Mastering MySQL: A Comprehensive Step-by-Step Tutorial for Beginners
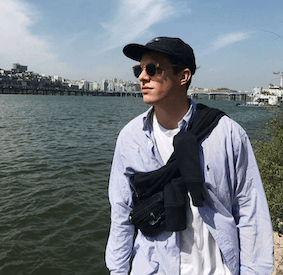
MySQL stands out as one of the most popular relational database management systems (RDBMS) globally, especially for web applications, thanks to its reliability and user-friendly nature. This detailed tutorial will guide you through the various aspects of MySQL, from foundational concepts to advanced features, ensuring you're well-prepared to manage MySQL databases effectively.
Understanding MySQL Basics: What You Need to Know
Before diving into MySQL, it’s crucial to grasp the concept of a relational database. A relational database organizes data into tables, which consist of rows and columns. MySQL utilizes Structured Query Language (SQL) to facilitate data management and manipulation.
Core Architecture of MySQL
MySQL operates on a client-server model, where the MySQL server manages databases, while client applications send requests to perform operations like querying or updating data. This architecture enhances scalability and security.
The Role of SQL in MySQL
SQL is the standardized language for interacting with MySQL databases, featuring commands such as SELECT, INSERT, UPDATE, and DELETE for various operations. Familiarity with SQL syntax and structure is essential for effective database management.
Understanding Primary Keys and Indexes
Primary keys uniquely identify each record in a table, maintaining data integrity and optimizing query performance. Indexes further enhance data retrieval speed by providing an organized access method.
For more information on relational databases, check out the Wikipedia page (opens in a new tab).
Installing and Configuring MySQL: A Step-by-Step Guide
To begin using MySQL, you must first install it on your system. The installation process varies by operating system.
Installation on Different Operating Systems
-
Windows:
- Download the MySQL Installer from the official MySQL website.
- Run the installer and follow the setup wizard to install MySQL Server and MySQL Workbench.
-
macOS:
- Use Homebrew for installation:
brew install mysql
- Start MySQL:
brew services start mysql
- Use Homebrew for installation:
-
Linux:
- Use the package manager to install MySQL. For example, on Ubuntu:
sudo apt update sudo apt install mysql-server
- Use the package manager to install MySQL. For example, on Ubuntu:
Configuring MySQL for Optimal Performance
Post-installation, configure MySQL for optimal performance with these essential steps:
- Set a strong root password for enhanced security.
- Configure MySQL to start automatically on system boot.
- Use MySQL Workbench for a graphical interface to manage databases.
- Regularly update MySQL to the latest version for security and performance enhancements.
For detailed installation guides, refer to the MySQL documentation (opens in a new tab).
Creating and Managing Databases: Your First Steps
After installing and configuring MySQL, you can start creating and managing databases.
Creating a New Database
To create a new database, run the following SQL command:
CREATE DATABASE my_database;
Defining Tables
Once a database is created, you can define tables within it. Here’s how to create a table:
USE my_database;
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(50) NOT NULL,
email VARCHAR(100) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
Inserting, Updating, and Deleting Records
Manipulate records in your tables using SQL commands:
-
Insert a record:
INSERT INTO users (username, email) VALUES ('john_doe', 'john@example.com');
-
Update a record:
UPDATE users SET email = 'john.doe@example.com' WHERE username = 'john_doe';
-
Delete a record:
DELETE FROM users WHERE username = 'john_doe';
Transactions and Data Integrity
To maintain data integrity, especially during multiple operations, use transactions:
START TRANSACTION;
INSERT INTO users (username, email) VALUES ('jane_doe', 'jane@example.com');
UPDATE users SET email = 'jane.doe@example.com' WHERE username = 'jane_doe';
COMMIT;
Managing Metadata with INFORMATION_SCHEMA
MySQL's INFORMATION_SCHEMA
database contains metadata about all databases on the server. Query it for information:
SELECT TABLE_NAME FROM INFORMATION_SCHEMA.TABLES WHERE TABLE_SCHEMA = 'my_database';
Querying Data with MySQL: Mastering Data Retrieval
Querying data is a fundamental functionality within MySQL.
Using the SELECT Statement
To retrieve data from a table, employ the SELECT
statement:
SELECT * FROM users;
Filtering Data with WHERE Clauses
Utilize the WHERE
clause to filter records based on conditions:
SELECT * FROM users WHERE email LIKE '%example.com';
Sorting Query Results
Sort query results with the ORDER BY
clause:
SELECT * FROM users ORDER BY created_at DESC;
Aggregate Functions
MySQL supports various aggregate functions such as COUNT
, SUM
, and AVG
:
SELECT COUNT(*) AS total_users FROM users;
Joining Tables
Retrieve data from multiple tables using joins. Here’s an example of an inner join:
SELECT users.username, orders.amount
FROM users
JOIN orders ON users.id = orders.user_id;
Subqueries and Derived Tables
Subqueries enable you to nest queries within each other:
SELECT username FROM users WHERE id IN (SELECT user_id FROM orders WHERE amount > 100);
GROUP BY and HAVING Clauses
Group your data and apply conditions on aggregated results:
SELECT email, COUNT(*) AS user_count
FROM users
GROUP BY email
HAVING user_count > 1;
Optimizing Queries
Use the EXPLAIN
statement to understand how MySQL executes your queries:
EXPLAIN SELECT * FROM users WHERE email = 'john@example.com';
Advanced MySQL Features: Unlocking Enhanced Functionality
MySQL offers several advanced features that can significantly enhance database functionality.
