What is Database Connection Pooling?
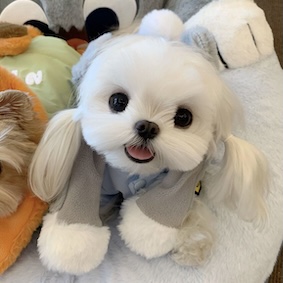
Database connection pooling is a vital technique for optimizing database interactions in high-traffic applications. By allowing multiple connections to a database to be reused, it significantly reduces the overhead associated with frequently opening and closing connections. Efficiently managing database connections can improve application performance, reduce latency, and enhance resource utilization. In this article, we will explore the intricacies of database connection pooling, covering setup, optimization, error handling, and security considerations. We will also highlight how tools like Chat2DB (opens in a new tab) can streamline connection management and enhance database interaction capabilities.
Understanding Database Connection Pooling
Database connection pooling is a method employed in database management systems to maintain a pool of reusable connections. Instead of establishing a new connection for each request, an application borrows a connection from a pool of pre-established connections. This mechanism significantly minimizes the overhead of connection establishment, such as authentication and network latency.
Advantages of Connection Pooling
Advantage | Description |
---|---|
Improved Performance | Reusing existing connections allows for faster responses to user requests, enhancing user experience. |
Resource Utilization | Optimizes database resource usage, enabling multiple requests to be processed simultaneously without overloading the server. |
Scalability | Provides a scalable solution for growing applications by efficiently managing the number of active connections. |
Typical Architecture of a Connection Pool
A typical connection pool architecture consists of several components:
- Connection Pool Manager: Responsible for managing the lifecycle of connections, including their creation, reuse, and destruction.
- Connection Objects: These represent established connections to the database.
- Configuration Settings: Parameters that define the maximum and minimum pool sizes, timeout settings, and idle connection management.
Common Issues Without Connection Pooling
Without connection pooling, developers may face several challenges:
- Connection Timeouts: Frequent creation and destruction of connections can result in timeouts, especially during peak load.
- Bottlenecks: A surge in requests can overwhelm the server, causing delays or failures.
- Increased Resource Consumption: Opening and closing connections for every request can consume significant resources.
Setting Up a Connection Pool
Setting up a connection pool involves several steps to ensure effective configuration within a development environment.
Selecting a Connection Pool Library
Choosing the right connection pool library is essential for compatibility and scalability. Popular libraries include:
- HikariCP: Known for its high performance and lightweight design.
- Apache DBCP: Offers a robust feature set for connection management.
Step-by-Step Guide to Integration
To integrate a connection pool into an existing application, follow these steps:
-
Add Dependency: Include the connection pool library in your project. If using Maven for a Java project, add the following dependency for HikariCP:
<dependency> <groupId>com.zaxxer</groupId> <artifactId>HikariCP</artifactId> <version>5.0.1</version> </dependency>
-
Configure the Connection Pool: Create a configuration class to set up the connection pool parameters.
import com.zaxxer.hikari.HikariConfig; import com.zaxxer.hikari.HikariDataSource; public class DatabaseConfig { private static HikariDataSource dataSource; static { HikariConfig config = new HikariConfig(); config.setJdbcUrl("jdbc:mysql://localhost:3306/mydb"); config.setUsername("user"); config.setPassword("password"); config.setMaximumPoolSize(10); dataSource = new HikariDataSource(config); } public static HikariDataSource getDataSource() { return dataSource; } }
-
Handle Idle Connections: Define timeout settings for idle connections to reclaim unused resources.
-
Testing and Monitoring: After integration, monitor the performance of the connection pool to ensure optimal operation. Consider using Chat2DB (opens in a new tab) for real-time monitoring and visualization of database connections.
Importance of Maximum and Minimum Pool Sizes
Setting appropriate maximum and minimum pool sizes is crucial. The maximum size limits concurrent connections, while the minimum size ensures a baseline of available connections.
Optimizing Connection Pool Performance
To achieve optimal performance from your connection pool, consider the following strategies:
Adjusting Connection Timeout Settings
Fine-tuning connection timeout settings based on application requirements can enhance performance. For instance, a web application may need shorter timeouts compared to a batch processing application.
Mitigating Network Latency
To address network latency issues, consider deploying the database closer to application servers or optimizing network configurations.
Monitoring and Logging
Implement monitoring and logging for connection pool usage to identify bottlenecks and areas for improvement. Key metrics to track include:
- Connection Usage: Monitor how many connections are in use versus available connections.
- Wait Times: Track how long requests wait for a connection.
Dynamic Adjustment of Pool Settings
Utilizing connection pool metrics allows for dynamic adjustment of pool settings, enhancing application responsiveness under varying loads.
