Understanding PostgreSQL Enum: A Comprehensive Guide to Effectively Using Enumerated Types
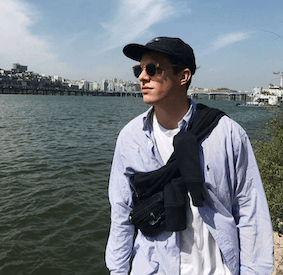
Introduction to PostgreSQL Enumerated Types (Enums)
In the world of database management, particularly with PostgreSQL (opens in a new tab), enumerated types—commonly referred to as enums—provide a robust mechanism for defining a set of predefined values. These enums stand apart from other data types such as VARCHAR
or INTEGER
, offering unique benefits in terms of data integrity and type safety. By restricting the permissible values that can be stored in a column, enums significantly minimize the risk of data entry errors, thus enhancing the overall quality of your data.
Enums are especially beneficial when representing specific categories or status codes. For example, if you need to track the status of a project, you might define an enum named project_status
with values like pending
, in_progress
, and completed
. This approach not only enhances the readability of your queries but also ensures a consistent representation of data across your application.
Key Advantages of Using Enums in PostgreSQL
- Improved Data Integrity: Enums help maintain consistent and valid data entries by limiting the possible values for a column.
- Enhanced Readability: Enums make your SQL queries more self-explanatory by using meaningful identifiers instead of arbitrary strings or numbers.
- Type Safety: Enums provide compile-time checks, ensuring that only valid values are utilized in your database operations.
Common Use Cases for Enums
Enums can be particularly advantageous in various scenarios, including:
- Status Tracking: Representing states like
active
,inactive
, orarchived
. - Categorization: Using enums for product categories such as
electronics
,clothing
, orfurniture
. - User Roles: Defining roles like
admin
,editor
, orviewer
.
Streamlining Queries with PostgreSQL Enums
Utilizing enums can lead to simpler and more efficient queries. For instance, if you have a table named projects
with a status
column defined as an enum, filtering projects by status becomes straightforward:
SELECT * FROM projects WHERE status = 'in_progress';
This method enhances readability and guarantees that only valid statuses are queried, further supporting data integrity.
Creating and Managing Enums in PostgreSQL
Creating an enum in PostgreSQL is straightforward with the CREATE TYPE
command. Here’s how to define an enum type:
CREATE TYPE project_status AS ENUM ('pending', 'in_progress', 'completed');
Best Practices for Naming Conventions
When establishing enums, it’s essential to adopt clear and consistent naming conventions. This practice aids in maintaining organization within your database schema. For instance, prefixing enum names with the related table name can enhance clarity:
CREATE TYPE task_priority AS ENUM ('low', 'medium', 'high');
Altering Enums with ALTER TYPE
PostgreSQL allows you to modify existing enums using the ALTER TYPE
command. You can add new values as follows:
ALTER TYPE project_status ADD VALUE 'on_hold';
However, be cautious—removing values from an enum directly is not permitted. A common workaround involves creating a new type and migrating data.
Migrating Data When Updating Enums
When updating enums, it’s critical to migrate existing data to maintain consistency. Here’s a step-by-step guide:
- Create a new enum type with the desired values.
- Update the table column to utilize the new enum type.
- Migrate data from the old enum type to the new type.
- Drop the old enum type if it’s no longer needed.
Tools for Managing Enums
To simplify the creation and management of enums, consider leveraging tools like Chat2DB (opens in a new tab). This AI-powered database management tool streamlines processes, allowing developers and database administrators to manage their PostgreSQL enums more efficiently.
