Understanding SQL Schema: A Beginner's Guide to Database Structure
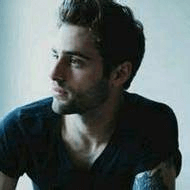
In the realm of database management, understanding SQL schema is crucial for anyone looking to design, implement, or manage databases effectively. A schema serves as a blueprint for database architecture, guiding the logical configuration of data. It encompasses various database objects such as tables, views, indexes, and procedures, facilitating data integrity, security, and efficient data retrieval. This article delves deeply into what a schema in SQL is, exploring its components, best practices for design, management techniques, security considerations, and its application in software development. We will also introduce Chat2DB, an AI-driven database management tool that enhances the efficiency and intelligence of database operations.
The Role of SQL Schema in Database Design
The SQL schema plays a pivotal role in structuring and organizing databases. It serves as the foundation upon which all database objects are built. The schema defines the logical framework of the database, providing clarity and consistency across development teams. Key components of a schema include:
Component | Description |
---|---|
Tables | The primary building blocks of a schema, where data is stored. |
Columns | Define the attributes of data stored in tables. |
Primary Keys | Uniquely identify records in a table. |
Foreign Keys | Establish relationships between tables and ensure referential integrity. |
Constraints | Enforce data rules and standards, such as unique and check constraints. |
Indexes | Optimize query performance and speed up data access. |
Schema and Data Integrity
The design of a schema directly impacts data integrity. By carefully defining relationships through primary and foreign keys, a schema prevents the occurrence of orphaned records and maintains data relationships. The use of constraints further ensures that only valid data is entered into the database. For example, consider the following SQL code that creates a simple schema for a library system:
CREATE TABLE Authors (
AuthorID INT PRIMARY KEY,
Name VARCHAR(100) NOT NULL,
BirthDate DATE
);
CREATE TABLE Books (
BookID INT PRIMARY KEY,
Title VARCHAR(100) NOT NULL,
AuthorID INT,
FOREIGN KEY (AuthorID) REFERENCES Authors(AuthorID)
);
In this case, the Authors table is linked to the Books table through the AuthorID foreign key, ensuring that each book must be associated with a valid author.
Components of a SQL Schema
Understanding the components of a SQL schema is essential for effective database design. Here are the key elements that comprise a schema:
Tables
Tables are the core component of a schema. They store data in rows and columns. Each column has a defined data type, which specifies the kind of data that can be stored. For instance, a column can be defined as an integer, string, date, or boolean.
Data Types and Constraints
When defining a table, it is important to choose appropriate data types and constraints for each column. Here’s an example of how to create a table with different data types and constraints:
CREATE TABLE Employees (
EmployeeID INT PRIMARY KEY,
FirstName VARCHAR(50) NOT NULL,
LastName VARCHAR(50) NOT NULL,
HireDate DATE DEFAULT CURRENT_DATE,
Salary DECIMAL(10, 2) CHECK (Salary > 0)
);
Indexes
Indexes are special database objects that improve the speed of data retrieval operations on a table at the cost of additional space and slower writes. Here’s how to create an index:
CREATE INDEX idx_lastname ON Employees (LastName);
This index will optimize queries that search for employees by their last name.
Views
A view is a virtual table that provides a way to present data from one or more tables. Views can simplify complex queries and provide a level of security by restricting access to specific data. For example:
CREATE VIEW EmployeeSalaries AS
SELECT FirstName, LastName, Salary
FROM Employees
WHERE Salary > 50000;
Stored Procedures and Functions
Stored procedures and functions are programmable elements that enhance the functionality of a schema. They allow for reusable SQL code that can be executed with specific parameters. Here’s an example of a stored procedure that retrieves employees by salary range:
CREATE PROCEDURE GetEmployeesBySalaryRange
@MinSalary DECIMAL(10, 2),
@MaxSalary DECIMAL(10, 2)
AS
BEGIN
SELECT * FROM Employees
WHERE Salary BETWEEN @MinSalary AND @MaxSalary;
END;
Schema Design Best Practices
Designing an effective SQL schema requires adherence to best practices that ensure clarity, simplicity, and scalability. Here are some key considerations:
Clarity and Simplicity
A well-designed schema should be clear and simple, making it easy for developers and database administrators to understand and maintain. Avoid unnecessary complexity by using straightforward naming conventions and limiting the number of relationships where possible.
