Advanced SQL Techniques for Data Aggregation and Grouping
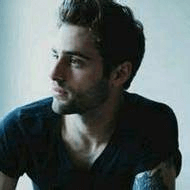
Introduction
Structured Query Language (SQL) is a core tool for data management and analysis, mainly used for data collection, modification and query. Mastering complex SQL aggregation techniques allows you to perform complex data analysis more effectively, although data extraction and processing still rely on basic SQL commands. This article will explore complex SQL aggregation techniques in depth, providing detailed explanations and code examples.
1-SQL Aggregation Overview
SQL aggregation is an important component of database query and analysis, which enables users to extract and compress information from large data sets. The essence of aggregation is to combine multiple rows of data into a single result. To achieve this, SQL provides a series of aggregate functions that are used to calculate the value of the entire data set or grouping of rows. These functions facilitate analysis and decision-making by integrating data in a relevant way.
The main components of SQL aggregation include:
- Aggregate functions: SQL provides some built-in aggregate functions for performing specific calculations on data. They mainly include:
- COUNT: Calculates the number of rows in the result set.
- SUM: Calculates the sum of the values in the result set.
- AVG: Calculates the average of the values in the result set.
- MIN: Finds the minimum value in the result set.
- MAX: Identifies the maximum value in the result set.
These functions apply to columns of various data types, including numeric, text, and time data, providing flexibility for aggregation tasks.
- GROUP BY clause: The GROUP BY clause is used to divide a data set into different groups based on the values of the specified columns. When used in conjunction with aggregate functions, it allows users to perform calculations within each group, thereby generating summary statistics and group-level analysis.
- HAVING clause: The HAVING clause is used to filter group-level results based on specific conditions. Unlike filtering individual rows through the WHERE clause before aggregation, the HAVING clause processes the data after aggregation, enabling users to specify which groups should be included or excluded from the final result.
- Window functions: Window functions provide a way to perform calculations on a set of rows related to the current row. Unlike traditional aggregate functions, window functions do not combine rows into a single result, but instead calculate values based on a defined window or frame. Common window functions include ROW_NUMBER, RANK, and SUM with an OVER clause.
- Advanced aggregation techniques: SQL also provides advanced aggregation techniques such as CUBE, ROLLUP, and GROUPING SETS, which allow multiple levels of grouping to be generated in a single query. These features are particularly useful in generating subtotal and total rows in reports, providing a comprehensive summary of the data.
Overall, SQL aggregation is a powerful tool for summarizing and analyzing a variety of complex data sets. By leveraging aggregate functions, grouping, filtering, window functions, and advanced aggregation techniques, users can gain valuable insights and make informed decisions based on them.
2-Advanced SQL Aggregate Functions
Advanced SQL aggregate functions go beyond the basic COUNT, SUM, AVG, MIN, and MAX to provide more complex analytical capabilities. These functions are particularly useful when performing statistical analysis, handling NULL values, or calculating specific percentiles. Next, we will explore the application of some advanced aggregate functions in depth.
- STDDEV() and STDDEV_POP(): These functions calculate the standard deviation of a set of values. STDDEV() calculates the sample standard deviation, while STDDEV_POP() calculates the population standard deviation.
SELECT
STDDEV(order_amount) AS sample_std_dev,
STDDEV_POP(order_amount) AS population_std_dev
FROM orders_data;
- VARIANCE() and VAR_POP(): Similar to standard deviation, VARIANCE() calculates sample variance, while VAR_POP() calculates population variance.
SELECT
VARIANCE(order_total) AS sample_variance,
VAR_POP(order_total) AS population_variance
FROM orders_data;
- MEDIAN(): The MEDIAN() function calculates the median (middle) of a set of values. This function is particularly useful for analyzing the distribution of data
SELECT MEDIAN(order_amount) AS median_order
FROM orders_data;
- PERCENTILE_CONT() and PERCENTILE_DISC(): These functions calculate percentiles for a set of values. PERCENTILE_CONT() calculates continuous percentiles, while PERCENTILE_DISC() calculates discrete percentiles.
SELECT
PERCENTILE_CONT(0.5) WITHIN GROUP (ORDER BY order_amount) AS median_order,
PERCENTILE_DISC(0.75) WITHIN GROUP (ORDER BY order_amount) AS third_quartile_order
FROM orders_data;
- COVAR_POP() and COVAR_SAMP(): These functions calculate the population covariance and sample covariance, respectively, between two sets of values.
