Essential PostgreSQL Cheat Sheet: Key Commands and Tips for Beginners
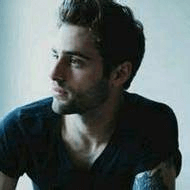
Understanding PostgreSQL
PostgreSQL is an advanced open-source relational database management system (RDBMS) that has gained immense popularity among developers due to its robustness and extensive feature set. Initially developed in the 1980s at the University of California, Berkeley, PostgreSQL has evolved significantly over the years, becoming a preferred choice for modern application development.
One of the key features that sets PostgreSQL apart is its compliance with SQL standards, making it a versatile tool that supports various programming languages and platforms. It allows developers to interact with databases seamlessly, which enhances productivity. PostgreSQL is licensed under the PostgreSQL License, which is similar to the MIT License, promoting wide use and collaboration within the community.
The PostgreSQL community is active and continuously contributes to the platform's growth, ensuring that it remains reliable and secure with regular updates. Developers often prefer PostgreSQL over other database systems due to its strong focus on standards compliance, extensibility, and the ability to handle complex queries effectively.
Setting Up Your Environment
Setting up PostgreSQL is an essential step for any developer looking to work with this powerful database system. The installation process varies slightly depending on the operating system, whether it's Windows, macOS, or Linux.
Installing PostgreSQL on Windows
- Download the Installer: Visit the official PostgreSQL website (opens in a new tab) and download the installer.
- Run the Setup: Execute the downloaded file and follow the prompts to install PostgreSQL.
- Configure the Superuser: During installation, you will be prompted to set up a superuser account. Choose a strong password for the
postgres
user. - Adjust Firewall Settings: Ensure that PostgreSQL can communicate through your firewall.
Installing PostgreSQL on macOS
- Using Homebrew: If you have Homebrew installed, you can easily run the following command in your terminal:
brew install postgresql
- Start the Service: Once installed, start the PostgreSQL service:
brew services start postgresql
Installing PostgreSQL on Linux
For Linux users, you can install PostgreSQL using the package manager. For example, on Ubuntu, you can run:
sudo apt update
sudo apt install postgresql postgresql-contrib
Initial Configuration
After installation, you need to configure PostgreSQL to ensure it is secure and operates correctly.
-
Accessing PostgreSQL: You can access the PostgreSQL prompt using:
sudo -u postgres psql
-
Creating a New Database: To create a new database, use the command:
CREATE DATABASE mydatabase;
-
Configuring Access Permissions: Modify the
pg_hba.conf
file to set user access permissions.
Using Docker for PostgreSQL
For development and testing, using Docker can simplify the setup process. You can quickly create an isolated PostgreSQL environment with the following command:
docker run --name postgres-container -e POSTGRES_PASSWORD=mysecretpassword -d postgres
For managing your database connections and simplifying interactions, consider using Chat2DB (opens in a new tab). This AI-powered database visualization tool enhances your PostgreSQL experience by streamlining database management tasks.
Basic SQL Commands
Once PostgreSQL is set up, you can start interacting with your databases using SQL commands. Here are some fundamental SQL commands essential for beginners:
Command Type | SQL Command Example |
---|---|
Create Database | CREATE DATABASE mydatabase; |
Create Table | CREATE TABLE employees (id SERIAL PRIMARY KEY, name VARCHAR(100), age INT, department VARCHAR(50)); |
Insert Data | INSERT INTO employees (name, age, department) VALUES ('Alice', 30, 'HR'), ('Bob', 25, 'Engineering'); |
Select Data | SELECT * FROM employees; |
Update Data | UPDATE employees SET age = 31 WHERE name = 'Alice'; |
Delete Data | DELETE FROM employees WHERE name = 'Bob'; |
Filter Data | SELECT * FROM employees WHERE age > 28 ORDER BY name ASC; |
Join Tables | SELECT employees.name, departments.department_name FROM employees JOIN departments ON employees.department = departments.department_name; |
Aggregate Functions | SELECT department, COUNT(*) AS employee_count FROM employees GROUP BY department; |
Advanced SQL Techniques
As you gain more experience, you'll encounter advanced SQL techniques that greatly enhance data manipulation and analysis in PostgreSQL.
