SQL Joins Explained: A Comprehensive Guide to Understanding and Applying Joins
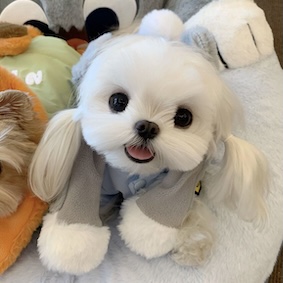
Introduction
Mastering SQL joins is essential for developers working with relational databases. A 'join' in SQL is a powerful tool that allows you to combine rows from two or more tables based on a related column. Understanding the different types of joins can significantly optimize your database queries, making your applications more efficient.
In this guide, we will explore the various types of SQL joins, their applications, and best practices. We will also highlight how tools like Chat2DB can simplify database interactions for developers, making it easier to manage complex queries.
Understanding SQL Joins
SQL joins are fundamental in database management as they facilitate querying data from multiple tables. When dealing with relational databases, it is common to retrieve data that spans across several tables. This is where joins come into play.
Primary Keys and Foreign Keys
To understand joins, you need to know about primary keys and foreign keys. A primary key is a unique identifier for a record in a table, while a foreign key is a field in one table that links to the primary key of another. These relationships are crucial for performing joins, as they define how the tables are related.
Joins play a vital role in ensuring data integrity and reducing redundancy. By using joins, you can avoid storing the same information in multiple places. This allows for cleaner, more efficient databases.
Types of SQL Joins
This guide will cover several types of SQL joins, including:
- Inner Joins
- Left Joins
- Right Joins
- Full Joins
- Self Joins
- Cross Joins
We will discuss each type in detail, including their syntax and practical applications.
Cartesian Product
Before diving into the types of joins, it's important to mention the Cartesian product. A Cartesian product occurs when you join two tables without any conditions, resulting in every possible combination of rows from both tables. While this can be useful in specific scenarios, it often leads to large datasets and should be used with caution.
Inner Joins
Inner joins are one of the most commonly used types of joins in SQL. They return rows when there is a match in both tables.
Syntax
The basic syntax for an inner join is as follows:
SELECT columns
FROM table1
INNER JOIN table2
ON table1.common_column = table2.common_column;
Example
Consider two tables: Customers
and Orders
. Each order is linked to a customer through a CustomerID
. Here’s how you would retrieve all customers and their orders:
SELECT Customers.CustomerName, Orders.OrderID
FROM Customers
INNER JOIN Orders
ON Customers.CustomerID = Orders.CustomerID;
Performance Optimization
To optimize inner joins for performance, ensure that you have appropriate indexes on the columns used in the join condition. This can significantly speed up query execution.
Common Pitfalls
One common pitfall when using inner joins is forgetting to include the join condition, which can lead to a Cartesian product. Always specify how the tables are related to avoid this issue.
Use Case
Inner joins are particularly beneficial when you need to retrieve related data from multiple tables, such as fetching user details along with their purchase history.
Left Joins
Left joins, also known as left outer joins, return all rows from the left table and the matched rows from the right table. If there is no match, NULL values are returned for the right table.
Syntax
The syntax for a left join is:
SELECT columns
FROM table1
LEFT JOIN table2
ON table1.common_column = table2.common_column;
Example
Using the same Customers
and Orders
tables, if you want to get a list of all customers and their orders (if any), you would write:
SELECT Customers.CustomerName, Orders.OrderID
FROM Customers
LEFT JOIN Orders
ON Customers.CustomerID = Orders.CustomerID;
Finding Unmatched Records
Left joins are useful for finding unmatched records in the right table. For instance, you could identify customers who have not placed any orders by checking for NULL values in the OrderID
field.
Performance Considerations
Left joins can be less efficient than inner joins if the right table is large, as they still need to return all rows from the left table. Proper indexing can help mitigate performance issues.
Real-World Example
A scenario where left joins are advantageous is in reporting applications where you want to show all entities (e.g., users) regardless of whether they have related records (e.g., transactions).
Right Joins
Right joins, or right outer joins, are similar to left joins but return all rows from the right table and matched rows from the left table.
Syntax
The syntax for a right join is:
SELECT columns
FROM table1
RIGHT JOIN table2
ON table1.common_column = table2.common_column;
Example
Assuming you want to get a list of all orders and the corresponding customer details, you could use:
SELECT Customers.CustomerName, Orders.OrderID
FROM Customers
RIGHT JOIN Orders
ON Customers.CustomerID = Orders.CustomerID;
Scenarios for Right Joins
Right joins are preferable when the right table is the primary focus of your query, such as when you need to list all orders regardless of whether they have associated customers.
