A Comprehensive Guide to PostgreSQL Arrays
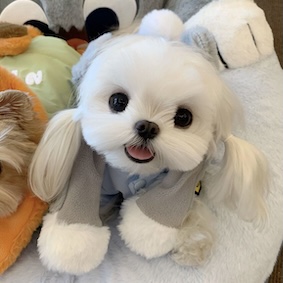
Arrays in PostgreSQL are a powerful feature that allows users to store multiple values within a single field, making them particularly useful for complex data structures like JSON. This article will explore how to effectively utilize arrays in PostgreSQL, covering topics such as creating and manipulating arrays, querying arrays, advanced array functions, performance considerations, and specifically working with JSON arrays. We will also highlight how tools like Chat2DB (opens in a new tab) can enhance your experience with PostgreSQL arrays by integrating AI functionalities for easier database management.
Understanding the Importance of Arrays in PostgreSQL
In PostgreSQL, arrays serve as a data type that allows users to store a list of values in a single column, differentiating them from arrays in many other programming languages where arrays usually represent a fixed-size collection of elements. PostgreSQL arrays can hold any data type, including integers, text, and even other arrays, which provides significant flexibility in data modeling.
One of the primary benefits of using arrays is their ability to reduce the need for multiple rows in a table to represent related data. For example, if you wanted to store a user's favorite colors, instead of creating a separate row for each color, you can store all colors in a single array field. This can simplify data retrieval and manipulation.
Creating Arrays in PostgreSQL
Creating arrays in PostgreSQL can be accomplished using the ARRAY
constructor or the array_agg
function. Below are examples of both methods:
-- Using the ARRAY constructor
SELECT ARRAY[1, 2, 3, 4, 5] AS int_array;
-- Using array_agg to create an array from a set of values
SELECT array_agg(color) AS color_array
FROM colors_table;
When inserting array data into tables, you can directly assign the array to a column defined with the array type:
CREATE TABLE users (
id SERIAL PRIMARY KEY,
name TEXT,
favorite_colors TEXT[]
);
INSERT INTO users (name, favorite_colors)
VALUES ('Alice', ARRAY['red', 'green', 'blue']);
Understanding array dimensions is crucial, as they can be single-dimensional or multi-dimensional. For example, a two-dimensional array can store a matrix of values:
CREATE TABLE matrix_example (
id SERIAL PRIMARY KEY,
matrix INTEGER[][]
);
INSERT INTO matrix_example (matrix)
VALUES (ARRAY[[1, 2, 3], [4, 5, 6]]);
Manipulating Arrays in PostgreSQL
Manipulating arrays in PostgreSQL involves several array-specific SQL functions. Key functions include:
array_append
: Attaches an element to the end of an array.array_remove
: Removes all occurrences of a specified element from an array.array_cat
: Concatenates two arrays.
Here are examples demonstrating these functions:
-- Appending an element
UPDATE users
SET favorite_colors = array_append(favorite_colors, 'yellow')
WHERE name = 'Alice';
-- Removing an element
UPDATE users
SET favorite_colors = array_remove(favorite_colors, 'green')
WHERE name = 'Alice';
-- Concatenating two arrays
SELECT array_cat(favorite_colors, ARRAY['purple', 'orange'])
FROM users
WHERE name = 'Alice';
Querying Arrays in PostgreSQL
Effective querying of arrays requires an understanding of various functions available in PostgreSQL. One powerful function is unnest
, which expands array elements into individual rows:
SELECT unnest(favorite_colors) AS color
FROM users
WHERE name = 'Alice';
You can also filter array data using the ANY
and ALL
operators:
SELECT *
FROM users
WHERE 'red' = ANY(favorite_colors);
A unique aspect of PostgreSQL is its ability to handle JSON arrays. To extract elements from JSON arrays, you can use the ->
and ->>
operators. For example:
SELECT jsonb_array_elements(favorite_colors_json) AS color
FROM users_json
WHERE name = 'Alice';
Advanced Array Functions and Operations
PostgreSQL provides advanced capabilities for working with arrays, such as array slicing and element searching using the array_position
function:
-- Finding the position of an element
SELECT array_position(favorite_colors, 'red') AS position
FROM users
WHERE name = 'Alice';
-- Slicing an array
SELECT favorite_colors[1:2] AS sliced_colors
FROM users
WHERE name = 'Alice';
Using set-returning functions can also produce complex query results involving arrays. For instance, you can use generate_series
to create a series of numbers and then work with arrays:
SELECT generate_series(1, 5) AS numbers,
ARRAY[1, 2, 3, 4, 5] AS num_array;
Handling nested arrays requires understanding how to access elements effectively:
CREATE TABLE nested_example (
id SERIAL PRIMARY KEY,
nested_array INTEGER[][]
);
INSERT INTO nested_example (nested_array)
VALUES (ARRAY[[ARRAY[1, 2], ARRAY[3, 4]], [ARRAY[5, 6], ARRAY[7, 8]]]);
-- Accessing an element in a nested array
SELECT nested_array[1][1][2] AS value
FROM nested_example;
Performance Considerations with Arrays
While arrays provide flexibility, there are performance considerations to keep in mind. Using arrays can lead to increased complexity in indexing and querying. The choice between arrays and other data types, such as JSONB (opens in a new tab), depends on the specific use case.