Normalization
Normalization is the process of organizing data to reduce redundancy and improve data integrity. This typically involves dividing large tables into smaller ones and defining relationships between them. However, it is also important to balance normalization with performance needs. For instance, denormalization may be beneficial in read-heavy applications.
Indexing Strategies
Proper indexing can significantly enhance query performance. Analyze the queries that are run most frequently and create indexes accordingly. However, avoid over-indexing, as this can slow down write operations.
Managing and Modifying SQL Schemas
As business requirements evolve, schemas must be managed and modified accordingly. Here are strategies for effective schema management:
Schema Migration and Versioning
Schema migration involves tracking and implementing changes to schemas systematically. Tools like Chat2DB facilitate this process, allowing users to visually manage schema changes and maintain version control.
Testing Schema Changes
Before applying schema changes, it is crucial to test them in a staging environment. This helps identify potential issues and ensures that existing functionality is not disrupted.
Backup and Recovery Plans
Always have backup and recovery plans in place to safeguard schema integrity. Regularly back up your database and ensure that restoration procedures are documented and tested.
Auditing Schema Changes
Maintain a comprehensive change history by auditing schema changes. This provides accountability and helps track modifications over time.
Security and Permissions in SQL Schemas
Security is a critical aspect of database management. Schemas play a vital role in managing permissions and access controls. Here are some key considerations:
Segregation of Data
Schemas allow for the segregation of data within a database. This can enhance security by limiting access to sensitive information based on user roles and privileges.
Implementing Least Privilege
Adopt the principle of least privilege, granting users only the access necessary to perform their duties. This minimizes security risks and helps protect sensitive data.
Auditing and Monitoring Access
Implement auditing and monitoring strategies to detect and prevent unauthorized access to schema objects. Regularly review access logs and maintain a proactive stance towards security.
Leveraging SQL Schemas in Application Development
SQL schemas are integral to application development. They provide a structured foundation for data models and queries, facilitating the integration of databases with applications. Here’s how schemas contribute to application development:
ORM Frameworks
Object-Relational Mapping (ORM) frameworks bridge the gap between application objects and database schemas. They simplify data manipulation and enhance productivity by allowing developers to work with objects instead of raw SQL queries.
Supporting Multi-Tenancy
Schemas can support multi-tenancy applications by logically separating data for different tenants. This ensures data privacy and security while allowing for efficient resource utilization.
Optimizing Application Performance
Efficient schema design and query tuning can dramatically improve application performance. Regularly analyze query performance and adjust index strategies as needed.
Continuous Integration and Deployment
Integrating schema migrations into CI/CD pipelines helps align application updates with database changes. This ensures that the application and database remain synchronized throughout the development lifecycle.
FAQ
-
What is a SQL schema? A SQL schema is a blueprint that defines the structure of a database, including tables, columns, data types, and relationships.
-
Why is schema design important? Schema design is crucial for maintaining data integrity, optimizing performance, and ensuring that the database can adapt to evolving business needs.
-
How do I create a schema in SQL? You can create a schema using the
CREATE SCHEMA
statement followed by defining tables, relationships, and other objects within that schema. -
What are the best practices for schema design? Best practices include maintaining clarity and simplicity, normalizing data, implementing effective indexing strategies, and documenting changes.
-
How can Chat2DB assist with SQL schema management? Chat2DB is an AI-driven database management tool that enhances the efficiency of schema management by providing visual tools for schema modifications, migrations, and documentation. Its intelligent features, such as natural language processing for SQL generation and optimized query suggestions, set it apart from traditional tools like DBeaver, MySQL Workbench, and DataGrip.
By leveraging the insights presented in this article, along with tools like Chat2DB, you can enhance your understanding of what a schema in SQL is and improve your database management capabilities. Embrace these practices to ensure a robust and efficient database design that meets your organization's needs.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!