Stored Procedures
Stored procedures encapsulate complex SQL logic for reuse:
DELIMITER //
CREATE PROCEDURE GetUser(IN user_id INT)
BEGIN
SELECT * FROM users WHERE id = user_id;
END //
DELIMITER ;
Triggers
Triggers automate actions in response to specific database events:
CREATE TRIGGER before_user_insert
BEFORE INSERT ON users
FOR EACH ROW
BEGIN
SET NEW.created_at = NOW();
END;
Views
Views present data in different formats without altering the underlying tables:
CREATE VIEW active_users AS
SELECT * FROM users WHERE status = 'active';
Full-Text Search
Leverage MySQL's full-text search capabilities for efficient data retrieval:
SELECT * FROM users WHERE MATCH(username) AGAINST('john');
Partitioning Strategies
Partitioning splits large tables into smaller, manageable pieces, enhancing query performance:
CREATE TABLE orders (
id INT,
order_date DATE,
customer_id INT
) PARTITION BY RANGE (YEAR(order_date)) (
PARTITION p2021 VALUES LESS THAN (2022),
PARTITION p2022 VALUES LESS THAN (2023)
);
Replication for Data Redundancy
MySQL supports replication, creating copies of databases for redundancy and failover solutions, crucial for high availability.
Event Scheduler
Automate routine tasks with the Event Scheduler:
CREATE EVENT daily_backup
ON SCHEDULE EVERY 1 DAY
DO
BEGIN
-- Backup logic here
END;
MySQL Performance Tuning: Achieving Optimal Efficiency
Performance tuning is vital for efficient database operations.
Identifying Performance Bottlenecks
Analyze slow queries to identify and optimize performance bottlenecks.
Using Indexes
Indexes dramatically speed up data retrieval:
CREATE INDEX idx_email ON users(email);
Query Cache
Reduce server load and improve response times by utilizing MySQL’s query cache:
SET GLOBAL query_cache_size = 1048576; -- 1 MB
Monitoring Tools
Employ monitoring tools like MySQL Workbench or third-party solutions to track performance metrics.
Regular Maintenance Tasks
Engage in regular maintenance tasks such as vacuuming and reindexing to keep your database performant.
Integrating MySQL with Applications: Building Connections
Connecting MySQL with various programming languages is essential for application development.
Connecting to MySQL
Here’s how to connect to a MySQL database in different programming languages:
-
PHP:
$conn = new mysqli($servername, $username, $password, $dbname);
-
Python:
import mysql.connector conn = mysql.connector.connect(user='username', password='password', host='localhost', database='my_database')
-
Java:
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/my_database", "username", "password");
Prepared Statements
Prepared statements enable executing parameterized queries securely:
PREPARE stmt FROM 'SELECT * FROM users WHERE username = ?';
SET @username = 'john_doe';
EXECUTE stmt USING @username;
Object-Relational Mapping (ORM)
ORM tools simplify interactions with MySQL databases in an object-oriented manner. Popular choices include Hibernate for Java and SQLAlchemy for Python.
Managing Database Connections
Efficiently manage database connections to optimize resource usage. Connection pooling is a useful technique.
Handling Exceptions and Errors
Handle exceptions and errors gracefully during database operations to ensure application stability.
JSON Support in MySQL
MySQL supports semi-structured data using JSON:
CREATE TABLE json_data (
id INT AUTO_INCREMENT PRIMARY KEY,
data JSON
);
FAQs: Your MySQL Questions Answered
1. What is MySQL?
MySQL is an open-source relational database management system that uses SQL for data manipulation and management.
2. How do I install MySQL?
You can install MySQL on various operating systems like Windows, macOS, and Linux by following the respective installation instructions.
3. What are primary keys and indexes in MySQL?
Primary keys uniquely identify records in a table, while indexes improve the speed of data retrieval operations.
4. How can I connect to a MySQL database using Python?
Use the mysql.connector
module to connect to a MySQL database in Python.
5. What is Chat2DB?
Chat2DB (opens in a new tab) is an AI-powered database visualization management tool that enhances database management efficiency through natural language processing and intelligent SQL editing.
In conclusion, mastering MySQL involves understanding its fundamental concepts, installation, configuration, and advanced features. Whether you're a beginner or looking to refine your skills, this tutorial provides a comprehensive overview to help you become proficient in MySQL. Don't forget to explore tools like Chat2DB (opens in a new tab) for an enhanced database management experience. By leveraging these insights and practical examples, you can effectively navigate MySQL and optimize your database management tasks.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!