Mastering SQL Constraints: Ensuring Database Integrity and Reliability
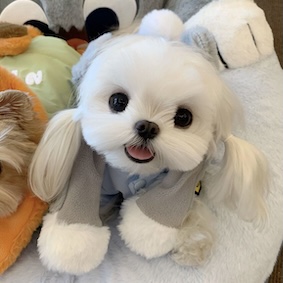
Introduction
SQL constraints play a vital role in maintaining the integrity of databases, which is crucial for developers working with relational databases. By enforcing rules at the database level, constraints prevent invalid data entry and ensure consistency across the database. Mastering these constraints is essential for robust database design, aiding in efficient query performance. In modern development environments, SQL constraints significantly impact data reliability.
Understanding SQL Constraints
SQL constraints are rules applied to columns in a database table to ensure the accuracy and reliability of data. The most common types of constraints include:
- Primary Key Constraints: Uniquely identify each record in a table.
- Foreign Key Constraints: Establish and enforce relationships between tables.
- Unique Constraints: Prevent duplicate entries in specified columns.
- Not Null Constraints: Ensure that a column cannot have a null value.
- Check Constraints: Enforce specific conditions on data entered into a table.
- Default Constraints: Assign default values to columns when no value is provided.
Each type of constraint serves a specific purpose and is crucial for different aspects of database design and integrity.
Primary Key Constraints
Primary keys are fundamental in relational databases for uniquely identifying records in a table. Characteristics of a primary key include uniqueness and non-nullability, preventing duplicate and null entries. Composite primary keys, which consist of multiple columns, are used when a single column does not suffice to uniquely identify records.
Code Example
CREATE TABLE Employees (
EmployeeID INT NOT NULL,
FirstName VARCHAR(100),
LastName VARCHAR(100),
PRIMARY KEY (EmployeeID)
);
Choosing appropriate primary keys is critical. Avoid using large texts or changing data as primary keys since this can lead to performance issues and data integrity problems.
Foreign Key Constraints
Foreign keys maintain relational integrity by enforcing parent-child relationships between tables. They uphold referential integrity, ensuring that relationships between tables remain consistent.
Code Example
CREATE TABLE Orders (
OrderID INT NOT NULL,
OrderDate DATE,
CustomerID INT,
PRIMARY KEY (OrderID),
FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID)
);
Foreign keys can also define cascade actions, such as ON DELETE CASCADE
, which automatically delete related records, preventing orphaned records and maintaining data consistency.
Unique and Not Null Constraints
Unique constraints ensure that all values in a column are different. They are similar to primary keys but allow for null values unless specified otherwise.
Code Example
CREATE TABLE Products (
ProductID INT NOT NULL,
ProductName VARCHAR(100) UNIQUE,
Price DECIMAL(10, 2) NOT NULL
);
Not null constraints guarantee that a column always contains a value, contributing to data completeness and application logic integrity.
Check Constraints
Check constraints enforce domain-specific rules by validating data based on predefined conditions. They are essential for maintaining data quality and preventing erroneous entries.
Code Example
CREATE TABLE Accounts (
AccountID INT NOT NULL,
Balance DECIMAL(10, 2),
CHECK (Balance >= 0)
);
While powerful, check constraints have limitations in complex validation scenarios. They should be implemented carefully to avoid hindering database performance.
Default Constraints
Default constraints streamline data entry processes by automatically assigning values to columns when no explicit value is provided, reducing the occurrence of null values.
Code Example
CREATE TABLE Orders (
OrderID INT NOT NULL,
OrderDate DATE DEFAULT GETDATE(),
Status VARCHAR(20) DEFAULT 'Pending'
);
Default constraints enhance data consistency and minimize manual data entry errors, playing a crucial role in application logic and data processing.
Advanced Constraint Management
Managing SQL constraints in complex databases requires advanced techniques. Temporarily disabling constraints for bulk data operations can be beneficial, provided data integrity is not compromised. Altering constraints efficiently in evolving database schemas is also critical.
Code Example for Temporarily Disabling Constraints
ALTER TABLE Orders NOCHECK CONSTRAINT ALL;
-- Perform bulk operations
ALTER TABLE Orders CHECK CONSTRAINT ALL;
Database management tools like Chat2DB offer features for constraint visualization and optimization, aiding in effective constraint management.
Encouragement for Further Learning
Understanding and mastering SQL constraints is vital for developers aiming to ensure database integrity and reliability. Well-designed constraints prevent data anomalies and enhance application performance. Developers are encouraged to leverage tools like Chat2DB for efficient constraint management and optimization, ensuring data accuracy and consistency in modern data-driven applications.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!