Performance Issues
Right joins can lead to performance issues similar to left joins, especially if the left table is large. Indexing the join columns is key to improving performance.
Use Case
A useful application of right joins is in reporting systems where you want to display all transactions, even if some transactions do not have associated customers (due to data integrity issues).
Full Joins
Full joins, or full outer joins, combine the results of both left and right joins. They return all records when there is a match in either table.
Syntax
The syntax for a full join is:
SELECT columns
FROM table1
FULL OUTER JOIN table2
ON table1.common_column = table2.common_column;
Example
To retrieve all customers and all orders, regardless of whether there is a match, you would write:
SELECT Customers.CustomerName, Orders.OrderID
FROM Customers
FULL OUTER JOIN Orders
ON Customers.CustomerID = Orders.CustomerID;
Complete Data Sets
Full joins are particularly useful when you need to obtain complete datasets from multiple tables, ensuring that you do not miss any records.
Performance Implications
Full joins can be performance-heavy as they require the database to combine and return all records from both tables. Proper indexing can help mitigate these costs.
Scenario for Full Joins
A practical scenario for using full joins is when performing data analysis across multiple tables where you want to ensure that all potential records are included, such as in a comprehensive customer and orders report.
Self Joins
Self joins are a unique type of join that allows you to join a table with itself. This is useful for comparing rows within the same table.
Syntax
The syntax for a self join is:
SELECT a.columns, b.columns
FROM table a, table b
WHERE a.common_column = b.common_column;
Example
Consider an employee table, where each employee has a manager who is also listed in the same table. You can retrieve a list of employees along with their managers using a self join:
SELECT e.EmployeeName AS Employee, m.EmployeeName AS Manager
FROM Employees e
INNER JOIN Employees m ON e.ManagerID = m.EmployeeID;
Hierarchical Data Structures
Self joins are particularly useful for hierarchical data structures, where you need to represent relationships within the same entity.
Challenges and Best Practices
When implementing self joins, ensure that your aliases (like e
and m
in the example) are used clearly to avoid confusion. Performance can be a concern, so indexing the join columns is vital for efficiency.
Real-World Example
Self joins can be applied in organizational charts where you want to display the hierarchy of employees and their respective managers.
Cross Joins
Cross joins produce a Cartesian product of the two tables. This means that every row from the first table is combined with every row from the second table.
Syntax
The syntax for a cross join is:
SELECT columns
FROM table1
CROSS JOIN table2;
Example
If you have a Products
table and a Categories
table, and you want to generate a list of all possible combinations of products and categories, you could use:
SELECT Products.ProductName, Categories.CategoryName
FROM Products
CROSS JOIN Categories;
Intentional Use Cases
Cross joins are rarely used but can be useful in specific scenarios, such as generating all possible combinations of two datasets, like pairing products with categories for marketing campaigns.
Performance Impact and Pitfalls
Cross joins can lead to very large result sets, especially if both tables contain numerous rows. Be cautious and ensure that a cross join is necessary before using it.
Case Where Cross Joins are Beneficial
A practical case for using cross joins could be in a scenario where you need to create a comprehensive list of combinations for an A/B testing framework.
Best Practices and Optimization
To optimize SQL joins for better performance, consider the following strategies:
-
Choose the Right Join Type: Select the appropriate join type based on your query's requirements to improve efficiency.
-
Use Indexing: Implement indexing on the columns involved in join conditions to speed up query execution.
-
Write Clean Queries: Structure your join queries clearly and concisely to enhance readability and maintainability.
-
Avoid Unnecessary Joins: Only join tables that are necessary for your query to avoid performance degradation.
-
Use Tools for Complex Queries: Leverage tools like Chat2DB to simplify complex join queries and enhance your productivity.
Optimized Join Queries
Here’s an example of an optimized join query using indexing:
CREATE INDEX idx_customer_id ON Orders(CustomerID);
SELECT Customers.CustomerName, COUNT(Orders.OrderID) AS OrderCount
FROM Customers
LEFT JOIN Orders ON Customers.CustomerID = Orders.CustomerID
GROUP BY Customers.CustomerName;
This query retrieves the number of orders per customer while utilizing an index to improve performance.
Further Learning
Understanding SQL joins is crucial for effective database management. Mastering these concepts will enhance your ability to work with relational databases and develop efficient applications.
For developers looking to streamline their database interactions, consider using Chat2DB. This tool simplifies complex queries and helps you manage your databases more effectively, allowing you to focus on building robust applications.
By practicing and experimenting with various join types, you will deepen your understanding and improve your skills in SQL.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!