Mastering Oracle SQL: A Comprehensive Guide for Efficient Database Management
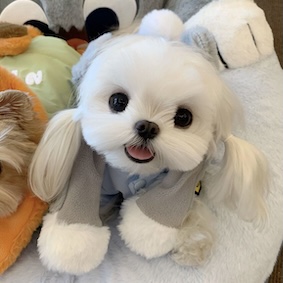
Introduction
Oracle SQL is a powerful language used for managing and manipulating relational databases. It plays a core role in database management and development, enabling users to perform various operations such as querying data, modifying data, and defining data structures. Oracle SQL is widely recognized for its robustness and versatility, making it a preferred choice among developers and data analysts.
In this article, we will explore the fundamental concepts of Oracle SQL and its importance in the realm of databases. We will also discuss Chat2DB, a tool that enhances Oracle SQL development efficiency by providing intuitive functionalities that streamline database operations. By the end of this guide, you will have a solid understanding of Oracle SQL and practical insights into using Chat2DB to improve your workflow.
Oracle SQL Basics
Oracle SQL consists of several key components, primarily focusing on four essential commands: SELECT, INSERT, UPDATE, and DELETE. Each of these commands serves a specific purpose in database interactions.
-
SELECT: This command retrieves data from one or more tables. It allows users to specify which columns to retrieve and can include filtering conditions.
-
INSERT: This command adds new records to a table. Users can specify values for each column in the new row.
-
UPDATE: This command modifies existing records within a table. It requires specifying which records to update and the new values for the specified columns.
-
DELETE: This command removes records from a table based on specified conditions.
In Oracle SQL, key terms such as tables, rows, columns, and views are fundamental. A table is a collection of related data entries consisting of rows and columns. Each row represents a single record, while each column corresponds to a specific attribute of the record.
A view is a virtual table created by a query that selects data from one or more tables. Views do not store data themselves but display data stored in tables.
Example of Basic Oracle SQL Syntax
Here’s a simple example demonstrating the use of these commands:
-- Create a table
CREATE TABLE employees (
employee_id NUMBER PRIMARY KEY,
first_name VARCHAR2(50),
last_name VARCHAR2(50),
department VARCHAR2(50)
);
-- Insert a record
INSERT INTO employees (employee_id, first_name, last_name, department)
VALUES (1, 'John', 'Doe', 'Sales');
-- Update a record
UPDATE employees
SET department = 'Marketing'
WHERE employee_id = 1;
-- Select records
SELECT * FROM employees;
-- Delete a record
DELETE FROM employees
WHERE employee_id = 1;
Differences Between Oracle SQL and Other SQL Dialects
While SQL is a standard language used across various database systems, Oracle SQL has unique features that distinguish it from other dialects such as MySQL and PostgreSQL. For example, Oracle SQL supports advanced features like PL/SQL (Procedural Language/SQL), which allows for the creation of complex stored procedures and functions. In contrast, MySQL and PostgreSQL have different implementations and do not support all Oracle-specific features.
Building Complex Queries
Complex queries in Oracle SQL enable users to extract meaningful insights from multiple tables. This involves using JOIN, GROUP BY, and HAVING clauses.
JOIN Types
-
INNER JOIN: Combines rows from two or more tables based on a related column. It returns only the rows with matching values in both tables.
-
OUTER JOIN: Returns all rows from one table and the matched rows from the other table. If there is no match, NULL values are returned for columns of the table without a match.
-
CROSS JOIN: Returns the Cartesian product of two tables, combining every row from the first table with every row from the second table.
Example of Complex Queries
Here’s how to construct complex queries using JOINs:
-- Create tables
CREATE TABLE departments (
department_id NUMBER PRIMARY KEY,
department_name VARCHAR2(50)
);
-- Insert sample data
INSERT INTO departments (department_id, department_name)
VALUES (1, 'Sales'), (2, 'Marketing');
-- INNER JOIN example
SELECT e.first_name, e.last_name, d.department_name
FROM employees e
INNER JOIN departments d ON e.department = d.department_name;
-- LEFT OUTER JOIN example
SELECT e.first_name, e.last_name, d.department_name
FROM employees e
LEFT OUTER JOIN departments d ON e.department = d.department_name;
-- GROUP BY example
SELECT department, COUNT(*) as employee_count
FROM employees
GROUP BY department;
-- HAVING example
SELECT department, COUNT(*) as employee_count
FROM employees
GROUP BY department
HAVING COUNT(*) > 1;
Subqueries and Union Queries
Subqueries are queries nested within another SQL query. They can be used in SELECT, INSERT, UPDATE, or DELETE statements. Union queries combine the results of two or more SELECT statements.