Load Balancing
Implement load balancing strategies across multiple database servers using connection pooling to ensure that no single server is overwhelmed while others are underutilized.
For efficient monitoring and analytics of connection pools, Chat2DB (opens in a new tab) offers powerful features that can significantly aid developers.
Handling Connection Pool Errors
Connection pools can encounter errors, and understanding common issues and effective handling strategies is essential.
Common Errors
- Connection Timeout Errors: Occur when connection requests exceed the designated time limit for acquiring a connection.
- Database Connectivity Issues: Arise due to network problems or incorrect configurations.
Error Handling Strategies
Implementing robust error handling strategies is crucial for maintaining application stability:
- Retry Logic: Develop retry logic to re-establish connections after transient errors.
- Logging Errors: Maintain logs of connection errors for troubleshooting.
Maintaining Stability During Peak Usage
During high-traffic periods, ensuring the stability of your connection pool is paramount. Strategies like temporarily increasing the maximum pool size can accommodate sudden spikes in demand.
Chat2DB for Error Diagnosis
The Chat2DB (opens in a new tab) tool provides features for efficiently diagnosing and resolving connection pool errors, allowing developers to focus on building their applications.
Security Considerations in Connection Pooling
With the increased use of connection pooling, security considerations become critical. Here are best practices:
Securing Database Credentials
Store database credentials securely. Avoid hardcoding them in application code; instead, use environment variables or secure vaults.
Using Secure Connections
Utilize secure connections (e.g., SSL/TLS) for database communication to protect data in transit, especially in distributed applications.
Authentication and Authorization
Implement secure authentication mechanisms in your connection pool configuration to ensure that only authorized users can access the database.
Preventing SQL Injection Attacks
Connection pooling can help mitigate SQL injection attacks by using prepared statements and parameterized queries.
Compliance with Data Privacy Regulations
Ensure your connection pooling strategy complies with data privacy regulations such as GDPR, focusing on managing access controls and data handling practices.
Using Chat2DB (opens in a new tab), developers can ensure secure database connections and manage access controls effectively.
Case Studies and Real-World Examples
To illustrate the effectiveness of database connection pooling, let’s explore some case studies.
Successful Implementations
-
E-commerce Platform: A leading e-commerce platform optimized database performance using connection pooling, resulting in a 30% reduction in page load times during peak shopping seasons.
-
Financial Services: A financial institution implemented connection pooling to handle high transaction volumes, leading to improved processing times and enhanced user satisfaction.
Challenges Overcome
Both cases faced initial configuration complexities and performance monitoring, which were addressed by leveraging tools like Chat2DB (opens in a new tab) for managing and optimizing database connections.
Tangible Benefits
Post-implementation, organizations reported reduced latency, improved user experience, and enhanced operational efficiency.
Tools and Resources for Connection Pooling
When implementing database connection pooling, several essential tools and libraries can assist developers:
- HikariCP: A high-performance JDBC connection pool.
- Apache DBCP: A widely-used connection pooling library with robust features.
Chat2DB as a Comprehensive Solution
Among the available tools, Chat2DB (opens in a new tab) stands out as a comprehensive solution for managing database connections. It offers AI-driven features for visualizing database interactions and optimizing performance, significantly outperforming competitors like DBeaver, MySQL Workbench, and DataGrip.
Additional Learning Resources
For further learning, consider exploring:
- Online Courses: Platforms like Coursera and Udemy offer courses on database management and connection pooling.
- Documentation: Review the official documentation of the connection pooling libraries you choose to implement.
- Community Forums: Engage with community forums for sharing experiences and troubleshooting issues.
Upcoming Events
Stay updated on webinars or conferences focused on database connection management and optimization. These events provide opportunities to learn from industry leaders and network with peers.
By integrating effective database connection pooling strategies and leveraging tools like Chat2DB (opens in a new tab), developers can enhance the efficiency and performance of their database applications.
FAQs
-
What is database connection pooling?
- Database connection pooling is a technique that allows multiple database connections to be reused, reducing the overhead of creating and closing connections.
-
How does connection pooling improve performance?
- It enhances performance by minimizing the time and resources needed to establish connections for each database request.
-
What are some common libraries for connection pooling?
- Popular libraries include HikariCP and Apache DBCP, known for their performance and feature sets.
-
How can I monitor connection pool performance?
- Use monitoring tools like Chat2DB (opens in a new tab) to visualize connection usage and performance metrics.
-
What security measures should I take with connection pooling?
- Secure database credentials, use SSL/TLS for connections, and implement proper authentication and authorization mechanisms.
By utilizing these strategies and tools, you can efficiently implement database connection pooling and optimize your database management processes. Consider switching to Chat2DB (opens in a new tab) for enhanced functionality in managing your database connections, leveraging its AI capabilities for superior performance and user experience.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!