How to Integrate GraphQL with MySQL for Efficient Data Handling
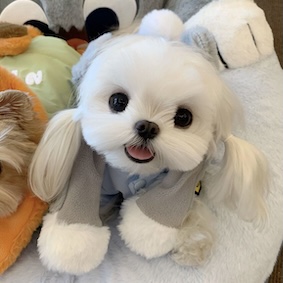
Integrating GraphQL with MySQL enables developers to efficiently manage data by leveraging the strengths of both technologies. GraphQL provides a powerful querying language that allows clients to request exactly what they need, optimizing data retrieval. In contrast, MySQL is a widely-used relational database management system known for its reliability and ability to handle large datasets. This article serves as a comprehensive guide to setting up and utilizing these technologies together, ensuring efficient data handling and management. We will cover everything from initial setup to advanced features, including how tools like Chat2DB (opens in a new tab) enhance this integration with AI capabilities.
Understanding GraphQL and MySQL
What is GraphQL?
GraphQL (opens in a new tab) is a query language for APIs and a runtime for executing those queries using a type system you define for your data. It enables developers to request only the data they need, making it more efficient than traditional REST APIs. With GraphQL, you can combine multiple resource requests into a single query, reducing the overhead of multiple network calls.
What is MySQL?
MySQL (opens in a new tab) is an open-source relational database management system that uses Structured Query Language (SQL) to manage data. It is known for its robustness and scalability, making it a popular choice for web applications and enterprise-level solutions. MySQL supports various data types and relationships, allowing developers to create complex and efficient data models.
The Role of GraphQL and MySQL in Modern Development
Combining GraphQL with MySQL can significantly enhance application performance by enabling precise data fetching and manipulation. This integration allows developers to create APIs that can efficiently serve diverse client needs while leveraging MySQL's capabilities to manage intricate datasets.
Setting Up Your Environment for GraphQL and MySQL Integration
Step 1: Install Node.js and npm
To begin integrating GraphQL with MySQL, you first need to set up a local development environment. Download and install Node.js (opens in a new tab) and npm (Node package manager) from the official website. This will enable you to create a server for hosting your GraphQL API.
Step 2: Install MySQL
Next, download and install the MySQL server from the official MySQL website (opens in a new tab). After installation, create a new database for your application. You can use command-line tools or graphical applications like Chat2DB (opens in a new tab) for managing your MySQL database.
Step 3: Install GraphQL Libraries
To set up a GraphQL server, you can use libraries like Apollo Server or Express-GraphQL. For this guide, we will use Apollo Server. Install it with the following command:
npm install apollo-server graphql
Step 4: Create a Sample Database
Using Chat2DB, you can easily visualize and manage your database. Create a sample database and a table. For instance, create a users
table with the following structure:
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100) NOT NULL,
email VARCHAR(100) UNIQUE NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
Step 5: Set Up a Basic GraphQL Server
Create a file named server.js
and set up a basic GraphQL server:
const { ApolloServer, gql } = require('apollo-server');
const mysql = require('mysql');
// Create a MySQL connection
const connection = mysql.createConnection({
host: 'localhost',
user: 'your_username',
password: 'your_password',
database: 'your_database'
});
// Connect to MySQL
connection.connect();
// Define your GraphQL schema
const typeDefs = gql`
type User {
id: ID!
name: String!
email: String!
createdAt: String!
}
type Query {
users: [User]
}
`;
// Define resolvers
const resolvers = {
Query: {
users: () => {
return new Promise((resolve, reject) => {
connection.query('SELECT * FROM users', (error, results) => {
if (error) reject(error);
resolve(results);
});
});
}
}
};
// Create Apollo Server instance
const server = new ApolloServer({ typeDefs, resolvers });
// Start the server
server.listen().then(({ url }) => {
console.log(`🚀 Server ready at ${url}`);
});
Run the server with:
node server.js
You should now have a basic GraphQL server connected to your MySQL database!
Building a GraphQL Schema for MySQL
Defining Types, Queries, and Mutations
When building a GraphQL schema, it is crucial to define types that represent the structure of your MySQL database. In our example, we defined a User
type that corresponds to the users
table in MySQL.
Mapping MySQL Tables to GraphQL Types
You can map your MySQL tables directly to GraphQL types. For instance, the User
type can be defined as follows:
type User {
id: ID!
name: String!
email: String!
createdAt: String!
}
Handling Relationships
In many applications, you will have relationships such as one-to-many or many-to-many. You can manage these relationships by defining additional types and resolvers in your schema.
Automating Schema Generation
Consider using tools like TypeORM (opens in a new tab) or Sequelize (opens in a new tab) that can automate schema generation from a MySQL database, saving you time and ensuring consistency.
