How to Optimize Your Database: Effective Solutions for the N+1 Query Problem
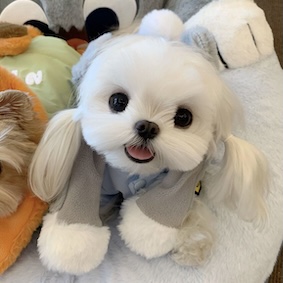
Optimizing database performance is essential for any application that relies on data retrieval. One common issue faced by developers is the N+1 query problem, which arises when an application executes one query to retrieve a collection, followed by an additional query for each item in that collection. This can lead to increased response times and resource consumption, ultimately degrading the performance of the application. Addressing the N+1 query problem is crucial for creating scalable and efficient applications. In this article, we will explore various aspects of the N+1 query problem, including identification, strategies for resolution, and the advantages of using tools like Chat2DB (opens in a new tab) to enhance database management and optimize queries.
Understanding the N+1 Query Problem
The N+1 query problem is a well-known performance issue that arises in database-driven applications, particularly when using Object-Relational Mapping (ORM) frameworks. When an application retrieves a collection of items, it often results in a separate query for each item in that collection, leading to N additional queries (where N is the number of items). This can significantly slow down response times and increase database load.
How It Occurs
The N+1 query problem typically occurs when developers fail to anticipate the number of queries that will need to be executed. For instance, in a blog application built with an ORM like Django or Ruby on Rails, when retrieving a list of blog posts along with their associated comments, the application may first fetch the posts with a single query. However, it then executes an additional query for each post to retrieve its comments. If you have 100 posts, this leads to a total of 101 queries (1 for the posts + 100 for the comments).
Negative Impact on Performance
The impact of the N+1 query problem can be severe, leading to:
- Increased response times, as each additional query adds latency.
- Higher resource consumption on the database server, which can lead to slowdowns or crashes under heavy load.
- Difficulty in scaling applications due to inefficient database interactions.
To illustrate, consider a popular framework like Django, where developers may overlook this issue during the initial development phases, ultimately leading to performance bottlenecks that can be challenging to resolve later.
Importance of Query Optimization
Addressing the N+1 query problem is vital for ensuring that applications can scale effectively and perform efficiently. Query optimization techniques can help minimize the number of database queries executed, improving overall application performance.
Identifying N+1 Queries in Your Application
Detecting N+1 query problems is the first step in resolving them. Here are several techniques that developers can use to identify these issues in their applications:
Technique | Description |
---|---|
Logging and Monitoring Tools | Utilize tools to analyze logs and spot multiple queries executed in quick succession. |
ORM Tools | Understand your ORM; tools like django-debug-toolbar can visualize executed queries. |
Profiling Tools | Use tools like New Relic or Skylight to identify performance bottlenecks. |
Analyzing Query Execution Plans | Understand how the database executes queries to reveal N+1 query impacts. |
Common Signs | Look for slow loading times or high database load during application usage. |
Strategies for Solving N+1 Query Problems
Once N+1 query problems have been identified, several effective strategies can be employed to resolve them:
Eager Loading
Eager loading is a technique that allows developers to load related data in a single query, thus reducing the total number of queries executed. Many ORMs provide built-in features for eager loading. For example, in Django, you can use the select_related()
and prefetch_related()
methods:
# Example in Django
from blog.models import Post
# Eager load comments when retrieving posts
posts = Post.objects.prefetch_related('comments').all()
Batch Processing Techniques
Batch processing techniques can minimize the number of database hits by grouping queries. For instance, you can fetch all related items in one go instead of fetching each one individually. This can be achieved using the IN
clause in SQL:
-- Example of batch processing in SQL
SELECT * FROM comments WHERE post_id IN (1, 2, 3, ..., 100);
Caching Mechanisms
Caching can alleviate database load caused by repetitive queries. By storing the results of expensive queries in memory, subsequent requests can be served faster without hitting the database.
Denormalization of Tables
In some cases, denormalization may be an effective strategy to resolve N+1 issues. By storing redundant data in a single table, the need for complex joins can be minimized.
Trade-offs Between Strategies
Each strategy has its trade-offs, and developers must carefully evaluate their application-specific requirements before implementation. Testing and validating solutions is essential to ensure they effectively address the N+1 query problem.
Case Study: Optimizing Databases with Chat2DB
To illustrate how to address and optimize N+1 query problems, we can look at Chat2DB (opens in a new tab), an AI database visualization management tool that enhances database management efficiency.
