Comprehensive Guide: Understanding and Using SQL Server Native Client Effectively
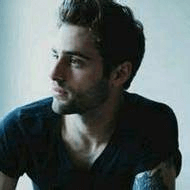
Understanding SQL Server Native Client
SQL Server Native Client is an essential data access technology that merges the functionalities of the SQL OLE DB provider and the SQL ODBC driver into a unified dynamic-link library (DLL). This integration allows developers to connect to and communicate with SQL Server databases efficiently.
The SQL Server Native Client acts as a bridge between applications and SQL Server, offering native data access to features such as new data types, enhanced performance, and secure connectivity. Its compatibility with various programming environments, including .NET applications, makes it a versatile choice for developers.
Unlike other connectivity tools, SQL Server Native Client is specifically designed for SQL Server, ensuring optimized performance. By utilizing SQL Server Native Client, developers can take advantage of the latest SQL Server features without compromising on speed or security.
Installation and Configuration of SQL Server Native Client
Installing and configuring SQL Server Native Client is a straightforward process. Follow these steps to get started:
Step | Description |
---|---|
1. Download the Appropriate Version | Visit the Microsoft Download Center (opens in a new tab) to download the version compatible with your system architecture and SQL Server version. |
2. Installation Process | Run the installer and follow the prompts. Ensure that you have administrative privileges on your machine and verify that your system meets the necessary requirements. |
3. Post-Installation Configuration | Set environment variables as needed. Configure connection strings for optimal performance. An example connection string for SQL Server Native Client is shown below: Provider=SQLNCLI11;Server=myServerAddress;Database=myDataBase;Uid=myUsername;Pwd=myPassword; |
4. Troubleshooting Common Issues | If you encounter issues such as missing dependencies or version conflicts, check the installation logs for details. Keeping the SQL Server Native Client updated is important to leverage the latest features and security patches. |
Utilizing ODBC and OLE DB with SQL Server Native Client
Developers can effectively use both ODBC (Open Database Connectivity) and OLE DB (Object Linking and Embedding, Database) interfaces with SQL Server Native Client.
Configuring ODBC Data Sources
To create and manage ODBC data sources, follow these steps:
- Open the ODBC Data Source Administrator on your system.
- Choose between User DSN or System DSN based on your needs.
- Click on "Add" and select SQL Server Native Client as the driver.
- Fill in the required information, including Data Source Name (DSN), server, and authentication details.
Utilizing OLE DB Connection Strings
OLE DB connection strings can be configured similarly. Here’s an example:
Provider=SQLNCLI11;Data Source=myServerAddress;Initial Catalog=myDataBase;Integrated Security=SSPI;
Coding Practices
The following code examples demonstrate how to establish connections, execute queries, and handle transactions using both interfaces:
Using ODBC
using System;
using System.Data.Odbc;
class Program
{
static void Main()
{
string connectionString = "Driver={SQL Server Native Client 11.0};Server=myServerAddress;Database=myDataBase;Uid=myUsername;Pwd=myPassword;";
using (OdbcConnection connection = new OdbcConnection(connectionString))
{
connection.Open();
OdbcCommand command = new OdbcCommand("SELECT * FROM myTable", connection);
OdbcDataReader reader = command.ExecuteReader();
while (reader.Read())
{
Console.WriteLine(reader["ColumnName"]);
}
}
}
}
Using OLE DB
using System;
using System.Data.OleDb;
class Program
{
static void Main()
{
string connectionString = "Provider=SQLNCLI11;Data Source=myServerAddress;Initial Catalog=myDataBase;Integrated Security=SSPI;";
using (OleDbConnection connection = new OleDbConnection(connectionString))
{
connection.Open();
OleDbCommand command = new OleDbCommand("SELECT * FROM myTable", connection);
OleDbDataReader reader = command.ExecuteReader();
while (reader.Read())
{
Console.WriteLine(reader["ColumnName"]);
}
}
}
}
Performance Considerations
When choosing between ODBC and OLE DB, consider the specific requirements of your application. ODBC is generally preferred for cross-platform applications, while OLE DB may offer better performance in certain scenarios.
Advanced Features of SQL Server Native Client
SQL Server Native Client boasts advanced features that enhance data access and application performance.
