How to Implement Auto Increment in PostgreSQL: A Step-by-Step Guide
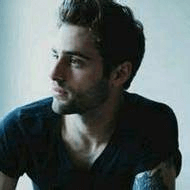
Understanding Auto Increment in PostgreSQL
In PostgreSQL, the concept of auto increment is essential for automating the generation of unique values in tables. Unlike some other database systems, such as MySQL, which features a straightforward AUTO_INCREMENT
attribute, PostgreSQL employs a different mechanism. It utilizes sequences, which are special database objects designed to generate a sequence of unique numbers. This system is integral to maintaining data integrity and facilitating efficient database operations, particularly when managing primary keys.
Importance of Auto Increment
The significance of auto increment lies in its role in simplifying the assignment of unique identifiers to rows in a table. In relational databases, primary keys are essential for uniquely identifying records, and the auto increment functionality streamlines this process. By automatically generating these values, PostgreSQL reduces the likelihood of manual data entry errors, ensuring consistency and reliability across the dataset.
It's also important to distinguish between surrogate keys and natural keys. Surrogate keys are unique identifiers created by the database (like auto incremented values), while natural keys are derived from the data itself. Using auto incremented values as surrogate keys can lead to a more efficient and straightforward database design.
Creating a Sequence in PostgreSQL for Auto Increment
To implement auto increment in PostgreSQL, the first step is to create a sequence. This can be accomplished using the CREATE SEQUENCE
command. Below is a step-by-step guide to creating a sequence:
CREATE SEQUENCE my_sequence
INCREMENT BY 1
START WITH 1
MINVALUE 1
MAXVALUE 10000
CACHE 1;
Sequence Parameters Explained
Parameter | Description |
---|---|
INCREMENT BY | Determines how much the sequence will increase with each call (set to 1). |
START WITH | Defines the initial value of the sequence (starts at 1). |
MINVALUE | Sets the minimum value the sequence can produce (1). |
MAXVALUE | Sets the maximum value the sequence can produce (10000). |
CACHE | Improves performance by preallocating a set number of sequence values. |
Best practices suggest naming sequences clearly to maintain organization in your database management tasks.
Creating Sequences for Different Use Cases
Here's another example to illustrate creating a sequence tailored for a specific use case:
CREATE SEQUENCE order_id_seq
INCREMENT BY 1
START WITH 1000
MINVALUE 1000
MAXVALUE 9999
CACHE 10;
In this example, the sequence is designed specifically for an order ID system. It starts at 1000 and can generate values up to 9999, suitable for many e-commerce platforms.
Using a Sequence in a Table
Once a sequence is created, the next step is to integrate it into a table to enable auto increment functionality. The DEFAULT NEXTVAL
expression is used to automatically assign sequence values to a column in a table.
Here’s an example of how to alter a table to use a sequence for its primary key:
CREATE TABLE orders (
order_id INT DEFAULT nextval('order_id_seq') PRIMARY KEY,
order_date DATE NOT NULL,
customer_id INT NOT NULL
);
Data Types: SERIAL and BIGSERIAL
In PostgreSQL, you can also use the SERIAL
and BIGSERIAL
data types as shorthand for creating integer columns with auto increment capability. This is particularly useful when defining primary keys:
CREATE TABLE customers (
customer_id SERIAL PRIMARY KEY,
customer_name VARCHAR(100) NOT NULL
);
The SERIAL
type automatically creates a sequence for the column, simplifying the implementation process.
Managing Sequences in PostgreSQL
Effective management of sequences is essential to maintain the integrity of your database. Various operations can be performed to manage sequences, including resetting their values and altering properties.
Resetting a Sequence
To reset a sequence's value, you can use the ALTER SEQUENCE
command:
ALTER SEQUENCE order_id_seq RESTART WITH 1000;
Importance of Sequence Maintenance
Maintaining sequences is crucial to avoid conflicts or gaps in value assignments. For instance, if records are deleted from a table, the corresponding sequence values may need to be adjusted to prevent gaps.
Dropping a Sequence
If a sequence is no longer needed, it can be removed using the DROP SEQUENCE
command:
DROP SEQUENCE order_id_seq;
When dropping a sequence, consider any dependent objects that may rely on it.
