How to Efficiently Implement an Inverted Index for Faster Search Results
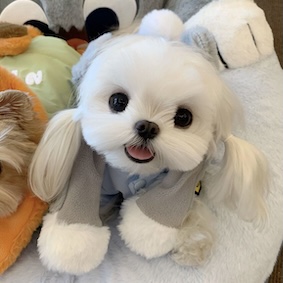
What is an Inverted Index?
An inverted index is a powerful data structure that maps content, such as words or phrases, to their locations within a database, document, or collection of documents. This structure significantly enhances search performance, enabling quick full-text searches. The key components of an inverted index are:
- Index: A data structure designed to improve retrieval speed.
- Term: A unique word or phrase stored in the index.
- Document: Any piece of content that contains these terms.
- Posting List: A list of document identifiers that include a specific term.
Inverted indexes outperform traditional indexes by enabling faster keyword searches, making them indispensable for large-scale datasets. Their evolution has been pivotal in commercial search engines and databases, enhancing both efficiency and speed.
Components and Structure of an Inverted Index
An inverted index comprises several essential components:
- Term Dictionary: A list of unique terms found in the documents, with each term linked to a corresponding posting list.
- Posting List: Contains identifiers for documents that contain the corresponding term, allowing rapid lookups.
- Term Frequency (TF): Measures how often a term appears in a document, assisting in evaluating the term's importance.
- Document Frequency (DF): Counts how many documents contain a term and helps compute the inverse document frequency (IDF) for ranking search results.
- Skip Pointers: Utilized within posting lists to enable the search algorithm to skip over certain entries, thereby improving search speed.
To tackle complexities like synonyms, stop-words, and stemming, various strategies are employed. Stemming, for example, reduces words to their base form, optimizing search accuracy.
Example of an Inverted Index Structure
Below is a simplified representation of an inverted index:
Term Dictionary:
-----------------------------------------------
| Term | Posting List |
|---------|------------------------------------|
| cat | [1, 2, 4] |
| dog | [2, 3, 4] |
| mouse | [1, 3] |
-----------------------------------------------
In this representation, the term "cat" appears in documents 1, 2, and 4, while "dog" is found in documents 2, 3, and 4, and "mouse" in documents 1 and 3.
Implementing an Inverted Index
To effectively implement an inverted index, the following steps should be followed:
-
Tokenizing Text Data: Split the text into individual terms using libraries like NLTK in Python.
import nltk from nltk.tokenize import word_tokenize sample_text = "The cat and the dog are friends." tokens = word_tokenize(sample_text.lower()) print(tokens) # Output: ['the', 'cat', 'and', 'the', 'dog', 'are', 'friends', '.']
-
Normalizing Terms: Convert terms to a consistent format (e.g., lowercase) and remove stop-words.
-
Constructing the Index: Build the index using a hash table or B-tree.
from collections import defaultdict inverted_index = defaultdict(list) documents = [ "The cat sat on the mat.", "The dog barked at the cat.", "The mouse ran away from the cat and dog." ] for doc_id, text in enumerate(documents): for term in word_tokenize(text.lower()): inverted_index[term].append(doc_id) print(dict(inverted_index))
-
Choosing Data Structures: Depending on requirements, choose appropriate data structures for posting lists. Arrays provide faster access, while linked lists are better for dynamic operations.
-
Parallel Processing: For large datasets, leverage distributed systems like Apache Hadoop or Apache Spark to enhance performance.
-
Merging Indexes: Use efficient algorithms to maintain data integrity when combining multiple indexes.
Example of Merging Two Posting Lists
def merge_posting_lists(list1, list2):
merged_list = sorted(set(list1) | set(list2))
return merged_list
list1 = [1, 2, 4]
list2 = [2, 3, 4]
print(merge_posting_lists(list1, list2)) # Output: [1, 2, 3, 4]
Optimizing Search with Inverted Indexes
To further improve search performance, consider the following optimization techniques:
- Caching: Cache frequently accessed index segments to reduce latency using tools like Redis or Memcached.
- Query Optimization: Rewrite queries for better relevance; for example, instead of searching for "dog", search for "dogs" using stemming.
- Hybrid Indexes: Combine inverted indexes with other data structures to support complex queries.
- Machine Learning: Integrate machine learning techniques to predict search patterns and prefetch relevant data.
Example of Query Optimization
def optimized_query_search(query, inverted_index):
# Simple stemming function
stemmed_query = query.rstrip('s') # naive stemming for pluralization
return inverted_index.get(stemmed_query, [])
query = "dogs"
search_results = optimized_query_search(query, inverted_index)
print(search_results) # Output: [2, 3, 4]
Challenges and Solutions in Inverted Index Implementation
Developers may encounter several challenges when implementing inverted indexes:
- Handling Large Volumes of Data: Utilize sharding to distribute data across multiple servers, improving manageability and performance.
- Managing Dynamic Updates: Employ strategies for handling updates and deletions efficiently, such as maintaining a secondary index.
- Language-Specific Nuances: Address variations in language through processing techniques that consider grammar and context.
- Security Concerns: Protect sensitive data using encryption and access control measures to ensure privacy.
Example of Sharding Implementation
def shard_data(data, num_shards):
return [data[i::num_shards] for i in range(num_shards)]
data = [1, 2, 3, 4, 5, 6, 7, 8]
shards = shard_data(data, 3)
print(shards) # Output: [[1, 4, 7], [2, 5, 8], [3, 6]]
Enhancing Inverted Index Implementation with Chat2DB
Chat2DB is an AI-driven database management tool that simplifies database management and enhances search capabilities. It integrates seamlessly with inverted indexes, providing developers with:
- Natural Language Processing: Generate SQL queries using natural language, making database interactions intuitive.
- AI-Driven Insights: Analyze data and generate visualizations automatically, facilitating deeper insights into search results.
- Efficient Data Retrieval: Chat2DB’s intelligent SQL editor optimizes queries by leveraging the capabilities of inverted indexes.
Example of Using Chat2DB for SQL Generation
With Chat2DB, you can generate SQL queries using natural language commands. For instance, if you want to find all documents containing "cat" and "dog", simply input:
"Show me all documents that contain both 'cat' and 'dog'."
Chat2DB will transform this into an SQL query automatically:
SELECT * FROM documents WHERE content LIKE '%cat%' AND content LIKE '%dog%';
Future Trends in Search Technologies and Inverted Indexes
Emerging trends in search technologies are shaping the future of inverted indexes. Key advancements include:
- AI and Machine Learning: Ongoing improvements in AI will enhance search precision and personalization, making inverted indexes even more efficient.
- Big Data and IoT: As data volumes increase, inverted indexes must adapt to manage larger and more complex datasets effectively.
- Voice Search: The rise of voice search necessitates supporting natural language queries, requiring more advanced language processing capabilities.
- Blockchain Technology: Innovations in blockchain may lead to more secure and transparent search solutions.
By staying informed about these trends and leveraging tools like Chat2DB, developers can enhance their database management capabilities and improve search efficiency.
For more information on implementing advanced search features and optimizing your database management, explore Chat2DB's robust functionalities and AI capabilities.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!