How to Effectively Implement Database Constraints in Your System
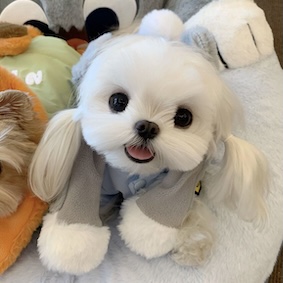
Implementing database constraints is vital for maintaining data integrity and ensuring that your database operates efficiently. Database constraints, including primary keys, foreign keys, unique constraints, check constraints, not null constraints, and default constraints, are essential in preventing invalid data entry and ensuring that your data adheres to specific rules. This article explores the various types of database constraints, practical implementation strategies, common challenges, and advanced topics surrounding them. Additionally, we will examine how tools like Chat2DB (opens in a new tab), with its AI capabilities, can enhance the management of database constraints effectively.
Understanding Database Constraints
Database constraints are rules that enforce specific conditions on the data in a database. They safeguard the accuracy and integrity of data stored in relational databases. By employing constraints, developers ensure that the database adheres to defined standards, preventing erroneous entries.
Types of Database Constraints
Each constraint serves a unique purpose and helps maintain data integrity:
Type of Constraint | Purpose | Example |
---|---|---|
Primary Key | Uniquely identifies rows in a table | user_id INT PRIMARY KEY |
Foreign Key | Maintains relationships between tables | FOREIGN KEY (user_id) REFERENCES Users(user_id) |
Unique | Ensures all values in a column are distinct | email VARCHAR(100) UNIQUE |
Check | Enforces specific conditions on values | CHECK (age >= 18) |
Not Null | Ensures a column cannot have NULL values | username VARCHAR(50) NOT NULL |
Default | Assigns a default value if none is provided | DEFAULT 0.00 FOR price |
Detailed Explanation of Each Constraint
-
Primary Key Constraints: A primary key uniquely identifies each row in a table, ensuring that no two rows have the same value in the primary key column. For example, in a
Users
table, theuser_id
field may serve as a primary key.CREATE TABLE Users ( user_id INT PRIMARY KEY, username VARCHAR(50) NOT NULL, email VARCHAR(100) NOT NULL );
-
Foreign Key Constraints: Foreign keys create relationships between two tables, ensuring that a value in one table corresponds to a value in another, thus maintaining referential integrity. For instance, if you have an
Orders
table, it might reference theUsers
table.CREATE TABLE Orders ( order_id INT PRIMARY KEY, user_id INT, FOREIGN KEY (user_id) REFERENCES Users(user_id) );
-
Unique Constraints: Unique constraints ensure that all values in a column are distinct, which is essential for fields such as email addresses in a
Users
table, where duplicates should not exist.CREATE TABLE Users ( user_id INT PRIMARY KEY, username VARCHAR(50) NOT NULL, email VARCHAR(100) UNIQUE );
-
Check Constraints: Check constraints enforce specific conditions on a column's value. For instance, you can ensure that the age of a user must be greater than 18.
CREATE TABLE Users ( user_id INT PRIMARY KEY, username VARCHAR(50) NOT NULL, age INT CHECK (age >= 18) );
-
Not Null Constraints: Not null constraints ensure that a column cannot have a NULL value, which is crucial for mandatory fields such as
username
. -
Default Constraints: Default constraints assign a default value to a column when no value is provided during data insertion.
Implementing Constraints in Your System
Implementing database constraints involves a structured approach to defining these rules within your database schema. Below are practical steps and examples for integrating various constraints.
Defining Primary Key Constraints
When creating a table, define primary key constraints to ensure unique identification of each row.
CREATE TABLE Products (
product_id INT PRIMARY KEY,
product_name VARCHAR(100) NOT NULL,
price DECIMAL(10, 2) NOT NULL
);
Adding Foreign Key Constraints
To establish relationships between tables, add foreign key constraints. This ensures data consistency across related tables.
ALTER TABLE Orders
ADD CONSTRAINT fk_user
FOREIGN KEY (user_id) REFERENCES Users(user_id);
Implementing Unique Constraints
To add uniqueness to a column, implement unique constraints directly when creating or altering a table.
ALTER TABLE Users
ADD CONSTRAINT unique_username UNIQUE (username);
Using Check Constraints
Check constraints can be added to enforce business rules, such as limiting the values in a specific range.
ALTER TABLE Users
ADD CONSTRAINT check_age CHECK (age >= 0);
Setting Not Null Constraints
To ensure mandatory fields are populated, add not null constraints.
ALTER TABLE Users
MODIFY username VARCHAR(50) NOT NULL;
Applying Default Constraints
Default constraints streamline data entry by providing default values.
