Optimizing Django Applications with PostgreSQL: A Comprehensive Guide
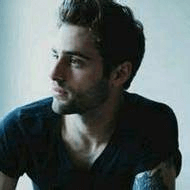
The Powerful Combination of Django and PostgreSQL for Web Development
Django and PostgreSQL create a dynamic environment for web application development, offering a combination of speed, reliability, and advanced features that developers appreciate. Django, a high-level Python web framework, facilitates rapid application development with its clean design and pragmatic approach. PostgreSQL, an advanced open-source relational database system, is renowned for its stability, robustness, and a rich feature set that caters to diverse application needs.
A significant feature of Django is its Object-Relational Mapping (ORM) system that allows developers to interact with databases using Python code, eliminating the need for writing SQL directly. This abstraction simplifies database operations, making it easier to perform CRUD (Create, Read, Update, Delete) actions. By leveraging PostgreSQL's advanced capabilities, such as JSONB (opens in a new tab) for efficient semi-structured data storage and full-text search functionalities, developers can build sophisticated applications with enhanced performance and user experience.
Moreover, PostgreSQL’s compliance with ACID (Atomicity, Consistency, Isolation, Durability) guarantees data reliability and integrity, which is vital for applications requiring transactional safety. Its scalability makes it an ideal choice for Django applications that anticipate growth.
Here’s a brief overview of key concepts associated with the Django and PostgreSQL synergy:
Key Term | Definition |
---|---|
ORM | Object-Relational Mapping, a programming technique that converts data between incompatible type systems. |
ACID | A set of properties that ensures reliable processing of database transactions. |
JSONB | A binary format in PostgreSQL for storing JSON data, facilitating efficient querying and indexing. |
Setting Up Your Environment for Optimal Performance with Django and PostgreSQL
To achieve optimal performance in your Django application with PostgreSQL, start with a proper setup. Configure your Django project to connect to your PostgreSQL database, typically done in the settings.py
file.
Here’s a sample configuration:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.postgresql',
'NAME': 'your_database_name',
'USER': 'your_database_user',
'PASSWORD': 'your_password',
'HOST': 'localhost', # Or your database host
'PORT': '5432', # Default PostgreSQL port
}
}
Environment-Specific Configurations
Maximize performance by implementing connection pooling using libraries like psycopg2
or django-db-geventpool
. Connection pooling minimizes the overhead of establishing new database connections, enhancing application responsiveness.
Consider using Docker for consistent development environments. Containerizing your Django application with PostgreSQL ensures uniformity across all development setups.
Utilize virtual environments to manage dependencies effectively, isolating your project dependencies from system-wide packages and minimizing version conflicts.
Environment variables are essential for securing sensitive information like database credentials. Instead of hardcoding them in your settings, store them as environment variables for better security.
For efficient management and querying of your PostgreSQL database, consider employing tools like Chat2DB (opens in a new tab). Chat2DB is an AI-powered database visualization and management tool that enhances database management efficiency through features like natural language processing and intelligent SQL editing.
Advanced Database Design and Optimization Techniques for Django Applications
Designing an optimal database schema is pivotal for your Django application's performance. Here are some advanced strategies to consider:
Normalization and Denormalization
Normalization organizes the database to reduce redundancy, enhancing data integrity. Conversely, denormalization can optimize performance by minimizing the number of joins in queries.
Indexing Strategies
Indexes are crucial for accelerating query performance. PostgreSQL supports various index types, including:
- B-tree indexes: Default type, suitable for equality and range queries.
- GIN (Generalized Inverted Index): Effective for indexing arrays and full-text searches.
- GiST (Generalized Search Tree): Ideal for indexing complex data types, like geometric data.
Example of creating a GIN index:
CREATE INDEX idx_gin ON your_table USING GIN (your_jsonb_column);
Partitioning
Partitioning manages large datasets by dividing them into smaller, more manageable sections, thus enhancing query performance.
Materialized Views
Employing materialized views can significantly improve performance for reporting and analytics by storing results of complex queries.
Foreign Keys and Constraints
Defining foreign keys and constraints ensures data integrity, preventing invalid data entries and maintaining table relationships.
Selecting the Right Data Types
Choosing appropriate data types impacts storage efficiency and query performance. For example, using integer
instead of bigint
can save space in large tables.
