How to Use MongoDB Aggregate: The Ultimate MongoDB Aggregation Guide
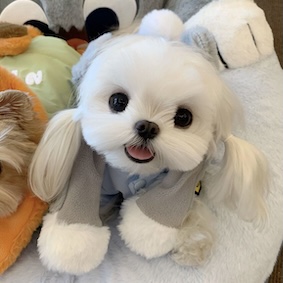
In the realm of data analysis, MongoDB Aggregate stands out as a robust framework, enabling developers and analysts to process and transform data efficiently. This article dives deep into the intricacies of the MongoDB aggregation framework, emphasizing its power, flexibility, and practical applications. We will explore the significance of aggregation pipelines, stages, and operators, while providing detailed code examples to illustrate their usage. Additionally, we will highlight how tools like Chat2DB (opens in a new tab) enhance the user experience of working with MongoDB aggregation, particularly through AI-driven capabilities.
Understanding the MongoDB Aggregation Framework
The MongoDB Aggregation Framework (opens in a new tab) serves as a powerful tool for processing and analyzing data stored in collections. By leveraging this framework, users can perform complex queries that transform and aggregate data across multiple documents. Key concepts such as pipeline, stages, and operators form the foundation of this framework, allowing for flexible data manipulation.
What is an Aggregation Pipeline?
An aggregation pipeline is a sequence of data processing stages that transform the input documents into the desired output. Each stage in the pipeline performs a specific operation on the data. For instance, consider a collection of sales
documents in a MongoDB database:
{
"_id": 1,
"item": "apple",
"quantity": 5,
"price": 1.2
}
An aggregation pipeline might look like this:
db.sales.aggregate([
{ $match: { item: "apple" } },
{ $group: { _id: null, totalQuantity: { $sum: "$quantity" } } }
])
This pipeline first filters for documents where the item is "apple" and then groups the results to calculate the total quantity sold.
Key Aggregation Stages in MongoDB
Understanding the various stages in the aggregation pipeline is crucial for effective data analysis. Here are some essential stages commonly used in MongoDB aggregation:
1. Match Stage
The match stage filters documents based on specified criteria, similar to the SQL WHERE
clause. For example:
db.sales.aggregate([
{ $match: { price: { $gt: 1.0 } } }
])
This command retrieves all sales where the price is greater than 1.0.
2. Project Stage
The project stage reshapes documents, allowing you to include or exclude specific fields. Here’s how you can use it:
db.sales.aggregate([
{ $project: { item: 1, totalPrice: { $multiply: ["$quantity", "$price"] } } }
])
This command selects the item
field and calculates the total price for each sale.
3. Group Stage
The group stage is pivotal for aggregating data. It resembles the SQL GROUP BY
clause and allows for operations such as summation, averaging, and counting. For instance:
db.sales.aggregate([
{ $group: { _id: "$item", totalSales: { $sum: "$quantity" } } }
])
This code groups the sales by item and calculates the total sales quantity for each item.
4. Sort Stage
The sort stage orders documents based on specified fields, akin to SQL’s ORDER BY
clause:
db.sales.aggregate([
{ $sort: { totalSales: -1 } }
])
This command sorts the sales in descending order based on total sales.
5. Limit and Skip Stages
These stages are used for pagination. The limit stage restricts the number of documents returned, while the skip stage skips a specified number of documents:
db.sales.aggregate([
{ $sort: { totalSales: -1 } },
{ $skip: 10 },
{ $limit: 5 }
])
This command skips the first 10 results and limits the output to the next 5.
6. Unwind Stage
The unwind stage deconstructs array fields into separate documents, which is useful for working with arrays:
db.orders.aggregate([
{ $unwind: "$items" },
{ $group: { _id: "$items.product", totalQuantity: { $sum: "$items.quantity" } } }
])
This example unwinds the items
array in each order and groups the results by product.
Advanced Aggregation Techniques
Once you grasp the basic stages, you can explore advanced techniques that enhance the capabilities of the aggregation pipeline.
Lookup Stage
The lookup stage allows you to perform left outer joins between collections, similar to SQL joins:
db.orders.aggregate([
{
$lookup: {
from: "products",
localField: "productId",
foreignField: "_id",
as: "productDetails"
}
}
])
This command retrieves product details for each order based on productId
.
Facet Stage
The facet stage is incredibly powerful for executing multiple aggregation pipelines within a single query:
db.sales.aggregate([
{
$facet: {
totalSales: [{ $group: { _id: null, total: { $sum: "$quantity" } } }],
salesByItem: [{ $group: { _id: "$item", total: { $sum: "$quantity" } } }]
}
}
])
This command generates both total sales and a breakdown by item in one query.
Bucket and BucketAuto Stages
These stages categorize data into specified ranges or automatically calculated buckets. For instance:
db.sales.aggregate([
{
$bucket: {
groupBy: "$price",
boundaries: [0, 1, 2, 3],
default: "Other",
output: { count: { $sum: 1 } }
}
}
])
This command categorizes sales by price ranges.