ALTER TABLE Products
ADD CONSTRAINT default_price DEFAULT 0.00 FOR price;
Challenges and Best Practices
While implementing database constraints can significantly enhance data integrity, developers often face challenges. Understanding these challenges and adopting best practices can lead to improved database management.
Performance Implications
Constraints can affect database performance, particularly with large datasets. It's essential to analyze the performance impact and optimize queries accordingly.
Handling Constraint Violations
When users attempt to enter data that violates constraints, it can lead to errors. Implementing user-friendly error messages can help guide users in correcting their inputs.
Impact on Database Updates and Deletions
Constraints can complicate updates and deletions, especially if foreign key relationships are involved. Plan for cascading actions and the possible need for ON DELETE CASCADE options.
Importance of Thorough Testing
Testing your database schema and constraints is vital to ensure they work as intended. Thorough testing can catch potential issues before deployment.
Documentation
Maintaining clear documentation regarding the constraints in your database can help future developers understand the structure and rules governing the data.
Tools for Managing Constraints
Using database management tools simplifies the process of implementing and managing constraints. Tools like Chat2DB (opens in a new tab) provide visual interfaces and AI-driven features to enhance database management, making it easier to define and adjust constraints as needed.
Advanced Topics in Database Constraints
As database systems evolve, understanding advanced concepts related to constraints can further enhance your database's robustness and efficiency.
Composite Keys
Composite keys consist of two or more columns that together uniquely identify a record. They are beneficial in complex databases where single columns may not suffice.
CREATE TABLE CourseEnrollments (
student_id INT,
course_id INT,
PRIMARY KEY (student_id, course_id)
);
Cascading Actions
Cascading actions in foreign key constraints allow for automatic updates or deletions in related tables, preserving data integrity.
ALTER TABLE Orders
ADD CONSTRAINT fk_user
FOREIGN KEY (user_id) REFERENCES Users(user_id) ON DELETE CASCADE;
Deferrable Constraints
Deferrable constraints provide flexibility by allowing constraints to be checked at a later point in time, rather than immediately.
Indexing for Performance
Indexing is critical for optimizing constraint performance, particularly with large datasets. Proper indexing can drastically improve query performance.
Partial Constraints
Partial constraints allow you to apply constraints to a subset of rows, offering more granularity in data validation.
Constraints in Distributed Databases
Understanding how to manage constraints in distributed databases is essential for maintaining global data integrity across multiple locations.
Regular Reviews and Updates
Regularly reviewing and updating constraint definitions ensures they remain relevant and effective as business requirements change.
Tools and Technologies for Managing Database Constraints
Several tools and technologies are available to assist in managing database constraints efficiently.
Database Management Systems (DBMS)
Most DBMS, such as MySQL, PostgreSQL, and Oracle, provide built-in functionalities for implementing constraints within database schemas.
Chat2DB
Chat2DB (opens in a new tab) stands out as an AI-driven database management tool that simplifies constraint management. Its intuitive interface allows users to visualize and manage constraints easily. The AI capabilities of Chat2DB enhance the overall experience, offering natural language processing to generate SQL queries, making it accessible even to those with limited technical expertise. Users can streamline their workflow, automate routine tasks, and leverage intelligent suggestions to optimize their database management processes.
SQL Statements
Using SQL statements effectively is crucial for defining and managing constraints. Knowledge of SQL syntax and structure ensures proper implementation.
Version Control Systems
Version control systems can help manage schema changes, including constraints, allowing teams to track modifications and revert to previous iterations if necessary.
Automated Testing Tools
Automated testing tools play a significant role in validating constraint implementations, ensuring they function as expected without introducing errors.
In conclusion, effectively implementing database constraints is essential for maintaining data integrity and ensuring the reliability of your systems. Utilizing tools like Chat2DB (opens in a new tab) can significantly enhance your ability to manage these constraints with ease and efficiency.
FAQ
-
What are database constraints? Database constraints are rules that enforce conditions on the data in a database to ensure data integrity and validity.
-
What types of constraints are commonly used? Common types include primary keys, foreign keys, unique constraints, check constraints, not null constraints, and default constraints.
-
How do I implement constraints in a SQL database? Constraints can be implemented using SQL statements during table creation or by altering existing tables to add constraints.
-
What challenges might I face when using constraints? Challenges include performance implications, handling constraint violations, and maintaining data integrity during updates and deletions.
-
How can Chat2DB help with database constraints? Chat2DB provides AI-driven features that simplify the management of database constraints, allowing for easy visualization, SQL generation, and overall enhanced database management.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!