How to Effectively Use MySQL LEFT JOIN in Your Queries
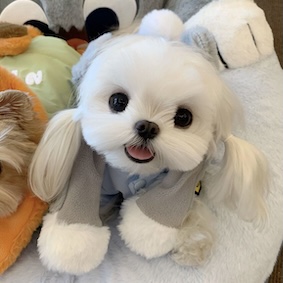
To effectively implement MySQL LEFT JOIN in your queries, it's essential to understand its purpose and functionality. The LEFT JOIN combines rows from two or more tables based on a related column, returning all rows from the left table and matched rows from the right table. If no match is found, NULL values are returned for columns from the right table. This article will delve into the syntax and advantages of MySQL LEFT JOIN, explore common use cases, and provide detailed guidance on how to implement it in your queries, including performance optimization techniques and troubleshooting tips.
Understanding MySQL LEFT JOIN
In MySQL, the LEFT JOIN is a powerful tool that allows developers to merge data from multiple tables. Unlike the INNER JOIN, which only returns rows with matching values in both tables, the LEFT JOIN ensures that all records from the left table are included in the result set, even if there are no corresponding records in the right table. This can be particularly useful in scenarios where you want to display all data from one table, regardless of whether there is related data in another table.
Syntax of LEFT JOIN
The basic syntax for a LEFT JOIN is as follows:
SELECT columns
FROM table1
LEFT JOIN table2
ON table1.common_field = table2.common_field;
Here, table1
is the left table and table2
is the right table. The common_field
is the column that the two tables share, allowing the join to occur.
Example of LEFT JOIN
Let's consider a practical example. Suppose you have two tables: customers
and orders
. The customers
table contains information about each customer, while the orders
table contains details about their orders. You want to retrieve a list of all customers and their orders, including those customers who have not placed any orders.
SELECT customers.customer_id, customers.customer_name, orders.order_id
FROM customers
LEFT JOIN orders
ON customers.customer_id = orders.customer_id;
In this example, all customer records will be returned, and where there are no matching orders, the order_id
will show as NULL.
Advantages of Using LEFT JOIN
Using LEFT JOIN has several advantages, particularly in specific scenarios:
Advantage | Explanation |
---|---|
Complete Dataset | Retrieves all rows from the left table. |
Simplified Queries | Reduces complexity by avoiding subqueries. |
Preserves Data Integrity | Ensures no data is lost during the join process. |
Performance Considerations | Improves efficiency in large datasets. |
Common Use Cases for LEFT JOIN
Understanding when to use LEFT JOIN is crucial for effective database management. Here are some common use cases:
1. Reporting
When generating reports that need to include all entries from one table, such as employee records with or without associated performance metrics, LEFT JOIN is invaluable.
Example:
SELECT employees.employee_id, employees.employee_name, performance_metrics.metric_score
FROM employees
LEFT JOIN performance_metrics
ON employees.employee_id = performance_metrics.employee_id;
In this query, all employee records will be displayed even if there are no performance metrics recorded for them.
2. Combining Data
When combining data from multiple tables where some may have missing information, LEFT JOIN ensures no data is excluded. This is particularly useful in data analysis scenarios.
Example:
SELECT products.product_name, categories.category_name
FROM products
LEFT JOIN categories
ON products.category_id = categories.category_id;
In this case, all products will be listed, even those that do not belong to any category.
Implementing LEFT JOIN in Your Queries
Implementing LEFT JOIN effectively requires a clear understanding of the tables involved and the relationships between them. Here’s a step-by-step guide:
Step 1: Identify the Tables
Determine which tables you need to join. For example, if you need customer and order data, you will work with the customers
and orders
tables.
Step 2: Identify the Joining Column
Identify the common field that links the two tables. This is typically a primary key in one table and a foreign key in the other.
Step 3: Write the SQL Code
With the tables and joining columns identified, you can write the SQL code using the LEFT JOIN syntax.
Here’s a complete example that retrieves all customers and their orders:
SELECT
customers.customer_id,
customers.customer_name,
orders.order_id
FROM
customers
LEFT JOIN
orders
ON
customers.customer_id = orders.customer_id;
Handling NULL Values
When using LEFT JOIN, it is common to encounter NULL values for columns from the right table. You can handle these values using the COALESCE
function, which allows you to return a default value instead of NULL.
