How to Efficiently Use SQL Aliases for Cleaner Code
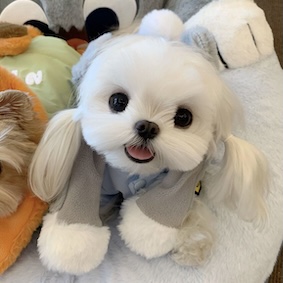
SQL aliases are a powerful feature that can significantly enhance the readability and maintainability of your SQL queries. By providing temporary names for tables and columns, SQL aliases simplify complex queries and improve code clarity. In this article, we will explore the various applications of SQL aliases, best practices for their usage, and advanced techniques that leverage this feature. We will also discuss how tools like Chat2DB (opens in a new tab) enhance the experience of working with SQL aliases through artificial intelligence capabilities. Throughout the article, we will provide detailed code examples and practical scenarios to demonstrate the value of SQL aliases in real-world applications.
Understanding SQL Aliases: Enhancing Clarity and Simplicity
SQL aliases serve as temporary names for tables or columns in SQL queries. They are essential for simplifying complex queries and improving readability. The syntax for creating aliases involves using the AS
keyword, although it is optional and can be omitted in many cases. For example, the following SQL snippet demonstrates how to create an alias for a column:
SELECT first_name AS name
FROM employees;
In this query, first_name
is given the alias name
, making the output more readable. Similarly, you can create an alias for a table:
SELECT e.name
FROM employees AS e;
Using aliases is particularly beneficial in large datasets and complicated joins, where they help prevent confusion and reduce query length. For instance, when joining multiple tables, aliases can clarify which columns belong to which tables, thus preventing ambiguity.
Common Misconceptions About SQL Aliases
A common misconception is that aliases impact database performance. In reality, aliases do not change the database schema or the actual data; they merely provide a temporary reference in the query context. Additionally, the scope of an alias is limited to the query in which it is defined, meaning it cannot be referenced outside that query.
Practical Applications of SQL Aliases: Enhancing Readability
SQL aliases are particularly beneficial in several scenarios, especially when dealing with multiple tables. For example, consider the following SQL query that joins two tables:
SELECT e.first_name, d.department_name
FROM employees AS e
JOIN departments AS d ON e.department_id = d.id;
In this case, e
and d
are aliases for the employees
and departments
tables, respectively. This usage makes the query cleaner and easier to understand, especially for those who may not be familiar with the underlying table structures.
Self-Joins and Derived Columns
Aliases are also essential in scenarios where a table is joined with itself, known as a self-join. Here’s an example:
SELECT e1.first_name AS employee_name, e2.first_name AS manager_name
FROM employees AS e1
JOIN employees AS e2 ON e1.manager_id = e2.id;
In this query, e1
and e2
allow us to differentiate between the employee and their manager, enhancing clarity.
Moreover, when dealing with derived columns, aliases provide meaningful names that enhance both readability and understanding. For instance:
SELECT salary * 1.1 AS updated_salary
FROM employees;
Here, updated_salary
is a derived column that clearly indicates the purpose of this calculation.
Role of Aliases in Subqueries
Aliases also play a crucial role in integrating results from subqueries into main queries. For example:
SELECT e.name, (SELECT AVG(salary) FROM employees) AS average_salary
FROM employees AS e;
In this case, average_salary
is defined as an alias for the subquery result, making it easy to reference in the context of the main query.
Best Practices for Using SQL Aliases: Naming and Documentation
To effectively incorporate aliases into your SQL code, consider adopting the following best practices:
Naming Conventions
Use short, descriptive names for your aliases to enhance clarity and maintainability. Avoid reserved keywords as alias names to prevent syntax errors. For example:
SELECT e.first_name AS employee
FROM employees AS e;
This approach makes it clear that employee
refers to the first name of an employee.
Consistency in Naming
Maintain consistency in alias naming across different queries or projects. This practice facilitates easier understanding and collaboration among team members. Document alias usage in code comments, especially in complex queries, to aid future code reviews and maintenance.
Common Aliasing Patterns
Adopt common aliasing patterns that enhance readability. For instance, prefixing table aliases with t
or column aliases with c
can help differentiate them quickly. Here’s an example:
SELECT t1.first_name AS c_name, t2.department_name AS c_department
FROM employees AS t1
JOIN departments AS t2 ON t1.department_id = t2.id;
Combining Aliases with SQL Best Practices
Consider using aliases in conjunction with other SQL best practices, such as proper formatting and indentation, for optimal code clarity. This combination ensures your SQL queries are not just functional but also easy to understand at a glance.
Advanced SQL Techniques Leveraging Aliases: Enhancing Functionality
The use of SQL aliases can extend into advanced SQL techniques that enhance functionality. Here are a few scenarios where aliases shine.