Integrating Enums into Your PostgreSQL Database Schema
Integrating enums into your database schema is seamless. You can use enums as column data types when creating tables. Here’s an example of a tasks
table that incorporates the task_priority
enum:
CREATE TABLE tasks (
id SERIAL PRIMARY KEY,
title VARCHAR(255) NOT NULL,
priority task_priority NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
Combining Enums with Other Data Types
Enums can be combined with other data types to create complex structures. For example, consider a table that includes both enums and integers:
CREATE TABLE projects (
id SERIAL PRIMARY KEY,
name VARCHAR(255) NOT NULL,
status project_status NOT NULL,
budget INTEGER CHECK (budget > 0)
);
Referencing Enums in SQL Queries
When querying data, you can reference enums just like any other data type. For instance, to fetch all tasks with a high priority, you would use:
SELECT * FROM tasks WHERE priority = 'high';
Enforcing Data Validity with CHECK Constraints
Enums can also be utilized with CHECK
constraints to ensure data validity. Here’s an example:
CREATE TABLE users (
id SERIAL PRIMARY KEY,
username VARCHAR(50) UNIQUE NOT NULL,
role user_role NOT NULL,
CHECK (role IN ('admin', 'editor', 'viewer'))
);
Indexing Strategies for Enum Columns
To optimize performance, consider indexing enum columns, particularly if you frequently query them. Here’s how you can create an index on the status
column of the projects
table:
CREATE INDEX idx_project_status ON projects(status);
Advanced Usage of Enums in PostgreSQL
Enums in PostgreSQL can be leveraged in various advanced scenarios, including stored procedures and functions. For instance, you can create a stored procedure that utilizes enums to handle business logic:
CREATE OR REPLACE FUNCTION update_project_status(p_id INT, p_status project_status)
RETURNS VOID AS $$
BEGIN
UPDATE projects SET status = p_status WHERE id = p_id;
END;
$$ LANGUAGE plpgsql;
Enums and Triggers
Enums can interact with database triggers to automate data validation. For example, you could create a trigger that checks the status of a project before updating:
CREATE FUNCTION check_project_status()
RETURNS TRIGGER AS $$
BEGIN
IF NEW.status NOT IN ('pending', 'in_progress', 'completed', 'on_hold') THEN
RAISE EXCEPTION 'Invalid project status';
END IF;
RETURN NEW;
END;
$$ LANGUAGE plpgsql;
CREATE TRIGGER validate_status
BEFORE UPDATE ON projects
FOR EACH ROW EXECUTE FUNCTION check_project_status();
Working with JSONB and Enums
Enums can also be utilized alongside the JSONB data type, simplifying the management of complex data structures. For example, you can store project details as JSONB while using enums for status:
CREATE TABLE project_details (
id SERIAL PRIMARY KEY,
details JSONB,
status project_status NOT NULL
);
Enums in PostgreSQL Extensions
PostgreSQL allows for the use of enums in extensions and custom types. This flexibility enables developers to create tailored solutions that leverage the benefits of enums in their applications.
Partitioning and Sharding Strategies
When dealing with large datasets, enums can play a significant role in partitioning and sharding strategies. By using enums to determine data distribution, you can optimize query performance and storage efficiency.
Performance Considerations and Best Practices
Using enums can have performance implications compared to other data types. Enums are generally more efficient in terms of storage and querying, especially with large datasets. However, it’s vital to monitor and optimize their usage to avoid potential pitfalls.
Monitoring Enum Usage
To effectively monitor enum usage, consider utilizing tools like Chat2DB (opens in a new tab), which provides features for tracking performance metrics and database health.
Avoiding Common Performance Pitfalls
When working with enums, be wary of:
- Excessive Type Casting: Avoid frequent type conversions, as this can impede performance.
- Inefficient Indexing: Properly index enum columns to enhance query performance.
Documenting and Maintaining Enums
Thorough documentation of your enums is essential for long-term success. Clearly document the purpose and usage of each enum, along with any constraints or dependencies.
Testing and Validating Enum Implementations
Before deploying enums in production, it’s important to thoroughly test and validate their implementations. This includes checking for edge cases and ensuring that your enums integrate seamlessly with your application logic.
Supporting Database Migrations
Enums can significantly aid in database migrations and version control. By maintaining a clear structure and consistent naming conventions, you can easily track changes and ensure backward compatibility.
Case Studies and Real-World Applications of Enums in PostgreSQL
Enums have been effectively implemented across various industries, enhancing data management and application performance. For instance, in an e-commerce platform, enums can manage product categories and inventory status effectively.
Lessons Learned from Successful Implementations
Organizations that have adopted enums report improvements in data integrity and readability. They highlight the importance of clear naming conventions and thorough documentation.
Simplifying Complex Queries
Many developers find that enums simplify complex queries. For example, a logistics company used enums to manage shipment statuses, leading to more efficient data retrieval and processing.
Enhancing Collaboration Among Development Teams
Enums facilitate better communication among development teams by providing a clear and consistent representation of data. This clarity leads to fewer misunderstandings and a more cohesive development process.
Feedback from Developers
Developers who have integrated enums into their projects often emphasize their ease of use and the improved data integrity they provide. The ability to enforce valid values through enums has transformed data management for many teams.
By using tools like Chat2DB (opens in a new tab), developers can further enhance their experience with enums, streamlining management and monitoring processes.
Frequently Asked Questions (FAQ)
-
What is an enum in PostgreSQL? An enum in PostgreSQL is a data type consisting of a static, ordered set of values. It enforces data integrity by restricting the possible values that can be stored in a column.
-
How do you create an enum in PostgreSQL? You can create an enum using the
CREATE TYPE
command followed by the enum name and its possible values. For example:CREATE TYPE project_status AS ENUM ('pending', 'in_progress', 'completed');
-
Can I alter an existing enum? Yes, you can use the
ALTER TYPE
command to add new values to an existing enum. However, removing values directly is not allowed without workarounds. -
What are the advantages of using enums? Enums improve data integrity, enhance readability, and provide type safety by restricting the values that can be stored in a column.
-
How can Chat2DB help with managing enums? Chat2DB is an AI-powered database management tool that simplifies the creation, management, and monitoring of enums in PostgreSQL, enhancing overall database efficiency.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!