database schema, there’s a single source of truth for your validation rules. |
Better Performance | Constraints can be optimized by the database engine, allowing for faster data validation compared to application-level checks. |
Common Use Cases
Check constraints can be applied in various scenarios such as:
- Validating numerical ranges.
- Ensuring strings match specific patterns (e.g., email formats).
- Enforcing relationships between columns (e.g., start date must be before end date).
Combining Multiple Check Constraints
PostgreSQL allows multiple check constraints on a single table. You can combine these constraints to enforce complex rules:
CREATE TABLE products (
id SERIAL PRIMARY KEY,
name VARCHAR(100),
price NUMERIC CHECK (price > 0),
stock INTEGER CHECK (stock >= 0),
expiration_date DATE CHECK (expiration_date > CURRENT_DATE)
);
This example shows how to enforce multiple rules on a product's attributes to maintain data integrity.
Implementing Check Constraints in Your Database
Step-by-Step Process
When adding check constraints to an existing PostgreSQL database, follow these steps:
- Assess Current Data: Before implementing new constraints, assess existing data to ensure compliance with the new rules.
- Use the ALTER TABLE Command: You can add a check constraint to an existing column using the
ALTER TABLE
command. For instance:
ALTER TABLE employees
ADD CONSTRAINT check_salary
CHECK (salary >= 30000);
- Use WITH NO CHECK Option: If you're concerned about existing data violating the new constraint, you can add it with the
WITH NO CHECK
option initially. This allows you to enforce the constraint on new data while assessing existing records.
ALTER TABLE employees
ADD CONSTRAINT check_salary
CHECK (salary >= 30000) WITH NO CHECK;
- Data Cleanup: If you find existing data that violates the new constraint, plan for data cleanup to ensure compliance.
- Testing: Always test new constraints in a development environment before deploying them to production.
Documenting Constraints
It's essential to document your check constraints within the database schema. This documentation serves as a reference for future developers and helps in maintaining the database structure.
Optimizing Check Constraints for Performance
Performance Implications
While check constraints are invaluable, they can have performance implications. The complexity of the constraint expression can affect query performance, especially during data modification operations.
Strategies for Optimization
-
Simplify Expressions: Whenever possible, simplify the constraint expressions to improve performance.
Example of a simple constraint:
CHECK (price > 0)
-
Use Indexed Columns: If your constraint can leverage indexed columns, it can enhance performance significantly.
-
Test Performance Impacts: Always test the performance impacts of constraints in a staging environment to anticipate their behavior in production.
-
Monitor Performance: Utilize PostgreSQL's built-in tools and logging capabilities to monitor the impact of constraints on database performance.
When Not to Use Check Constraints
In some scenarios, check constraints may not be the best solution. For complex business rules, consider using triggers or application logic instead.
Advanced Use Cases and Examples
Validating String Formats with Regular Expressions
Check constraints can also utilize regular expressions for validating string formats. For example, to ensure an email address is valid, you can apply a constraint like this:
CREATE TABLE users (
id SERIAL PRIMARY KEY,
email VARCHAR(255) CHECK (email ~ '^[^@]+@[^@]+\.[^@]+$')
);
Cross-Column Validation
You can create constraints that reference other columns within the same table. For example:
CREATE TABLE events (
id SERIAL PRIMARY KEY,
start_date DATE,
end_date DATE CHECK (end_date > start_date)
);
Dynamic Range Validation
For more advanced checks, you can incorporate logic that determines the range dynamically:
CREATE TABLE sales (
id SERIAL PRIMARY KEY,
discount NUMERIC,
price NUMERIC CHECK (discount <= price * 0.5)
);
External Factors in Check Constraints
Constraints can also be based on external factors, such as the current date and time:
CREATE TABLE promotions (
id SERIAL PRIMARY KEY,
promotion_end DATE CHECK (promotion_end > CURRENT_DATE)
);
Integrating with Other Database Features
You can combine check constraints with foreign keys for comprehensive validation rules. For instance, ensuring that a related record exists while enforcing specific conditions can enhance data integrity.
CREATE TABLE orders (
id SERIAL PRIMARY KEY,
customer_id INTEGER REFERENCES customers(id),
order_date DATE CHECK (order_date <= CURRENT_DATE)
);
Utilizing Chat2DB for Managing Check Constraints
Introduction to Chat2DB
Chat2DB (opens in a new tab) is an AI-driven database management tool that simplifies the process of managing PostgreSQL databases, including the implementation and optimization of check constraints. Its user-friendly interface allows developers and database administrators to define and modify check constraints effortlessly.
Features of Chat2DB
-
Visual Schema Exploration: Chat2DB provides a visual representation of your database schema, making it easy to identify where constraints are applied.
-
Constraint Validation Tools: The tool offers features that validate constraints and check for compliance with your defined rules.
-
Query Builder: Use Chat2DB’s query builder to test check constraints in a sandbox environment before deploying them into production. This feature allows you to experiment with queries safely.
-
Automated Documentation: Chat2DB can generate automated documentation for your database schema, including detailed constraint definitions, helping maintain clarity and organization.
-
Performance Monitoring: The tool’s capabilities in monitoring constraint performance and troubleshooting issues as they arise provide an added layer of reliability.
Case Study
Consider a development team that successfully managed check constraints using Chat2DB. They leveraged the tool's AI features to quickly implement constraints, ensuring data integrity while minimizing errors. The visual schema exploration made identifying and optimizing constraints seamless, ultimately leading to improved database performance.
Conclusion
Implementing and optimizing PostgreSQL check constraints is essential for maintaining data integrity. By understanding their importance and leveraging tools like Chat2DB (opens in a new tab), you can streamline your database management processes and enhance the overall efficiency of your data operations. Transitioning to Chat2DB not only simplifies your database tasks but also provides AI-driven insights that traditional tools may lack.
FAQ
-
What are PostgreSQL check constraints?
- Check constraints are rules that enforce specific conditions on the values within a table's columns.
-
How do I add a check constraint to an existing table?
- You can use the
ALTER TABLE
command to add a check constraint to an existing column.
- You can use the
-
Can I use complex expressions in check constraints?
- Yes, PostgreSQL supports complex expressions, including regular expressions and cross-column validations.
-
How does Chat2DB assist with check constraints?
- Chat2DB simplifies the management of check constraints with visual tools, automated documentation, and performance monitoring features.
-
Are there alternatives to check constraints?
- For complex business logic, you may consider using triggers or application-level validations as alternatives to check constraints. Transitioning to Chat2DB can enhance your database management experience significantly.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!