Subqueries
Subqueries are nested queries that allow you to perform complex operations. For instance:
SELECT name FROM employees WHERE age > (SELECT AVG(age) FROM employees);
Transactions
Understanding transactions and ACID properties is crucial for ensuring data integrity. A transaction can be initiated using:
BEGIN;
-- Your SQL commands
COMMIT; -- or ROLLBACK;
Indexes
Indexes are essential for speeding up query performance. You can create an index on a column with:
CREATE INDEX idx_department ON employees(department);
Views
Creating views simplifies complex queries and enhances security:
CREATE VIEW employee_view AS
SELECT name, age FROM employees WHERE age > 25;
Stored Procedures and Functions
Stored procedures and functions allow you to encapsulate complex operations. For example:
CREATE FUNCTION get_employee_count() RETURNS INT AS $$
BEGIN
RETURN (SELECT COUNT(*) FROM employees);
END;
$$ LANGUAGE plpgsql;
Triggers
Triggers automate tasks based on specific events within the database:
CREATE TRIGGER update_age
AFTER UPDATE ON employees
FOR EACH ROW EXECUTE FUNCTION log_age_change();
Window Functions
Window functions enable advanced analytical queries:
SELECT name, age, AVG(age) OVER (PARTITION BY department) AS avg_age
FROM employees;
PostgreSQL Administration
Managing PostgreSQL databases involves several essential administrative tasks to ensure smooth operation.
Backups and Restores
Regular backups are crucial for data safety. Use pg_dump
to create a backup:
pg_dump mydatabase > mydatabase_backup.sql
Restore the database using:
psql mydatabase < mydatabase_backup.sql
Monitoring and Optimization
Monitoring your database's performance is vital. Tools like pgAdmin
and Chat2DB (opens in a new tab) can help in optimizing database management. Chat2DB’s AI capabilities provide insights into performance bottlenecks and suggest improvements.
User Management
Managing user roles is crucial for security. Create a new role with:
CREATE ROLE new_user WITH LOGIN PASSWORD 'securepassword';
Maintenance Tasks
Regular maintenance tasks like vacuuming and reindexing help keep your database healthy:
VACUUM; -- Cleans up dead tuples
REINDEX TABLE employees; -- Rebuilds indexes
Replication and High Availability
Setting up replication and high availability ensures your database is reliable. Consider using streaming replication for this purpose.
Partitioning
Partitioning helps manage large datasets efficiently. You can partition a table like this:
CREATE TABLE sales (
id SERIAL PRIMARY KEY,
sale_date DATE
) PARTITION BY RANGE (sale_date);
Tips for Optimizing PostgreSQL Performance
Optimizing PostgreSQL performance is key to enhancing application efficiency. Here are some practical tips:
Query Optimization Techniques
Analyzing query plans can help identify bottlenecks:
EXPLAIN SELECT * FROM employees WHERE age > 30;
Data Types
Choosing appropriate data types can improve storage efficiency and performance.
Connection Pooling
Connection pooling minimizes the overhead of establishing connections, which is crucial for high-traffic applications.
Hardware Considerations
Allocating sufficient memory and CPU resources can greatly enhance performance.
Caching Mechanisms
Implement caching to reduce load on your database.
Identifying Slow Queries
Use pg_stat_statements
to identify and optimize slow-performing queries.
Tuning Configuration Settings
Adjusting PostgreSQL configuration settings can lead to significant performance improvements.
Learning Resources and Community Support
To further enhance your PostgreSQL knowledge, consider the following resources:
- Official PostgreSQL Documentation: A comprehensive resource for users at any level.
- Online Courses: Platforms like Coursera, Udemy, and Khan Academy offer valuable courses on PostgreSQL.
- Books: "PostgreSQL: Up and Running" provides deep insights into PostgreSQL.
- Community Forums: Engage with peers on platforms like Stack Overflow and Reddit.
- Conferences and Meetups: Participate in PostgreSQL conferences for networking and learning opportunities.
Additionally, Chat2DB (opens in a new tab) offers community support and resources, making it easier to learn and manage PostgreSQL databases.
FAQ
1. What is PostgreSQL?
PostgreSQL is an advanced open-source relational database management system known for its robustness and support for complex queries.
2. How do I install PostgreSQL on my computer?
You can install PostgreSQL on various operating systems using installers or package managers. Detailed steps are available in the installation section above.
3. What are some basic SQL commands I should know?
Key commands include CREATE
, INSERT
, SELECT
, UPDATE
, and DELETE
. Familiarity with these commands is essential for interacting with PostgreSQL.
4. How can I optimize PostgreSQL performance?
Optimizing performance can be achieved through query optimization, indexing, connection pooling, and tuning configuration settings.
5. What is Chat2DB?
Chat2DB (opens in a new tab) is an AI-driven database visualization management tool that simplifies database management tasks and enhances productivity for developers and data analysts, offering features such as natural language query generation and intelligent suggestions for query optimization.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!