Performance Optimization
When constructing complex queries, it’s crucial to consider performance optimization. Inefficient queries can lead to slow performance and increased resource consumption. Here are a few strategies to optimize your Oracle SQL queries:
-
Indexes: Create indexes on frequently queried columns. Indexes speed up data retrieval but can slow down INSERT, UPDATE, and DELETE operations.
-
EXPLAIN PLAN: Use the EXPLAIN PLAN command to analyze how Oracle executes a query. This helps identify performance bottlenecks.
Example of Performance Optimization
-- Create an index
CREATE INDEX idx_department ON employees(department);
-- Analyze query performance
EXPLAIN PLAN FOR
SELECT * FROM employees WHERE department = 'Sales';
Data Manipulation and Transaction Management
Data Manipulation Language (DML) in Oracle SQL includes commands that manage data stored in a database. The fundamental DML operations are INSERT, UPDATE, and DELETE.
Transaction Management
Oracle SQL adheres to the ACID properties:
-
Atomicity: Ensures that a transaction is treated as a single unit, which either completes entirely or not at all.
-
Consistency: Guarantees that the database remains in a valid state before and after the transaction.
-
Isolation: Ensures that transactions do not interfere with each other.
-
Durability: Guarantees that once a transaction is committed, it remains permanent, even in the case of a system failure.
Example of Transaction Management
BEGIN;
INSERT INTO employees (employee_id, first_name, last_name, department)
VALUES (2, 'Jane', 'Smith', 'Finance');
UPDATE employees
SET department = 'HR'
WHERE employee_id = 2;
COMMIT;
-- If an error occurs, roll back the transaction
ROLLBACK;
Error Handling
Oracle SQL provides mechanisms for error handling through exception handling in PL/SQL blocks. Proper error handling ensures the integrity of data and the smooth execution of database transactions.
Performance Optimization Techniques
Optimizing Oracle SQL queries is crucial for maintaining efficient database operations. Below are several techniques for optimizing query performance:
Using Indexes
Indexes significantly enhance the speed of data retrieval operations. There are various types of indexes in Oracle SQL, such as:
-
B-tree Index: The default index type, effective for most queries involving equality and range conditions.
-
Bitmap Index: Useful for columns with low cardinality, such as gender or status.
Query Rewriting
Rewriting queries can often lead to better performance. This involves modifying the structure of the query to reduce complexity or improve execution plans.
Analyzing Execution Plans
Using the EXPLAIN PLAN command helps visualize how Oracle will execute a query. This insight allows developers to identify potential performance issues before executing the query.
Example of Performance Analysis
-- Analyze a query's execution plan
EXPLAIN PLAN FOR
SELECT * FROM employees WHERE first_name = 'John';
-- Display the execution plan
SELECT * FROM TABLE(DBMS_XPLAN.DISPLAY());
The Advantages of Using Chat2DB
Chat2DB is a powerful tool designed to enhance the Oracle SQL development experience. It offers various features that streamline database operations and improve productivity.
User-Friendly Interface
Chat2DB provides an intuitive interface that simplifies the process of database management. Users can easily navigate through different functionalities, making it accessible for both new and experienced developers.
Automation Features
The automation capabilities of Chat2DB help reduce the workload for developers. Automated query generation and data visualization tools allow users to focus on more critical tasks while minimizing manual effort.
Connecting to Databases
Chat2DB simplifies the connection process to various databases, including Oracle SQL. Users can quickly establish connections and start executing queries without complex configurations.
Example of Using Chat2DB
Here’s how to connect to an Oracle database using Chat2DB:
- Open Chat2DB.
- Select "Create New Connection."
- Choose "Oracle" as the database type.
- Enter the connection details, including hostname, port, and credentials.
- Click "Connect" to establish the database connection.
Successful Use Cases
Many developers have successfully leveraged Chat2DB to enhance their Oracle SQL development. For instance, a team working on a large-scale application reported a 30% increase in productivity after integrating Chat2DB into their workflow. The automation features allowed them to generate complex queries quickly and visualize data effectively.
Further Learning and Exploration
As you delve into the world of Oracle SQL, consider exploring advanced topics such as PL/SQL programming, performance tuning, and database security. Continuous learning is essential for keeping up with the evolving landscape of database technologies.
Utilizing tools like Chat2DB can further enhance your development experience, allowing you to focus on building robust database applications efficiently. Embrace the power of Oracle SQL and leverage the capabilities of Chat2DB to elevate your database management skills.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!