Leveraging PostgreSQL Features for Enhanced Django Application Functionality
PostgreSQL offers various features that can enrich the functionality of your Django applications:
Full-Text Search
Integrating full-text search capabilities can greatly enhance user experience by allowing efficient searches across large text fields. Here’s how to set it up in Django using PostgreSQL:
from django.contrib.postgres.search import SearchVector
from your_app.models import YourModel
results = YourModel.objects.annotate(
search=SearchVector('your_text_field'),
).filter(search='search_term')
Advanced Data Types
PostgreSQL supports advanced data types like arrays and JSONB, allowing flexible data modeling. Here’s an example of utilizing JSONB in Django:
from django.contrib.postgres.fields import JSONField
from django.db import models
class YourModel(models.Model):
data = JSONField()
Common Table Expressions (CTEs)
CTEs simplify complex queries, enhancing readability and maintainability.
Triggers
PostgreSQL triggers automate tasks in response to table events, such as data insertion or updates.
Stored Procedures
Using stored procedures encapsulates business logic within the database, improving performance by reducing data sent over the network.
Performance Tuning and Monitoring of Django Applications with PostgreSQL
Optimizing your Django application's performance requires continuous tuning and monitoring. Here are some effective strategies:
Query Optimization
Utilize the EXPLAIN
statement to analyze query execution plans and identify performance bottlenecks.
EXPLAIN ANALYZE SELECT * FROM your_table WHERE your_column = 'value';
Regular Maintenance
Routine database maintenance tasks, such as vacuuming and analyzing statistics, are critical for sustaining performance.
Monitoring Slow Queries
Tools like pg_stat_statements
help identify slow queries needing optimization.
Caching Strategies
Implementing caching reduces database load and improves response times. Django offers built-in caching capabilities that can be leveraged.
Server Configuration
Tweaking server parameters, such as shared_buffers
and work_mem
, can significantly influence performance.
For real-time monitoring and query analysis, consider utilizing Chat2DB (opens in a new tab). Chat2DB provides advanced AI features that facilitate performance monitoring by offering insights into database health and helping identify slow queries effortlessly.
Ensuring Security and Data Integrity in Django Applications
Security and data integrity are vital in application development. Here are some best practices:
SSL/TLS Encryption
Implement SSL/TLS to encrypt data in transit, safeguarding sensitive information from interception.
Parameterized Queries
Utilizing parameterized queries is crucial for preventing SQL injection attacks.
Database Roles and Permissions
Define roles and permissions to control access to sensitive data effectively.
Auditing and Logging
Implement auditing and logging to track data access and changes, essential for compliance and security.
Backups and Disaster Recovery
Regular backups and a disaster recovery plan are crucial for protecting against data loss.
Data Validation
Incorporate data validation in your Django application to maintain data quality and integrity.
Scalability and High Availability Strategies for Django Applications
As your application scales, consider these strategies:
Horizontal and Vertical Scaling
Explore horizontal scaling options like sharding and replication, or opt for vertical scaling by upgrading server resources.
Read Replicas
Using read replicas can alleviate read queries from the primary database, enhancing performance.
Load Balancing
Implement load balancing strategies to distribute traffic across multiple database instances.
Connection Pooling
Connection pooling solutions help manage multiple database connections efficiently.
Automated Failover
Automated failover solutions ensure high availability, minimizing downtime during failures.
Cloud-Based Solutions
Consider cloud-based PostgreSQL solutions for effective database scalability and management.
By leveraging advanced tools like Chat2DB (opens in a new tab), you can enhance your database management capabilities with AI features that simplify complex database tasks and improve overall efficiency.
FAQ
-
What is the advantage of using Django with PostgreSQL?
- The combination of Django's ORM and PostgreSQL's advanced features creates a powerful environment for building scalable and reliable web applications.
-
How can I optimize my Django application’s database performance?
- Utilize indexing, connection pooling, and caching strategies while regularly monitoring and optimizing your queries.
-
What is JSONB and how can I use it in Django?
- JSONB is a binary format for storing JSON data in PostgreSQL, allowing efficient querying. You can use Django's
JSONField
to work with JSONB data.
- JSONB is a binary format for storing JSON data in PostgreSQL, allowing efficient querying. You can use Django's
-
What monitoring tools are recommended for PostgreSQL?
- Tools like
pg_stat_statements
and Chat2DB (opens in a new tab) offer valuable insights into query performance and overall database health.
- Tools like
-
How can I ensure the security of my Django application?
- Implement SSL/TLS, use parameterized queries, define database roles, and maintain regular backups to secure your data.
Using Chat2DB not only streamlines your database management with its AI capabilities but also offers a superior alternative to traditional tools like DBeaver, MySQL Workbench, and DataGrip, making it the preferred choice for modern developers.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!