How to Efficiently Implement SQL Upsert Operations for Data Management
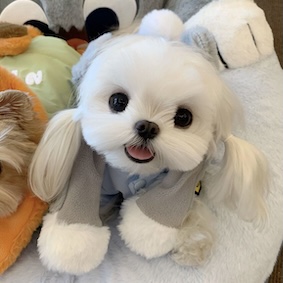
Efficiently implementing SQL Upsert operations is essential for modern data management strategies. The term 'upsert' combines 'update' and 'insert', allowing developers to execute a single command that either inserts a new record or updates an existing one based on specific conditions. This article explores the significance of SQL upsert operations in data management, various implementations across different SQL databases, optimization strategies, best practices, and common pitfalls. Moreover, we will highlight how tools like Chat2DB (opens in a new tab) enhance the upsert process through advanced AI capabilities.
Understanding SQL Upsert Operations
SQL upsert operations streamline data handling by enabling developers to manage database records efficiently. The fundamental idea behind upsert is that it allows for the simultaneous execution of insertion and updating actions, thus minimizing redundancy and maintaining data integrity. This is particularly useful in scenarios with unique constraints, such as user accounts or product inventories.
Basic Syntax of SQL Upsert
While the syntax for upsert can vary across different databases, the core concept remains consistent. Below are examples of how different SQL databases implement upsert functionality.
Database | Upsert Syntax |
---|---|
PostgreSQL | sql INSERT INTO products (product_id, product_name, stock) VALUES (1, 'Gadget', 100) ON CONFLICT (product_id) DO UPDATE SET stock = stock + EXCLUDED.stock; |
MySQL | sql INSERT INTO products (product_id, product_name, stock) VALUES (1, 'Gadget', 100) ON DUPLICATE KEY UPDATE stock = stock + VALUES(stock); |
Microsoft SQL | sql MERGE INTO products AS target USING (SELECT 1 AS product_id, 'Gadget' AS product_name, 100 AS stock) AS source ON target.product_id = source.product_id WHEN MATCHED THEN UPDATE SET target.stock = target.stock + source.stock WHEN NOT MATCHED THEN INSERT (product_id, product_name, stock) VALUES (source.product_id, source.product_name, source.stock); |
Challenges of Implementing Upsert Operations
While upsert operations are powerful, developers may encounter several challenges. For instance, managing primary key conflicts effectively is essential to prevent transactions from failing. Additionally, handling race conditions in concurrent environments can lead to data integrity issues. These challenges can be mitigated with proper database management tools, such as Chat2DB (opens in a new tab), which provide intuitive interfaces and advanced error handling capabilities.
The Role of SQL Upsert in Data Management
The implementation of SQL upsert operations plays a pivotal role in efficient data management. By allowing a single command to handle both insertion and updating of records, upserts minimize the need for multiple operations, thus simplifying application code.
Atomic Transactions and Data Integrity
Upsert operations maintain atomicity in transactions, ensuring that either all parts of the operation are executed or none at all. This is crucial for maintaining data integrity across various applications, such as e-commerce platforms, where inventory management relies heavily on accurate data representation.
Impact on Database Performance
Efficient upsert operations can significantly enhance database performance. By reducing the complexity of application logic and the number of queries sent to the database, developers can improve response times and reduce overall load on the database system. Proper indexing can further optimize the performance of upsert operations. For example, ensuring that unique constraints are indexed can speed up lookup times during both insertions and updates.
Real-World Applications of Upsert
Upsert functionality is widely used in real-world applications. For instance, in e-commerce systems, managing product inventories requires frequent updates and insertions. The ability to handle these operations with a single upsert command streamlines workflows and reduces the risk of data inconsistencies.
Implementing SQL Upsert in Different SQL Databases
As previously mentioned, SQL databases have different implementations of the upsert operation. Below, we delve deeper into how various systems handle this functionality.
PostgreSQL Upsert Implementation
PostgreSQL's ON CONFLICT
clause is particularly powerful. It allows for conflict resolution strategies, which can be customized based on the application's requirements. Here’s an example of a more complex scenario:
INSERT INTO products (product_id, product_name, stock)
VALUES (1, 'Gadget', 100)
ON CONFLICT (product_id)
DO UPDATE SET stock = GREATEST(products.stock, EXCLUDED.stock);
In this scenario, PostgreSQL updates the stock to the maximum value between the existing stock and the new value provided.
