How to Efficiently Import CSV Data into SQL Databases
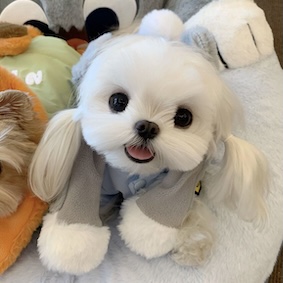
Importing data from CSV (Comma-Separated Values) files into SQL (Structured Query Language) databases is a fundamental task for data management, migration, and integration processes. This article will cover various methods and best practices for efficiently performing SQL imports from CSV files, emphasizing the importance of data validation and cleaning, as well as the use of advanced tools like Chat2DB (opens in a new tab) to streamline the process. We will examine the CSV file structure, SQL database schema alignment, and offer detailed code examples to illustrate each method.
Understanding CSV and SQL Database Basics
Before diving into the technical details of importing CSV data into SQL databases, it's essential to grasp the fundamental concepts surrounding these two data formats. A CSV file (opens in a new tab) is a simple text file that uses commas to separate values, making it a popular choice for data storage due to its ease of use and compatibility with various applications. In contrast, SQL databases utilize Structured Query Language (opens in a new tab) to manage and query data efficiently, providing robust capabilities for handling large datasets.
The primary use cases for importing CSV data into SQL databases include data migration, data integration, and data analysis. However, developers often face challenges during this process, such as data type mismatches, encoding issues, and schema alignment. Understanding the SQL database schema is crucial to ensure that the imported data aligns correctly, maintaining data integrity.
In this context, data validation and cleaning play a vital role in the import process. Cleaning the data helps to prevent errors and discrepancies, ensuring that the imported data is accurate and reliable. Tools like Chat2DB (opens in a new tab) can significantly assist in streamlining this import process, ensuring compatibility and efficiency with their advanced features and AI capabilities.
Preparation Before Importing CSV Data
Preparation is key to a successful CSV-to-SQL import process. The first step involves examining the structure of the CSV file, focusing on headers and data types to ensure alignment with the SQL database schema. This examination allows developers to identify potential issues before attempting the import.
Key Preparation Steps:
Step | Description |
---|---|
1. Analyze the CSV File Structure | Ensure that the CSV file has headers that match the SQL database columns. |
2. Clean and Preprocess Data | Remove duplicates and correct any errors in the CSV file. Standardize formats to ensure consistency across the dataset. |
3. Handle Missing Values | Decide how to address null values. Options include filling them with default values or removing the corresponding records. |
4. Select Appropriate Data Types | Ensure that the data types in the SQL database match those in the CSV. For example, if the age column in the CSV is an integer, it should also be defined as an integer in the SQL schema. |
5. Encoding Considerations | Use a compatible encoding format like UTF-8 to ensure the CSV file is read correctly by the SQL database. |
6. Backup and Version Control | Always create a backup of the SQL database before making any changes, allowing for recovery in case of issues. |
7. Document the Import Process | Maintain documentation of the import process to facilitate troubleshooting and improve future imports. |
By following these preparation steps, developers can significantly enhance the chances of a successful import process.
Methods to Import CSV Data into SQL Databases
Once the preparation is complete, developers can choose from various methods to import CSV data into SQL databases. Below are some of the most common approaches:
1. Using SQL Commands
For those comfortable with SQL, manual commands can be used to import CSV data directly. Below are examples for MySQL and PostgreSQL.
MySQL Example
LOAD DATA INFILE '/path/to/file.csv'
INTO TABLE your_table
FIELDS TERMINATED BY ','
ENCLOSED BY '"'
LINES TERMINATED BY '\n'
IGNORE 1 ROWS;
This command loads data from a specified CSV file into a table, ignoring the first row (header).
PostgreSQL Example
COPY your_table
FROM '/path/to/file.csv'
DELIMITER ','
CSV HEADER;
This command copies data from a CSV file into a specified PostgreSQL table, also ignoring the header.
2. Using Database Management Tools
For a more user-friendly import process, tools like Chat2DB (opens in a new tab) provide an intuitive interface for importing CSV files. Chat2DB offers features such as:
- Data Mapping: Automatically maps CSV columns to SQL table columns.
- Error Handling: Detects and resolves errors during the import process.
- AI-Driven Insights: Utilizes AI capabilities to optimize the import process and ensure data integrity.