GraphLookup Stage
The graphLookup stage is useful for recursive searches within a collection, ideal for hierarchical data:
db.employees.aggregate([
{
$graphLookup: {
from: "employees",
startWith: "$managerId",
connectFromField: "managerId",
connectToField: "_id",
as: "subordinates"
}
}
])
This command retrieves all subordinates for each employee.
AddFields and Set Stages
The addFields and set stages allow you to add new fields or modify existing ones:
db.sales.aggregate([
{ $addFields: { totalPrice: { $multiply: ["$quantity", "$price"] } } }
])
This command adds a new field totalPrice
to each document.
Performance Optimization in Aggregation
Optimizing MongoDB aggregation operations is crucial for improving performance. Below are some strategies to enhance your aggregation queries:
Strategy | Description |
---|---|
Indexing | Index fields used in the match and sort stages to speed up query performance. |
Reduce Document Size | Filtering documents early in the pipeline can significantly enhance speed. |
Use Projection | Limit the fields processed in subsequent stages with the project stage. |
Analyze with Explain | Use MongoDB’s explain() method to analyze and optimize your aggregation queries. |
Efficient Use of Stages | Carefully select stages and operators to minimize unnecessary data processing. |
Real-World Use Cases of MongoDB Aggregation
MongoDB aggregation proves invaluable across various industries and applications. Here are some practical scenarios:
E-commerce
In e-commerce, aggregation can generate sales reports, perform customer segmentation, and analyze inventory levels. For example, a retailer might use aggregation to track sales trends over time, segment customers based on buying behavior, and manage inventory effectively.
Social Media Analytics
Aggregation helps analyze user behavior and engagement metrics on social media platforms. By aggregating data on likes, shares, and comments, businesses can gauge content performance and user interaction.
Financial Services
In financial services, MongoDB aggregation is used for fraud detection and transaction analysis. By aggregating transaction data, institutions can identify unusual patterns that may indicate fraudulent activity.
IoT Data Processing
For IoT applications, aggregation facilitates processing and analyzing large volumes of sensor data. Aggregation pipelines can help summarize data, detect anomalies, and visualize trends over time.
Healthcare
In healthcare, aggregation can be employed for patient data aggregation and trend analysis. Hospitals may aggregate patient records to identify health trends and improve patient care.
Content Management
Aggregation aids in understanding user preferences and content performance in content management systems. By analyzing user interactions with content, organizations can optimize their strategies.
Integrating Aggregation with Chat2DB
Chat2DB (opens in a new tab) enhances the MongoDB aggregation experience by providing a user-friendly interface that simplifies crafting and executing aggregation queries. The tool’s AI capabilities allow users to generate SQL queries from natural language input, making it accessible for those less familiar with MongoDB syntax.
Key Features of Chat2DB
-
Visual Query Builder: Chat2DB offers a visual query builder that allows users to construct aggregation pipelines graphically, reducing the complexity of writing raw queries.
-
Result Visualization: The tool visualizes aggregation results, aiding in data interpretation and decision-making.
-
Multiple Connections: Users can manage multiple MongoDB connections and switch between different environments effortlessly.
-
Collaborative Data Analysis: Chat2DB enables team members to share and review aggregation pipelines, fostering collaboration.
-
AI-Powered SQL Generation: The AI functionality allows users to generate SQL queries simply by typing in natural language, significantly reducing the time spent on query construction.
By utilizing Chat2DB, users can harness the full potential of MongoDB aggregation while enjoying an intuitive and efficient interface for data analysis. Unlike other tools, Chat2DB's AI capabilities streamline the user experience, making complex queries easier to manage and execute effectively.
Future Trends in MongoDB Aggregation
As data analysis needs evolve, the MongoDB aggregation framework will continue to adapt. Potential future developments include:
-
Real-Time Data Processing: The demand for real-time data processing is growing, and MongoDB is likely to enhance its aggregation capabilities to meet this requirement.
-
Machine Learning Integration: Incorporating machine learning algorithms within aggregation pipelines will provide advanced data insights, allowing for predictive analytics.
-
Performance Improvements: Continuous improvements in aggregation performance and scalability will enhance user experience.
-
Community Contributions: The MongoDB community plays a vital role in enhancing aggregation capabilities through plugins and extensions.
-
Cloud Service Integration: Greater integration with cloud services will facilitate scalable and efficient data processing.
By staying informed about these trends and leveraging tools like Chat2DB, data professionals can ensure they are equipped to handle emerging data analysis requirements.
FAQs
-
What is MongoDB aggregation? MongoDB aggregation is a framework for processing and transforming data stored in collections, allowing users to perform complex queries and analyses.
-
What are aggregation pipelines? Aggregation pipelines are sequences of stages that process input documents and transform them into a desired output format.
-
How does the match stage work? The match stage filters documents based on specified criteria, similar to the SQL WHERE clause.
-
What is the purpose of the lookup stage? The lookup stage performs left outer joins between collections, enabling users to combine data from different sources.
-
How can Chat2DB improve my MongoDB experience? Chat2DB provides an intuitive interface, AI-driven SQL generation, and visual query building, making it easier to work with MongoDB aggregation and analyze data efficiently.
Incorporating these insights and leveraging the power of Chat2DB (opens in a new tab) can elevate your data analysis capabilities with MongoDB aggregation, making the process more efficient and effective.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!