SELECT COVAR_POP(revenue, cost) AS population_covariance,
COVAR_SAMP(revenue, cost) AS sample_covariance
FROM financial_data;
- CORR(): The CORR() function calculates the correlation coefficient between two sets of values, indicating the strength and direction of their linear relationship.
SELECT CORR(revenue, advertising_budget) AS correlation_coefficient
FROM financial_records;
- BIT_AND() and BIT_OR(): These functions perform bitwise AND and OR operations, respectively, on a set of binary values.
SELECT BIT_AND(status_flag) AS bitwise_and_result,
BIT_OR(status_flag) AS bitwise_or_result
FROM status_records;
- **JSON_ARRAYAGG() and JSON_OBJECTAGG():**These functions aggregate JSON values into a JSON array or object, respectively.
SELECT JSON_ARRAYAGG(course_name) AS course_names,
JSON_OBJECTAGG(course_id, enrollment_count) AS enrollments_by_course
FROM courses_data
GROUP BY department_id;
These advanced SQL aggregate functions enable users to perform complex analysis and gain insight into data for informed decision making and strategic planning.
3- Grouping Data Using GROUP BY
The SQL GROUP BY
clause is a powerful tool that aggregates information and simplifies results by grouping data based on common values in one or more columns. By applying aggregate functions on each group of rows with the same value, users can easily calculate summary statistics and generate valuable reports. Here is a simple example to demonstrate the use of the GROUP BY
clause:
Basic GROUP BY
Example:
Suppose we have a table called sales_data that records information about sales transactions, including Product_id, category_id, and sales_amount. We want to calculate the total sales for each product category.
SELECT category_id, SUM(sales_amount) AS total_sales
FROM sales_data
GROUP BY category_id;
In this example, the GROUP BY clause groups the rows by the category_id column. The SUM() function then calculates the total sales for each category.
Grouping by multiple columns:
We can also group data by multiple columns to create more granular summaries. For example, we might want to calculate the total sales for each product category within each month.
SELECT category_id, DATE_TRUNC('month', order_date) AS month, SUM(sales_amount) AS total_sales
FROM sales_data
GROUP BY category_id, DATE_TRUNC('month', order_date);
Here, we use both category_id and order_date columns in the GROUP BY clause to group the data by category and month.
Filtering grouped data using HAVING:
The HAVING clause can be used to filter groups based on an aggregate condition. For example, suppose we want to find the categories with total sales exceeding $10,000.
SELECT category_id, SUM(sales_amount) AS total_sales
FROM sales_data
GROUP BY category_id
HAVING SUM(sales_amount) > 10000;
The HAVING clause filters the grouped results, ensuring that only categories with total sales greater than $10,000 are included in the final output.
Using Aggregate Functions with GROUP BY:
Aggregate functions such as COUNT, AVG, MIN, and MAX can be combined with the GROUP BY clause to calculate various summary statistics for each group. For example, we can find the number of sales transactions and the average sales amount for each product category.
SELECT category_id, COUNT(*) AS num_transactions, AVG(sales_amount) AS avg_sales
FROM sales_data
GROUP BY category_id;
Here, we use the COUNT(*) function to count the number of transactions and the AVG(sales_amount) function to calculate the average sales for each category.
Grouping with expressions:
You can also group data using expressions or functions. For example, we may want to group sales transactions by year in addition to category.
SELECT category_id, DATE_PART('year', order_date) AS year, SUM(sales_amount) AS total_sales
FROM sales_data
GROUP BY category_id, DATE_PART('year', order_date);
In this query, we leverage the DATE_PART
function to extract the year from the order_date column and group the data based on category and year.
The SQL GROUP BY
clause is a flexible tool that aggregates and summarizes data based on common values in one or more columns. By using GROUP BY
, users are able to calculate summary statistics, generate meaningful reports, and gain insights from data sets. Combining aggregate functions, filters, and expressions, users can perform complex analysis to extract valuable information from data.
4- Using HAVING to filter grouped data
By using the SQL HAVING
clause, users can effectively filter grouped data based on specific conditions. The HAVING clause allows for more precise control over the aggregated results, while the WHERE
clause filters rows before grouping. Here is an example to demonstrate the use of the HAVING
clause:
Basic HAVING example:
Suppose we have a table called sales_data that records information about sales transactions, including Product_id, category_id, and sales_amount. Our goal is to find the product categories with total sales exceeding $12,000.