MySQL Upsert Implementation
MySQL's INSERT ... ON DUPLICATE KEY UPDATE
mechanism provides a straightforward approach to handle upserts. It is essential to ensure that the relevant columns are indexed:
CREATE TABLE products (
product_id INT PRIMARY KEY,
product_name VARCHAR(100),
stock INT
);
Inserting or updating a product can be efficiently done as follows:
INSERT INTO products (product_id, product_name, stock)
VALUES (1, 'Gadget', 100)
ON DUPLICATE KEY UPDATE stock = stock + 50;
Microsoft SQL Server Upsert Implementation
The MERGE
statement in SQL Server is versatile but can introduce complexity. It is crucial to handle potential conflicts carefully. Here’s an example of handling multiple conditions:
MERGE INTO products AS target
USING (SELECT 1 AS product_id, 'Gadget' AS product_name, 100 AS stock) AS source
ON target.product_id = source.product_id
WHEN MATCHED AND target.stock < 100 THEN
UPDATE SET target.stock = 100
WHEN NOT MATCHED THEN
INSERT (product_id, product_name, stock)
VALUES (source.product_id, source.product_name, source.stock);
Optimizing SQL Upsert for Performance
To achieve optimal performance from upsert operations, several strategies can be employed:
Indexing Strategies
Proper indexing can dramatically improve the performance of upsert operations. By ensuring that columns involved in conflict resolution are indexed, SQL databases can quickly locate existing records rather than performing full table scans.
Batch Processing
When handling large datasets, batch processing can significantly enhance performance. Instead of executing multiple individual upsert commands, grouping operations into batches reduces the number of transactions and overhead.
Database Normalization
Normalization can affect the efficiency of upsert operations. While it is essential to reduce redundancy, overly normalized databases may lead to complex joins that can hinder performance. Striking a balance between normalization and performance is critical.
Monitoring and Profiling
Regular monitoring and profiling of upsert queries can help identify bottlenecks. By utilizing tools like Chat2DB (opens in a new tab), developers can gain insights into query performance metrics and optimize their upsert strategies accordingly.
Best Practices and Common Pitfalls in SQL Upsert
When implementing upsert operations, following best practices is vital to ensure efficiency and effectiveness:
Understanding Syntax and Behavior
Each SQL database has its own upsert syntax and behavior. Familiarizing oneself with these idiosyncrasies is crucial to avoid unexpected errors.
Constraints and Indexes
Properly defining constraints and indexes ensures the success of upsert operations. For example, unique constraints must be indexed to avoid conflicts during insertions.
Handling Primary Key Conflicts
Managing primary key conflicts is essential. Developers must ensure that their application logic can handle cases where multiple transactions attempt to insert the same key.
Avoiding Race Conditions
In concurrent environments, race conditions can lead to inconsistencies. Implementing locking mechanisms or using serializable transactions can mitigate these issues.
Testing Thoroughly
Before deploying upsert operations in production, thorough testing in a staging environment is essential. This helps identify potential pitfalls and ensure that the operations perform as expected.
Utilizing Advanced Database Tools
Tools like Chat2DB (opens in a new tab) can significantly enhance the management of upsert operations. With AI-driven features, developers can streamline their database interactions, generate optimized SQL queries, and visualize data more effectively. Chat2DB's capabilities include natural language processing for query generation, intelligent error handling, and a user-friendly interface that simplifies complex database tasks.
Conclusion
In this article, we explored the significance of SQL upsert operations in data management, various implementations across different SQL databases, optimization strategies, and best practices. As the demand for efficient data handling continues to grow, leveraging advanced tools like Chat2DB (opens in a new tab) can help developers enhance their upsert strategies and achieve optimal performance in their database applications. By transitioning to Chat2DB, you can utilize its cutting-edge AI features to improve your database management experience.
FAQs
-
What is an SQL Upsert? SQL Upsert is an operation that allows you to insert a new record or update an existing one based on whether a conflict occurs on specified constraints.
-
How does PostgreSQL handle Upsert? PostgreSQL uses the
ON CONFLICT
clause to manage upsert operations, allowing developers to specify how to handle conflicts. -
Can I use Upsert in MySQL? Yes, MySQL supports upsert functionality through the
INSERT ... ON DUPLICATE KEY UPDATE
syntax. -
What are the performance considerations for Upsert operations? Important considerations include proper indexing, batch processing, and continuous monitoring of query performance.
-
How can Chat2DB assist with Upsert operations? Chat2DB enhances upsert management through AI-driven insights, optimized SQL generation, and visualization tools, making database management more efficient.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!