Example:
SELECT
customers.customer_id,
customers.customer_name,
COALESCE(orders.order_id, 'No Orders') AS order_id
FROM
customers
LEFT JOIN
orders
ON
customers.customer_id = orders.customer_id;
In this example, if a customer has no orders, 'No Orders' will be displayed instead of NULL.
Performance Optimization Techniques
Optimizing the performance of queries using LEFT JOIN is crucial for large datasets. Here are some techniques to consider:
1. Indexing
Index the columns used in the JOIN condition to speed up data retrieval. Proper indexing can significantly improve query performance by reducing search times.
2. Analyze Query Execution Plans
Use tools such as EXPLAIN
to analyze your query execution plans. This will help identify any bottlenecks and allow you to make necessary adjustments.
3. Database Normalization
Ensure your database is properly normalized to reduce redundancy and improve performance. However, sometimes denormalization may be beneficial for read-heavy applications.
4. Use Chat2DB for Query Analysis
Using tools like Chat2DB (opens in a new tab) can further enhance your query analysis and optimization efforts. Chat2DB provides AI-driven insights that help you write efficient SQL queries and visualize performance metrics.
Advanced LEFT JOIN Strategies
As you become more comfortable with LEFT JOIN, consider exploring advanced strategies for using it effectively:
1. Chaining Multiple LEFT JOINs
You can chain multiple LEFT JOINs to combine data from several tables in a single query. Here’s an example:
SELECT
customers.customer_id,
customers.customer_name,
orders.order_id,
payments.payment_id
FROM
customers
LEFT JOIN
orders ON customers.customer_id = orders.customer_id
LEFT JOIN
payments ON orders.order_id = payments.order_id;
This query retrieves customer information, their orders, and payment details, even if some customers have no orders or payments.
2. Implementing Conditional Logic
You can use conditional logic within the JOIN clause to filter results based on specific criteria.
Example:
SELECT
customers.customer_id,
customers.customer_name,
orders.order_id
FROM
customers
LEFT JOIN
orders ON customers.customer_id = orders.customer_id AND orders.order_date > '2023-01-01';
This query retrieves customers and their orders, but only for orders placed after January 1, 2023.
3. Aggregate Functions with LEFT JOIN
You can also use aggregate functions and the GROUP BY
clause with LEFT JOIN for complex data analysis. For instance:
SELECT
customers.customer_id,
COUNT(orders.order_id) AS total_orders
FROM
customers
LEFT JOIN
orders ON customers.customer_id = orders.customer_id
GROUP BY
customers.customer_id;
This query counts the total number of orders for each customer, including those with no orders.
Troubleshooting and Debugging LEFT JOIN Queries
Despite its advantages, developers may face challenges when using LEFT JOIN. Here are some common issues and how to resolve them:
1. Unexpected NULL Values
If you encounter unexpected NULL values, ensure that your join conditions are correct. Verify that the columns you are joining on have matching data types and values.
2. Incorrect Data Results
Double-check the relationships between your tables. Make sure that the primary and foreign key relationships are established correctly.
3. Performance Problems
If your queries are running slowly, consider optimizing your indexes and analyzing your execution plans. Look for areas where you can reduce the dataset before applying the LEFT JOIN.
4. Use Chat2DB's Debugging Tools
Chat2DB (opens in a new tab) offers debugging tools that can help you troubleshoot issues with your queries. Its AI capabilities can provide insights into query performance and suggest optimizations.
FAQ
1. What is the difference between LEFT JOIN and INNER JOIN?
LEFT JOIN includes all records from the left table and matched records from the right table, while INNER JOIN only returns records with matching values in both tables.
2. Can I use multiple LEFT JOINs in a single query?
Yes, you can chain multiple LEFT JOINs to retrieve data from several tables.
3. How do I handle NULL values in LEFT JOIN results?
You can use the COALESCE
function to replace NULL values with a default value.
4. What are some performance optimization techniques for LEFT JOIN?
Indexing, analyzing query execution plans, and using tools like Chat2DB (opens in a new tab) can help improve performance.
5. How can Chat2DB assist with MySQL LEFT JOIN queries?
Chat2DB provides AI-driven insights and visualization tools that make writing and optimizing SQL queries easier and more efficient.
By mastering MySQL LEFT JOIN, you can significantly enhance your database queries and analysis capabilities. Explore the potential of tools like Chat2DB (opens in a new tab) to streamline your SQL operations and elevate your database management experience.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!