How to Optimize SQL Query Performance: Essential Techniques and Tips with DataGrip and Chat2DB
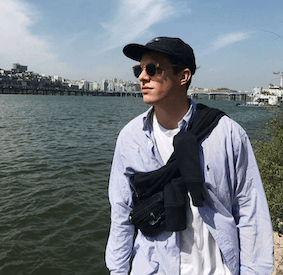
Understanding SQL Query Performance Optimization with DataGrip
In today's data-driven environment, optimizing SQL query performance is increasingly essential for developers, database administrators, and data analysts. Effective optimization directly influences application efficiency and resource usage. Key concepts like query execution plans, indexing, and cost-based optimization form the foundation of this process. Poorly optimized SQL queries can lead to sluggish application performance, increased latency, and higher operational costs.
DataGrip, as a powerful Integrated Development Environment (IDE), facilitates database management and enables users to optimize their SQL queries with ease. It provides various tools to analyze and enhance query performance effectively. This guide will explore essential techniques for performance tuning SQL queries specifically within DataGrip.
Analyzing SQL Queries with Execution Plans in DataGrip
One of the most vital steps in optimizing SQL queries is analyzing the execution plan. DataGrip allows users to generate and interpret execution plans easily. These plans serve as a roadmap, illustrating how a query is executed by the database engine.
To generate an execution plan in DataGrip, execute your query and click on the "Explain Plan" button. This action will present a visual representation of the execution strategy, highlighting expensive operations such as full table scans.
Tips for Analyzing Execution Plans
Tip | Description |
---|---|
Identify Expensive Operations | Look for operations that consume significant resources. Full table scans are common culprits. |
Evaluate Join Efficiency | Spot inefficient joins and consider rewriting them for optimal performance. |
Avoid Unnecessary Data Retrieval | Ensure only necessary columns are selected to improve performance. |
-- Example: Inefficient query with unnecessary columns
SELECT * FROM employees WHERE department_id = 3;
-- Optimized version
SELECT first_name, last_name FROM employees WHERE department_id = 3;
By leveraging DataGrip's visualization tools, you can gain valuable insights into your query's execution and make informed adjustments.
Leveraging Indexing Strategies for Optimal Performance
Indexing plays a crucial role in enhancing SQL query performance. An index is a data structure that improves the speed of data retrieval operations. Understanding how to implement effective indexing strategies can lead to significant performance gains.
Types of Indexes
Index Type | Description |
---|---|
Single-Column Index | An index on a single column useful for filtering queries. |
Composite Index | An index on multiple columns effective for filtering on several attributes. |
Unique Index | Ensures that all values in a column are distinct, enforcing data integrity. |
DataGrip provides tools to analyze index usage effectively. You can quickly identify missing or redundant indexes by reviewing the execution plans of your queries.
Balancing Read and Write Performance
While indexing improves read performance, it can negatively impact write operations due to the overhead of maintaining the indexes. Therefore, it is essential to find a balance between the two.
-- Creating an index on the department_id column
CREATE INDEX idx_department ON employees(department_id);
This index will enhance the performance of queries filtering by department_id
. However, be cautious about adding too many indexes, as it can slow down insert and update operations.
Introducing Chat2DB: A Modern Alternative to DataGrip
Advantages of Using Chat2DB
Chat2DB represents a new generation of database management tools that bring AI-powered capabilities to SQL query optimization. As an alternative to DataGrip, Chat2DB offers several advantages:
- AI-Powered Optimization Suggestions: Chat2DB analyzes query patterns and provides intelligent suggestions for improving performance without requiring deep technical knowledge from the user.
- Advanced Monitoring Features: With Chat2DB, you get real-time monitoring and alerts based on machine learning algorithms that can predict and prevent performance issues before they become critical.
- Simplified User Interface: Designed for ease of use, Chat2DB simplifies complex database operations, making it accessible even to less experienced users.
- Cross-Platform Compatibility: Unlike DataGrip, which primarily supports JetBrains IDEs, Chat2DB is compatible across different platforms and integrates seamlessly with various database systems.
- Collaboration and Support: Chat2DB fosters a community-driven approach, allowing team members to collaborate more effectively and providing support through integrated communication channels.
Optimizing Joins and Subqueries in SQL Queries
Joins and subqueries are fundamental concepts in SQL. However, they can also introduce performance challenges if not handled correctly.