Using currval and setval
PostgreSQL provides functions such as currval
and setval
to manage sequences actively. Below are examples of how to use these functions:
SELECT currval('order_id_seq'); -- Get the current value of the sequence
SELECT setval('order_id_seq', 5000); -- Set the sequence to a specific value
Advanced Auto Increment Techniques
For more complex requirements, advanced techniques can be employed to implement auto increment functionality in PostgreSQL. This includes using custom functions and triggers to create sophisticated auto increment logic.
Conditional Auto Increment
You can implement conditional auto increment based on specific business logic or data state. For instance, you might want to increment the order ID only under certain conditions:
CREATE OR REPLACE FUNCTION conditional_increment() RETURNS TRIGGER AS $$
BEGIN
IF NEW.customer_id IS NOT NULL THEN
NEW.order_id := nextval('order_id_seq');
END IF;
RETURN NEW;
END;
$$ LANGUAGE plpgsql;
CREATE TRIGGER trigger_conditional_increment
BEFORE INSERT ON orders
FOR EACH ROW EXECUTE FUNCTION conditional_increment();
Integration with Partitioned Tables
Auto increment can also be integrated with partitioned tables to handle large datasets efficiently. This allows you to distribute data across multiple tables while maintaining unique identifiers.
Common Challenges and Solutions
While implementing auto increment in PostgreSQL, developers may encounter several challenges. Below are some common issues and their solutions:
Sequence Value Exhaustion
One of the primary challenges is the exhaustion of sequence values. This can occur if the maximum value is reached. To mitigate this risk, ensure that your sequences have appropriate maximum values set, or consider using BIGSERIAL
for larger datasets.
Reseeding Sequences
If sequence values need to be reseeded or reset, use the ALTER SEQUENCE
command strategically. Regular monitoring of sequences can help identify when reseeding is necessary.
Database Monitoring Tools
Utilizing database monitoring tools like Chat2DB (opens in a new tab) can significantly aid in identifying and resolving sequence-related issues promptly. Chat2DB's AI capabilities enhance your ability to manage sequences effectively, offering insights into performance bottlenecks and potential conflicts.
Real-World Applications of Auto Increment in PostgreSQL
Auto increment functionality is widely applied across various industries. Here are some case studies highlighting its effectiveness:
E-commerce Platforms
In e-commerce platforms, auto increment is utilized for order tracking and inventory management. Each order is assigned a unique order ID, facilitating seamless operations and record-keeping.
Customer Relationship Management (CRM)
In CRM systems, auto increment helps uniquely identify customer records, ensuring that each entry is distinct and easily retrievable.
Financial Systems
Financial databases benefit from auto increment in tracking transactions and maintaining audit trails. Each transaction can be assigned a unique identifier, enhancing oversight and compliance.
Healthcare Databases
In healthcare, auto increment ensures accurate patient record management. Each patient is given a unique identifier, which is critical for maintaining medical history and treatment records.
PostgreSQL's sequence-based auto increment system provides the flexibility and scalability needed to support diverse business needs.
Frequently Asked Questions
-
What is the main difference between auto increment in PostgreSQL and MySQL?
- PostgreSQL uses sequences for auto increment functionality, while MySQL has a built-in
AUTO_INCREMENT
attribute.
- PostgreSQL uses sequences for auto increment functionality, while MySQL has a built-in
-
Can I reset a sequence after it has been used?
- Yes, you can reset a sequence using the
ALTER SEQUENCE RESTART
command.
- Yes, you can reset a sequence using the
-
How do I create a sequence for a primary key?
- You can create a sequence and use the
DEFAULT NEXTVAL
expression when defining the primary key column in your table.
- You can create a sequence and use the
-
Are there performance implications when using sequences?
- Generally, sequences are efficient, but performance can vary based on how they are managed. Monitoring tools like Chat2DB (opens in a new tab) can help optimize performance.
-
What should I do if my sequence values are exhausted?
- Ensure that your sequence has an adequate maximum value or consider using larger data types like
BIGSERIAL
.
- Ensure that your sequence has an adequate maximum value or consider using larger data types like
By leveraging the features of Chat2DB, you can enhance your PostgreSQL management experience with its intelligent automation and user-friendly interface, making it a superior choice compared to traditional tools like DBeaver, MySQL Workbench, or DataGrip.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!