Unique Features of Chat2DB
Chat2DB offers several unique features that assist developers in identifying and resolving N+1 queries:
- Natural Language Processing: Developers can generate SQL queries using natural language, making it easier to construct complex queries quickly.
- Intelligent SQL Editor: This feature provides real-time suggestions and optimizations, helping to avoid common pitfalls such as N+1 queries.
- Data Analysis: Chat2DB facilitates data analysis with natural language, allowing developers to glean insights without extensive coding.
Integration Capabilities
Chat2DB integrates seamlessly with various databases and development frameworks, providing a unified platform for database management. Its AI-driven functionalities allow developers to enhance their applications' performance effectively.
Success Stories
Many developers have experienced improved application performance by using Chat2DB to optimize their database queries. Testimonials highlight how the tool has streamlined query generation and analysis, ultimately leading to better resource management and faster response times.
Step-by-Step Guide Using Chat2DB
- Connect to Your Database: Start by connecting Chat2DB to your existing database.
- Identify Queries: Use the intelligent SQL editor to identify queries that may be contributing to N+1 problems.
- Optimize Queries: Leverage suggestions from Chat2DB to optimize identified N+1 queries.
- Analyze Performance: Use built-in analysis tools to track the performance of your queries over time.
Limitations of Chat2DB
While Chat2DB offers numerous advantages, developers should be aware of its limitations, such as potential compatibility issues with less common databases. However, the benefits of using Chat2DB often outweigh these concerns.
Tools and Resources for Database Optimization
A variety of tools and resources are available for optimizing database queries and solving N+1 query issues:
Database Management Systems (DBMS)
DBMS play a crucial role in query optimization. Popular systems like MySQL (opens in a new tab), PostgreSQL (opens in a new tab), and MongoDB (opens in a new tab) provide features that can assist in optimizing queries.
Popular Optimization Tools
Tools like Hibernate for Java and ActiveRecord for Ruby are popular choices among developers for optimizing ORM interactions. These frameworks offer built-in methods for eager loading and batch processing.
Online Resources and Communities
Developers should take advantage of online resources, courses, and communities to learn more about query optimization techniques. Websites like Stack Overflow (opens in a new tab) and forums dedicated to database technologies can provide valuable insights.
Continuous Learning
Staying updated with the latest developments in database technology is essential. Continuous learning and experimentation will help developers master database optimization techniques.
Ensuring Ongoing Database Efficiency
Maintaining efficient database performance over time requires regular monitoring and audits. Here are some strategies to ensure ongoing efficiency:
Regular Monitoring
Implementing monitoring tools can help catch new N+1 query problems early. Tools like New Relic provide real-time insights into database performance.
Automated Testing
Encouraging automated testing practices can help developers identify performance regressions related to database queries. This ensures that any changes made to the codebase do not inadvertently introduce new issues.
Documentation and Knowledge Sharing
Maintaining documentation and fostering knowledge sharing among development teams can prevent recurring issues. A shared understanding of best practices can lead to better overall application performance.
Collaborating with Database Administrators (DBAs)
Working closely with DBAs can optimize query performance and ensure that the database is configured for optimal efficiency. Collaboration can lead to a culture of performance awareness within development teams.
FAQs
-
What is the N+1 query problem? The N+1 query problem occurs when an application executes one query to retrieve a collection and additional queries for each item in that collection, leading to performance degradation.
-
How can I identify N+1 queries in my application? You can identify N+1 queries by using logging and monitoring tools, analyzing query execution plans, and utilizing profiling tools.
-
What are effective strategies for resolving N+1 query issues? Effective strategies include eager loading, batch processing, caching mechanisms, and sometimes denormalization of tables.
-
How does Chat2DB help with N+1 query optimization? Chat2DB assists developers by offering features like natural language query generation, intelligent SQL editing, and data analysis capabilities.
-
What tools can I use for database optimization? Popular tools for database optimization include Hibernate, ActiveRecord, and various monitoring and profiling tools.
By understanding the N+1 query problem and employing effective strategies to mitigate its impact, developers can enhance the performance of their applications. Tools like Chat2DB (opens in a new tab) can significantly aid in this process, enabling smarter and more efficient database management. Switch to Chat2DB for an advanced AI-driven experience in optimizing your database queries and managing your data more effectively.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!