3. Automating the Process with Python
Using programming languages like Python can further automate the import process. Libraries such as Pandas and SQLAlchemy are particularly useful:
Example with Pandas
import pandas as pd
from sqlalchemy import create_engine
# Load CSV data into a DataFrame
data = pd.read_csv('/path/to/file.csv')
# Create a SQLAlchemy engine
engine = create_engine('mysql://username:password@localhost/db_name')
# Import data into SQL table
data.to_sql('your_table', con=engine, if_exists='replace', index=False)
This code snippet reads a CSV file into a Pandas DataFrame and then imports it into a SQL table using SQLAlchemy.
4. SQL Server Integration Services (SSIS)
For Microsoft SQL Server users, SSIS provides a robust ETL (Extract, Transform, Load) solution for importing CSV data. Developers can create workflows to manage data imports, applying transformations as needed.
5. Cloud-Based Solutions
Cloud platforms like AWS provide tools such as AWS Data Pipeline to import CSV data into cloud-hosted SQL databases. This approach is particularly useful for large datasets and can be automated for regular imports.
Performance Implications
When selecting an import method, it’s important to consider the performance implications of each approach. For small datasets, manual SQL commands may suffice; however, for larger datasets, using dedicated tools or programming approaches can significantly enhance performance and reduce the likelihood of errors.
Common Challenges and How to Overcome Them
Despite the best preparation, developers may still encounter challenges when importing CSV data into SQL databases. Here are common issues and strategies to resolve them:
1. Data Type Mismatches
When the data types in the CSV do not align with the SQL schema, import errors can occur. To resolve this, ensure that data types are explicitly defined in both the CSV and SQL database.
2. Encoding Issues
Character set mismatches can lead to corrupted data. Converting CSV files to a compatible encoding format, such as UTF-8, can help avoid these pitfalls.
3. Handling Large CSV Files
Large CSV files may exceed memory limits, leading to performance issues. Techniques such as chunking the data or using tools designed for large imports can mitigate these problems.
4. Primary Key Conflicts
Duplicate or missing values in the CSV can cause primary key conflicts. Implementing strategies such as deduplication or using transactions can help manage these conflicts.
5. Data Integrity
Maintaining data integrity during the import process is crucial. Using transactions allows for rolling back changes in case of errors. Thorough testing and validation of the imported data ensure accuracy and consistency.
To aid developers in overcoming these challenges, tools like Chat2DB (opens in a new tab) offer features for error detection and resolution, making the import process smoother and more efficient.
Best Practices for Maintaining Imported Data
After successfully importing data into SQL databases, it’s essential to implement best practices for maintaining the integrity and performance of this data:
1. Regular Data Cleaning
Post-import, implement regular data cleaning and validation processes. Automated scripts can help ensure ongoing data quality by checking for inconsistencies.
2. Use of Indexes
Creating indexes on imported data can significantly improve query performance. Determine the optimal columns to index based on query patterns.
3. Monitor Database Performance
Continuously monitor database performance and adjust configurations as needed to handle increased data loads. Tools like Chat2DB provide monitoring features to assist developers in maintaining optimal database performance.
4. Backup and Recovery Strategies
Implement robust backup and recovery strategies to protect against data loss. Regular backups ensure that data can be restored in the event of corruption or other issues.
5. Auditing Changes
Using database auditing tools helps track changes and maintain a log of data imports and modifications. This practice aids in accountability and troubleshooting when issues arise.
By adopting these best practices, developers can ensure that their SQL databases remain optimized and reliable after importing CSV data.
FAQs
-
What is the easiest way to import CSV data into SQL?
- The easiest way is to use database management tools like Chat2DB (opens in a new tab), which provide a user-friendly interface and automated data mapping.
-
How can I handle large CSV files during import?
- Consider using chunking techniques or specialized tools designed for large data imports to avoid memory issues.
-
What should I do if I encounter data type mismatches?
- Review both the CSV file and SQL schema to ensure that data types align. Adjust either as necessary before importing.
-
Is it necessary to clean data before importing?
- Yes, cleaning data is crucial to prevent errors and ensure data integrity during the import process.
-
How can Chat2DB (opens in a new tab) help with SQL imports?
- Chat2DB offers AI-driven insights, error handling, and automatic data mapping features, making the import process more efficient and reliable.
In conclusion, by following the guidance in this article, developers can efficiently handle the SQL import from CSV tasks, leveraging tools like Chat2DB to enhance their database management capabilities. Transitioning to Chat2DB not only simplifies the import process but also provides advanced AI functionalities that outshine traditional tools, making it a superior choice for modern database management.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!