Support for New SQL Server Data Types
The client supports various new SQL Server data types, including spatial data, XML, and table-valued parameters. Here’s an example of how to use table-valued parameters:
CREATE TYPE MyTableType AS TABLE (ID INT, Name NVARCHAR(50));
Connection Pooling
Connection pooling is implemented for efficient resource management. This feature reduces connection overhead, allowing applications to reuse existing connections.
Multiple Active Result Sets (MARS)
MARS allows multiple requests on a single connection. Utilize this feature for concurrent data processing scenarios:
-- Enable MARS in the connection string
ConnectionString="Server=myServerAddress;Database=myDataBase;User Id=myUsername;Password=myPassword;MultipleActiveResultSets=True;"
Security Enhancements
SQL Server Native Client incorporates security enhancements, including support for encryption protocols and secure connections. To establish a secure connection, you can configure your connection string as follows:
Encrypt=True;TrustServerCertificate=False;
Distributed Transactions
Utilize SQL Server Native Client for distributed transactions and high-availability configurations, which are critical for enterprise-level applications.
Integrating SQL Server Native Client with Chat2DB
Integrating SQL Server Native Client with Chat2DB (opens in a new tab) enhances the application's database connectivity. Chat2DB is an AI-powered database visualization management tool that simplifies database operations and improves efficiency.
Benefits of Using SQL Server Native Client with Chat2DB
Using SQL Server Native Client within Chat2DB provides users with improved data access speed and reliability. Here’s a step-by-step guide to configuring Chat2DB:
- Open Chat2DB and navigate to the settings menu.
- Select "Database Connections" and choose SQL Server Native Client from the list.
- Enter the required connection details, including server address, database name, and credentials.
Optimizations and Features
Chat2DB leverages SQL Server Native Client’s capabilities to optimize database interactions, including intelligent query suggestions and natural language processing for SQL generation. These AI features allow users to generate SQL queries effortlessly and visualize data intuitively.
Challenges and Solutions
While integrating SQL Server Native Client with Chat2DB, users may encounter issues related to connection settings. Ensure that the connection strings are correctly configured and that the necessary drivers are installed.
Troubleshooting and Optimization Techniques
Effective troubleshooting and optimization techniques are vital when using SQL Server Native Client. Below are some common issues and their solutions:
Common Connectivity Problems
- Timeouts: Increase the connection timeout in the connection string.
- Failed Connections: Verify the server address and authentication credentials.
Diagnostic Tools
Utilize tools like SQL Server Profiler (opens in a new tab) to monitor and analyze performance.
Optimization Techniques
- Query Tuning: Analyze execution plans and optimize queries.
- Index Management: Ensure that indexes are created and maintained effectively.
- Connection Pooling: Monitor and adjust settings for optimal performance.
Future Trends and Developments
The future of SQL Server Native Client is promising, with anticipated developments in response to emerging database technologies and cloud-based solutions.
Advances in Machine Learning and AI
As machine learning and AI continue to evolve, SQL Server Native Client is expected to integrate more seamlessly with data-driven applications, enhancing data manipulation and analysis capabilities.
Commitment to Evolution
Microsoft is committed to evolving SQL Server Native Client to meet the changing needs of developers and organizations. Staying updated with the latest developments through official channels (opens in a new tab) and community forums is essential.
FAQ
-
What is SQL Server Native Client?
SQL Server Native Client is a data access technology that combines OLE DB and ODBC to facilitate connections to SQL Server databases. -
How can I install SQL Server Native Client?
You can download it from the Microsoft Download Center and follow the installation prompts. -
What are the benefits of using SQL Server Native Client with Chat2DB?
It improves data access speed and reliability, along with providing AI-driven features for database management. -
What troubleshooting steps should I take for connection issues?
Verify your connection string, check server address and credentials, and increase the connection timeout if necessary. -
Why should I choose Chat2DB over other database management tools?
Chat2DB offers unique AI capabilities that enhance the database management experience, making it easier to generate queries and analyze data visually.
For a more intuitive and efficient database management experience, consider using Chat2DB (opens in a new tab). Its AI capabilities can significantly streamline your workflows and improve productivity, making it a superior choice over other tools like DBeaver, MySQL Workbench, or DataGrip.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!