Understanding Normal Forms in DBMS: A Comprehensive Guide to Efficient Database Design
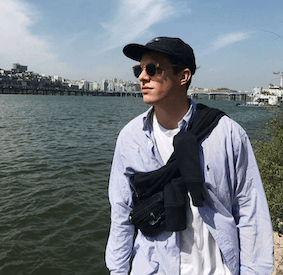
In the realm of database management systems (DBMS), normal forms are essential for designing efficient and effective databases. These forms serve as guidelines for organizing data, aiming to minimize redundancy and enhance data integrity. The journey of normal forms begins with the first normal form (1NF) and progresses through to the fifth normal form (5NF). This article explores each normal form, detailing their significance in database design, the anomalies they seek to prevent, and their overall impact on database performance and storage efficiency.
Why Normalization Matters in Database Management Systems
Normalization is the systematic process of organizing a database to reduce redundancy and improve data integrity. This process is critical in preventing various anomalies, such as insertion, update, and deletion anomalies, which can compromise the quality of data stored in databases. Normal forms are the foundation of normalization, providing a structured approach to database design. For further insights into normalization, refer to the Wikipedia page on Database Normalization (opens in a new tab).
First Normal Form (1NF): Ensuring Atomicity of Data
The first normal form (1NF) mandates that all table columns contain atomic values, effectively eliminating repeating groups. Atomicity is crucial because it guarantees that each field holds indivisible values. For instance, consider a table storing customer data that includes multiple phone numbers in a single column. This structure violates 1NF. To achieve compliance with 1NF, we can split the phone numbers into separate rows.
Here’s a code example illustrating this concept:
-- Original table violating 1NF
CREATE TABLE Customers (
CustomerID INT,
CustomerName VARCHAR(100),
PhoneNumbers VARCHAR(100) -- Violating 1NF by having multiple phone numbers
);
-- Transformed table adhering to 1NF
CREATE TABLE Customers (
CustomerID INT,
CustomerName VARCHAR(100)
);
CREATE TABLE CustomerPhones (
CustomerID INT,
PhoneNumber VARCHAR(15)
);
In this example, the CustomerPhones
table allows us to store multiple phone numbers while remaining compliant with 1NF.
Second Normal Form (2NF): Tackling Partial Dependency
Building on the principles of 1NF, the second normal form (2NF) addresses partial dependency. A table is considered to be in 2NF if all non-key attributes are fully functionally dependent on the primary key. This means that each non-key attribute must rely on the entire primary key, not just a portion of it.
Consider a scenario involving an orders table:
-- Original table violating 2NF
CREATE TABLE Orders (
OrderID INT,
ProductID INT,
ProductName VARCHAR(100),
Quantity INT,
CustomerID INT
);
Here, ProductName
is partially dependent on ProductID
. To convert the table to 2NF, we separate the product information into a distinct table:
-- Transformed tables adhering to 2NF
CREATE TABLE Orders (
OrderID INT,
ProductID INT,
Quantity INT,
CustomerID INT
);
CREATE TABLE Products (
ProductID INT,
ProductName VARCHAR(100)
);
With this structure, both tables comply with 2NF, eliminating partial dependencies and enhancing data integrity.
Third Normal Form (3NF): Removing Transitive Dependency
The third normal form (3NF) aims to eliminate transitive dependency, ensuring that non-key attributes do not depend on other non-key attributes. To achieve 3NF, tables must be restructured to remove these dependencies.
For example, consider the following table:
-- Original table violating 3NF
CREATE TABLE Employees (
EmployeeID INT,
EmployeeName VARCHAR(100),
DepartmentID INT,
DepartmentName VARCHAR(100)
);
In this instance, DepartmentName
depends on DepartmentID
, which is not a primary key attribute. To convert this table into 3NF, we create a separate Departments
table:
-- Transformed tables adhering to 3NF
CREATE TABLE Employees (
EmployeeID INT,
EmployeeName VARCHAR(100),
DepartmentID INT
);
CREATE TABLE Departments (
DepartmentID INT,
DepartmentName VARCHAR(100)
);
This restructuring effectively removes transitive dependencies and enhances data integrity.
Boyce-Codd Normal Form (BCNF): A Stricter Standard for Data Integrity
The Boyce-Codd normal form (BCNF) represents a stricter version of 3NF, addressing anomalies that arise from overlapping candidate keys. A table is in BCNF if, for every functional dependency, the left side is a superkey.
