How to Use Inner Join in PostgreSQL: A Comprehensive Guide to SQL Joins
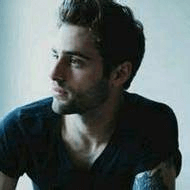
Understanding Inner Join in PostgreSQL
Inner Join is a pivotal concept in relational databases, particularly in PostgreSQL, as it enables the combination of rows from two or more tables based on a related column. Mastering how to use Inner Join effectively is essential for querying multiple tables and retrieving comprehensive datasets.
In PostgreSQL, an Inner Join returns rows when there is a match between the tables involved in the join. This means that the result set will only include rows that meet the join condition. Inner Join is often contrasted with other types of joins, such as Left Join, Right Join, and Full Join, which handle unmatched rows differently.
For those new to database normalization and data integrity, grasping the role of Inner Join is crucial. It enforces relationships between tables, ensuring that data remains organized and consistent. To explore what Inner Join is and how it differs from other types, check out the Wikipedia page on SQL Joins (opens in a new tab).
Syntax and Structure of Inner Join
The syntax for performing an Inner Join in PostgreSQL is straightforward. The basic structure is as follows:
SELECT columns
FROM table1
INNER JOIN table2
ON table1.column_name = table2.column_name;
In this structure, columns
refers to the specific columns we want to retrieve from the joined tables. The ON
clause is crucial; it establishes the relationship between the two tables based on their common column.
To optimize performance, select specific columns rather than using SELECT *
, which retrieves all columns. For example:
SELECT employee.name, department.name
FROM employee
INNER JOIN department ON employee.department_id = department.id;
This query retrieves the names of employees along with their corresponding department names. Using aliases can enhance readability:
SELECT e.name AS employee_name, d.name AS department_name
FROM employee AS e
INNER JOIN department AS d ON e.department_id = d.id;
Here, e
and d
are aliases for employee
and department
, respectively, simplifying the query and improving readability.
Practical Examples of Inner Join in PostgreSQL
Real-world applications of Inner Join can significantly enhance your database queries. One common scenario is joining two tables with a common key. Let’s say you have an employees
table and a departments
table. You can retrieve data about employees and their department names using an Inner Join.
Example 1: Joining Two Tables
Assuming the following two tables:
employees
id | name | department_id |
---|---|---|
1 | Alice | 1 |
2 | Bob | 2 |
3 | Charlie | 1 |
departments
id | name |
---|---|
1 | Engineering |
2 | Marketing |
You can execute the following query:
SELECT e.name AS employee_name, d.name AS department_name
FROM employees AS e
INNER JOIN departments AS d ON e.department_id = d.id;
This returns:
employee_name | department_name |
---|---|
Alice | Engineering |
Bob | Marketing |
Charlie | Engineering |
Example 2: Joining Multiple Tables
You can also join more than two tables by chaining multiple Inner Joins. For instance, if you have a projects
table:
projects
id | project_name | employee_id |
---|---|---|
1 | Project A | 1 |
2 | Project B | 2 |
3 | Project C | 3 |
You can join the employees
, departments
, and projects
tables:
SELECT e.name AS employee_name, d.name AS department_name, p.project_name
FROM employees AS e
INNER JOIN departments AS d ON e.department_id = d.id
INNER JOIN projects AS p ON e.id = p.employee_id;
This allows you to retrieve a comprehensive dataset that includes employee names, their department names, and the projects they are working on.
Performance Optimization with Inner Join
Optimizing performance for Inner Join operations in PostgreSQL is crucial, especially when dealing with large datasets. Here are several techniques to enhance query performance:
-
Indexing: Ensure that the columns frequently used in join conditions are indexed. Indexing can significantly speed up query execution.
-
Using Query Planners: PostgreSQL employs query planners to determine the most efficient way to execute a query. Understanding how to examine execution plans can lead to performance improvements. You can use the following command to analyze your query:
EXPLAIN ANALYZE SELECT e.name, d.name FROM employees AS e INNER JOIN departments AS d ON e.department_id = d.id;
-
Avoiding Cartesian Products: Ensure the join conditions are set correctly to avoid unintended Cartesian products, which can drastically increase the result set size and reduce performance.