SELECT category_id, SUM(sales_amount) AS total_sales
FROM sales_data
GROUP BY category_id
HAVING SUM(sales_amount) > 12000;
In this example, the HAVING clause filters the grouped results, ensuring that only categories with total sales greater than $10,000 are included in the final output.
HAVING with aggregate functions:
The HAVING clause can be used with aggregate functions to filter groups based on an aggregate condition. For example, suppose we want to find categories with average sales greater than $400.
SELECT category_id, AVG(sales_amount) AS avg_sales
FROM sales_data
GROUP BY category_id
HAVING AVG(sales_amount) > 400;
Here, the HAVING clause filters the grouped results, ensuring that only categories with average sales greater than $500 are included in the final output.
Combining HAVING with WHERE:
You can also use both the WHERE and HAVING clauses in the same query to filter rows before grouping and aggregate the results after grouping. For example, let's find categories with total sales greater than $10,000 and average sales greater than $800.
SELECT category_id, SUM(sales_amount) AS total_sales, AVG(sales_amount) AS avg_sales
FROM sales_data
WHERE sales_date BETWEEN '2024-10-01' AND '2024-12-31'
GROUP BY category_id
HAVING SUM(sales_amount) > 10000 AND AVG(sales_amount) > 800;
In this query, the WHERE clause filters rows based on the sales date, while the HAVING clause filters the aggregate results based on the total sales and average sales conditions.
5-Window Functions for Advanced Aggregation
Window functions in SQL provide a powerful tool for performing complex aggregation and analysis tasks without combining multiple rows into a single result. These functions operate on a set of rows associated with the current row (called a window or frame) and can be used to generate calculations such as ranking, moving average, cumulative sum, etc. Here are some examples to illustrate how window functions work:
Basic Syntax:
The basic syntax of a window function consists of the function itself, followed by the OVER
clause, which is used to define the window specification. The window specification dictates how the rows are partitioned and ordered within the window frame.
SELECT employee_id, department_id,
ROW_NUMBER() OVER (PARTITION BY department_id ORDER BY salary DESC) AS salary_rank
FROM employees;
6- Advanced aggregation using CUBE, ROLLUP, and GROUPING SETS
Advanced aggregation techniques such as CUBE
, ROLLUP
, and GROUPING SETS
enhance the power of the GROUP BY
clause in SQL, enabling users to generate multiple levels of grouping and subtotal rows in a single query. These extensions are particularly useful in creating comprehensive summaries and reports. Below is an example description of each technique:
- CUBE:
The CUBE
extension generates all possible grouping combinations, including subtotal and grand total rows. It provides a comprehensive view of the data by considering all dimensions.
SELECT department, gender, COUNT(*) AS num_employees
FROM employees
GROUP BY CUBE (department, gender);
In this example, the CUBE(department, gender) clause generates subtotals for each department, gender, and their combination, as well as a grand total.
- Rollup:
The ROLLUP
extension generates subtotal rows for each grouping level, from the most detailed level to the grand total.
SELECT department, gender, COUNT(*) AS num_employees
FROM employees
GROUP BY ROLLUP (department, gender);
Here, the ROLLUP(department, sex)
clause generates subtotals for each department, sex, and total, but it does not include all combinations like CUBE
.
- Grouping Sets:
The GROUPING SETS
extension allows users to explicitly specify multiple grouping sets, providing the flexibility to define custom grouping levels.
SELECT department, gender, COUNT(*) AS num_employees
FROM employees
GROUP BY GROUPING SETS ((department), (gender), (department, gender));
In this example, we explicitly specify three grouping sets: department, gender, and a combination of department and gender. This provides finer control over the grouping level than CUBE and ROLLUP.
Use Cases:
CUBE
,ROLLUP
, andGROUPING SETS
are very effective in generating comprehensive summaries and reports with subtotals and grand totals.- These techniques can be applied to a variety of scenarios such as financial reporting, sales analysis, and business intelligence.
With the powerful extension capabilities of SQL, users can implement multiple levels of grouping and subtotals in a single query. By leveraging these complex aggregation methods, users can generate detailed reports and summaries to gain in-depth insights into the data. Whether analyzing sales data, financial transactions, or customer information, CUBE
, ROLLUP
, and GROUPING SETS
provide flexible and efficient solutions.
These advanced SQL aggregation methods provide powerful support for analyzing and summarizing data across multiple dimensions. By applying these techniques to real-world use cases such as sales analysis, financial reporting, customer segmentation, employee performance evaluation, and inventory management, organizations can gain valuable insights and make data-driven decisions to optimize business processes and improve efficiency and profitability.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!