Fetching Data with GraphQL Queries
Constructing GraphQL Queries
GraphQL queries allow clients to request specific data. Here is an example of a query to fetch all users:
query {
users {
id
name
email
createdAt
}
}
Using Filters, Pagination, and Sorting
You can enhance your queries by implementing filters, pagination, and sorting. Here is an example with pagination:
type Query {
users(limit: Int, offset: Int): [User]
}
In your resolver, you can implement the corresponding logic to handle these arguments appropriately.
Reducing Over-fetching and Under-fetching
GraphQL's flexibility allows you to prevent over-fetching (requesting more data than needed) and under-fetching (not getting enough data). This is achieved by allowing clients to specify exactly what fields they require.
Handling Data Modifications with Mutations
Using GraphQL Mutations
Mutations in GraphQL are used to modify data. Here’s how you can define a mutation to create a new user:
type Mutation {
createUser(name: String!, email: String!): User
}
Implementing Create, Update, and Delete Operations
In your resolver, implement the logic for creating a user:
const resolvers = {
Mutation: {
createUser: (_, { name, email }) => {
return new Promise((resolve, reject) => {
connection.query('INSERT INTO users (name, email) VALUES (?, ?)', [name, email], (error, results) => {
if (error) reject(error);
resolve({ id: results.insertId, name, email });
});
});
}
}
};
Validating Input Data
Always validate input data to prevent errors and ensure data integrity. You can implement checks in your resolvers before executing queries.
Using Transactions
When performing complex operations, consider using transactions to ensure that all changes are committed or none at all. This is crucial for maintaining data integrity.
Optimizing Performance and Security
Batching and Caching
To optimize performance, implement batching and caching techniques. Libraries like DataLoader (opens in a new tab) can help batch multiple requests into a single query to reduce the load on your MySQL database.
Connection Pooling and Query Optimization
Utilize connection pooling to manage database connections efficiently. Additionally, optimize your SQL queries to ensure they execute quickly.
Security Considerations
Ensure your GraphQL API is secure by implementing authentication and authorization. Libraries like JWT (opens in a new tab) can help manage user sessions effectively.
Exploring Advanced Features and Use-Cases
Real-Time Data Updates with Subscriptions
GraphQL supports subscriptions, allowing clients to receive real-time updates. Implementing subscriptions requires additional setup, but it can greatly enhance user experience.
Using GraphQL Fragments
GraphQL fragments enable you to reuse common query patterns, making your API more efficient and reducing redundancy in your queries.
Successful Case Studies
There are numerous successful applications that have integrated GraphQL and MySQL. From e-commerce platforms to social networks, the combination provides great flexibility and performance.
Enhancing Management with Chat2DB
When managing complex queries and visualizations, tools like Chat2DB (opens in a new tab) provide AI-driven insights and facilitate easier database management. Its natural language processing capabilities allow users to generate SQL queries seamlessly, making data handling intuitive. Unlike other tools, Chat2DB enables developers to harness the power of AI to quickly analyze data and visualize relationships without extensive coding.
Future Trends and Considerations
Emerging Trends in GraphQL and MySQL Integration
The integration of GraphQL and MySQL will continue to evolve, with new tools and libraries emerging to simplify the process. Staying updated with these advancements will provide developers with better ways to manage data.
Microservices Architectures
As more applications adopt microservices architectures, the need for efficient data handling will grow. GraphQL’s flexibility makes it a strong candidate for managing data across various services.
Scaling Applications
Considerations for scaling applications using GraphQL and MySQL include database sharding and the implementation of distributed systems. Understanding these concepts will help developers build robust applications that can handle increased loads.
FAQ
Question | Answer |
---|---|
What is GraphQL? | GraphQL is a query language for APIs that allows clients to request exactly the data they need. |
How does MySQL work with GraphQL? | GraphQL acts as an API layer that communicates with a MySQL database, allowing for efficient data retrieval and manipulation. |
What are mutations in GraphQL? | Mutations are operations used to modify data, such as creating, updating, or deleting records in a database. |
How can I optimize the performance of my GraphQL API? | You can optimize performance by implementing caching, batching requests, and optimizing your SQL queries. |
What is Chat2DB? | Chat2DB (opens in a new tab) is an AI-driven database management tool that streamlines the process of managing databases, offering features like natural language SQL generation and visual data analysis. |
By integrating GraphQL with MySQL, developers can create powerful applications that efficiently manage and manipulate data. Tools like Chat2DB further facilitate this process, making it easier to handle complex queries and visualize data. Embrace the future of database management with Chat2DB, where advanced AI capabilities meet intuitive user experiences.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!