How to Implement MongoDB Vector Search for Enhanced Performance: A Comprehensive Guide
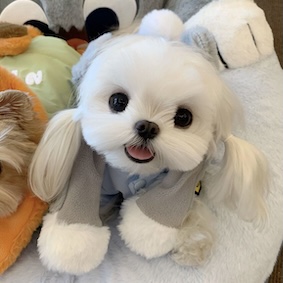
MongoDB has evolved into a powerful NoSQL database that supports advanced data processing needs, particularly with the integration of vector search capabilities. By utilizing vector search, applications can retrieve data based on similarity rather than traditional keyword matching, making it an invaluable tool in fields such as AI and machine learning. This article delves into the evolution of MongoDB and the significance of vector search, offering a comprehensive guide on implementing it for enhanced performance. We will explore essential concepts, practical implementation steps, optimization techniques, and real-world applications, while also highlighting the advantages of using Chat2DB (opens in a new tab) for seamless database management.
The Evolution of MongoDB and Its Vector Search Capabilities
Since its inception, MongoDB (opens in a new tab) has been at the forefront of NoSQL database technology, providing flexibility and scalability for developers. Vector search has emerged as a critical feature in modern applications, allowing for efficient data retrieval based on the similarity of vectors. This contrasts sharply with traditional keyword-based search methods, which often fail to deliver relevant results in complex scenarios.
The importance of vector search has skyrocketed in recent years, especially in AI-driven applications where understanding relationships between data points is essential. For instance, in recommendation systems, vector search can vastly improve the accuracy of suggestions by analyzing user behavior and preferences in a multidimensional space. The integration of vector search within MongoDB not only enhances performance but also opens up new avenues for data exploration and analysis.
Key Terminology Defined
- Vector Search: A method of retrieving data based on the similarity of vector representations rather than exact matches.
- Similarity Search: Finding items that are similar to a given query based on defined metrics.
- NoSQL: A class of database management systems that do not use traditional relational database structures.
Understanding Vector Space Models
Vector space models are the backbone of vector search technologies. In these models, data is represented as vectors in a multidimensional space, allowing for complex similarity computations. The mathematical foundation of vector space models includes:
- Cosine Similarity: Measures the cosine of the angle between two vectors, providing a metric for similarity.
- Euclidean Distance: Represents the straight-line distance between two points in space.
These calculations enable systems to determine how similar two data points are, which is particularly useful in applications such as e-commerce, where product recommendations depend on user preferences.
Advantages Over Traditional Systems
Vector space models offer several advantages over traditional keyword-based retrieval systems:
- Higher Accuracy: By relying on the mathematical relationships between data points, vector search provides more relevant results.
- Better Handling of Synonyms: Vector search can identify similar items even when different terms are used.
- Enhanced User Experience: Applications powered by vector search often lead to improved user satisfaction and engagement.
Implementing MongoDB Vector Search: A Step-by-Step Guide
To implement vector search in MongoDB, follow these steps:
Prerequisites
Before diving into implementation, ensure you have the necessary software and hardware:
- A running instance of MongoDB.
- Suitable hardware to handle the expected data load.
Step 1: Setting Up Your MongoDB Database
To begin, you need to set up your MongoDB database to support vector search. This involves defining your data schema appropriately.
// Example: Defining a collection with vector data
db.createCollection("products");
db.products.insertMany([
{
name: "Product A",
vector: [0.1, 0.2, 0.3]
},
{
name: "Product B",
vector: [0.4, 0.5, 0.6]
}
]);
Step 2: Integrating Vector Search Capabilities
You can utilize MongoDB's native features or third-party libraries for vector search. Below is an example of performing a similarity search using cosine similarity.
// Function to calculate cosine similarity
function cosineSimilarity(vecA, vecB) {
let dotProduct = 0;
let normA = 0;
let normB = 0;
for (let i = 0; i < vecA.length; i++) {
dotProduct += vecA[i] * vecB[i];
normA += vecA[i] * vecA[i];
normB += vecB[i] * vecB[i];
}
return dotProduct / (Math.sqrt(normA) * Math.sqrt(normB));
}
// Querying products based on a vector
const queryVector = [0.1, 0.2, 0.3];
db.products.find().forEach(doc => {
const similarity = cosineSimilarity(queryVector, doc.vector);
print(`Product: ${doc.name}, Similarity: ${similarity}`);
});
Step 3: Defining Vector Representations
To perform vector-based queries, you need to define how your data will be represented as vectors. This often involves leveraging machine learning models to transform raw data into vector formats.
