How to Use the HAVING Clause in SQL: A Comprehensive Guide
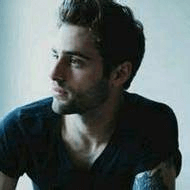
The HAVING clause is a vital component of SQL queries, especially for filtering results after aggregation. In this article, we will explore how to effectively use the HAVING clause in SQL, its relationship with the WHERE clause, practical examples, and advanced techniques for complex queries. Additionally, we will highlight the integration of AI-powered tools like Chat2DB (opens in a new tab) to enhance database management and query execution. Keywords such as HAVING clause, SQL queries, GROUP BY, and aggregate functions will be emphasized for SEO optimization.
Understanding the Role of the HAVING Clause in SQL
The HAVING clause allows users to filter aggregated data after performing grouping operations. Unlike the WHERE clause, which filters records before aggregation, the HAVING clause applies after aggregation. This makes it especially useful in queries that involve GROUP BY and various aggregate functions such as COUNT, SUM, AVG, MIN, and MAX.
For example, in a sales database, if you want to find sales representatives who have made more than a certain number of sales, the following SQL query demonstrates the use of the HAVING clause:
SELECT sales_rep, COUNT(*) AS total_sales
FROM sales
GROUP BY sales_rep
HAVING COUNT(*) > 10;
This query filters out any sales representatives who made 10 or fewer sales, returning only those who meet the criteria.
Key Differences Between HAVING and WHERE
Clause | Filters Before Aggregation | Filters After Aggregation |
---|---|---|
WHERE | Yes | No |
HAVING | No | Yes |
Understanding this distinction is crucial for writing effective SQL queries and ensuring accurate results.
Step-by-step Guide to Implementing the HAVING Clause in SQL Queries
To illustrate how to implement the HAVING clause in SQL queries, let’s walk through a step-by-step example. We will create a sample database table and demonstrate how to use the HAVING clause effectively.
Step 1: Create a Sample Database Table
First, we need to create a sample database table called orders. This table will contain information about various orders made by customers.
CREATE TABLE orders (
order_id INT PRIMARY KEY,
customer_id INT,
order_date DATE,
total_amount DECIMAL(10, 2)
);
Step 2: Insert Sample Data
Next, we’ll insert some sample data into the orders table.
INSERT INTO orders (order_id, customer_id, order_date, total_amount) VALUES
(1, 101, '2023-01-01', 250.00),
(2, 102, '2023-01-05', 150.00),
(3, 101, '2023-01-10', 200.00),
(4, 103, '2023-01-15', 300.00),
(5, 102, '2023-01-20', 50.00);
Step 3: Write a Simple SQL Query Using GROUP BY
Now, let’s write a simple query to calculate the total amount spent by each customer without using the HAVING clause.
SELECT customer_id, SUM(total_amount) AS total_spent
FROM orders
GROUP BY customer_id;
This query will return the total amount spent by each customer. If we want to filter out customers who have spent less than $300, we can introduce the HAVING clause.
Step 4: Introduce the HAVING Clause
Let’s modify the query to include the HAVING clause to filter the results.
SELECT customer_id, SUM(total_amount) AS total_spent
FROM orders
GROUP BY customer_id
HAVING SUM(total_amount) >= 300;
In this modified query, only customers who have spent $300 or more will be included in the results.
Code Snippets for Different Aggregate Functions
Here are additional examples showcasing the versatility of the HAVING clause with different aggregate functions:
Using COUNT
SELECT customer_id, COUNT(order_id) AS total_orders
FROM orders
GROUP BY customer_id
HAVING COUNT(order_id) > 1;
Using AVG
SELECT customer_id, AVG(total_amount) AS average_spent
FROM orders
GROUP BY customer_id
HAVING AVG(total_amount) > 100;
Common Mistakes and How to Avoid Them When Using the HAVING Clause
While the HAVING clause is a powerful tool, many programmers make common mistakes. Here are some frequent pitfalls and how to avoid them:
Mistake 1: Confusing HAVING with WHERE
One of the most common mistakes is confusing the HAVING clause with the WHERE clause. Remember, HAVING is used for filtering aggregated data, while WHERE filters individual records before aggregation.
Mistake 2: Using HAVING Without Aggregate Functions
Another mistake is using the HAVING clause without any aggregate functions. The HAVING clause should always be used in conjunction with an aggregate function.