-
Database Tuning: Regularly analyze and tune your database to ensure optimal performance for join operations.
Advanced Inner Join Techniques
For more complex scenarios, advanced techniques for using Inner Join can be highly beneficial. Here are a few advanced applications:
Subqueries
You can use Inner Join in conjunction with subqueries to filter data more effectively. For example:
SELECT e.name, d.name
FROM employees AS e
INNER JOIN departments AS d ON e.department_id = d.id
WHERE e.id IN (SELECT employee_id FROM projects WHERE project_name = 'Project A');
Self-Joins
A self-join is a special case where a table is joined with itself. This is useful for hierarchical data. For example:
SELECT e1.name AS employee_name, e2.name AS manager_name
FROM employees AS e1
INNER JOIN employees AS e2 ON e1.manager_id = e2.id;
Using Window Functions
Combining Inner Join with window functions allows for complex analytical queries. For instance, you can use window functions to rank employees within their departments:
SELECT e.name, d.name, RANK() OVER (PARTITION BY d.id ORDER BY e.salary DESC) AS salary_rank
FROM employees AS e
INNER JOIN departments AS d ON e.department_id = d.id;
Troubleshooting Common Issues with Inner Join
While working with Inner Join, developers may encounter several common issues. Here’s how to troubleshoot them:
-
Ambiguous Column References: When two tables have columns with the same name, use table aliases to avoid ambiguity.
SELECT e.name, d.name FROM employees AS e INNER JOIN departments AS d ON e.department_id = d.id;
-
Incorrect Join Results: If you’re missing or seeing duplicate rows, double-check your join conditions and ensure they are correct.
-
Handling NULL Values: Understand how NULL values affect join conditions. You can use
COALESCE
to handle NULL values effectively. -
Debugging Queries: Use logs and query execution plans to debug and test join queries, ensuring they return accurate results.
Leveraging Chat2DB for Inner Join Operations
To enhance your experience with PostgreSQL, consider using Chat2DB (opens in a new tab), an AI-powered database visualization management tool. Chat2DB simplifies the process of writing and testing Inner Join queries with its intuitive interface and advanced features.
Benefits of Using Chat2DB
-
Natural Language SQL Generation: Chat2DB allows you to generate SQL queries using natural language. Simply describe the data you need, and Chat2DB will produce the corresponding SQL, including Inner Joins. This feature is particularly useful for beginners or those looking to streamline their query writing process.
-
Visual Query Execution Plans: This feature helps you understand how your queries are executed, making it easier to optimize performance. Instead of deciphering complex execution plans, Chat2DB presents them visually, allowing for quicker insights.
-
Collaboration: Chat2DB supports collaboration among team members, allowing you to share queries and results seamlessly. This is especially valuable in team environments where database management tasks are shared.
-
Performance Insights: It provides insights into query performance, enabling you to fine-tune your joins and other SQL operations effectively. This can lead to significant performance improvements in your database management tasks.
By utilizing Chat2DB, you can streamline your database management tasks, improve your SQL skills, and optimize your Inner Join operations. Unlike other tools like DBeaver, MySQL Workbench, or DataGrip, Chat2DB leverages AI to enhance your productivity, making it an excellent choice for database professionals.
FAQ
-
What is an Inner Join in PostgreSQL?
- An Inner Join combines rows from two or more tables based on a related column, returning only the rows that match in both tables.
-
How do I write an Inner Join query?
- The basic syntax is
SELECT columns FROM table1 INNER JOIN table2 ON table1.column_name = table2.column_name;
.
- The basic syntax is
-
Can I join more than two tables?
- Yes, you can chain multiple Inner Joins to join more than two tables.
-
What are some common issues with Inner Joins?
- Common issues include ambiguous column references, incorrect join results, and handling NULL values.
-
How can Chat2DB assist in using Inner Joins?
- Chat2DB simplifies query writing, provides visual execution plans, and offers performance insights, making it easier to work with Inner Joins in PostgreSQL.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!