Step 4: Executing Vector-Based Queries
Once your data is represented as vectors, executing vector-based queries becomes straightforward. MongoDB allows for flexible querying, enabling you to retrieve data based on similarity effectively.
Best Practices
- Indexing: Proper indexing strategies can significantly enhance the performance of vector search queries. Consider using techniques such as creating indexes on vector fields.
- Caching: Implement caching mechanisms to reduce response times for frequently accessed data.
- Monitoring: Utilize monitoring tools to track performance metrics and identify bottlenecks.
Optimizing MongoDB for Vector Search Performance
Optimizing your MongoDB setup for vector search involves several techniques:
Data Modeling and Schema Design
A well-designed schema can facilitate efficient vector operations. Organize your data in a way that minimizes redundant computations and optimizes access patterns.
Indexing Strategies
Implement indexing strategies tailored for vector search. For example, consider using geospatial indexes if your vectors represent spatial data.
Query Optimization
Optimize your queries by reducing the amount of data processed. Use aggregation frameworks to filter and sort data efficiently.
Sharding and Replication
Distributing vector search workloads through sharding and replication can enhance scalability. Ensure that your infrastructure is capable of handling increased loads effectively.
Caching Mechanisms
Implement caching to improve query response times. Caching frequently accessed vectors can significantly reduce the time it takes to retrieve results.
Optimization Technique | Description |
---|---|
Data Modeling | Proper schema design to facilitate vector operations. |
Indexing Strategies | Use of specialized indexes to enhance search performance. |
Query Optimization | Techniques to reduce data processing and improve efficiency. |
Sharding | Distributing workloads for scalability. |
Caching | Storing frequently accessed vectors to improve response times. |
Real-World Use Cases and Applications
MongoDB vector search is being applied across various industries to enhance performance and user experience. Here are some notable examples:
Healthcare
In healthcare, vector search is used to analyze patient data for better diagnosis and treatment recommendations. It allows for personalized healthcare solutions by finding similar cases and outcomes.
Finance
Financial institutions leverage vector search for fraud detection by analyzing transaction patterns and identifying anomalies that deviate from typical behavior.
Entertainment
In the entertainment industry, vector search powers recommendation systems that suggest content based on user preferences, enhancing engagement and satisfaction.
E-commerce
E-commerce platforms use vector search to improve the accuracy of product recommendations, leading to better conversion rates and customer retention.
Future Trends and Innovations in MongoDB Vector Search
The future of MongoDB vector search is promising, with ongoing innovations and trends shaping its development. Some key areas to watch include:
- Deep Learning Integrations: Advances in deep learning and neural network embeddings are enhancing the capabilities of vector search.
- Real-Time Data Processing: The need for real-time analytics is driving innovations in vector search technologies.
- AI-Driven Analytics: The integration of AI-driven analytics with vector search will further improve decision-making processes.
As these technologies evolve, developers and organizations must stay ahead by adopting future-ready strategies that leverage the full potential of vector search.
FAQ
-
What is vector search in MongoDB? Vector search in MongoDB is a method of retrieving data based on the similarity of vector representations, improving accuracy and relevance in search results.
-
How can I implement vector search in my application? You can implement vector search by defining vector representations of your data, integrating querying capabilities, and optimizing your MongoDB setup.
-
What are the advantages of using Chat2DB for MongoDB management? Chat2DB (opens in a new tab) enhances database management through AI features, including natural language SQL generation, intelligent SQL editing, and automated data analysis. These capabilities streamline database operations, reduce development time, and improve overall productivity compared to traditional tools.
-
Can vector search be used in real-time applications? Yes, vector search is increasingly being used in real-time applications, particularly in fields like finance and e-commerce where timely insights are crucial.
-
What tools should I use for vector search optimization? Utilize monitoring tools, caching mechanisms, and indexing strategies to optimize your MongoDB setup for vector search performance.
In conclusion, by implementing these strategies and utilizing tools like Chat2DB (opens in a new tab), you can significantly enhance the performance and efficiency of your MongoDB vector search applications, ultimately leading to better user experiences and data-driven insights. Embrace the power of vector search and let Chat2DB transform your database management journey today.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!