To illustrate this, consider a table structured as follows:
-- Original table violating BCNF
CREATE TABLE CourseEnrollments (
StudentID INT,
CourseID INT,
InstructorID INT,
PRIMARY KEY (StudentID, CourseID)
);
In this table, if an instructor teaches multiple courses, anomalies may occur. To achieve BCNF, we can separate the instructor information:
-- Transformed tables adhering to BCNF
CREATE TABLE CourseEnrollments (
StudentID INT,
CourseID INT,
InstructorID INT
);
CREATE TABLE Instructors (
InstructorID INT,
CourseID INT,
InstructorName VARCHAR(100)
);
This structure effectively eliminates overlapping candidate keys and enhances data consistency.
Advanced Normal Forms: Fourth (4NF) and Fifth (5NF) Normal Forms
The fourth normal form (4NF) addresses multi-valued dependencies, while the fifth normal form (5NF) focuses on join dependencies. Both forms are applicable in complex database scenarios where managing data integrity and redundancy is crucial.
For 4NF, consider a table storing information about students and their hobbies:
-- Original table violating 4NF
CREATE TABLE StudentHobbies (
StudentID INT,
Hobby VARCHAR(100),
Sport VARCHAR(100)
);
To comply with 4NF, we separate hobbies and sports into distinct tables:
-- Transformed tables adhering to 4NF
CREATE TABLE StudentHobbies (
StudentID INT,
Hobby VARCHAR(100)
);
CREATE TABLE StudentSports (
StudentID INT,
Sport VARCHAR(100)
);
For 5NF, consider a scenario where students enroll in courses that may require multiple instructors. We need a structure that supports this relationship without introducing redundancy:
-- Original table violating 5NF
CREATE TABLE CourseInstructorAssignments (
CourseID INT,
InstructorID INT,
StudentID INT
);
To achieve 5NF, we create dedicated tables for courses, instructors, and their assignments:
-- Transformed tables adhering to 5NF
CREATE TABLE CourseInstructor (
CourseID INT,
InstructorID INT
);
CREATE TABLE CourseEnrollments (
CourseID INT,
StudentID INT
);
Enhancing Normalization with Tools like Chat2DB
Utilizing advanced tools can significantly streamline the normalization process. One such tool is Chat2DB (opens in a new tab), an AI-powered database visualization management tool that supports over 24 databases. By combining natural language processing with database management functionalities, Chat2DB allows developers and database administrators to leverage features such as natural language SQL generation and intelligent SQL editing to enhance their normalization efforts.
For example, Chat2DB enables users to visualize their database structure, identify normalization issues, and receive automatic suggestions for improvements. Integrating Chat2DB into your workflow can enhance database management efficiency and ensure compliance with various normal forms.
Best Practices for Maintaining a Normalized Database
Maintaining a normalized database necessitates regular audits and updates to ensure compliance with desired normal forms. Here are some best practices for developers:
- Conduct Regular Audits: Periodically review database structures to identify normalization issues.
- Utilize Automated Tools: Employ tools like Chat2DB to automate the detection of normalization problems.
- Maintain Comprehensive Documentation: Keep detailed documentation of database schemas to track changes and rationalize design decisions.
- Provide Training: Ensure team members are well-versed in normalization principles and the importance of each normal form.
- Monitor Performance: Regularly assess database performance to ensure that normalization enhances efficiency without introducing latency.
By adhering to these practices, you can maintain a well-structured and efficient database that aligns with normalization principles.
Frequently Asked Questions (FAQ)
-
What are normal forms in DBMS? Normal forms are guidelines for organizing data within a database to minimize redundancy and enhance integrity.
-
What is the purpose of the first normal form (1NF)? 1NF requires all table columns to contain atomic values and eliminates repeating groups.
-
How does the second normal form (2NF) improve database design? 2NF ensures that all non-key attributes are fully functionally dependent on the primary key, thereby eliminating partial dependencies.
-
What is the significance of the Boyce-Codd normal form (BCNF)? BCNF addresses anomalies arising from overlapping candidate keys, ensuring each functional dependency has a superkey on the left side.
-
How can Chat2DB assist in the normalization process? Chat2DB offers AI-driven tools for visualizing database structures and identifying normalization issues, streamlining overall management.
By implementing these strategies and utilizing tools like Chat2DB, you can optimize your database design for enhanced performance and integrity while adhering to normal forms in DBMS.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!