Corrective Measures
To avoid these mistakes, always check the context of your HAVING clause usage. Here’s an example of incorrect usage followed by the corrected version:
Incorrect Usage
SELECT customer_id
FROM orders
GROUP BY customer_id
HAVING customer_id = 101; -- This is incorrect
Corrected Version
SELECT customer_id
FROM orders
GROUP BY customer_id
HAVING COUNT(order_id) > 0; -- This is correct
Advanced Techniques for Using the HAVING Clause in Complex SQL Queries
Once comfortable with the HAVING clause basics, you can explore more advanced techniques. Here are ways to leverage the HAVING clause for complex SQL queries.
Combining HAVING with Multiple Aggregate Functions
You can use the HAVING clause with multiple aggregate functions in a single query. For example:
SELECT customer_id, COUNT(order_id) AS total_orders, AVG(total_amount) AS average_spent
FROM orders
GROUP BY customer_id
HAVING COUNT(order_id) > 1 AND AVG(total_amount) > 100;
Using HAVING with Other SQL Clauses
The HAVING clause can also be combined with other SQL clauses such as WHERE, ORDER BY, and JOIN. Here’s an example:
SELECT o.customer_id, SUM(o.total_amount) AS total_spent
FROM orders o
JOIN customers c ON o.customer_id = c.id
WHERE c.status = 'active'
GROUP BY o.customer_id
HAVING SUM(o.total_amount) >= 300
ORDER BY total_spent DESC;
Performance Considerations
When working with large datasets, be mindful of the performance implications of using the HAVING clause. Optimizing your queries by ensuring efficient use of aggregate functions can help improve performance.
Real-world Applications and Use Cases of the HAVING Clause
The HAVING clause has numerous real-world applications across various industries. Here are practical scenarios where it can be effectively utilized:
Reporting and Analytics
In reporting and analytics, the HAVING clause is frequently used to generate specific sales reports. For instance, businesses can filter sales representatives who have achieved targets over a specific period.
Financial Services
In the financial sector, the HAVING clause can be used to calculate customer balances above a certain threshold, providing valuable insights for decision-making.
E-commerce
E-commerce platforms can use the HAVING clause to filter products based on aggregated reviews or ratings, allowing customers to quickly find top-rated items.
Data Warehousing
In data warehousing, the HAVING clause is beneficial for summarizing large volumes of data, helping organizations extract actionable insights from their data.
Tips and Tricks for Mastering the HAVING Clause in SQL
To truly master the HAVING clause in SQL, here are practical tips and tricks:
Understand Your Data Structure
Before implementing the HAVING clause, ensure that you have a solid understanding of your data structure and the relationships between tables. This knowledge will help you write more efficient queries.
Write Clean and Efficient SQL Queries
Aim to write clean, efficient SQL queries that are easy to read and maintain. This practice will save you time in the long run.
Debugging and Optimization
If you encounter issues with your queries, take the time to debug and optimize them. Use tools like Chat2DB (opens in a new tab) to simplify this process, as it offers AI-powered SQL editing and debugging features.
Explore Learning Resources
To enhance your SQL skills, consider exploring various learning resources, including online courses, tutorials, and community forums. Regular practice and experimentation will deepen your understanding of the HAVING clause.
FAQ
-
What is the HAVING clause in SQL? The HAVING clause is used to filter results after aggregation in SQL queries, typically used with GROUP BY.
-
How does HAVING differ from WHERE? The WHERE clause filters records before aggregation, while the HAVING clause filters records after aggregation.
-
Can I use HAVING without aggregate functions? No, the HAVING clause should always be used in conjunction with aggregate functions.
-
What are some common mistakes when using HAVING? Common mistakes include confusing HAVING with WHERE and using it without aggregate functions.
-
How can Chat2DB help with SQL queries? Chat2DB (opens in a new tab) is an AI-powered database management tool that simplifies SQL query creation, provides intelligent editing features, and enhances overall database management efficiency. With its user-friendly interface and natural language processing capabilities, Chat2DB allows users to generate complex SQL queries quickly and effectively, making it a superior choice compared to other tools.
By mastering the HAVING clause and utilizing tools like Chat2DB (opens in a new tab), you can significantly improve your SQL proficiency and efficiency in managing data.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!