How to Leverage Prisma ORM for Efficient Database Management: A Comprehensive Guide
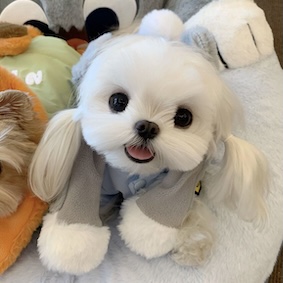
Prisma ORM is an open-source database toolkit designed to streamline database management for developers by automating repetitive tasks and integrating seamlessly into modern development workflows. This article explores the core components of Prisma ORM, including Prisma Client, Prisma Migrate, and Prisma Studio, while providing detailed setup instructions. Additionally, we'll discuss how integrating Prisma ORM with Chat2DB, an AI-driven database management tool, can significantly enhance efficiency in database operations.
Understanding Prisma ORM: Key Components and Features
Prisma ORM is designed to enhance productivity by automating tedious tasks that developers often encounter. Its core components include:
Component | Description |
---|---|
Prisma Client | An auto-generated and type-safe database client that simplifies database interactions. |
Prisma Migrate | A powerful migration tool that allows developers to manage database schema changes effectively. |
Prisma Studio | A web-based GUI for interacting with the database, providing a user-friendly way to inspect and manipulate data. |
Prisma ORM supports various databases, including PostgreSQL, MySQL, SQLite, and SQL Server, ensuring versatility across different project environments. Its community-driven development approach allows for continuous improvement and feature expansion, making it a valuable asset in any developer's toolkit.
Setting Up Prisma ORM in Your Project
To integrate Prisma ORM into your application, follow these steps:
-
Install Prisma CLI: Use npm to install the Prisma CLI:
npm install prisma --save-dev
-
Initialize a Prisma project:
npx prisma init
This command creates a new directory with a basic configuration.
-
Configure
schema.prisma
: Define your data model and database connection settings in this file. Here’s a sample configuration:datasource db { provider = "postgresql" // Choose your database provider url = env("DATABASE_URL") // Your database URL } generator client { provider = "prisma-client-js" } model User { id Int @id @default(autoincrement()) name String email String @unique }
-
Connect to your database: Ensure that your environment variables are set correctly to manage database credentials securely.
-
Generate Prisma Client:
npx prisma generate
-
Create migrations: Manage schema changes without data loss:
npx prisma migrate dev --name init
This command creates migration files based on your schema and applies them to your database.
Utilizing Prisma Client for Type-Safe Database Access
Prisma Client provides a powerful API for database operations, emphasizing type safety. Here’s how to perform common CRUD operations:
Create
const { PrismaClient } = require('@prisma/client');
const prisma = new PrismaClient();
async function main() {
const newUser = await prisma.user.create({
data: {
name: 'Alice',
email: 'alice@example.com',
},
});
console.log(newUser);
}
main()
.catch(e => console.error(e))
.finally(async () => {
await prisma.$disconnect();
});
Read
async function getUsers() {
const users = await prisma.user.findMany();
console.log(users);
}
getUsers();
Update
async function updateUser(id, newName) {
const updatedUser = await prisma.user.update({
where: { id: id },
data: { name: newName },
});
console.log(updatedUser);
}
updateUser(1, 'Bob');
Delete
async function deleteUser(id) {
const deletedUser = await prisma.user.delete({
where: { id: id },
});
console.log(deletedUser);
}
deleteUser(1);
Prisma Client simplifies complex queries with fluent API methods, allowing you to structure queries effectively with clauses like select
, include
, where
, and orderBy
. For example:
const user = await prisma.user.findFirst({
where: { email: 'alice@example.com' },
select: { id: true, name: true },
});
Streamlining Database Migrations with Prisma Migrate
Prisma Migrate plays a crucial role in managing database schema changes. It follows a declarative data modeling approach, translating your schema into database migrations. The workflow involves:
-
Creating a migration:
npx prisma migrate dev --name add-age-field
-
Applying migrations: Prisma Migrate applies changes and updates your database schema.