Types of Joins
Join Type | Description |
---|---|
Inner Join | Returns rows with matches in both tables, commonly used and performs best. |
Outer Join | Returns all rows from one table and matched rows from the other, can be slower. |
Cross Join | Returns the Cartesian product of two tables, should be avoided unless necessary. |
Using Common Table Expressions (CTEs) can simplify complex queries and enhance performance:
WITH DepartmentEmployees AS (
SELECT e.first_name, e.last_name, d.department_name
FROM employees e
JOIN departments d ON e.department_id = d.id
)
SELECT * FROM DepartmentEmployees WHERE department_name = 'Sales';
DataGrip’s query profiling tools can help identify performance bottlenecks related to joins and subqueries, enabling you to make data-driven optimizations.
Utilizing Query Caching and Partitioning for Performance
Query caching and partitioning are advanced techniques to enhance SQL query performance.
Benefits of Query Caching
Query caching temporarily stores the results of executed queries, allowing subsequent executions to retrieve results faster without hitting the database. This technique is particularly beneficial for frequently executed queries.
Partitioning Large Datasets
Partitioning involves dividing a large dataset into smaller, more manageable pieces. This approach can significantly improve query performance by reducing the amount of data scanned during execution.
In DataGrip, you can configure caching and partitioning settings to optimize your database performance.
Refactoring SQL Queries for Better Performance
Refactoring SQL queries is an essential practice for enhancing performance. Simplifying complex queries makes them easier to read and execute.
Principles of Query Refactoring
Principle | Description |
---|---|
Break Down Complex Queries | Divide complex queries into smaller parts for better readability. |
Use Aliases | Aliasing makes queries shorter and more readable. |
Temporary Tables | Utilize temporary tables to store intermediate results. |
Here’s an example of refactoring a complex query:
-- Original complex query
SELECT e.first_name, e.last_name, d.department_name
FROM employees e
JOIN departments d ON e.department_id = d.id
WHERE d.department_name IN (SELECT name FROM departments WHERE location = 'New York');
-- Refactored query using a temporary table
CREATE TEMPORARY TABLE NewYorkDepartments AS
SELECT name FROM departments WHERE location = 'New York';
SELECT e.first_name, e.last_name, d.department_name
FROM employees e
JOIN NewYorkDepartments n ON e.department_id = n.id;
DataGrip's query editor supports these refactoring techniques, making it easier to test different query structures and identify the most efficient versions.
Monitoring and Profiling Query Performance in DataGrip
Maintaining optimal SQL query performance requires continuous monitoring and profiling. DataGrip offers several tools to help track query execution times and resource usage.
Setting Up Performance Alerts
You can set up alerts to notify you when query performance degrades. This proactive approach allows for timely intervention and adjustments.
Analyzing Historical Performance Data
DataGrip enables users to analyze historical performance data, providing insights into trends and patterns that may indicate underlying issues.
Integrating third-party tools with DataGrip can enhance your monitoring capabilities. For instance, Chat2DB offers advanced monitoring features, including AI-driven analysis to identify performance issues and suggest optimizations.
Conclusion
In conclusion, optimizing SQL query performance is a multi-faceted process involving execution plan analysis, effective indexing, join optimization, caching, refactoring, and continuous monitoring. By leveraging the powerful features of DataGrip and incorporating AI-driven tools like Chat2DB, developers can significantly enhance their database performance and efficiency. Chat2DB not only simplifies complex database operations but also provides intelligent suggestions for query optimizations, making it a superior choice for database management.
FAQ
-
What is SQL query optimization? SQL query optimization is the process of improving the performance of SQL queries to ensure faster execution and reduced resource consumption.
-
How do I analyze execution plans in DataGrip? You can analyze execution plans in DataGrip by executing your query and clicking on the "Explain Plan" button, which provides a visual representation of the query execution strategy.
-
What are the benefits of indexing in SQL? Indexing improves data retrieval speeds, reduces query execution times, and enhances overall database performance.
-
How can Chat2DB assist with SQL performance tuning? Chat2DB offers advanced AI features that streamline database management, including query optimization suggestions and performance monitoring tools, making it a powerful alternative to traditional tools.
-
What is the role of query caching in performance optimization? Query caching stores the results of frequently executed queries, allowing for faster retrieval without re-executing the query against the database, thus improving response times and lowering resource usage.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!