Window Functions
When using window functions, aliases can be instrumental in providing clear output labels. For example:
SELECT e.first_name,
AVG(salary) OVER (PARTITION BY department_id) AS avg_department_salary
FROM employees AS e;
In this query, avg_department_salary
serves as a clear label for the average salary calculated per department.
Recursive Queries
Aliases are also useful in recursive queries, such as common table expressions (CTEs). Consider the following example:
WITH RECURSIVE employee_hierarchy AS (
SELECT id, first_name, manager_id
FROM employees
WHERE manager_id IS NULL
UNION ALL
SELECT e.id, e.first_name, e.manager_id
FROM employees AS e
JOIN employee_hierarchy AS eh ON e.manager_id = eh.id
)
SELECT * FROM employee_hierarchy;
In this example, employee_hierarchy
serves as an alias for the CTE, allowing us to reference it clearly throughout the query.
Pivot Tables and Cross-Tab Reports
Aliases can simplify the creation of pivot tables or cross-tab reports. For instance:
SELECT department_id,
SUM(CASE WHEN gender = 'M' THEN 1 ELSE 0 END) AS male_count,
SUM(CASE WHEN gender = 'F' THEN 1 ELSE 0 END) AS female_count
FROM employees
GROUP BY department_id;
In this query, male_count
and female_count
are aliases that make the output clear and understandable.
Query Performance Optimization
Using aliases can also optimize performance by allowing for more efficient indexing and query execution plans. For example:
SELECT e.first_name, e.salary
FROM employees AS e
WHERE e.salary > (SELECT AVG(salary) FROM employees);
In this scenario, using e
as an alias can help SQL engines optimize the query execution.
Security Considerations
When using aliases, be aware of potential security implications, particularly concerning SQL injection attacks. Always validate and sanitize user inputs to mitigate these risks.
Integrating SQL Aliases with Chat2DB: The Superior Choice for SQL Alias Management
Chat2DB (opens in a new tab) is an AI database visualization management tool that elevates the experience of working with SQL aliases. This tool provides an intuitive interface for managing SQL queries and visualizing data, making it easier for developers to efficiently use aliases.
AI-Enhanced Features of Chat2DB
One of the standout features of Chat2DB is its AI capabilities. The tool offers autocomplete suggestions for alias names, significantly speeding up the query writing process. This feature is particularly beneficial when working with complex queries involving multiple tables and aliases.
Improved Readability with Chat2DB
The query editor in Chat2DB enhances the readability and organization of complex queries with alias usage. With clear formatting and structure, developers can quickly grasp the intent of their SQL code, reducing the likelihood of errors.
Collaboration and Query Management in Chat2DB
Chat2DB also facilitates the saving and sharing of queries that utilize aliases, promoting collaboration among development teams. By allowing team members to access and modify queries efficiently, Chat2DB enhances productivity and code quality.
Compatibility Across Platforms
Chat2DB is designed to integrate seamlessly with various database systems, ensuring compatibility with alias syntax and functionalities across platforms. This flexibility allows developers to work comfortably in their preferred environments.
User Feedback on Chat2DB
Users have reported enhanced productivity and code quality through effective alias management with Chat2DB. The AI-driven features streamline the querying process, making it a natural choice for developers looking to improve their SQL workflow.
Feature | Chat2DB | Other Tools (e.g., DBeaver, MySQL Workbench, DataGrip) |
---|---|---|
AI Autocomplete | Yes | Limited |
Readability Enhancement | High | Moderate to Low |
Collaboration Tools | Excellent | Varies |
Cross-Platform Support | Extensive | Varies |
FAQ
-
What are SQL aliases? SQL aliases are temporary names for tables or columns in SQL queries, used to simplify complex queries and improve readability.
-
How do I create an SQL alias? You can create an alias using the
AS
keyword, or simply by specifying the new name after the original column or table name. For example:SELECT column_name AS alias_name FROM table_name;
. -
Do SQL aliases affect database performance? No, SQL aliases do not change the database schema or actual data; they are only temporary references within the query context.
-
What are some best practices for naming aliases? Use short, descriptive names for clarity, avoid reserved keywords, and maintain consistency across different queries or projects.
-
How can Chat2DB help with SQL alias management? Chat2DB offers AI-powered features such as autocomplete for alias names, enhancing the querying process's efficiency and readability.
By mastering SQL aliases and utilizing tools like Chat2DB (opens in a new tab), developers can streamline their SQL coding practices, making their queries cleaner and more efficient. Switch to Chat2DB today for an enhanced SQL development experience that leverages the power of AI to take your SQL skills to the next level!
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!