-
Rollback capabilities: You can revert to previous migration versions if needed.
-
Migration history: Track schema evolution over time with a complete migration history.
This approach greatly reduces the chances of migration conflicts. It's essential to validate changes before applying them to the database, which Prisma Migrate allows through its migration preview feature.
Enhancing Developer Experience with Prisma Studio
Prisma Studio serves as a modern GUI-based database management tool, providing a visual interface for inspecting and editing database records. Key features include:
- Real-time data exploration and manipulation.
- Quick troubleshooting of issues.
- Prototyping and testing queries without writing code.
Prisma Studio integrates seamlessly with Prisma Client, enhancing the overall developer experience by facilitating intuitive interaction with the database. Developers can explore data relationships, making it easier to understand complex data models.
Integrating Prisma ORM with Chat2DB
Integrating Prisma ORM with Chat2DB (opens in a new tab) can significantly enhance your database management efficiency. Chat2DB is an AI-driven database visualization and management tool that supports over 24 databases, providing a seamless and intuitive experience for developers. The integration allows for real-time data updates and intuitive query building, utilizing natural language processing to generate SQL queries effortlessly.
Advantages of Using Chat2DB with Prisma ORM
-
AI-Powered Query Generation: Chat2DB assists developers in generating SQL queries using natural language, making it accessible for users unfamiliar with SQL syntax.
-
Multi-Database Support: Chat2DB's capabilities span across various databases, allowing for a consolidated management approach.
-
Data Visualization: The ability to generate visual charts and graphs enhances data analysis and presentation.
-
Collaborative Features: Chat2DB facilitates collaborative work among team members, improving workflow efficiency.
-
Intelligent SQL Editor: The intelligent SQL editor in Chat2DB provides suggestions and auto-completions, reducing the likelihood of errors.
By utilizing Chat2DB in conjunction with Prisma ORM, developers can significantly enhance their database management processes, making them more efficient and user-friendly.
Optimizing Performance and Scalability with Prisma ORM
Performance optimization is crucial when managing databases. Prisma ORM offers strategies for enhancing database performance:
-
Efficient Handling of Large Datasets: Use pagination and batching techniques to manage large datasets effectively.
-
Optimizing Query Performance: Leverage database indexes and efficient query plans to speed up data retrieval.
-
Monitoring and Profiling: Regularly monitor and profile database queries to identify and resolve performance bottlenecks.
-
Scaling Applications: Prisma ORM supports horizontal scaling, allowing you to handle increased loads smoothly.
-
Concurrency Management: Maintain data consistency in high-traffic applications by effectively managing concurrent operations.
By following best practices and leveraging the evolving features of Prisma ORM, developers can future-proof their database architecture and ensure sustainable performance.
FAQs
-
What is Prisma ORM?
- Prisma ORM is an open-source database toolkit that simplifies database management for developers through features like Prisma Client, Prisma Migrate, and Prisma Studio.
-
How does Prisma Client ensure type safety?
- Prisma Client automatically generates types based on your data models, ensuring consistency and reducing runtime errors across your application.
-
What is the role of Prisma Migrate?
- Prisma Migrate helps manage database schema changes efficiently, allowing developers to create, apply, and track migrations.
-
How can Chat2DB enhance my experience with Prisma ORM?
- Chat2DB provides AI-driven features for intuitive database interactions, including natural language query generation and real-time data visualization.
-
Is Prisma ORM compatible with all databases?
- Yes, Prisma ORM supports a variety of databases including PostgreSQL, MySQL, SQLite, and SQL Server.
With the right tools and strategies, effective database management becomes a streamlined process. Consider exploring Chat2DB (opens in a new tab) to leverage its AI capabilities alongside Prisma ORM for a comprehensive database experience.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!