Using Python to Automate the Process of Querying Database Schema
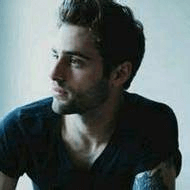
Introduction
In the realm of database management, the ability to efficiently query and analyze the database schema is crucial for maintaining data integrity and optimizing performance. Manual querying of database schema can be time-consuming and error-prone, especially in large-scale databases. This article delves into the utilization of Python to automate the process of querying database schema, offering a streamlined and effective solution for database administrators and developers.
Core Concepts and Background Information
Before delving into the automation process, it is essential to understand the key concepts related to database schema querying. The database schema represents the structure of the database, including tables, columns, relationships, and constraints. By querying the database schema, users can retrieve metadata about the database objects, enabling better understanding and management of the database.
Python, a versatile and powerful programming language, provides a wide range of libraries and tools that facilitate database interaction. Libraries such as SQLAlchemy, psycopg2, and pyodbc offer robust capabilities for querying database schema and executing SQL commands.
Practical Strategies and Solutions
Automating Database Schema Queries with Python
Python scripts can be used to automate the process of querying database schema, eliminating the need for manual intervention. By leveraging Python's database connectivity libraries, developers can establish connections to databases and execute SQL queries to retrieve schema information.
import psycopg2
# Establish a connection to the database
conn = psycopg2.connect(
dbname='database_name',
user='username',
password='password',
host='localhost'
)
# Create a cursor object
cur = conn.cursor()
# Execute a query to retrieve table names
cur.execute("SELECT table_name FROM information_schema.tables WHERE table_schema = 'public'")
# Fetch the results
tables = cur.fetchall()
# Print the table names
for table in tables:
print(table[0])
# Close the cursor and connection
cur.close()
conn.close()
Benefits of Automation
Automating the querying of database schema with Python offers several benefits, including:
- Efficiency: Automation reduces the time and effort required to retrieve schema information.
- Accuracy: Automated scripts ensure consistency and accuracy in querying database schema.
- Scalability: Python scripts can be easily scaled to handle large databases and complex queries.
Case Studies and Practical Examples
Case Study: Automating Schema Analysis
In a large e-commerce database, the database administrator needs to analyze the schema to identify redundant columns and optimize table structures. By using Python scripts, the administrator automates the process of querying the database schema and generates reports on schema analysis.
Practical Example: Querying Table Columns
import psycopg2
# Establish a connection to the database
conn = psycopg2.connect(
dbname='database_name',
user='username',
password='password',
host='localhost'
)
# Create a cursor object
cur = conn.cursor()
# Execute a query to retrieve column names of a specific table
cur.execute("SELECT column_name FROM information_schema.columns WHERE table_name = 'table_name'")
# Fetch the results
columns = cur.fetchall()
# Print the column names
for column in columns:
print(column[0])
# Close the cursor and connection
cur.close()
conn.close()
Conclusion
Automating the process of querying database schema using Python enhances efficiency, accuracy, and scalability in database management. By leveraging Python's capabilities, database administrators and developers can streamline the retrieval of schema information and optimize database performance. Embracing automation in database schema querying is a strategic approach to enhance productivity and data integrity.
FAQ
Q: Can Python be used to query different types of databases?
A: Yes, Python's database connectivity libraries support a wide range of databases, including MySQL, PostgreSQL, SQLite, and more. By installing the appropriate database drivers, Python can interact with various database systems.
Q: How can I schedule automated schema queries in Python?
A: You can use scheduling tools like cron jobs or Windows Task Scheduler to run Python scripts at specified intervals. By setting up scheduled tasks, you can automate the querying of database schema without manual intervention.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!