Best practices for optimizing performance include:
- Indexing Arrays: Use GIN (Generalized Inverted Index) for indexing array columns to speed up searches.
- Avoiding Overuse: In scenarios where relationships among entities are complex, consider using separate tables instead of arrays.
CREATE INDEX idx_users_favorite_colors ON users USING GIN (favorite_colors);
Working with JSON Arrays in PostgreSQL
Handling JSON arrays is an essential skill when working with modern databases. PostgreSQL supports two JSON data types: JSON
and JSONB
, with JSONB
offering better performance and indexing features.
To extract elements from JSON arrays, you can utilize functions like jsonb_array_elements
and jsonb_array_length
:
SELECT jsonb_array_length(favorite_colors_json) AS color_count
FROM users_json
WHERE name = 'Alice';
SELECT jsonb_array_elements(favorite_colors_json) AS color
FROM users_json
WHERE name = 'Alice';
Utilizing Chat2DB (opens in a new tab) can significantly simplify the management of JSON data and arrays, providing an intuitive interface and AI-powered features to generate SQL queries and visualizations effortlessly.
Real-World Use Cases and Applications of PostgreSQL Arrays
Arrays in PostgreSQL can be applied in numerous real-world scenarios, especially in web development. For instance, storing user preferences or product configurations can be effectively managed using arrays.
In data analytics, arrays facilitate complex data aggregation and transformation, enabling more insightful reporting and analysis. The ability to store and manipulate multiple values in a single field makes it easier to analyze relationships within the data.
Here is a comparison table demonstrating the benefits of using arrays versus traditional relational structures:
Feature | Arrays | Traditional Tables |
---|---|---|
Data Storage | Multiple values in one column | Each value in a separate row |
Query Complexity | Simpler data retrieval | More complex JOIN operations |
Space Efficiency | Reduces table rows | Requires more rows for related data |
Flexibility | Can hold any data type | Fixed schema structure |
For instance, in an e-commerce application, you could store a list of product tags in an array:
CREATE TABLE products (
id SERIAL PRIMARY KEY,
name TEXT,
tags TEXT[]
);
INSERT INTO products (name, tags)
VALUES ('Product 1', ARRAY['electronics', 'sale', 'new']);
The use of Chat2DB (opens in a new tab) can streamline the integration and manipulation of array data, allowing developers and data analysts to focus on insights rather than the complexities of SQL syntax. With AI-driven features such as natural language processing for generating SQL queries and visualizations, Chat2DB stands out from other tools like DBeaver, MySQL Workbench, and DataGrip, making it a superior choice for PostgreSQL users.
FAQs
-
What are arrays in PostgreSQL? Arrays are a data type in PostgreSQL that allows you to store multiple values in a single column.
-
How can I create an array in PostgreSQL? You can create arrays using the
ARRAY
constructor or thearray_agg
function. -
What functions can I use to manipulate arrays? Functions like
array_append
,array_remove
, andarray_cat
are commonly used for manipulating arrays. -
Can I work with JSON arrays in PostgreSQL? Yes, PostgreSQL has built-in functions to handle JSON arrays effectively.
-
How can Chat2DB help with PostgreSQL arrays? Chat2DB provides an AI-powered interface that simplifies database management, allowing users to generate queries and visualizations with ease.
By leveraging the capabilities of arrays in PostgreSQL and utilizing tools like Chat2DB (opens in a new tab), you can significantly enhance your database management experience and streamline your data operations.
Get Started with Chat2DB Pro
If you're looking for an intuitive, powerful, and AI-driven database management tool, give Chat2DB a try! Whether you're a database administrator, developer, or data analyst, Chat2DB simplifies your work with the power of AI.
Enjoy a 30-day free trial of Chat2DB Pro. Experience all the premium features without any commitment, and see how Chat2DB can revolutionize the way you manage and interact with your databases.
👉 Start your free trial today (opens in a new tab) and take your database operations to the next level!